Localization is a process when you create support for other languages for your application. Often you first make an application with an English-language interface and then localize it into other languages, for example, Japanese.
The localization process is laborious, and its steps slowly change as Xcode is updated. This post explains each step based on the
latest version of Xcode (7.3.1).
Transferred to AlconostBefore starting localization work, be sure to select the Use Base Internationalization option.
')

What is basic internationalization?
When you create a project, Xcode automatically generates the resources and file structure for the default language.

This is the so-called base language. If you are making an application for the global market, in your basic language resources there will usually be English texts.
Adding new localization
So, we have a default basic language resource structure. Let's add support for a new language.
Select your project file in the Navigator (Project Navigator), then select your project in the lists of projects (Project) and target parameters (Targets). Open the “Information” tab (Info) and click the “+” button under the “Localizations” block (Localizations). Now select the language you want to support from those presented in the drop-down list.
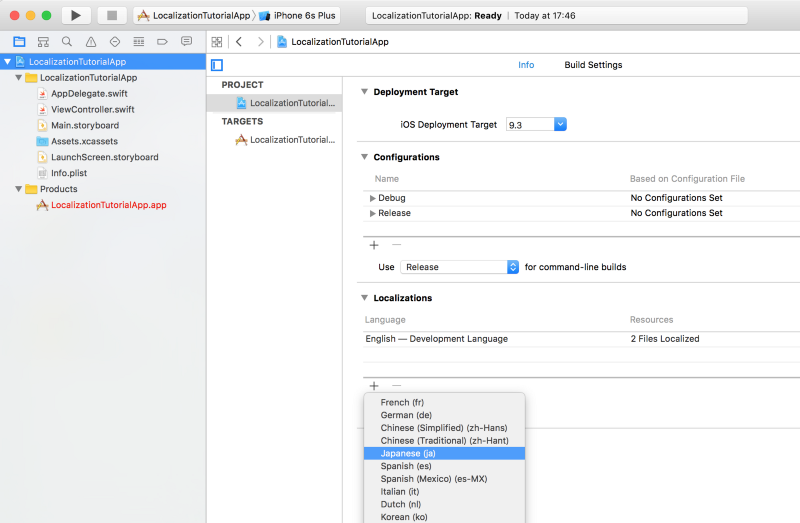
Xcode will open a dialog with a set of resources you need to add for the new language. Clicking the “Finish” button will create these files in the folder of the new language project called [new language] .lproj. (This example adds support for the Japanese language, respectively, the ja.lproj folder is created.)
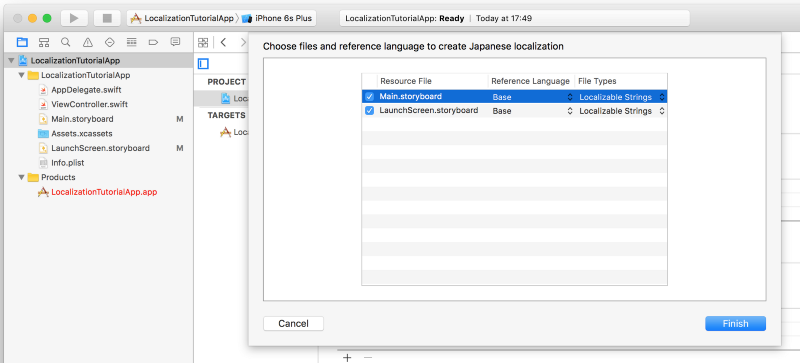
Now we have the file structure in the project folder, as in the example below.
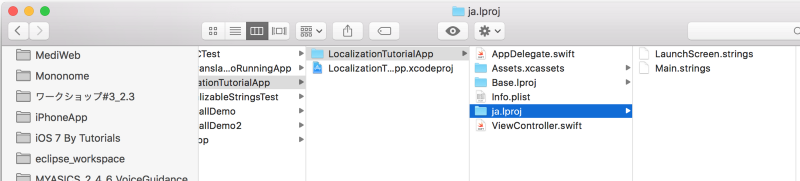
Where is the Localizable.strings file?
The Localizable.strings file is where you add the translation data as key / value pairs.
Early versions of Xcode by default generated the Localizable.strings file, copies of which could be easily created for other languages.
Recent versions of Xcode do not create the default Localizable.strings file.
To add the Localizable.strings file, select File → New → File, and then the Strings (Strings File) file on the Resource tab for iOS, set the name Localizable.strings and create the file.
