Hello! Once upon a time I did simple toys on Flash. For example: a toy - to hold the mouse cursor through the maze, without touching the walls and dodging any moving objects. Some of these objects move along a given trajectory, some chase the cursor, and some shoot the cursor with other moving objects.
Now I have become interested in programming for android and made about the same
toy . And faced with the same geometric puzzles that met then.
Task 1: paint the walls. And immediately
Task 2 arises
: to determine whether a point is touching the wall or not (you lost or continue the game).
')
For this, I divided the walls into shapes: rectangles and polygons.
Everything is simple with rectangles: Just draw:
canvas.drawRect(x1, y1, x2, y2, paint);
and just check whether the point is inside it or not.
public boolean isTouched(float x, float y){ return (x>x1)&&(x<x2)&&(y>y1)&&(y<y2); }
With a polygon, the situation is not so simple: to draw it is already a little more difficult.
Path path = new Path(); paint.setStyle(Paint.Style.FILL); path.moveTo(x1, y1); path.lineTo(x2, y2); path.lineTo(x3, y3); ..... path.lineTo(x1, y1);
And to check the touch with a point is even more difficult. I tried to remember the school course of geometry, then googled and found such a solution:
The polygon must be convex.

And it is necessary to describe it in a clockwise direction.
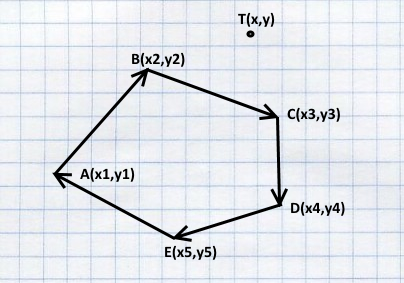
At the same time, each edge has initial and final coordinates, that is, in fact, is a vector. And you can determine whether the point is to the right or left of it.
For this there is a simple formula.
private boolean isLeftHandSituated(float dotX, float dotY, float x1, float y1, float x2, float y2){ float d = (dotX - x1) * (y2 - y1) - (dotY - y1) * (x2 - x1); return d>0; }
To determine whether a point is inside or outside, I loop through all the edges, and if at least one edge has a point on the left (in this case, this is BC), then it is outside, since the polyhedron is described clockwise.
Task 3: yellow smiley. If a point approaches it at a certain distance, then it chases a point. You can calculate this specific distance (distance) using the Pythagorean theorem:
float dx = dotX - smileX; float dy = dotY - smileY; double distance = Math.sqrt((dx * dx + dy * dy));
Smiley chases a point. What does it mean? This means that after each certain time interval (in the next frame) its coordinates are moved a certain distance closer to the point. This distance per frame is essentially speed. I called the variable speed.
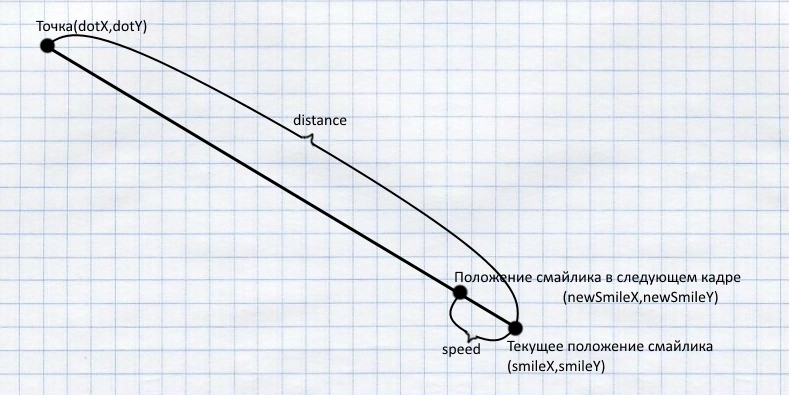
Calculate the coordinates of the emoticon in the next frame, you can:
private float speed=5; double rate = speed / distance; newSmileX = smileX + (float) (dx * rate); newSmileY = smileY + (float) (dy * rate);
Task 4: the gun is always aimed at the point. How to calculate the angle at which it should be rotated? Very simple. For this there is a method atan2.
float dx = dotX - cannonX; float dy = dotY - cannonY; double theta = Math.atan2(dx, dy);
Conclusion: The article was rather concise and short, but, I hope, useful to many novice game developers. Good luck to everyone in training and development!