On Habré there are many articles about react native, but I can not understand by whom and for whom they are written. Almost everyone has such a message: “Well, this is the same React as on the web, only on mobile phones”. But the people who are engaged in native development for mobile devices in the span, because to understand the reactor after Android
without two liters without in-depth study does not work. And I will tell for reakt relying, suddenly, not on web development, but on Android. All interested welcome under cat.
What for?
I understand that the main audience of react native is just the same web developers who wanted to poke into mobile devices. But why should a mobile developer look in the direction of react native? I’ll omit the moment when I stumbled upon react native, I’ll just say that I’ve been looking at it for several months and have been actively studying the last 4-5 days. I realized that the reactor is ready to create simple applications that receive and send data. And then I started to remember all my projects and realized that most of them just receive and send data, with rare exceptions. It turns out that most of the applications I could make a react? Aha, and plus there would be still applications on iOS.
Of course, native development is better in all respects, but there are a couple of BUT:
Firstly, native development for android is complex and full of a bunch of little things, the most banal example is the screen rotation, there are a lot of different solutions and libraries, even I myself built up my
crutches . And in the reactor everything is simple, I made the screen, but you can forget about the turns out of the box, everything works (as I understand it) not in the main thread, basically I only redraw the view, the screen rotation does not affect the life cycle of the components of the reactor and that’s fine.
')
The point is that I have been working on native development for android for quite some time and still have to face new strange (but, at times, interesting) problems. And if I need applications and for iOS, how long will it take for me to learn how to write natively under iOS? I have already studied iOS and encountered the problem of approaches: how do you write applications? Patterns, libraries, best practices, and more. And for each platform its own troubles. In the case of the reactor, too, their problems, but they are common to both platforms. So I made my choice: for simple applications, the forces of the reactor are enough, when difficulties begin, you can resort to the main trump card - to write a module natively for the desired platform. Well, the cherry on the cake is that the phrase: “Well, this is the same React as on the web, only on mobile phones” works in the opposite direction, studying react native, I use the same tools and approaches as on the web, it will be possible easier to change the direction of development on the web.
Instruments
Directly about the installation can be read
here . Further, I will assume that you have read that mini-tutorial.
Of course, after Android studio everything will not be so convenient, you will have to work a lot with the console (if you don’t run the studio at all), but you can get used to it. I personally use:
To command:
react-native run-android
The effect is that the virtual device is already running. Android studio does everything for us after clicking on the magic green button, and here we have to do everything manually. In order not to launch a studio each time to launch a virtual device, simply open the Android Virtual Device menu:

We start the device we need and look at the console in the studio, there will be something like:
/Users/spotlight1/Library/Android/sdk/tools/emulator -netdelay none -netspeed full -avd Nexus_5_API_22
We write down where necessary, and now we have a team with the help of which we can launch a virtual device. Then you can write a script to launch a specific application.
Immediately add a useful command:
react-native log-android
In order to see the logs in the terminal.
Components
I will not dwell on how to make the application work at all, about this later. I will also state that I will use examples from
here , be sure to read everything there yourself, but after this article, according to the idea, it will become much easier to grasp, for this I am writing an article.
The component is the basis of the basics. For a start, you should take the component as an Activity or Fragment (but a fragment is closer). Although, a component can be one single button. But later we'll figure it out.
The simplest component:
class Simple Component extends React.Component { render() { return ( <Text>Hello world!</Text>
This component will do something like this:
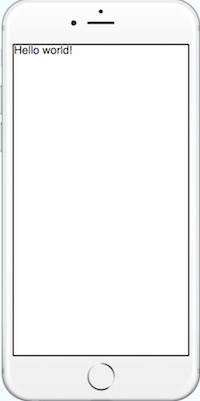
Any component has its own lifecycle, you can thoroughly read about it
here . We are mainly interested only in these:
- constructor () - speaks for itself, this is the component constructor, this is not an obligatory method, but I will return to it later;
- render () is an almost analog method of setContentView (R.layout.activity) from Android
- componentDidMount () is something like the onCreate () method, but in a slightly different way, it is called immediately after the render () method
componentDidMount () is just the place where you need to make requests to the server to get the data you need to display. It is also not a required method.
And everything is simple, but we want to work with the data? The component has 2 data sources:
Props can be perceived as an Intent, which we transmit when we open another activation. This is the data that is transmitted to the
component FROM out .
And now clearly:
class Greeting extends React.Component { render() { return ( <Text>Hello {this.props.name}!</Text>
Happened:

Let's figure it out. Props is called inside the component with the construction:
this.props.paramName
in this example, the parameter is called "name". Well, in curly brackets you need to call in general all the parameters, not just props.
Next, we need to pass props:
<Greeting name='Rexxar' />
Greeting is the name of our component that we call, and then, through the gap, we transmit all the props, in our case it is “name”.
Yes, it is unusual that we clearly do not indicate in the component what props we need (or I didn’t fully understand something), but immediately call them in the code as if they already exist. It is important to note that we can call props anywhere in the component, not only in the render () method, but also that the props cannot be changed, which ones have arrived, and such will remain. A typical application is to transfer the id of an item from the list we poked on to download some information.
Now about the state. State is the variable parameters of the component itself. They can not be transferred somewhere directly, just can not get from outside. From the point of view of Android, this is something like private parameters without setters and getters. State initialized inside the component constructor:
class Stopwatch extends React.Component { constructor(props) { super(props); this.state = {seconds: 0};
The beauty of it is that changing any state parameter results in a call to the render () method. Those. This code is enough so that we get a real stopwatch, the counter will be updated independently.
Well, actually, about the components of all. How does the application itself work? Everything is quite simple, there are two entry points: index.is.js and index.android.js, which is understandable for whom. And this line is the real entry point:
AppRegistry.registerComponent('folderName', () => App);
“FolderName” is the name of the project's root folder; you cannot change it. App is the name of the component that we want to use as the main one. It’s kind of something like a Main Activity.
Ideology of writing code
When I first began to write to the reactor, I stumbled on the most simple questions. Let's say the simplest login screen: 2 EditText and 1 button. I really don’t know why, but at the instinct level I tried to implement it as in Android vanilla, i.e. MVC without any libraries: at the touch of a button, we take the values of these EditText and send it to the right place, having first validated them. Don't even try to think that way.
After a while, a toggle switch clicked in my head and it dawned on me - react native and data binding in Android is almost the same thing (well, not really). However, the approach is practically 1 in 1. Already in my second article I highly recommend reading the data binding for android, if not yet. Otherwise, this article will not help you at all.
To begin with, let's see how xml looks on Android when using data binding:
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Login" android:text="@={model.login}" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Password" android:text="@={model.password}" /> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="@{() -> model.login()}" /> </LinearLayout>
And this is how it will look like on react native:
export default class LoginScreen extends React.Component { constructor(props) { super(props); this.state = { login: null,
So how do you like that? Personally, after that, all the questions about how to write under react native disappeared; here we write not in the imperative style, but in the reactive one. At once I will make a reservation that the TextInput component has a method onChangeText, but for some reason I did not return the entered text.
What's next?
I tried to highlight all the points that I had questions at the time of learning react native, then you should continue with
this , according to the idea, everything should become clearer.
Well, I will write an application for myself, as I receive and solve problems, I will write a couple more articles.
The second part .