The article is a very brief introduction to Pike. Admit it - few of you have heard of this language. However, the Pike language is even used in production (for Opera to work in Turbo mode).
Brief specifications:
- interpretable (you will not think what to do at compile time);
- syntax: based on C (with minimal differences);
- license - GNU GPL, GNU LGPL and MPL;
- object-oriented;
- with the garbage collector (which, by the way, you can prompt if you really need);
- ...
Story')
The language appeared as early as 1994. Posted by Fredrik HĂĽbinette. The ancestor is LPC (an object-oriented language based on C language, created primarily for game development -
LPC This is actually an interesting story - but there is no sense in copying and paste a wiki. In short, if not for the game, there would be no language.
I will say right away - the documentation is not all right. Not all examples (even for beginners) will work (and it can even be Hello world!).
To get started, you can run the interpreter. To do this, you can simply type pike in the console without parameters. And experiment. But we will not do that. Let's just write it down (for example, in a notebook).
Hello World!Program text:
int main() { write("Hello world!\n"); return 0; }
Save this text in hello.pike and run in the command line:
pike hello.pikeNow with a window:
int main() { GTK.setup_gtk(); object w = GTK.AboutDialog(); w.set_program_name("My GTK hello world program"); w.signal_connect("destroy", lambda(){exit(0);}); w.set_title("My first program"); w.set_comments("Pike is a dynamic programming language with a syntax similar to Java and C. "+ "It is simple to learn, does not require long compilation passes and has powerful built-in" + "data types allowing simple and really fast data manipulation."); array(string) arr1=({"Mr. Smith", "and others"}); array(string) arr2=({"Mrs. Smith", "and others"}); w.set_authors(arr1); w.set_artists(arr2); w.show_now(); return -1; }
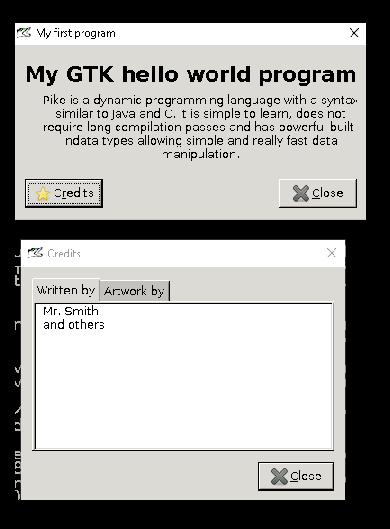
As you can see, the work goes through GTK. Returning -1 from the main function is necessary so that the program does not immediately stop working, otherwise you will not see the window. The exit and the program is done by the button closing the window using the lambda-function lambda () {exit (0);} attached.
GTK.Alert (“Hello world!”) Is used for this in the official tutorial, but this did not work for me (version 8.0) - apparently the tutorial is outdated.
Data structures:The syntax of working with basic data structures pleases.
Arrays :
int main() { array(string) arr1 = ({ "red", "green", "white" }); write(arr1); write("\n"); array(string) arr2 = ({ "red", "green", "yellow" }); write(arr2); write("\n"); write(arr2 + arr1);
Result :
redgreenwhite
redgreenyellow
redgreenyellowredgreenwhite
redgreen
redgreenyellowwhite
yellowwhite
yellow
Maps :
int main() { mapping map2 = (["red":4, "white":42, "blue": 88]); mapping map1 = (["red":4, "green":8, "white":15]); print_map(map2 + map1); print_map(map2 - map1); print_map(map2 & map1); print_map(map2 | map1); print_map(map2 ^ map1); return 0; } void print_map(mapping m){ array(string) arr; arr = indices(m); foreach(arr, string key){ write(key + ":" + m[key] + " "); write("\n"); }
Result :
red: 4 green: 8 white: 15 blue: 88
blue: 88
red: 4 white: 15
red: 4 white: 15 green: 8 blue: 88
green: 8 blue: 88
There is also the so-called
multiset . Essentially also mapping, but without values:
int main(){ multiset o = (< "", 1, 3.0, 1, "hi!" >); print_multiset(o); return 0; } void print_multiset(multiset m){ array(string) arr; arr = indices(m); foreach(arr, string key){ write(key + ":" + m[key] + " "); }; write("\n"); }
Result :
1: 1 1: 1 3.0: 1: 1 hi!: 1
Objects class car { public string color; public string mark; private string driver; void create(string c, string m, string d){ color = c; mark = m; driver = d; } string who(){ return mark + " " + color + "\n"; } } int main(){ car car1 = car("red", "vaz", "Mike"); write(car1.who()); car car2 = car("green", "mers", "Nik"); write(car2.who()); write(car2.mark); return 0; }
Result :
vaz red
mers green
mers
The create method plays the role of a constructor. There are access modifiers. But be careful with the static modifier. Not only does he mean something completely different from what you thought - he is also depricated.
Java connectionAnd now jerk the Java code (and why not if we can?):
int main() { float pi = Java.pkg.java.lang.Math.PI; write("Pi = " + pi + "\n"); object syst = Java.pkg.java.lang.System; write("time = " + syst.currentTimeMillis() + "\n"); object str = Java.pkg.java.lang.String("...Hello!..."); write((string)str.substring(3,str.length()-3) + "\n"); object map2 = Java.pkg.java.util.HashMap(); object key = Java.pkg.java.lang.String("oops"); object val = Java.pkg.java.lang.String("ha-ha"); map2.put(key, val); write((string) map2.get("oops") + "\n"); object map = Java.JHashMap(([ "one":1, "two":2 ])); write((string) map.get("two") + "\n"); return 0; }
As you can see from the example, the Java classes are accessed via Java.pkg. When printing, you must not forget to cast Java objects to a string using (string). You can invoke the usual Java methods. As you can see from the example, for HashMap there is even a special design to facilitate the work (which, however, is not surprising).
Work with the InternetDownload the page from the Internet and display in the console:
int main() { Protocols.HTTP.Query web_page; web_page = Protocols.HTTP.get_url("https://pike.lysator.liu.se/about/"); string page_contents = web_page->data(); write(page_contents); return 0; }
Pike remembers the USSR: int main(){ Geography.Countries.Country c = Geography.Countries.USSR; write(c.name + "\n"); return 0; }
Digression from the topic(While preparing this material, I experimented in parallel with the task of determining a country by IP address - at my own address, of course. And when I confused the programs, I was startled and the USSR came back).
ConclusionMy personal opinion is a curious language, but very raw. Or you can consider it as a language created for a specific project. Documentation is very lame. But let all the flowers grow.
Links→
Home→
Wikipedia (eng)→
Article about language→
github→
Roxen (web server on Pike)