The second part of the
Intel Software Guard Extensions (Intel SGX) series of tutorials is a general description of the application that we will develop: a simple password manager. Since we are creating this application from scratch, it is possible to foresee the use of Intel SGX from the very beginning. This means that in addition to the application requirements, we will look at how the requirements for the Intel SGX and the overall application architecture affect each other.
Read the
first part or list of all published educational materials in the article
Introducing a series of educational materials on Intel Software Guard Extensions .
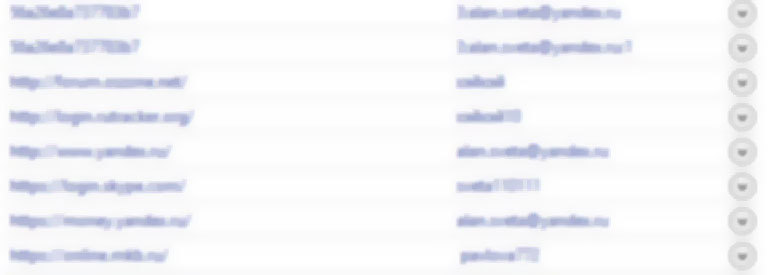
Password Managers Short Description
Users, as a rule, know what password managers are and what they serve for, but it is always helpful to once again go through general information before working on the details of the application device.
')
The main goals of the password manager:
- Reducing the number of passwords that the user needs to remember.
- Providing end users with the ability to create stronger passwords than they could think of on their own.
- Improve the usability of using different passwords for each account.
Password management is a topical issue for Internet users, and over the years several studies have been undertaken to address this problem. A
Microsoft study published in 2007 — almost a decade ago — indicated that each user had an average of 25 accounts for which passwords were required. Later, in 2014,
Dashlane specialists calculated that their users in the United States had an average of 130 accounts, and the average number of accounts per user worldwide was about 90. However, this does not end the problem: people as a rule,
strong passwords are rarely chosen , often
using the same password on multiple sites , which caused
scandalous attacks by intruders. All these problems are caused by only two main factors: first, it is often difficult for people to remember strong passwords that are resistant to cracking; secondly, if you use not one password, but a set, then the situation becomes even more complicated, because now, apart from the actual password, you also need to remember which account each password is associated with.
When using a password manager, it’s enough to remember just one very strong passphrase in order to gain access to the password database (password storage). After logging in to the password manager, you can find all the passwords stored there, copy and paste them into the authentication fields on the necessary sites. Of course, the main vulnerability of the password manager is the password database: this is the attacker's favorite target, since it contains all user passwords. Therefore, the password database is encrypted using strong encryption algorithms, and the main user passphrase is used to decrypt the data they contain.
Our goal in this tutorial is to create a simple password manager that provides the same basic functions as similar commercial products, while observing security recommendations and using this program as an exercise to learn Intel SGX. I decided to call this training password manager “Tutorial Password Manager with Intel Software Guard Extensions” (yes, it’s not easy to pronounce, but the name speaks for itself). This program is not intended to be used as a commercial product and, of course, will not contain all the protective mechanisms inherent in commercial solutions, but this level of detail is not required for training.
Basic application requirements
Basic application requirements will help limit the scope of your application so that you can focus on integrating Intel SGX, and not on the intricacies of designing and developing an application. Let me remind you that we are not faced with the task of creating a commercial product: the Tutorial Password Manager with the Intel SGX does not need to be able to run on multiple operating systems or on all possible CPU architectures. Only a valid starting point is required.
Therefore, the basic requirements for the application are:
Requirements for the solution and principles of operation | - The application should work on all platforms regardless of whether they support Intel SGX.
- A 64-bit version of Microsoft Windows Vista * or later is required (a 64-bit version of Windows 10 or later is required for Intel SGX support).
- An Intel processor that supports Intel Data Protection Technology (Intel DPT) with a secure key is required.
- The application should not rely on third-party libraries and utility programs.
|
The first requirement may seem strange if we consider that this series of training materials is devoted to the development of applications using Intel SGX, but applications designed for the real world should provide for the possibility of installation on outdated systems. For some applications, it may be advisable to allow execution only on platforms that support Intel SGX, but in the Tutorial Password Manager we have chosen a more flexible approach. A platform that supports Intel SGX will get a more secure runtime, but on other platforms the program will still work. This usage model is quite suitable for a password manager, since users can synchronize their passwords with other, older systems. In addition, it is an opportunity to learn how to implement double branches of code.
The second requirement gives us access to certain encryption algorithms in the non-Intel SGX-supported code branch and to some of the libraries we need. The requirement to use a 64-bit operating system simplifies application development by accessing 64-bit native code types. In addition, the speed of some encryption algorithms optimized for 64-bit code is improved.