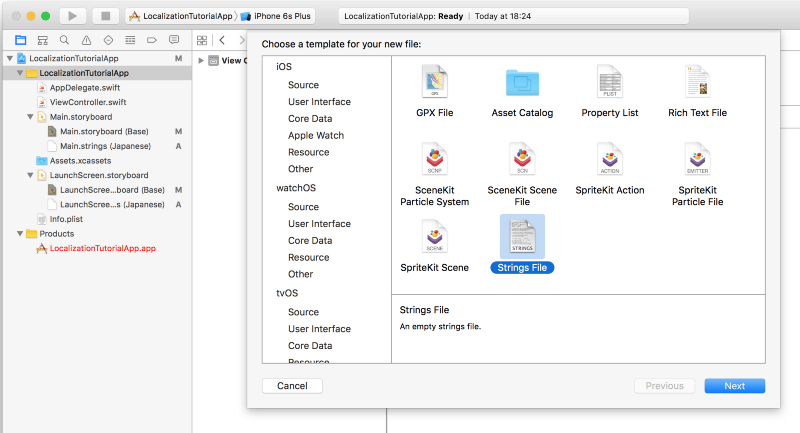
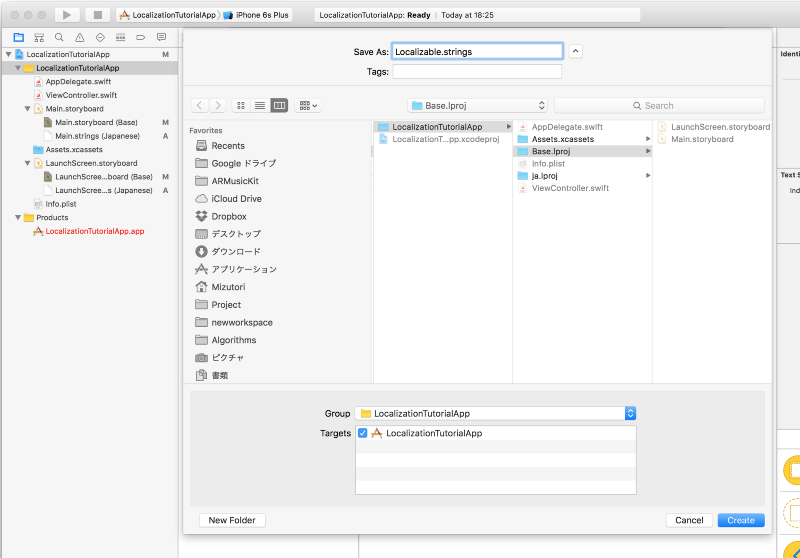
You now have the Localizable.strings file for the base (Base) language, as in the example below.
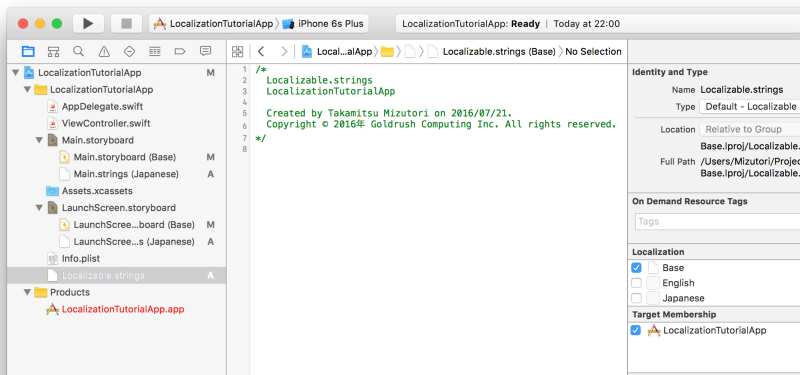
To add Localizable.strings for Japanese, select Japanese (Japanese) in the browser (File Inspector). This will create a new Localizable.strings file in the ja.lproj folder.

Now we have two Localizable.strings files: one in the Base.lproj folder, the other in the ja.lproj folder.
Let's add the words and phrases used in the application to the Localizable.strings file of the base language.
Below is an example of adding “Welcome” = “Welcome”;
The left side is the so-called key, with the help of which the NSLocalizedString method then extracts the text (value) from the right side. This is the type of data - key / value pairs.
