Alena Batitskaya, a senior graduate student and methodologist of the “
Programming ” faculty in “
Netology ”, made a review of innovations that appeared in JavaScript with the release of ECMAScript 2017. We have all been waiting for this for a long time!

In the history of the development of JavaScript there were periods of stagnation and rapid growth. Since the advent of the language (1995) and up to 2015, the updated specifications have not been issued regularly.
')
It's good that for the third year already we know exactly when to wait for the update. In June 2017, an updated specification was released: ES8 or, more correctly, ES2017. Let's consider together what updates to the language occurred in this version of the standard.
Asynchronous functions
Perhaps one of the most anticipated innovations in JavaScript. Now everything is official.
Syntax
To create an asynchronous function, the async keyword is used.
- Asynchronous function declaration:
async function asyncFunc() {}
- An expression with an asynchronous function:
const asyncFunc = async function () {}
; - Method with an asynchronous function:
let obj = { async asyncFunc() {} }
- Asynchronous switch function:
const asyncFunc = async () => {};
async
operator
Let's see how it works. Create a simple asynchronous function:
async function mainQuestion() { return 42; }
The
mainQuestion
function returns a promise, despite the fact that we are returning a number:
const result = mainQuestion(); console.log(result instanceof Promise);
If you have previously used generators from ES2015, then immediately recall that the generator function automatically creates and returns an iterator. With asynchronous function is exactly the same.
And where is the number 42 that we returned? They are absolutely clear way resolved promis, which we returned:
console.log(''); mainQuestion() .then(result => console.log(`: ${result}`)); console.log('');
Promis is resolved asynchronously, so we get the following output to the console:
: 42
And what happens if our asynchronous function doesn't return anything at all?
async function dumbFunction() {} console.log(''); dumbFunction() .then(result => console.log(`: ${result}`)); console.log('');
Promis will be resolved with “nothing”, which in JavaScript corresponds to the
undefined
type:
: undefined
Despite the name, the asynchronous function itself is called and executed synchronously:
async function asyncLog(message) { console.log(message); } console.log(''); asyncLog(' '); console.log('');
The output to the console will be like this, although many might have expected otherwise:
The asynchronous function returns a promise.
I hope you have already figured out what promises are. Otherwise, I recommend to pre-deal with them.
And what will happen if we in the body of the function also return the promise?
async function timeout(message, time = 0) { return new Promise(done => { setTimeout(() => done(message), time * 1000); }); } console.log(''); timeout(' 5 ', 5) .then(message => console.log(message)); console.log('');
It will join the chain to the promise, which is created automatically, as if we returned the promise inside the callback passed to the
then
method:
5 (_ 5 _)
But if we had written not the asynchronous function, but the usual one, then everything would work exactly the same as in the example above:
function timeout(message, time = 0) { return new Promise(done => { setTimeout(() => done(message), time * 1000); }); }
Then why do we need asynchronous functions? The coolest feature of an asynchronous function is the ability in the body of such a function to wait for the result (when promise is resolved) of another asynchronous function:
function rand(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } async function randomMessage() { const message = [ '', ' ?', ' ' ][rand(0, 2)]; return timeout(message, 5); } async function chat() { const message = await randomMessage(); console.log(message); } console.log(''); chat(); console.log('');
Notice that the body of the
chat
function looks like the body of a synchronous function, there is not even a single callback function. But
await randomMessage()
will not return us a promise, but wait 5 seconds and return the message itself, which promises are allowed. This is his role: “wait for the result of the right operand”.
?
The message
after calling the
chat
function is displayed immediately, without waiting for the message to be displayed in the body of the
chat
function. Therefore, it is logical to rewrite this part as:
console.log(''); chat() .then(() => console.log('')); console.log(' ');
await
operator
await
is a handy thing that allows you to beautifully use promises without callbacks. But it works only in the body of an asynchronous function. This code will generate a syntax error:
console.log(''); await chat(); console.log('');
The fact that asynchronous functions can be “stopped” is another similarity with generators. Using the
await
keyword in the body of an asynchronous function, we can wait (
await translates to
expect ) the result of executing another asynchronous function, just as with the help of
yield
we “wait” for the next call to the
next
iterator method.
But what if we “wait” for a synchronous function that returns a promise? Yes, you can:
<source lang="javascript">function mainQuestion() { return new Promise(done => done(42)); } async function dumbAwait() { const number = await mainQuestion(); console.log(number); } dumbAwait();
But what if we “expect” a synchronous function that returns a number (string or something else)? Yes, you can also:
function mainQuestion() { return 42; } async function dumbAwait() { const number = await mainQuestion(); console.log(number); } dumbAwait();
We can even "wait" for the number, although there is no special point in this:
async function dumbAwait() { const number = await 42; console.log(number); } dumbAwait();
The
await
operator has no difference what to expect. It works in the same way as the
then
callback method works:
- if promise returned: wait promise, and return the result;
- if no promise is returned: we wrap in
Promise.resolve
and onwards in a similar way.
await
sends the asynchronous function to asynchronous swimming:
async function longTask() { console.log(''); await null; console.log(''); for (const i of Array (10E6)) {} return 42; } console.log(''); longTask() .then(() => console.log('')); console.log(' ');
Please take this example as a demonstration of the work of
await
, and not as a “convenient” trick. The result of the work below:
Error processing
What if the promise we are “waiting for” with
await
not resolved? Then
await
will throw an exception:
async function failPromise() { return Promise.reject(''); } async function catchMe() { try { const result = await failPromise(); console.log(`: ${result}`); } catch (error) { console.error(error); } } catchMe();
We can catch this exception like any other, with
try-catch
and do something about it.
Application
The internal structure of asynchronous functions is similar to a mixture of promises and generators. In fact, an asynchronous function is syntactic sugar for a combination of these two cool features of a language. It is a logical replacement for related callbacks.
In the body of the asynchronous function, we can write sequential asynchronous calls as a flat synchronous code, and this is what we were waiting for:
async function fetchAsync(url) { const response = await fetch(url); const data = await response.json(); return data; } async function getUserPublicMessages(login) { const profile = await fetchAsync(`/user/${login}`); const messages = await fetchAsync(`/user/${profile.id}/last`); return messages.filter(message => message.isPublic); } getUserPublicMessages('spiderman') .then(messages => show(messages));
Try rewriting this code in promises, and evaluate the difference in readability.
Nicholas Bevacua in his article "
Understanding JavaScript's async await " in great detail examines the principles and peculiarities of asynchronous functions. The article is richly seasoned with code examples and user cases.
Support
Today, asynchronous functions
support all major browsers . So you can safely begin to use this feature of the language, if you have not already done so.

