Hi, Habr! I want to tell by examples about the simplest way of creating something complicated. The essence of the terrible word "prototyping" is reduced to the use of analogies or templates in the project Arduino.
I don’t want to frighten the novice users of Python-Arduino with long words, so let's go straight to the examples.
Buzzer - generates an audible alarm
Zoomer [1]. produces sound when supplied with a HIGH digital value (i.e., +5 V), which can be provided using Arduino digital pins [2].
')
However, instead of performing simple digital output, as was done with a motion sensor, we implement Python programming tricks to generate various sound patterns and create various sound effects.
Connections
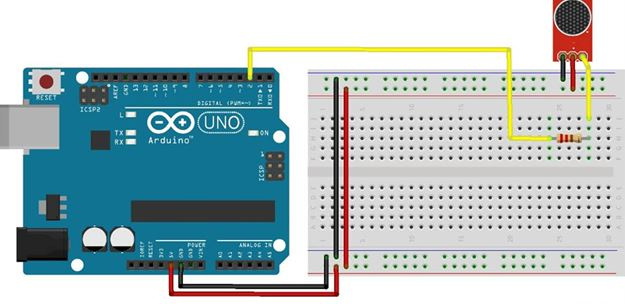
Python code
To perform these actions, we are going to implement a special Python function that will take a pin number, a repetition time, and a sample number as input.
Before proceeding to the explanation of the code, you must open the program file, buzzerPattern.py, from the code folder. At the beginning of the code, you can find the Python function buzzerPattern (), which will be called from the main program with the appropriate parameters. This feature is the core of the entire program.
The function contains two arrays of arrays with hard code, pattern1 and pattern2. Each of them contains the on and off times of the buzzer for a second, which is the duty cycle of the template.
For example, in pattern1, 0.8 represents the time during which the buzzer should be on, and 0.2 represents the opposite.
The function will repeat this buzzer pattern for the repetition periods specified by the function argument. After starting the for loop with a repetition value, the function checks the pattern number from the function argument and executes the pattern.
As soon as the entire repetition cycle is completed, once again completely disable the buzzer, if it is on, and safely disconnect the board using the exit () method:
def buzzerPattern(pin, recurrence, pattern): pattern1 = [0.8, 0.2] pattern2 = [0.2, 0.8] flag = True for i in range(recurrence): if pattern == 1: p = pattern1 elif pattern == 2: p = pattern2 else: print "Please enter valid pattern. 1 or 2." exit for delay in p: if flag is True: board.digital[pin].write(1) flag = False sleep(delay) else: board.digital[pin].write(0) flag = True sleep(delay) board.digital[pin].write(0) board.exit()
The rest of the program is relatively simple, because it contains code for the im-port of libraries and initialization of the Arduino board. As soon as the board is initialized, let's execute the function buzzerPattern () with the input argument (2, 10, 1). This argument will ask the function to play pattern1 10 times on pin number 2:
from pyfirmata import Arduino from time import sleep port = '/dev/cu.usbmodemfa1331' board = Arduino(port) sleep(5) buzzerPattern(2, 10, 1)
DC motor - motor speed control using PWM motors
DC [3] is widely used in robotic applications. They are available in a wide range of voltage characteristics, depending on the application.
In this example, we are using a 5 V DC motor, because we want to supply power through the Arduino board itself. Since the Arduino digital output can only have two states, that is, HIGH (+ 5V) or LOW (0V), it is not possible to control the motor speed using only the OUTPUT mode.
As a solution, we are going to implement the PWM mode using digital pins that are capable of supporting PWM. When using pyFirmata, pins configured in PWM mode take any floating-point input values from 0 to 1.0, which represent 0 and 5 V. respectively.
Connections
In order not to damage the Arduino board due to the large random current loss, we will use a transistor as a switch that uses only a small amount of current to control the high current in the DC motor.
To complete the connection of the circuit, as shown in the following diagram, you need a NPN transistor (TIP120, N2222 or similar), one diode (1N4001 or similar) and a 220 Ohm resistor with a DC motor.