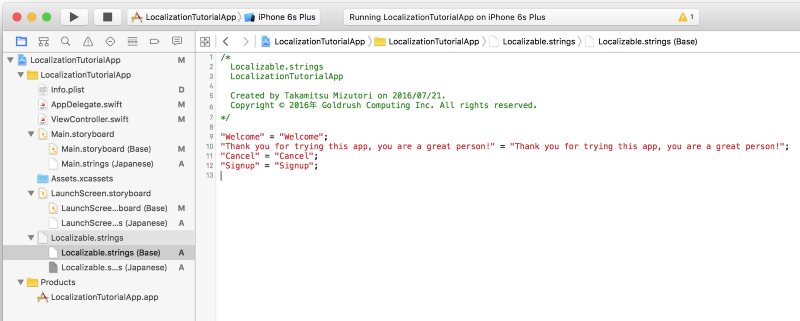
Below is an example of the NSLocalizedString method. We set the key as the first parameter of the method, thus allowing it to extract the corresponding value from the Localizable.strings file and return it. In this example, we get localized strings for the header, message, and notification buttons.
let alertTitle = NSLocalizedString("Welcome", comment: "") let alertMessage = NSLocalizedString("Thank you for trying this app, you are a great person!", comment: "") let cancelButtonText = NSLocalizedString("Cancel", comment: "") let signupButtonText = NSLocalizedString("Signup", comment: "") let alert = UIAlertController(title: alertTitle, message: alertMessage, preferredStyle: UIAlertControllerStyle.Alert) let cancelAction = UIAlertAction(title: cancelButtonText, style: UIAlertActionStyle.Cancel, handler: nil) let signupAction = UIAlertAction(title: signupButtonText, style: UIAlertActionStyle.Default, handler: nil) alert.addAction(cancelAction) alert.addAction(signupAction) presentViewController(alert, animated: true, completion: nil)
When launching the application, we see a notification with texts in English.
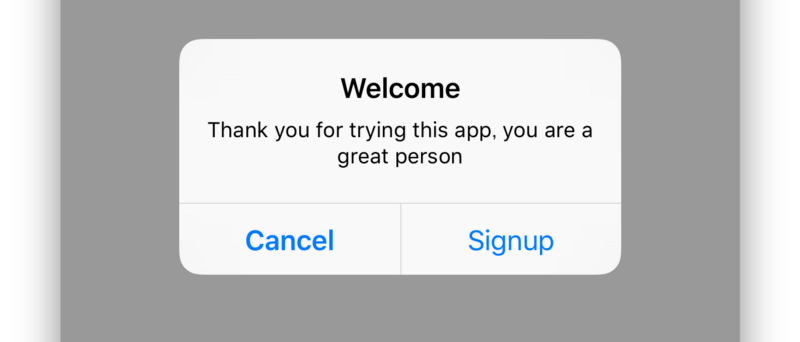
Next step: add Japanese texts to the Localizable.strings file in the ja.lproj folder. We use the same keys, but replace the values with the corresponding Japanese translations.
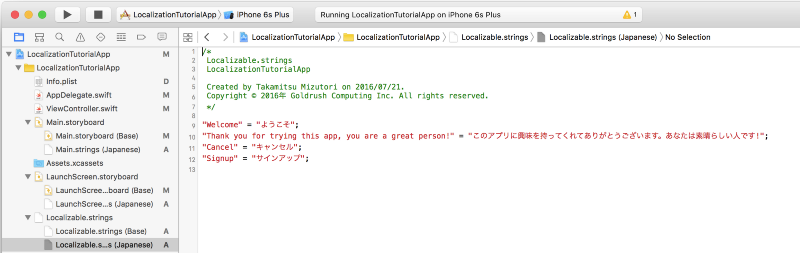
Then, in the iOS simulator, we switch the phone language to Japanese, start the application and see a notification with texts in Japanese.
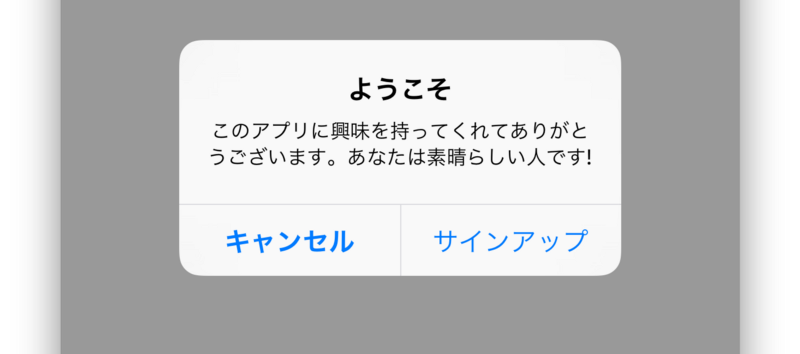
Switching the phone language each time you need to check the localization results is too time-consuming. Xcode provides a convenient way to switch languages only for the application when it is running in the iOS simulator.
To do this, select Edit Scheme from the drop-down menu in the upper left corner of the Xcode window and change the application language (Application Language) from the system language to Japanese (Japanese). (If lost, look at the screenshot below.)
This configuration will not switch the phone language in the simulator, but will change the language of the environment in the application to the one you specified. This is useful when you add multiple languages and want to switch between them to check the localization results.