Object.values
and Object.entries
These new features are primarily intended to facilitate the work with objects.
Object.entries()
This function returns an array of the object's own enumerated properties in the format [key, value].
If the structure of the object contains keys and values, then the output will be recoded into an array containing arrays with two elements: the first element is the key, and the second element is the value. The pairs [key, value] will be arranged in the same order as the properties in the object.
Object.entries({ : 1, : 2 });
The result of the code will be:
[ [ '', 1 ], [ '', 2 ] ]
If the data structure passed to
Object.entries()
does not contain keys, then in their place will be the index of the array element.
Object.entries(['n', 'e', 't', 'o', 'l', 'o', 'g', 'y']);
At the output we get:
[ [ '0', 'n' ], [ '1', 'e' ], [ '2', 't' ], [ '3', 'o' ], [ '4', 'l' ], [ '5', 'o' ], [ '6', 'g' ], [ '7', 'y' ] ]
Characters are ignored.
Note that the property whose key is the character will be ignored:
Object.entries({ [Symbol()]: 123, foo: 'bar' });
Result:
[ [ 'foo', 'bar' ] ]
Iteration over properties
The appearance of the
Object.entries()
function finally gives us a way to iterate over the properties of an object using the
for-of
loop:
let obj = { : 1, : 2 }; for (let [x,y] of Object.entries(obj)) { console.log(`${JSON.stringify(x)}: ${JSON.stringify(y)}`); }
Conclusion:
"": 1 "": 2
Object.values()
This function is close to
Object.entries()
. At the output we get an array consisting only of the values of our own properties, without keys. What, in principle, can be understood from the title.
Support

