Translation of the article by Valinda Chen .This is a collection of tips for novice developers who are looking at a blank screen and do not know where to start. Often you can hear from young developers working on solving some problems in programming that they are not sure what to grab. You understand the problem itself, the logic, the basics of syntax, and so on. If you see someone's code, or someone helps you, you can do everything yourself. But it happens that you are not confident in your abilities, or at first it is difficult for you to realize your thoughts in code, despite the fact that you know the syntax and logic.
Under the cut - a few tips to solve this problem that will help you in your daily work .
1. Read the conditions of the problem at least three times (or at least as many times as you like)
You cannot solve the problem if you do not understand it . There is a difference between a task and a task that you think you are solving. You can read the first few lines and build assumptions about the rest, since everything looks similar to what you have come across before. Even if you make a popular game like Hangman, make sure you read all its rules, even if you have played it before.
')
Sometimes you can try to explain a task to a friend and see if he understands your explanation. You do not want to go half the way and find that you have misunderstood the requirements. So it is better to spend more time in the beginning to clarify everything. The better you understand the problem, the easier it will be to solve it.
Suppose we create a simple
selectEvenNumbers
function that takes an array of numbers and returns an array of
evenNumbers
with only even numbers. If there are no even numbers in the source array, the
evenNumbers
array
evenNumbers
returned empty.
function selectEvenNumbers() {
What questions you can ask yourself:
- How can a computer say that a number is even? Divide by 2 and check that the result is whole.
- What do I transfer this function to? Array
- What does this array contain? One or more numbers.
- What are the data types of array elements? Numbers
- What is the purpose of this feature? What do I return at the end of its execution? The goal is to get all the even numbers and return them in the array. If there are no even numbers, then the array is returned empty.
2. Complete the task manually with at least three data sets.
Take a piece of paper and go through the task manually. Select at least three data sets to verify. Select the maximum permissible and extreme cases.
Maximum allowable cases : a problem or situation arising outside the normal parameters of functioning. For example, when several variables or states of the environment simultaneously have extreme values, even if each of the parameters is in its specific range.
Extreme cases : problems or situations that occur only with extreme (minimum or maximum) values of the parameters of functioning.
Here, for example, are several data sets to use:
[one]
[12]
[1, 2, 3, 4, 5, 6]
[-200.25]
[-800.1, 2000, 3.1, -1000.25, 42, 600]
When you first start, you often neglect some steps . Since our brain is already familiar with even numbers, we can just look at the set of numbers and immediately transfer to the array 2, 4, 6, and so on, without thinking about how our brain selects specific numbers. If you notice it behind you, it’s better to take a large set of data to prevent the brain from solving a problem simply by looking at the numbers. This will help adhere to the present algorithm.
Let's go through the array
[1]
- We look at the only element of the array
[1]
. - Determine whether it is even. Is not.
- Note that there are no other elements in the array.
- We define that there are no even numbers.
- Return an empty array.
Now go through the array
[1, 2]
- We look at the first element of the array
[1, 2]
- This is
1
. - Determine whether it is even. Is not.
- We look at the next item.
- This is
2
. - Determine whether it is even. Is an.
- Create an array
evenNumbers
and add 2
to it. - Note that there are no other elements in the array.
- We return an array evenNumbers -
[2]
.
You can go through the task several times. Note that the number of steps in the algorithm for
[1]
is different from the algorithm for
[1, 2]
. Therefore, it is recommended to go through several data sets. For example, with a single element; a mixture of integers and non-integers; multi-digit numbers; sets with negative numbers.
3. Simplify and optimize your algorithm.
Look for suitable patterns, maybe something can be generalized. Consider whether you can reduce the number of steps.
- Create a
selectEvenNumbers
function. - Create a new empty array
evenNumbers
to store even numbers. - We pass through each element of the array
[1, 2]
. - Find the first item.
- Divide it by 2 and determine whether it is even. If yes, then add to
evenNumbers
. - Find the next item.
- Repeat step number 4.
- Repeat steps # 5 and # 4 until the elements in the array run out.
- We return an array evenNumbers, regardless of whether there is something in it.
