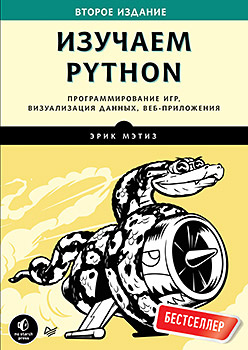
Hi, Habrozhiteli! That year we did a
review of the book by Eric Matis. At the moment a new edition has been released with corrected misprints and listings. The book itself shows the basic principles of programming, introduces lists, dictionaries, classes and cycles, learns to create programs and test code. In the second part of the book, you will begin to use knowledge in practice, working on three large projects: create your own "shooter" with the increasing complexity of levels, work on large data sets and master their visualization, and finally, create a full-featured Django web application, guaranteeing confidentiality of user information.
Inside an excerpt from the book "Meet Django"
Meet Django
Modern websites are in fact multifunctional applications that are quite close to full-fledged desktop applications. Python contains a rich set of tools for building web applications. In this chapter, you will learn how to use
Django to build a Learning Log project — a web-based journaling system for tracking information you have received on a particular topic.
We will write the specification for this project, and then define the models for the data with which the application will work. We will use the Django administrative system to enter some initial data, and then we will learn how to write views and templates, on the basis of which Django will build the pages of our site.
')
Django is a web infrastructure - a set of tools for building interactive websites. Django can respond to page requests, making it easier to read and write information to databases, manage users, and many other operations. In chapters 19 and 20, we will refine the Learning Log project, and then deploy it on the server so that you (and your friends) can use them.
Preparing to create a projectAt the beginning of work on the project it is necessary to describe the project in the specification. Then you will create a virtual environment for building the project.
Writing specificationThe full specification describes the objectives of the project, its functionality, as well as the appearance and user interface. Like any good project or business plan, the specification should focus on the most important aspects and ensure the smooth development of the project. Here we will not write the full specification, but formulate some clear goals that will set the direction of the development process. Here is the specification:
We will write a web application called Learning Log, with which the user can keep a journal of topics of interest to him and create journal entries while studying each topic. The Learning Log home page contains a description of the site and invites the user to register or enter their credentials. After successful login, the user is able to create new topics, add new entries, read and edit existing entries.While studying a new material, it may be useful to keep a journal of what you have learned — records will be useful for monitoring and returning to the necessary information. A good application improves the efficiency of this process.
Creating a virtual environmentTo work with Django, you must first create a virtual environment for working. The virtual environment is a subsection of the system in which you can install packages in isolation from all other Python packages. Separating libraries from one project from other projects will benefit from deploying the Learning Log to the server in Chapter 20.
Create a new directory for the project named learning_log, go to this directory in terminal mode and create a virtual environment. If you are working in Python 3, you can create a virtual environment with the following command:
learning_log$ python -m venv ll_env learning_log$
The command runs the venv module and uses it to create a virtual environment called ll_env. If this method worked, go to the section “Activating the Virtual Environment” on p. 382. If that doesn't work, read the next section, “Installing virtualenv.”
Install virtualenvIf you are using an earlier version of Python or your system is not configured to properly use the venv module, install the virtualenv package. Installing virtualenv is done with the following command:
$ pip install
You may have to use a slightly modified version of this command. (If you haven't used pip yet, see the section “Installing Python Packages Using pip” on page 227.)
NOTE
If you are using Linux, but this method did not work, install virtualenv using the package manager of your system. For example, in Ubuntu, to install virtualenv, use the command sudo apt-get install python-virtualenv.
Go to the learning_log directory in the terminal window and create a virtual environment with the following command:
learning_log$ virtualenv ll_env New python executable in ll_env/bin/python Installing setuptools, pip...done. learning_log$
If you have multiple versions of Python installed on your system, specify the version to be used by virtualenv. For example, the command virtualenv ll_env - python = python3 will create a virtual environment that uses Python 3.
Activation of the virtual environmentAfter the virtual environment is created, it must be activated with the following command:
learning_log$ source ll_env/bin/activate (1) (ll_env)learning_log$
The command runs the activate script from the ll_env / bin directory. When the environment is activated, its name is displayed in parentheses (1); Now you can install packages in the environment and use those packages that were installed earlier. Packages installed in ll_env will be available only while the medium remains active.
NOTE
If you are working on a Windows system, use the ll_env \ Scripts \ activate command (without the word source) to activate the virtual environment.
To finish using the virtual environment, enter the deactivate command:
(ll_env)learning_log$ deactivate learning_log$
The environment also becomes inactive when closing the terminal window in which it works.
