We offer you the translation of an article that will be interesting to novice developers.React Native is a JS framework for creating natively displayed iOS and Android applications. It is based on the React JS-library developed on Facebook and designed to create user interfaces. But instead of browsers, it is focused on mobile platforms. In other words, if you are a web developer, you can use React Native to write clean, fast mobile applications, without leaving the comfort of a familiar framework and a single JavaScript code base.
Of course, we have heard promises about universal application development, using frameworks like Cordova or Titanium. What about React Native? In this article we will look at this framework and the features of its work and talk about how convenient it is to use React Native to write iOS and Android applications.
So what is React Native?
Before we dive into the details of development, let's see what React Native is, and we’ll deal with its work a bit.
')
This is just React
React is a JS library for creating user interfaces, usually for web applications. It has been developed on Facebook and distributed under the open source license since 2013. React is widespread, and unlike the larger MVC frameworks, it solves a relatively narrow problem: interface
rendering .
The popularity of React has a number of reasons. It is compact and has high performance, especially when working with rapidly changing data. Due to the component structure, React encourages the writing of modular, reusable code.
React Native is the same React, but for mobile platforms. It has a number of differences: instead of the
div
tag, the
View
component is used, and instead of the
img
tag, the
Image
. The development process has remained the same. You may need knowledge of Objective-C or Java. In addition, mobile development has its own tricks (did I test on different devices? Are the large objects enough to comfortably press them?). Nevertheless, if you worked with React, then React Native will seem to you almost the same, just as comfortable.
He is truly native
The first thing that surprises people in React Native is that it is “truly” native. Other JavaScript-to-mobile-platform solutions simply wrap your JS code in a complimentary web presentation. They can re-implement some native behavior of the interface, for example, animation, but still it remains a web application.
In React, the component describes its own mapping, and then the library processes rendering for you. These two functions are separated by a clear level of abstraction. If you need to render components for the web, then React uses standard HTML tags. Due to the same level of abstraction - "bridge" - for rendering in iOS and Android React Native calls the appropriate API. In iOS, components are drawn into real UI views, and in Android, in native views.

You will write terrible looking code, very similar to standard JavaScript, CSS, and HTML. Instead of compiling into native code, React Native takes your application and runs it using the host platform JS engine, without blocking the main UI thread. You get the benefits of native performance, animation, and behavior without having to write in Objective-C or Java. Other methods of developing cross-platform applications, such as Cordova or Titanium, never achieve this level of native performance or display.
Developer Benefits
Compared to standard iOS and Android development, React Native has much more advantages. Since your application is mostly JavaScript, you can enjoy the many benefits of web development. For example, to see the changes made to the code, you can instantly “update” the application instead of waiting long for the traditional Rebuild to complete. It looks like a gift from above.
In addition, React Native provides a smart error reporting system and standard JavaScript debugging tools, which greatly facilitate the process of mobile development.

Multi-platform processing
React Native elegantly handles different platforms. The vast majority of APIs in the framework are cross-platform, so simply writing the React Native component is enough, and it will work without problems in iOS and Android. Facebook states that in their Ad Manager application,
87% of the code is reused on both platforms.
If you need to write a platform-specific code — due to different rules of interaction in iOS and Android, or because of the advantages of a platform-dependent API — then there will be no difficulty. React Native allows you to assign platform-specific versions of each component, which you can then integrate into your application.
Work with React Native
There is nothing difficult to write a real native application for iOS and Android, using a single code base JavaScript. How to work with React Native?
Start
First of all, you need to install the usual dependencies for iOS and Android development, as well as React Native itself. Good instructions can be found on
the framework
website . React Native is easy to set up. If you already have an updated version of Node installed, you can install the framework using the
npm install -g react-native-cli
.
After installing the dependencies, run the
react-native init ProjectName
. A new project template will be automatically generated.
There is one trick: when developing with React Native, you need OS X. For building iOS apps, Apple forces you to use a Mac, this is an inevitable restriction for most developers. If you plan to write only Android applications, then React Native provides experimental support for development on Windows and Linux.
Conventional React Components
After setting up the environment, we start writing applications.
As already mentioned, React Native is just React with a few major differences. From a browser point of view, React Native components look very similar to React components, but the basic building blocks are different. Instead of tags such as
div
,
img
and
p
, React Native uses basic components like
Text
and
View
. The following example uses the
ScrollView
,
TouchableHighlight
and
Text
components used to work with Android and iOS-specific views. With their help, you can easily create a scrolling view that correctly handles the touch:
If you have not had to deal with JSX - a jumble of HTML-like syntax and JavaScript - then this code may seem strange to you. Both React and React Native are forced to use JSX. Application rendering code is adjacent to JavaScript, which controls its behavior. This often puzzles newbies, but I highly recommend not to reject out of the way, but to try.
Since the React Native components are very similar to the normal React components, it will be very easy for you to switch to this framework.
Style sheets
To simplify rendering and increase its efficiency, as well as to maintain the support of the code, React Native has limited support for CSS. You do not need to learn specific ways of developing views for each platform; instead, it’s enough to master the use of React Native styles.
The main difference is that you can not worry about specific rules. Here, style inheritance is strictly limited and inline syntax is used.