Today,
Object.entries()
and
Object.values()
supported by major browsers.
"Hanging" commas in function parameters
Now it’s legal to leave commas at the end of the function argument list. When calling the function, the comma at the end is also outside of crime.
function randomFunc( param1, param2, ) {} randomFunc( 'foo', 'bar', );
Hanging commas are also allowed in arrays and objects. They are simply ignored and have no effect on the work.
Such a small, but certainly useful innovation!
let obj = { : '', : '', }; let arr = [ '', '', '', ];
Support

We'll have to wait a bit before leaving a comma at the end of the list of parameters.
"Stubs" for strings: we reach the desired length
In ES8, there are two new methods for working with strings:
padStart()
and
padEnd()
. The
padStart()
method substitutes additional characters before the beginning of the line, on the left. And
padEnd()
, in turn, on the right, after the end of the line.
str.padStart(, []); str.padEnd(, []);
Keep in mind that the length you specify as the first parameter will include the original string.
The second parameter is optional. If it is not specified, the string will be added with spaces (the default value).
If the source string is longer than the specified parameter, the string will remain unchanged.
''.padStart(6, '~');
Support

Beautiful picture!
Object.getOwnPropertyDescriptors()
function
The function returns an array with descriptors of all the object's own properties.
const person = { first: '', last: '', get fullName() { return ` , ${first} ${last}`; }, }; console.log(Object.getOwnPropertyDescriptors(person));
Result:
{ first: { value: '', writable: true, enumerable: true, configurable: true }, last: { value: '', writable: true, enumerable: true, configurable: true }, fullName: { get: [Function: get fullName], set: undefined, enumerable: true, configurable: true } }
Application area
- For copying object properties, including getters, setters, write-once properties.
- Copy object.
.getOwnPropertyDescriptor
can be used as the second parameter in Object.create()
. - Creating cross-platform object literals with a specific prototype.
Note that methods with
super
cannot be copied because they are closely related to the original object.
Support

Even IE is fine.
Memory Sharing and Atomics
Object
This innovation introduces the concept of shared memory in JavaScript. The new
SharedArrayBuffer
design and the already existing
TypedArray
and
DataView
help allocate available memory. This provides the necessary order of operations when multiple threads simultaneously use shared memory.
The
SharedArrayBuffer
object is a primitive building block for high-level abstractions. The buffer can be used to redistribute bytes between multiple worker threads. It has two distinct advantages:
- The speed of data exchange between workers is increased.
- Coordination between workers is faster and easier (compared to
postMessage()
).
Secure access to shared data
The new
Atomics
object cannot be used as a constructor, but it has a number of its own methods, which are designed to solve the security problem when performing various operations on
SharedArrayBuffer
typed arrays.
Support
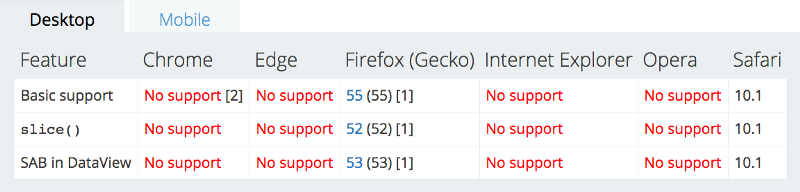
This "update" is still bad with support. Hope, believe, wait.
Alex Raushmayer described in detail the mechanism of this language feature in the article “
ES proposal: Shared memory and atomics ”.
According to a
survey conducted by StackOverflow in 2017, JavaScript is the most widely used programming language. Personally, I am very pleased with the current picture:
- new specification every year;
- browsers quickly innovate;
- The working group consists of representatives of corporations, which allows you to quickly exchange implementation experience and promptly correct either the descriptive or technical parts.
The language lives, develops, in the eyes turns into an even more convenient, flexible and powerful tool.
Frankly speaking, it is not always possible to keep up with this rapid growth and study, and most importantly use all the newest and most relevant in their work. That is why it is so important to get the most up-to-date information during the initial development and to enter the labor market with a savvy and up-to-date specialist.
My colleagues in
Netology and I understand that the advantage of online education is its flexibility and ability to adapt to the ever-changing realities of the development world. For this reason, we do not stop working on updating the materials of our courses and promptly talk about all the new features. And now we are finalizing the “
JavaScript: from zero to promise ”
course so that every student knows and knows how to work with the tools that appeared in ECMAScript 2017.
In preparing the article used materials: