Computer vision is, in a nutshell, a set of technologies based on the principles of human vision, which allow a computer to see and understand what it sees. At first glance, it seems to be simple, but in reality this is far from the case.
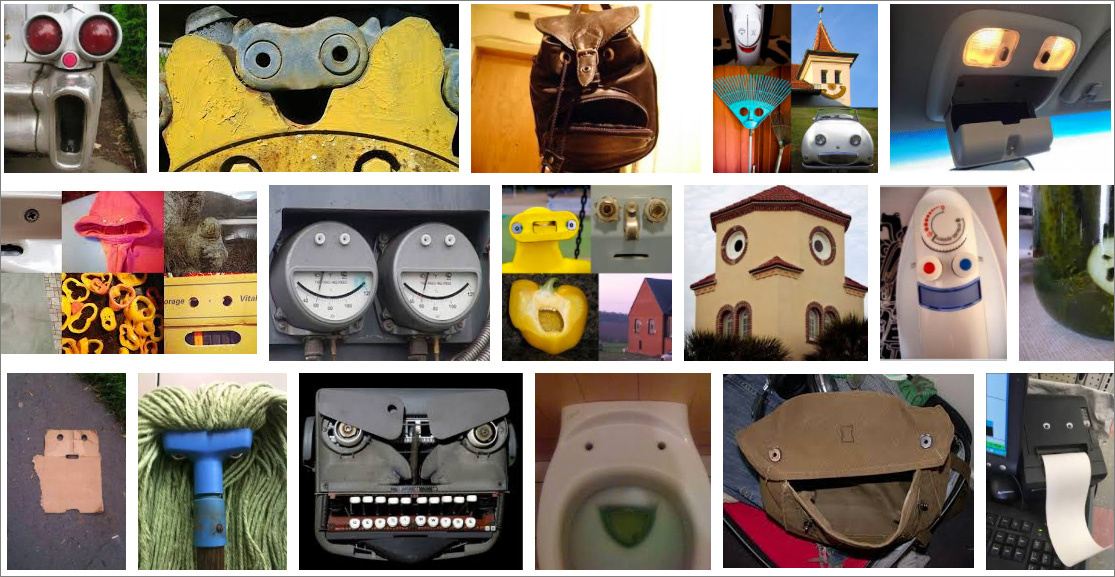
If you want to understand the importance of computer vision and learn about its applications, watch
this video .
')
As the saying goes: “it’s better to see once,” in this case, to see how Amazon uses this technology to create new generation shopping centers. Awesome, right?
If you want to join the technology of computer vision - I suggest to talk about how to create an interactive facial recognition system using a conventional webcam, Node.js and OpenCV.
About studying computer vision
Perhaps it is impossible to give a complete description of computer vision technologies in one material or even in a whole series of articles. Therefore, we consider now only the most important things that will allow us to start developing.
Of course, you can expand your knowledge in this area and on your own, for example, by signing up for the relevant courses, say, on the
EDX .
There is an organization called
OpenCV , working in the field of computer vision. She is developing an open source library of the same name. This library supports C, C ++ and Java. There is no official module for Node.js, however, there is a
node-opencv
module created by enthusiasts. We will use this module in the following sections of the material. Namely, below we consider the following questions:
- Installing OpenCV.
- Working with the OpenCV module for Node.js.
- Development of an interactive facial recognition system.
Install OpenCV
First you need to install the OpenCV library. If you use Ubuntu, the following commands will help you. I checked them on Ubuntu 16.04.
So let's start by installing the dependencies required for OpenCV.
sudo apt-get install build-essential sudo apt-get install cmake git libgtk2.0-dev pkg-config libavcodec-dev libavformat-dev libswscale-dev sudo apt-get install python-dev python-numpy libtbb2 libtbb-dev libjpeg-dev libpng-dev libtiff-dev libjasper-dev libdc1394-22-dev
Now install the OpenCV library itself.
sudo apt-get install libopencv-dev
You can install it in another way, using the
official installation
guide and the commands given in it.
The library
can be installed for Windows.
If you are working on a Mac, you can either compile OpenCV from source, or use
brew
.
brew tap homebrew/science brew install opencv
Node-opencv module
As already mentioned, OpenCV does not officially offer a driver for Node.js. However, in the npm registry there is a
node-opencv
module, which Peter Braden and other programmers are working on. This module is still in development, it does not yet support all OpenCV APIs.