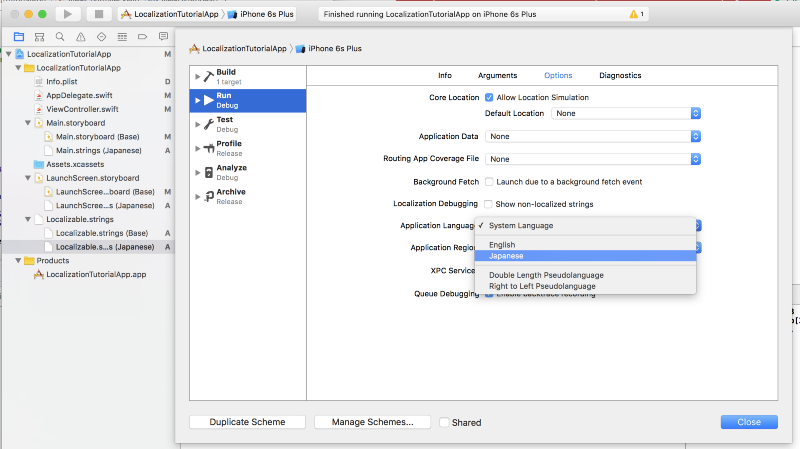
Localization of storyboards
Well, now we know how to extract localized texts using NSLocalizedString and how to prepare the data in the Localizalbe.strings files.
This is enough to programmatically display localized texts to users.
The next step is to support the localization of texts specified in Storyboards, for example, the names of buttons. If you set the names of buttons or shortcuts in the storyboards and do not change these texts programmatically in the view controllers (ViewControllers), you will have to localize your .storyboard files.
To add translation data to the words used in storyboards, first select the storyboard file in the Navigator (Project Navigator), then find and add Japanese (Japanese) to the browser (File Inspector) on the right. This will create a [StoryboardFileName] .strings file in the ja.lproj folder. In the example below, the file Main.strings (Japanese) is created for the Main.storyboard file.

In the file Main.strings you will see something similar.
/* Class = "UIButton"; normalTitle = "Get Started"; ObjectID = "qs4-6I-gUp"; */ "qs4-6I-gUp.normalTitle" = "Get Started";
Replace the “Get Started” part with the appropriate Japanese phrase.
/* Class = "UIButton"; normalTitle = "Get Started"; ObjectID = "qs4-6I-gUp"; */ "qs4-6I-gUp.normalTitle" = "始める";
Run the application. Make sure the button name is localized correctly in Japanese.
What is wrong with [Storyboard] .strings files
The only problem is that the Main.strings file is not updated when you add new user interface components to the storyboard file.
Because you always have to first determine the interface components and only then create the Main.strings, including, for example, the Japanese localization in the browser (File Inspector).
Localization of the application name
To localize the name of the application or anything else from the Info.plist file, create the InfoPlist.strings file.
Choose Go to File → New → File, then the Strings (Strings File) file on the Resource tab for iOS and set the name InfoPlist.strings. Save the InfoPlist.strings file in the Base.lproj folder. (Xcode will now assign this InfoPlist.strings file to the base language.)
Usually we localize these two values in the info.plist file:
- CFBundleDisplayName - the name of the application as it is displayed on the main screen;
- NSHumanReadableCopyright - copyright information (for example: 2014, Goldrush Computing Inc. All rights reserved).
Provide the application name and copyright information for these keys, as in the example below.
"CFBundleDisplayName" = "MyApp"; "NSHumanReadableCopyright" = "2016 Goldrush Computing Inc. All rights reserved.";
Then, in the browser (File Inspector), select Japanese to add InfoPlist.strings to the ja.lproj folder (for this, the InfoPlist.strings file must remain selected).

In the InfoPlist.strings (Japanese) file, replace the values with Japanese translations, as in the example.
"CFBundleDisplayName" = "マイアプリ"; "NSHumanReadableCopyright" = "2016年 Goldrush Computing Inc. All rights reserved.";
Run the application and see if the name of your application is localized in Japanese.

That's the whole process of localizing the application to another language.
New way to localize applications
I wrote a
lot of applications and worked a lot on localization. It takes a lot of work time, so I was always looking for ways to simplify it. Finally, we created a tool that facilitates the localization process — it is called InAppTranslation. If you're interested, go to our website -
inapptranslation.com .
About the translatorThe article is translated in Alconost.
Alconost is engaged in the
localization of applications, games and websites in 60 languages. Language translators, linguistic testing, cloud platform with API, continuous localization, 24/7 project managers, any formats of string resources.
We also make
advertising and training videos - for websites selling, image, advertising, training, teasers, expliners, trailers for Google Play and the App Store.
Read more:
alconost.com