This approach resembles
mathematical induction :
- We prove the truth for
n = 1
, n = 2
, ... - We assume that will be true for
n = k
. - We prove the truth for
n = k + 1
.
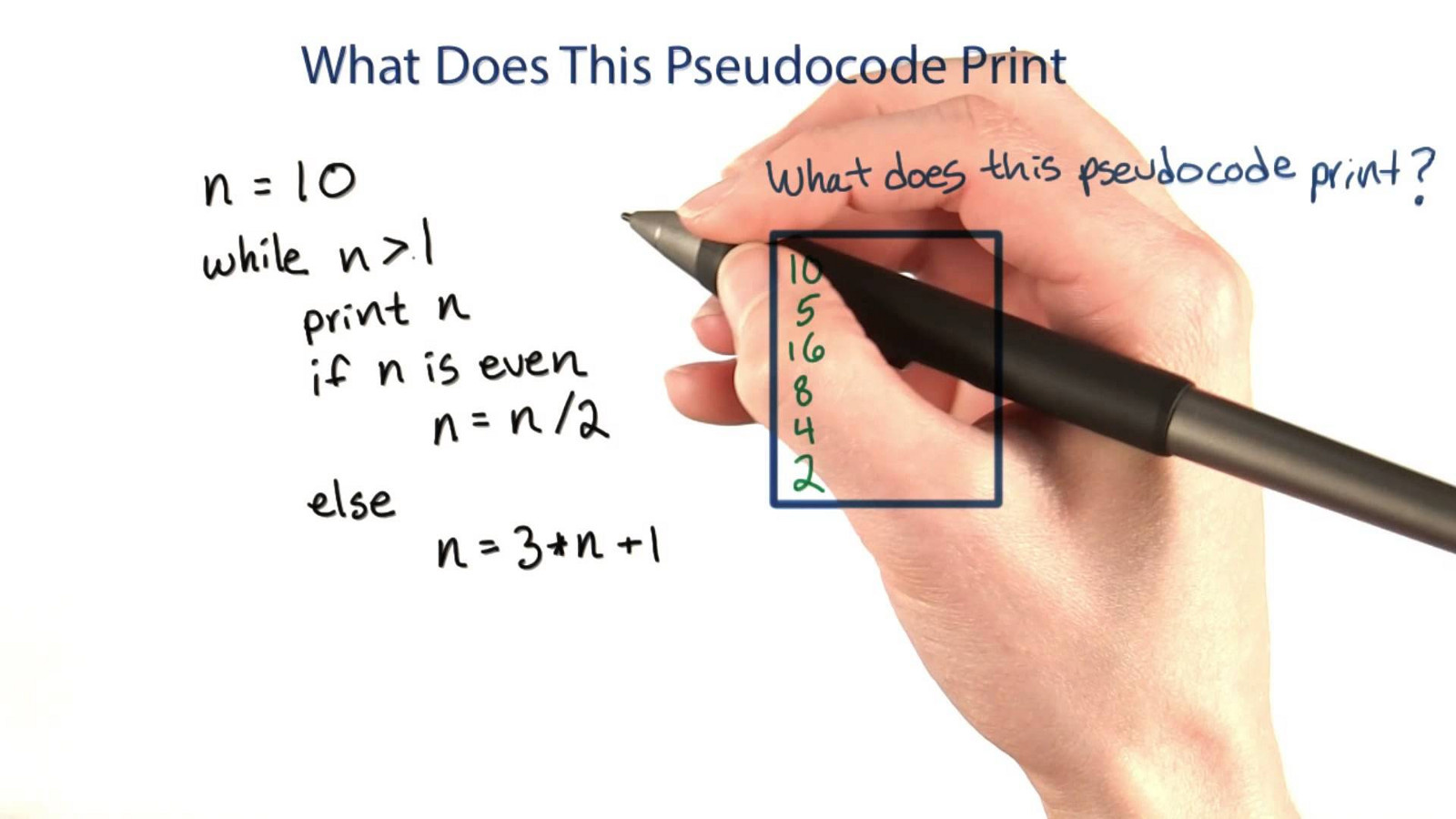
4. Write pseudocode
After working through the basic steps, write pseudocode that can be translated into real code. This will help determine the structure of the code, and generally make it easier to write.
