What to do frontend? The surest way to find the answer is to try the best libraries yourself. Ideally, it would be nice to start with the simplest and understand how the mysterious for the uninitiated designs turn into pages that are ready for conclusion. Then, armed with an understanding of the basics, you can read the documentation in a meaningful way and complicate your own experiments until the answer seems obvious.
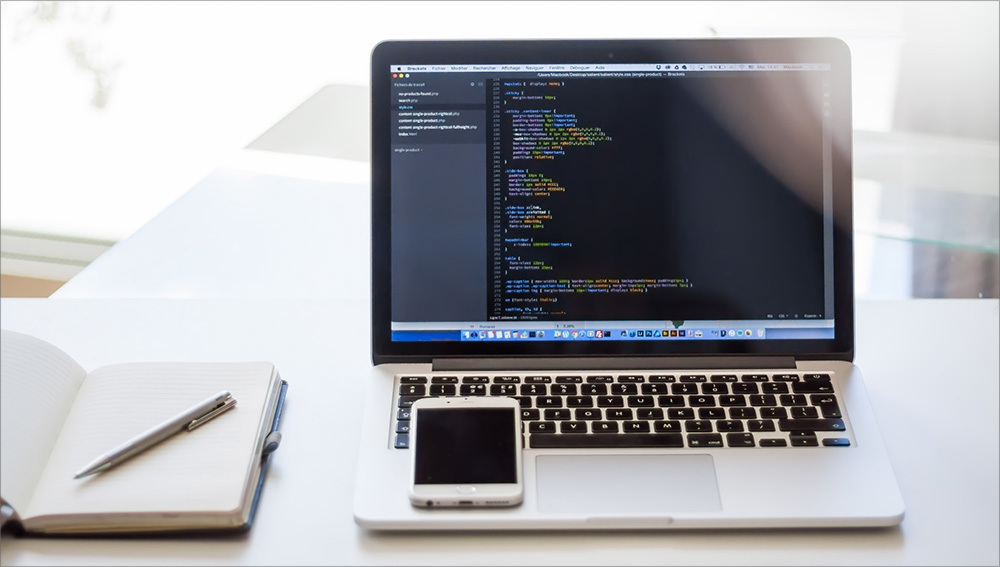
Today we will talk about React.js and Vue.js. This is one of the most popular JavaScript libraries in the world. Take a look at
this list, look at their GitHub repositories. Both have impressive capabilities and are used to create user interfaces. Working with them is quite simple, the main thing is to immediately understand what is happening, to make the right first step. As a matter of fact, this material is devoted to this first step in development using React and Vue.
At first glance, these libraries are very similar. Indeed, they serve the same purpose. Similarities are not limited to this. In particular, in the documentation for Vue.js there is a section dedicated to comparison with other frameworks. A special place, precisely because of the many common features, is occupied by the
comparison with React . However, we are more interested in the differences, and, concerning the practical aspects of the work. Namely, this is how exactly the user interface of the application is described using these libraries.
')
Allow yourself to quote the documentation for Vue, which, by the way, was prepared with the participation of the React community: “In React, all components describe their UI using render functions using JSX, a declarative XML-like syntax that works inside JavaScript.” In fact, That React mechanisms help embed HTML markup directly into JavaScript code. Vue basically takes a different approach. It uses templates that are located in HTML files and look like regular HTML markup. At the same time, JSX-descriptions on the web pages do not fall.
In order to deal with the basics of React and Vue, consider the solution to a simple task of outputting to the list page. First - a couple of words about how this is done, so to speak, manually.
Html and nothing else
Our task is to bring to the web page (add to the DOM) a list of names of the recently recruited employees of a certain company.
If you do this using only HTML, you first need to create a regular HTML file (let's call it index.html), in which, besides the service tags, there will be such a construction:
<ul> <li> John </li> <li> Sarah </li> <li> Kevin </li> <li> Alice </li> <ul>
There is nothing remarkable, this is a regular HTML list. Until there are only a few elements in it, manual editing of the labor code will not be. But what if we are talking about a list of several hundred or thousands of elements, without even considering that such a list may need, for example, a dynamic update? Without specialized tools, such as React and Vue, to cope with this amount of data is very difficult.
Now let's take a look at how to solve the same problem with React tools.
React JSX
So, we output the list by means of React.
The first step is to create another index.html file. But in it, instead of the tags forming the list, add the usual element
div
. It will serve as a container into which that which will be formed by means of React will be displayed.
This element must have a unique
ID
so that React can find it and work with it. In Facebook (if someone does not know - React comes from there), the key word “root” is usually used for identifiers of such elements, so we will do the same.
<div id='root'></div>
Go to the most important. Create a JavaScript file that will contain all the React code. Let's call it app.js. In it, we will write something that will allow us to list the new employees in the DOM using JSX.
First you need to create an array with the names of employees.
const names = ['John', 'Sarah', 'Kevin', 'Alice'];
Now you need to create an element React, which dynamically displays the entire list of names. Manually writing code to display each one is unnecessary.
const displayNewHires = ( <ul> {names.map(name => <li>{name}</li> )} </ul> );
The most important thing to pay attention to is that you don’t need to create separate
<li>
elements yourself. It is enough to describe how they should look, a React will do the rest. This is a very powerful mechanism, which is great for solving the above-mentioned “thousand list” problem. With the growth of data, the advantages of React over manual layout become quite obvious. Especially if the
<li>
elements are more complex than the ones we used here. The last piece of code that is needed to display data on the screen is the
ReactDom.render
function.
