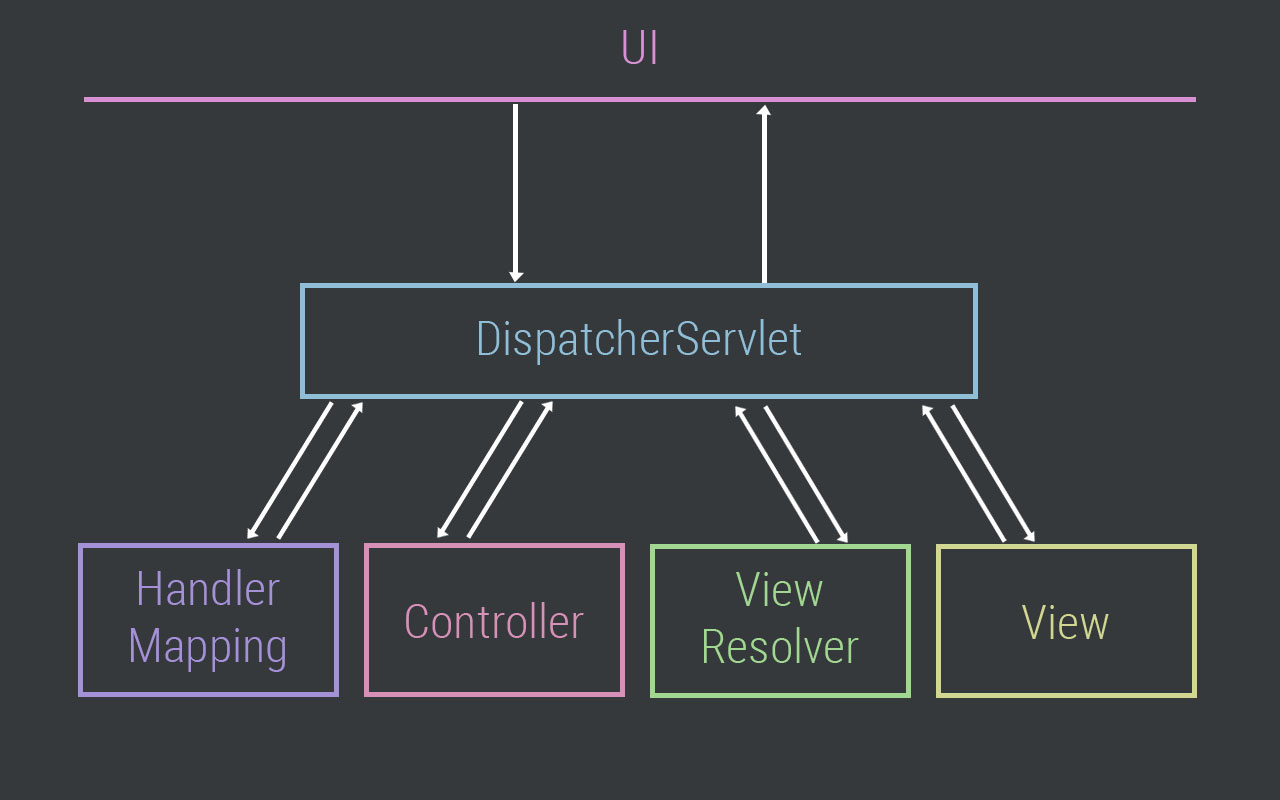
The
Spring MVC framework provides the Model - View - Controller pattern architecture (Model - Display (hereinafter - View) - Controller) with the help of loosely connected ready-made components. The MVC pattern separates the aspects of the application (input logic, business logic, and UI logic), while ensuring free communication between them.
- Model (Model) encapsulates (combines) data applications, in general, they will consist of POJO ("good old Java-objects", or bins).
- View (Display) is responsible for displaying Model data, typically generating the HTML that we see in our browser.
- The Controller (Controller) processes the user's request, creates the corresponding Model and sends it for display in the View.
DispatcherServlet
The entire logic of Spring MVC is built around
DispatcherServlet , which accepts and processes all HTTP requests (from the UI) and responses to them. The dispatcherServlet request processing workflow is illustrated in the following diagram:
')

Below is the sequence of events corresponding to the incoming HTTP request:
- After receiving the HTTP request, DispatcherServlet accesses the HandlerMapping interface, which determines which Controller should be invoked, and then sends the request to the correct Controller.
- The controller accepts the request and calls the appropriate service method based on GET or POST. The invoked method identifies the Model data based on the specific business logic and returns the View name to the DispatcherServlet.
- Using the ViewResolver DispatcherServlet interface, it determines which View to use based on the received name.
- After the View is created, DispatcherServlet sends the Model data as attributes to the View, which is ultimately displayed in the browser.
All of the above components, namely
HandlerMapping ,
Controller and
ViewResolver , are parts of the
WebApplicationContext interface extends the
ApplicationContext , with some additional features needed to create web applications.