Write pseudocode line by line . This can be done on paper or in the form of comments in the editor. If you are just starting out and think that a blank screen looks scary or distracting, then it’s better to write on paper.
In general, there are no rules for writing pseudo-code, but you can include the syntax from your language if it is more convenient.
But focus not on the syntax, but on the logic and steps of the algorithm .
In our case there are many different options. For example, you can use
filter
, but for the sake of simplicity, we will use a simple
for
loop (however, in the subsequent refactoring we will encounter a
filter
).
Here is an example of pseudo-code, mainly consisting of words:
function selectEvenNumbers evenNumbers , ( 2 ) evenNumbers return evenNumbers
But pseudocode in which words are much less:
function selectEvenNumbers evenNumbers = [] for i = 0 to i = length of evenNumbers if (element % 2 === 0) evenNumbers return evenNumbers
The main thing is to write code line by line and understand the logic of each line.
Return to the task to make sure you are on the right track.
5. Convert pseudocode to normal code and debug it.
When your pseudocode is ready, convert each line to a real code in your language. Here we will use javascript.
If you wrote on paper, then transfer everything to the editor in the form of comments, and then replace each line.
Now call the function and give it some of the previously used data sets. So you can check whether the code returns the desired result. You can also write tests to verify that the output matches the expected result.
selectEvenNumbers([1]) selectEvenNumbers([1, 2]) selectEvenNumbers([1, 2, 3, 4, 5, 6]) selectEvenNumbers([-200.25]) selectEvenNumbers([-800.1, 2000, 3.1, -1000.25, 42, 600])
After each variable or row, you can use
console.log()
. This will help
check if the values and code behave as expected before moving on . This way you catch any problems without going too far. Here is an example of what values can be checked at the beginning of work.
function selectEvenNumbers(arrayofNumbers) { let evenNumbers = [] console.log(evenNumbers)
Below is the code received after processing each line of pseudocode. The characters
//
represent lines from pseudocode.
The real JavaScript code is highlighted in
bold .
Remove the pseudocode, so as not to be confused.
function selectEvenNumbers(arrayofNumbers) { let evenNumbers = [] for (var i = 0; i < arrayofNumbers.length; i++) { if (arrayofNumbers[i] % 2 === 0) { evenNumbers.push(arrayofNumbers[i]) } } return evenNumbers }
Sometimes novice developers are so fond of the syntax that it’s hard for them to go further.
Remember that over time it will be easier for you to comply with the syntax, and there is nothing to be ashamed of later when writing code to refer to reference materials for correct observance of the syntax .
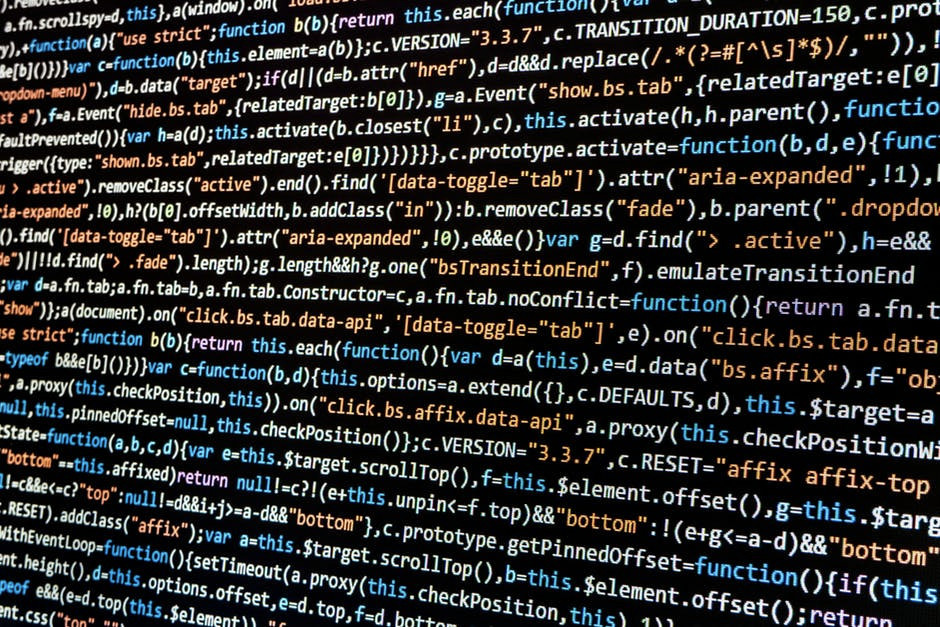
6. Simplify and optimize code
You may have noticed that simplification and optimization are repetitive topics.