An example of creating a style sheet in React Native:
var styles = StyleSheet.create({ container: { flex: 1, marginTop: 30 } });
Applying style with inline syntax:
<View style={styles.container}> ... </View>
The syntax is easy to read, but if you have previously been involved in web development, then it will seem quite alarming (and there is a reason for that!). From the
presentation by Christopher Shidou you can learn more about CSS problems and their solutions in React.
Customization for mobile development
This is the more difficult part of working with React Native. When working with the framework, you will need all the usual tools of a mobile developer, as well as tools for working with JavaScript: a text editor and debugging tools.
For iOS, you should have Xcode and a mobile emulator running, and for Android, Android Studio and a number of tools that can be run from the command line. Finally, you will need a React Native packer. The choice of text editor for your JavaScript-code is up to you.
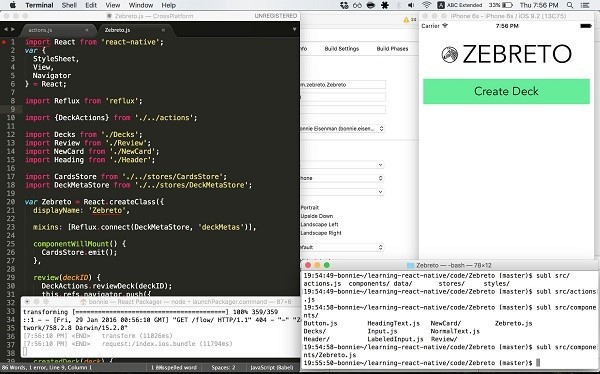
In general, you should have a lot of tools at hand. Sometimes it seems that too much, and the abundance of open windows begins to annoy. On the other hand, React Native at least does not hide from you any standard mobile development process.
Plunge into the native code
React Native provides a JS interface for existing platform APIs. That is, you can write almost the same code as in the case of React, and the React Native bridge will take care of the rest. But what if it is not enough?
In any new framework, inevitable API calls that are not supported by this framework. In the case of React Native, you can write a “native module” for interaction between the host platform and your JS code. Here is an example of the Objective-C module “Hello World”:
To use a native module from JavaScript, you need to query it like any other library:
This can be done if:
- the API you need is not yet supported,
- you want to integrate your React Native components with existing Objective-C or Java code,
- You need to write some kind of high-performance function to handle intensive graphical computing.
Fortunately, React Native is quite flexible and allows you to write so-called "native modules". Even if you didn’t have to work with Objective-C or Java before, writing the “bridge” code would be an excellent exercise in mastering native mobile development.
Deploying the application
In React Native, the deployment is almost the same as in the case of normal applications. That is, it is not easy, since the process of releasing mobile applications is known for its problems.
To create a package ready for deployment, you need to use bundled JavaScript instead of a version that supports live reloading development. To do this in iOS, you need to add one line to the file AppDelegate.m and execute
react-native bundle --minify
. And on Android, you just need to run
./gradlew assembleRelease
. The rest of the packaging process on both platforms is the same as in the case of conventional mobile applications. As a result, you will receive a package that is ready for placement in the appropriate app store.
Usually, approving the placement of React Native applications in stores takes as much time as it does for “traditional” applications. For example, the Zebretto app was approved in the AppStore for two weeks, and in the Play Store - less than one day.
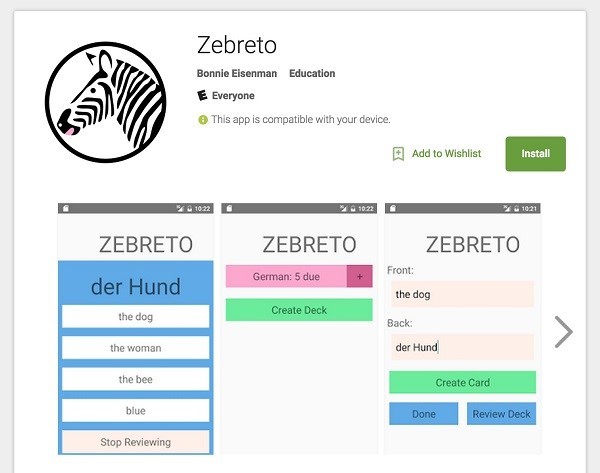
Apple allows apps to update themselves if the changes concern only JavaScript. This eliminates a number of problems during deployment. Microsoft recently released the
CodePush SDK, allowing developers using React Native to instantly release updates. Very seductive function, probably soon more and more applications will start using it.
Conclusions and recommendations
If you have web development experience, then you should like React Native. This framework turns any web developer into a potential developer of mobile applications, allowing you to significantly improve the process of their creation.
React Native has its drawbacks. This is a relatively new project, and the problems of all young libraries are peculiar to it: some functions are missing, the optimal methods of use have not yet been developed. From release to release, major changes are being introduced, although there are few of them and they are limited.
Nevertheless, React Native is already a fairly mature project, whose advantages are more significant than disadvantages. With this framework, you can use a single code base to create applications for iOS and Android, without sacrificing either quality or performance. Even if you are not experienced in JavaScript, you are unlikely to dispute the benefits of a faster development cycle and almost one hundred percent reuse of code. And since React Native allows you to move to a “normal” development, if necessary, you are not hampered by the limitations of the framework. In general, React Native provides high-quality cross-platform mobile development, and this tool should be seriously looked at.