It should be noted that currently the latest build with GitHub is not combined with the
node-opencv
npm module, therefore, installing a module from npm you may encounter problems. It is recommended to install the module directly from GitHub using the following command:
npm install peterbraden/node-opencv
Now let's do the programming.
Development of an interactive face recognition system
Before we recognize faces in the video stream of a webcam, let's deal with performing the same operation for a normal photo.
How to recognize faces in photos? OpenCV provides various classifiers that can be used to recognize faces, eyes, cars, and many other objects. These classifiers, however, are quite simple, they are not trained using machine learning technologies, therefore, when recognizing faces, we can count on accuracy of about 80%.
Here are photos of my friends from one of the usual office parties.
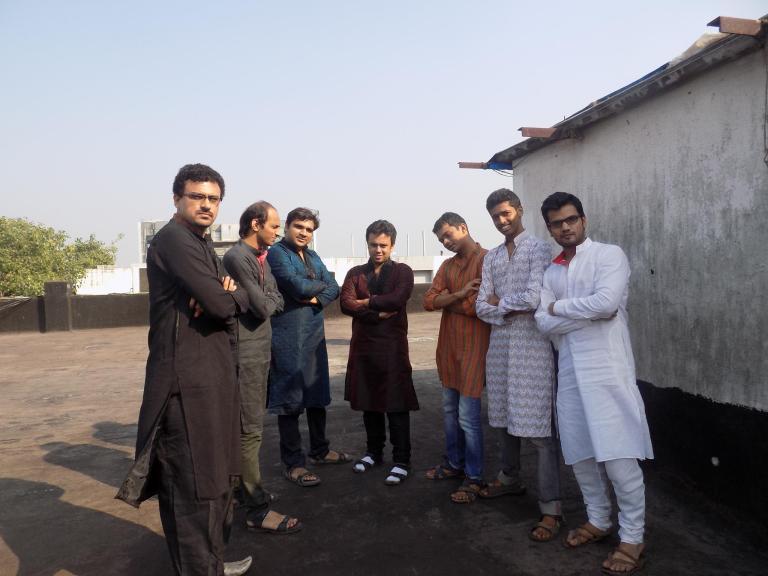
We write a program that will recognize the faces in this picture. Here is the code (
face-detector.js
file) that reads the original image from the disk and displays the new image with the faces marked on it.
var cv = require('opencv'); cv.readImage("./friends.jpg", function(err, im){ if (err) throw err; if (im.width() < 1 || im.height() < 1) throw new Error('Image has no size'); im.detectObject(cv.FACE_CASCADE,{}, function(err, faces){ if (err) throw err; for (var i = 0; i < faces.length; i++){ var face = faces[i]; im.ellipse(face.x + face.width / 2, face.y + face.height / 2, face.width / 2, face.height / 2); } im.save('./friends-faces.jpg'); console.log('Image saved as friends-faces.png'); }); });
This is how the program recognized the faces in the image.
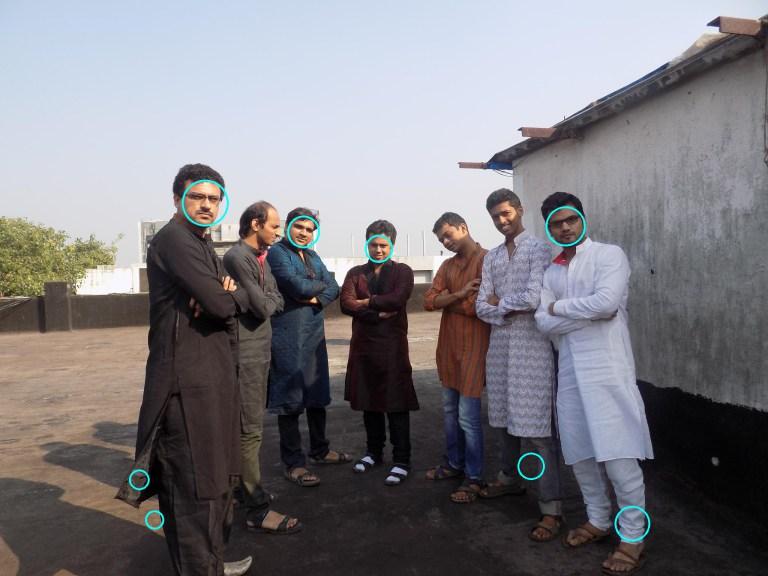
I know it didn't work out very precisely, but a start has been made, and that is good. Let's do the same thing now using a video stream from a webcam. First you need to connect to the camera and display the video. Here is the code (
camera.js
) that does it.