ReactDOM.render( displayNewHires, document.getElementById('root') );
Here we tell React that the
displayNewHires
content should be
displayNewHires
in a
div
element with the
root
identifier.
const names = ['John', 'Sarah', 'Kevin', 'Alice']; const displayNewHires = ( <ul> {names.map(name => <li>{name}</li> )} </ul> ); ReactDOM.render( displayNewHires, document.getElementById('root') );
Here it is important not to forget that, in front of us is the React code. That is, before execution, all this will be compiled into ordinary JavaScript.
'use strict'; var names = ['John', 'Sarah', 'Kevin', 'Alice']; var displayNewHires = React.createElement( 'ul', null, names.map(function (name) { return React.createElement( 'li', null, name ); }) ); ReactDOM.render(displayNewHires, document.getElementById('root'));
That's all. It turned out React-application, which displays a list of names of employees from the array. Nothing special, but this simple example should give you an idea of ​​what React can do. And how to do the same with Vue?
Vue Templates
Here, as last time, we will write an application that allows you to create a web page with a list of names.
Create another index.html. Inside the file we place an empty
div
element with the
root
identifier. It is worth noting that an identifier can be assigned to any element, it does not play a special role. The main thing is that this
ID
coincides with the one that will be used in the code.
The
div
element will play the same role as in the React application. It will tell the JS library, this time Vue, which part of the DOM to access when the output to the page begins.
After the HTML file is ready, it's time to create a JavaScript file that will contain Vue code. Let's call it, as before, app.js.
Now it's time to talk about exactly how Vue displays data on the page.
Vue, when it comes to manipulating the DOM, uses a template approach. This means that in the HTML file the
div
element will not be completely empty, as was the case with React. In fact, a considerable part of the code will be placed in the HTML file.
In order to better understand this, let's remember how to create a list of names using plain HTML.
The list is represented by a
<ul>
tag containing a number of
<li>
elements. In Vue, you need to do almost the same thing, but with some changes. So, create the
<ul>
element.
<ul> </ul>
Now, inside this element, create one empty
<li>
.
<ul> <li> </li> </ul>
So far, everything is quite familiar. Edit
<li>
to add the Vue directive, which looks like a tag attribute.
<ul> <li v-for='name in listOfNames'> </li> </ul>
Custom directives are an approach used in Vue to add JavaScript functionality directly to HTML. At the beginning of the directives are the characters
v-
, followed by a descriptive name that allows you to understand the roles of the directives. In this case, we have a
for
loop. For each name in
listOfNames
we want to copy the
<li>
element and replace it with a new
<li>
element containing this name.
Now, in order to make the code workable, only a very small amount remains. Namely, what is already written, will allow you to create an element
<li>
for each name in the list. But we have not yet informed the system that this element should contain the name from the list. In order to fix this, you need to add to the
<li>
element a structure whose syntax is similar to Mustache templates. Perhaps you have already seen this in other JS-libraries.
<ul> <li v-for='name in listOfNames'> {{name}} </li> </ul>
The
<li>
element is ready to go. Now each of the elements of the list formed by the Vue tools will output the name from
listOfNames
. It is worth remembering that the word "name" in this case is chosen arbitrarily. With the same success, you can use any other, say, «item». Whatever the keyword does, it serves as a placeholder field that will be used when processing the list of names.
It remains only to create a data set and initialize Vue in the application. To do this, create a new instance of Vue. Assign it to the
app
variable.
let app = new Vue({ });
The Vue object takes several parameters. The first, and perhaps most important, is
el
(that is, the element). It tells Vue where exactly in the DOM there is a place where new elements should be added. This is very similar to how React works.
let app = new Vue({ el:'#root', });
The final step is to add data to the Vue application. In this library, data is transferred to the application through an instance parameter of Vue. In addition, each instance of Vue can only have one parameter per view. Although there are quite a few parameters, in our example, the focus can only be given to two - this is the above-described
el
, and the
data
parameter.
let app = new Vue({ el:'#root', data: { listOfNames: ['Kevin', 'John', 'Sarah', 'Alice'] } });
The
data
object now contains an array of
listOfNames
. And when you need to use this dataset in an application, you only need to call it using a directive. It's pretty simple. Here is what the finished application looks like.
HTML:
<div id="root"> <ul> <li v-for='name in listOfNames'> {{name}} </li> </ul> </div>
Javascript:
new Vue({ el:"#root", data: { listOfNames: ['Kevin', 'John', 'Sarah', 'Alice'] } });
Conclusion
Now you know how to create simple applications using React and Vue. We hope you, with the help of our simple examples, have taken the first steps in the areas of development using React and Vue, and are ready to move on in search of "your" front-end library.
In the end, I want to say that both libraries offer an excellent range of features. Usually, although it is probably a matter of habit, Vue is somewhat easier to use. It is worth noting that Vue supports JSX, but this approach to creating Vue applications is used infrequently.
In any case, both React and Vue are powerful tools, any of which allows you to create great web applications, and, whatever you choose, you will definitely not lose.
Dear readers! What do you use for front end development?