The author of the material tells how using Google-tables to create a virtual machine that generates Fibonacci numbers.Recently, I noticed that Google Docs has a fairly comprehensive system of scripts called
Apps Script . It allows you to write some pretty useful things in JavaScript:
- Run code in response to events such as opening documents or changing cells
- Create custom table functions for formulas in Google Spreadsheets
- Use services such as Google Translator to translate text or Gmail to send email.
- Add new menu items to the Google Docs interface using custom features
Naturally, for this reason I had to create something interesting. Look at this: Google spreadsheet virtual machine that generates
Fibonacci numbers !
')
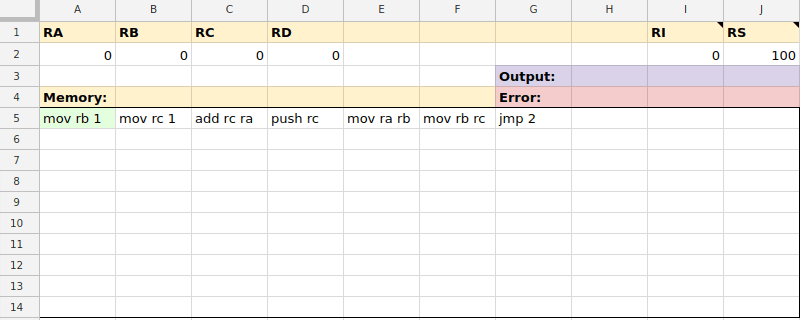
How does it work
A VM has a memory area of ​​100 cells, numbered from 0 to 99. Each cell can contain a command or an integer value.
There is also a stack that starts at the bottom of the memory area and grows up.
This is what a VM sheet looks like when it is empty:
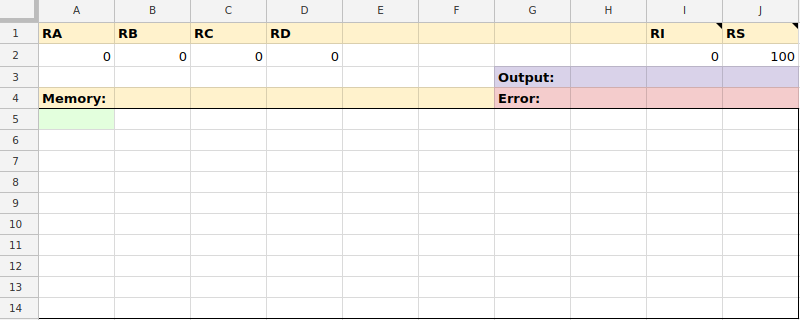
Note the following:
- RA, RB, RC and RD are general registers.
- RI is a pointer to a command. It indicates the next command to be executed in the memory area, which is highlighted in green.
- RS is a pointer to the stack. He points to a memory cell at the top of the stack. It is highlighted in blue.
- Output Displays the output of the program.
- Error (Error) displays any errors that occur when analyzing or executing a command.
- Memory (Memory) is an area of ​​100 memory cells.
To run a command, Apps Script checks the RI value in the background to understand which command should be executed next. It reads the command in the cell pointed to by RI and analyzes it.
There are commands for moving data between memory and registers, managing the stack, or executing conditional commands.
After the command is executed, the RI value is incremented to point to the next cell in memory.
Using
There is a special menu called "Computer" (Computer) with some functions that are used to control the VM:

- Run (Run) starts the current program before it ends or an error is detected.
- Step (Step) runs one command, and then pauses.
- Reset clears all registers and output fields, thus preparing the program for restarting.
- Load Factor Program (Load Factorial Program) loads factorial example from another table.
- Loading the Fibonacci Program loads the Fibonacci example from another sheet.
Teams
There are several implemented commands:
Are common- mov dst src copies the value from src to dst.
Mathematical- add dst src adds dst to src and stores the result in dst.
- sub dst src subtracts src from dst and stores the result in dst.
- mul dst src multiplies dst by src and stores the result in dst.
Stack operations- push src pushes src onto the stack.
- pop dst retrieves the value from the top of the stack and saves it to dst.
Transitions and conditional commands- jmp target goes to the command in the cell referenced by target.
- jl cmp1 cmp2 target compares cmp1 with cmp2. If cmp1 is less than cmp2, ​​execution proceeds to target.
Functions- call target is a function call. He loads the current command pointer onto the stack so that it can be returned later, and then goes to the target.
- ret returns a function. It retrieves the value from the stack and passes to it.
Other- output src writes src to Output: the interface section.
- end terminates the program.
Addressing Methods
Operands in the above commands can take several forms:
Immediates are literal values ​​embedded in the command. Examples: 7 and 123. For example, to copy the value 7 to the register ra:
mov ra 7
Registers refer to registers by name. Examples: ra, rb, rc. To copy a value from rc to rb:
mov rb rc
Memory refers to the value inside the memory location. Examples: $ 0, $ 10, $ 99. To copy a value from ra to the first memory location:
mov $0 ra
To copy the value from the last memory location to rd:
mov rd $99
Indirect refers to the value pointed to by the memory cell. Examples: @ 15, @ 50. Therefore, if memory cell 10 contains the value 20, and memory cell 20 contains the value 30, you can copy the value 30 into ra as follows:
mov ra @10
He checks memory cell 10 to find the value 20. Then he accesses memory cell 20 to find the value 30, and copies this value to ra.
Recursion
You can use the stack and the call and ret commands to make recursive calls. Here is an example that uses recursion to generate the factorial of the number 5:

Code starting with jl ra 2 50 is a function that accepts an input value in ra and returns the result in rd. It calls itself recursively to calculate the factorial of a value in ra.
How to get a copy
If you want to play with it yourself, you can make a copy of it
here .
You can see the Apps Script code by selecting “Tools” (Tools), and then “Script Editor”.
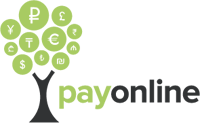