Connect the base of the transistor to digital output 3, which also supports PWM mode. Connect the remaining components as shown in the diagram:
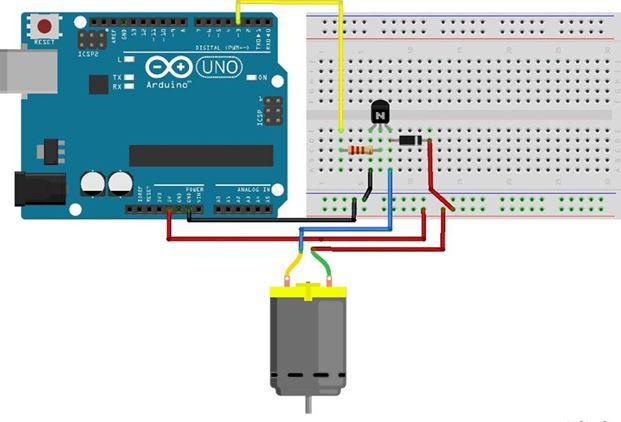
Python code
The user function,
dcMotorControl () , takes the speed and duration of the engine as input parameters, as described in the following code snippet:
def dcMotorControl(r, deltaT): pwmPin.write(r/100.00) sleep(deltaT) pwmPin.write(0)
Use the same code to import the required library and initialize the Arduino board.
After initialization, we assign the digital output mode 3 as PWM, as can be seen from the use of the get_pin ('d: 3: p') method. This code reflects the pinmode's indirect assignment mode, which we learned in the previous section:
In the process of collecting manual data from the user, we run a combination of the try / except operator (to free the board on exit) and the while instruction (to get continuous data from the user).
The code template enters the input () method to get user values (engine speed and engine start duration) from the Python interactive terminal. Once these values are received from the user, the program calls the dcMotorControl () function to perform the motor action: try:
try:
while True: r = input("Enter value to set motor speed: ") if (r > 100) or (r <= 0): print "Enter appropriate value." board.exit() break t = input("How long? (seconds)") dcMotorControl(r, t) except KeyboardInterrupt: board.exit() os._exit
LED - controlling LED brightness using PWM
In the previous pattern, we controlled the speed of the DC motor using PWM. Similarly, you can control the brightness of the LED. Instead of asking the user to enter brightness, we will use the Python module at random in this template.
We will use this module to generate a random number from 1 to 100, which will later be used to record this value on the output and randomly change the brightness of the LED.
This randint () function is really a useful function provided by a random module, and it is widely used in prototype testing by quickly sending random signals.
Connections
You will need a pull-up resistor to connect the LED to the Arduino contact. It is necessary to connect the anode of the LED (longer knife) to the digital output 11 through one resistor with a resistance of 220 Ohm and connect the cathode (shorter leg) to the ground:
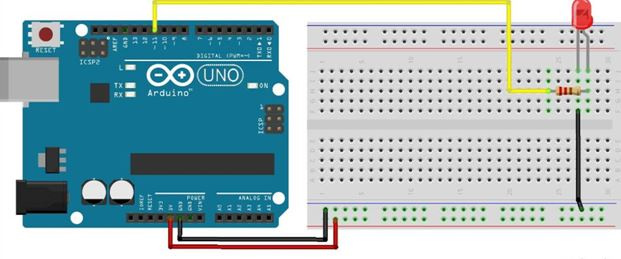
It is important to note that digital pin 11 on the Arduino Uno is also capable of performing PWM along with digital pins 3, 5, 6, 9 and 10.
Python code
Use the Python code with the name
ledBrightnessPWM.py. A float value between 0 and 1.0 is randomly selected before passing it to the PWM output.
The first few lines of code import the necessary libraries and initialize the board.
from pyfirmata import Arduino, INPUT, PWM from time import sleep import random port = '/dev/cu.usbmodemfa1311' board = Arduino(port) sleep(5)
In this example, we use the direct pin mode assignment method. In the following code snippet, a digital pin 11 is assigned to the PWM mode:
pin = 11 Board.digital [pin] .mode = PW
After the end of the cycle, you need to safely disable the Arduino board after turning off the LED for the last time.
board.digital[pin] .write(0) board.exit()
findings
In this publication, the simplest examples reveal the most common approach to programming sensors and control devices to them. Presents Python codes that can be easily used in your own Arduino projects. For clarity, the examples used various sensors and code templates.
Links
- Buzzer (Trema-module).
- Book: "Arduino Basic Connections".
- Arduino and engines.