Configuration
You will need to link (zamapit) requests that you want to process using DispatcherServlet, using the mapping URL in the file web.xml. Below is an example of the HelloWeb DispatcherServlet ad and map:
<web-app id = "WebApp_ID" version = "2.4" xmlns = "http://java.sun.com/xml/ns/j2ee" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <display-name>Spring MVC Application</display-name> <servlet> <servlet-name>HelloWeb</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>HelloWeb</servlet-name> <url-pattern>*.jsp</url-pattern> </servlet-mapping> </web-app>
The
web.xml file will be in the
WebContent / WEB-INF directory . After HelloWeb is initialized, the framework will attempt to load the application context from a file named
[servlet-name] -servlet.xml , located in the
WebContent / WEB-INF directory . In our case, this will be
HelloWeb-servlet.xml .
Further, the
<servlet-mapping> tag indicates which web addresses are processed by which DispatcherServlet. In our case, all HTTP requests ending in ".jsp" will be processed by HelloWeb.
If you do not want to use
[servlet-name] -servlet.xml /
WebContent / WEB-INF as the default file and directory, you can customize the file name and directory by adding the
ContextLoaderListener servlet listener in web.xml, as shown below:
<web-app...> .... <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/HelloWeb-servlet.xml</param-value> </context-param> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener> </web-app>
Now let's check the configuration for HelloWeb-servlet.xml, located in the WebContent / WEB-INF directory:
<beans xmlns = "http://www.springframework.org/schema/beans" xmlns:context = "http://www.springframework.org/schema/context" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package = "com.tutorialspoint" /> <bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name = "prefix" value = "/WEB-INF/jsp/" /> <property name = "suffix" value = ".jsp" /> </bean> </beans>
The following are important points in HelloWeb-servlet.xml:
- The file [servlet-name] -servlet.xml will be used to create labeled bins, with overriding definitions for each bin defined with the same name in the global scope.
- The <context: component-scan ...> tag will be used to activate the Spring MVC annotation scan feature, which allows you to use annotations such as Controller , @RequestMapping, and so on.
- View names will be defined using the InternalResourceViewResolver . In accordance with the above rule, the view named hello will be implemented at /WEB-INF/jsp/hello.jsp
The next section will show you how to create a
Controller ,
Model, and
View .
Controller Definition
DispatcherServlet sends a request to controllers to perform certain functions. The @Controllerannotation annotation indicates that a particular class is a controller. The @RequestMapping annotation is used for maping (binding) with a URL for the entire class or for a specific handler method.
@Controller @RequestMapping("/hello") public class HelloController { @RequestMapping(method = RequestMethod.GET) public String printHello(ModelMap model) { model.addAttribute("message", "Hello Spring MVC Framework!"); return "hello"; } }
Controller annotation defines a class as a Spring MVC Controller. In the first case, @RequestMapping indicates that all methods in this Controller refer to the URL "/ hello". The following annotation @RequestMapping (method = RequestMethod.GET) is used to declare the
printHello () method as the default method for processing HTTP GET requests (in this Controller). You can define any other method as a handler for all POST requests at a given URL.
You can write the above Controller differently by specifying additional attributes for the @RequestMapping annotation as follows:
@Controller public class HelloController { @RequestMapping(value = "/hello", method = RequestMethod.GET) public String printHello(ModelMap model) { model.addAttribute("message", "Hello Spring MVC Framework!"); return "hello"; } }
The “value” attribute indicates the URL with which we associate this method (value = "/ hello"), further specifies that this method will process GET requests (method = RequestMethod.GET). Also, you need to note the important points in relation to the above controller:
- You define the business logic within the associated service method. From it you can call any other methods.
- Based on a given business logic, within this method you create a Model. You can add Model attributes that will be added to the View. In the example above, we create a Model with the “message” attribute.
- This utility method returns the name of the view as a string. In this case, the requested View has the name "hello".
Creating a View (JSP)
Spring MVC supports many types of Views for various page display technologies. These include JSP, HTML, PDF, Excel, XML, Velocity templates, XSLT, JSON, Atom and RSS feeds, JasperReports, and so on. But the most commonly used templates are JSPs written using JSTL.
Let's write a simple “hello” view in /WEB-INF/hello/hello.jsp:
<html> <head> <title>Hello Spring MVC</title> </head> <body> <h2>${message}</h2> </body> </html>
In this case, the variable
$ {message} displays the same attribute that we set in the Controller. Inside the view you can display any number of attributes.
Examples of the implementation of the Spring MVC framework
Based on the above concepts, I propose to perform several important lessons that will further help us create Spring Web applications:
Spring MVC Hello World ExampleAn example explaining the writing of the simplest Hello World application.
Spring MVC Form Handling ExampleThis example explains how to write a Spring Web application using HTML forms, send data to the controller, and display the processed result.
Spring Page Redirection ExampleUse the page redirect function.
Spring Static Pages ExampleWe get access to static pages together with dynamic ones.
Spring Exception Handling ExampleException Handling.