var cv = require('opencv'); try { var camera = new cv.VideoCapture(0); var window = new cv.NamedWindow('Video', 0) setInterval(function() { camera.read(function(err, im) { if (err) throw err; console.log(im.size()) if (im.size()[0] > 0 && im.size()[1] > 0){ if (err) throw err; window.show(im); } window.blockingWaitKey(0, 50); }); }, 20); } catch (e){ console.log("Couldn't start camera:", e) }
Run this code with the following command.
node camera.js
If everything works as it should, you will see a window in which what the camcorder shoots will be displayed. In your case, the picture will be similar to the one shown below, only your face will get into the frame, not mine.
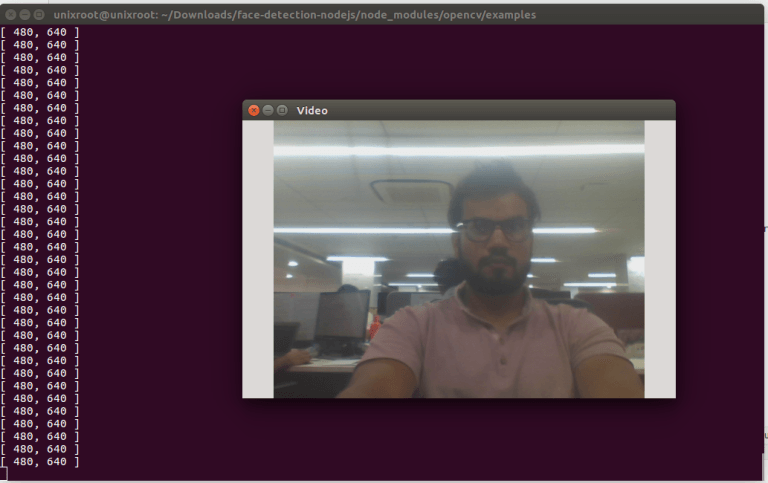
My webcam is not very good, but, honestly, in life I look much better. Let's analyze our program.
var camera = new cv.VideoCapture(0); var window = new cv.NamedWindow('Video', 0)
Here we are trying to access the video stream from the camera and create a new window to display the video.
Now, in order to show the video and not a static image, you need to display in the window a fresh image obtained from the camera. We do this by using the
setInterval()
function and updating the window every 20 milliseconds.
setInterval(function() { camera.read(function(err, im) { if (err) throw err; console.log(im.size()) if (im.size()[0] > 0 && im.size()[1] > 0){ if (err) throw err; window.show(im); } window.blockingWaitKey(0, 50); }); }, 20);
The first image has a size of 0x0 pixels, we do not need to display it. The
if
takes care of this.
So, we know how to recognize faces in photos and how to access video stream from a webcam. Now let's combine it all and our interactive face recognition system will be ready for work. Here is the code (
camera.js
file) that recognizes faces in a video stream.
var cv = require('opencv'); try { var camera = new cv.VideoCapture(0); var window = new cv.NamedWindow('Video', 0) // face detection properties var rectColor = [0, 255, 0]; var rectThickness = 2; setInterval(function() { camera.read(function(err, im) { if (err) throw err; console.log(im.size()) if (im.size()[0] > 0 && im.size()[1] > 0){ im.detectObject(cv.FACE_CASCADE, {}, function(err, faces) { if (err) throw err; for (var i = 0; i < faces.length; i++) { face = faces[i]; im.rectangle([face.x, face.y], [face.width, face.height], rectColor, rectThickness); } window.show(im); }); } window.blockingWaitKey(0, 50); }); }, 20); } catch (e){ console.log("Couldn't start camera:", e) }
Run it.
node camera.js
That's what eventually happened
Again, the recognition accuracy here is low, according to rough estimates - about 80%.
Results
OpenCV is a great open source project. It can be used for free to develop applications that can be used, for example, in areas such as motion recognition and face recognition. In addition, standard classifiers can be trained to improve recognition accuracy. I hope OpenCV will officially support Node.js, which will allow using more features of this wonderful library in this environment. If you want to delve into the technology of machine vision, then, in addition to various training courses, it will be useful to read
this book and look at the
materials from the official site of OpenCV.
Dear readers! Do you use machine vision technology in your projects?