"Simplicity is a prerequisite for reliability."
Edsger Dijkstra, a Dutch scientist and one of the pioneers in a number of computer science fieldsIn our example, one of the ways to optimize is to filter the elements in the array by returning a new array using
filter
. In this case, we do not need to define the
evenNumbers
variable, because
filter
will return a new array with copies of the elements that correspond to the filter. In this case, the original array will not change. Also, we do not need to use a
for
loop.
filter
will go through each element, and if it returns true, then the element will go into the array, and if
false
, it will be skipped.
function selectEvenNumbers(arrayofNumbers) { let evenNumbers = arrayofNumbers.filter(n => n % 2 === 0) return evenNumbers }
It may take several iterations to simplify and optimize the code as you find new ways.
Ask yourself these questions:
- What are the goals of simplification and optimization? Goals depend on your style or personal preference. Are you trying to maximize the code? Or do you want to make it more readable? In this case, you can add individual lines with the definition of a variable or the calculation of something, and not try to do everything in one line.
- How else can you make the code more readable?
- Can I still reduce the number of steps?
- Are there variables or functions that are not needed or not used?
- Any steps repeated? See if you can define in another function.
- Are there more efficient ways to handle extreme cases?
"Programs should be written in such a way that people read them, and only secondarily, in order for their machines to execute."
Gerald Sassman and Harold Abelson, authors of “Structure and Interpretation of Computer Programs”7. Debug
This step must be performed during the whole process. Debugging through will help you catch any syntax errors or logic flaws earlier. Take advantage of your IDE (Integrated Development Environment) and debugger. When detecting a bug, we recommend viewing the code line by line, trying to find unexpected things. Some tips:
- Read what is written in the error messages in the console. Sometimes they indicate line numbers for verification. This will give you a starting point for searches, although sometimes problems may be hidden in other lines.
- Comment on code or line blocks, as well as output, to quickly notice code behavior. If necessary, the code can always be uncommented.
- Use other sample data if unsolicited scripts occur.
- Save different versions of the file if you use other approaches. You shouldn’t lose what you’ve gained if you want to roll back to the previous solution!
“The most effective debugging tool is careful thinking in conjunction with reasonably placed display commands.”
Brian Kernigan, professor of computer science at Princeton University8. Write helpful comments.
In a month, you may not remember what each line of code means. And the one who will work with your code doesn't know this at all. Therefore, it is important to write useful comments to avoid problems and save time later when you have to return to this code again.
Avoid comments like this:
// . .
// .
Try to write short, high-level comments that will help you understand what is happening here, if it is not obvious. This is useful in solving complex problems, you can quickly understand what a particular function is doing, and why. Through the use of understandable comments and the names of variables and functions, you (and other people) will be able to understand:
- What is this code for?
- What is he doing?
9. Get feedback through revision code
Get feedback from colleagues, executives and other developers. Read the stack overflow. See how others solved similar problems and learn from them. Often there are several ways to solve the problem. Find out what they are, and it will be faster and easier for you to come to them yourself.
"No matter how slow you write clean code, you will always spend more time if you write dirty code."
Uncle Bob Martin, software engineer and co-author of the Agile manifesto10. Practice, practice, practice
Even experienced developers constantly practice and learn. If you get a useful response, implement it. Solve the problem again, or similar tasks. Force yourself.
With every problem you solve, you become better as a developer . Enjoy everyone success and do not forget how much you have already passed. Remember that programming, like any other activity, will eventually become easier and easier.
“Be proud of how much you have gone. Believe that you will pass even more. But don't forget to enjoy the journey. ”
Michael Josephson, founder of the Joseph and Edna Josephson Institute of Ethics