Install djangoAfter you have created your virtual environment and activated it, install Django:
(ll_env)learning_log$ pip install Django Installing collected packages: Django Successfully installed Django Cleaning up... (ll_env)learning_log$
Since you are working in a virtual environment, this command looks the same on all systems. It is not necessary to use the --user flag, as well as to use longer commands like python -m pip install package_name.
Remember that you can work with Django only while the environment remains active.
Creating a project in DjangoWithout leaving the active virtual environment (while ll_env is displayed in parentheses), enter the following commands to create a new project:
(1) (ll_env)learning_log$ django-admin.py startproject learning_log . (2) (ll_env)learning_log$ ls learning_log ll_env manage.py (3) (ll_env)learning_log$ ls learning_log __init__.py settings.py urls.py wsgi.py
Team (1) orders Django to create a new project called learning_log. The dot at the end of the command creates a new project with a directory structure that makes it easy to deploy the application to the server after development is complete.
NOTE
Do not forget the point, otherwise you may have problems with the configuration when you deploy the application. And if you still forget, delete the created files and folders (except for ll_env) and run the command again.
The ls (dir on Windows) command (2) shows that Django is creating a new directory named learning_log. A manage.py file is also created - a short program that receives commands and sends them to the appropriate part of Django for execution. We use these commands to manage tasks such as working with databases and running servers.
There are four files (3) in the learning_log directory, the most important of which are the settings.py, urls.py, and wsgi.py files. The settings.py file defines how Django interacts with your system and manages your project. We will change some of the existing settings and add some new settings during project development. The urls.py file tells Django which pages to build in response to browser requests. The wsgi.py file helps Django provide the generated files (the file name is short for “Web Server Gateway Interface”).
Database creation (ll_env)learning_log$ python manage.py migrate (1)Operations to perform: Synchronize unmigrated apps: messages, staticfiles Apply all migrations: contenttypes, sessions, auth, admin ... Applying sessions.0001_initial... OK (2)(ll_env)learning_log$ ls db.sqlite3 learning_log ll_env manage.py
Each database change is called a migration. The first execution of the migrate command tells Django to verify that the database matches the current state of the project. When we first run this command in a new project using SQLite (we’ll talk about SQLite in more detail soon), Django creates a new database for us. At point (1), Django reports the creation of database tables necessary for storing information used in the project (Synchronize unmigrated apps), and then verifies that the database structure corresponds to the current code (Apply all migrations).
Running ls shows that Django creates another file called db.sqlite3 (2). SQLite is a single file database; It is ideal for writing simple applications, because you do not need to especially monitor the management of the database.
View projectMake sure that the project was created correctly. Enter the runserver command:
(ll_env)learning_log$ python manage.py runserver Performing system checks... (1) System check identified no issues (0 silenced). July 15, 2015 - 06:23:51 (2) Django version 1.8.4, using settings 'learning_log.settings' (3) Starting development server at http://127.0.0.1:8000/ Quit the server with CONTROL-C.
Django starts the server so you can review the project on your system and check how it works. When you request a page by typing the URL in a browser, the Django server responds to the request; for this, it builds the appropriate page and sends the page to the browser.
At point (1), Django verifies the correctness of the created project; at point (2), the Django version and the name of the settings file used is displayed; at point (3), the URL for which the project is available is returned. The URL
127.0.0.1 : 8000 / means that the project listens for requests on port 8000 of the local host (localhost), that is, of your computer. The term “local host” refers to a server that processes only requests from your system; It does not allow anyone else to view the developed pages.
Now open a browser and enter the URL
localhost : 8000 / - or
127.0.0.1 : 8000 / if the first address does not work. You will see something like pic. 18.1 - the page that Django creates to let you know that everything is working properly for now. Do not shut down the server yet (but when you want to interrupt it, this can be done by pressing Ctrl + C).
NOTE
If you get the "Port already in use" error message, tell Django to use a different port; to do this, enter the python manage.py runserver 8001 command and continue to sort through the port numbers in ascending order until you find the open port.
»More information about the book can be found on
the publisher's website.»
Table of Contents»
ExcerptOther books on the subject:
Hitchhiking in pythonAuthors: K. Reitz, T. Schlusser. Link to
review .
Simple Python. Modern programming styleAuthor: B. Lyubanovich Link to
review .
Basics of Data Science and Big Data. Python and data scienceAuthors: D. Silen, A. Meisman, M. Ali. Link to
review .
For Habrozhiteley a discount of 20% for the coupon -
Python