The third requirement gives us access to the RDRAND instructions in the code branch without support for Intel SGX. This greatly simplifies the generation of random numbers and provides access to a source with high entropy. Systems that support the RDSEED instruction can use it. (For information about RDRAND and RDSEED instructions, see the
Intel Random Number Software Implementation Guide .)
The fourth requirement supports the utmost brevity of the list of software required by the developer (and user). No third-party libraries, platforms, applications, or utilities are required to download and install. However, this requirement does not have a very pleasant side effect: if we abandon third-party platforms, then we are left with only four options for creating a user interface. These options are:
- Win32 APIs
- Microsoft Foundation Classes (MFC)
- Windows Presentation Foundation (WPF)
- Windows forms
The first two options are implemented using native / unmanaged code, and the last two options require .NET *.
User interface platform
We will develop a graphical user interface for the Tutorial Password Manager program using Windows Presentation Foundation in C #. This decision will affect our requirements as follows:
Requirements for the solution and principles of operation | - WPF based graphical user interface.
- Microsoft .NET Framework 4.5.1 or later.
A 64-bit version of Microsoft Windows Vista * or later is required (a 64-bit version of Windows * 10 or later is required for Intel SGX support).
- A 64-bit version of Microsoft Windows Vista * with Service Pack 2 (SP2) or later is required (Intel® SGX support requires a 64-bit version of Windows * 10 or later).
|
Why decided to use WPF? Mainly due to the fact that it simplifies the design of the user interface and introduces the level of complexity we need. In particular, since the interface relies on the .NET Framework, we have the opportunity to discuss the mixing of managed code and, in particular, high-level languages ​​with the enclave code. Please note that the choice of WPF instead of Windows Forms was completely arbitrary: our environment would work in either case.
As you may remember, enclaves must be written in native C or C ++ code, and bridge functions interacting with an enclave must be native C functions (but not C ++). Both the Win32 and MFC APIs make it possible to develop a password manager using fully native C / C ++ code, but the development tasks associated with these two methods are useless for developers looking to learn how to develop applications using Intel SGX. Using a graphical user interface based on managed code, we get not only the benefits of integrated development tools, but also the opportunity to discuss specific issues that are useful to developers of Intel SGX applications. In short, you are not here to learn how to use MFC or Win32, but you may be interested in how to connect .NET with enclaves.
To bind managed and unmanaged code, we will use C ++ / CLI (that is, C ++ modified for Common Language Infrastructure). This greatly simplifies data packaging and is so convenient that many developers call this method “IJW” (It Just Works - it just works).
Figure 1. Minimum component structures for Intel Software Guard Extensions for native code and C #.In fig. Figure 1 shows the effect on the minimum mix of Intel SGX application components when moving from native code to C #. When using only native code, the application layer can directly interact with the enclave DLL library, since the functions of the enclave bridge can be embedded into the executable file of the application. In a mixed application, the functions of an enclave bridge will need to be separated from the managed code block, since these functions must be completely own code. On the other hand, a C # application cannot directly interact with bridge functions, and in the C ++ / CLI model, this means that you need to create another intermediate level: a DLL library that transfers data between the managed C # application and the enclave DLL library only from your own code.
Password Store Requirements
Password Manager is based on a password database, which we will also call the password store. This is an encrypted file that will contain information about end-user accounts and passwords. The basic requirements for our educational application are:
Requirements for the solution and principles of operation | - Password store should be portable.
- The storage must be encrypted during storage.
- All encryption requires the use of authenticated encryption.
|
The requirement of storage portability means that we should be able to copy the storage file to another computer and still access its contents regardless of whether the other computer supports the Intel SGX extensions. In other words, the capabilities of users should be the same: the password manager should work on any computer (of course, if the hardware and operating system meet the specified system requirements).
Storage encryption during storage means that the storage file must be encrypted when it is not in active use. In this case, the storage should be encrypted on the disk (if there was no portability requirement, then the encryption requirement could be solved using the sealing function in the Intel SGX), and should not be in the decrypted form in the RAM longer than necessary.
Authenticated encryption ensures that the encrypted storage has not been changed after encryption. Also, thanks to this, we get a convenient means of checking the user's password phrase: if the decryption key is incorrect, the decryption will fail to verify the authentication tag. In this case, we do not need to study the decrypted data to ensure that they are correct.
Passwords
Any account information is confidential for a variety of reasons, and not least the information about which accounts and sites you can attack, but passwords are probably the most important information in the repository. Knowing exactly what needs to be attacked is, of course, good, but if you know passwords, then you don’t need to attack at all, which is much better. Therefore, we introduce additional requirements for passwords stored in the repository:
Requirements for the solution and principles of operation | - Account passwords must be encrypted inside the vault.
- Decryption of account passwords occurs only on demand.
|
This is nested multi-level encryption. The passwords of all user accounts are encrypted when placed in storage, and the storage itself is encrypted when written to disk. This approach reduces the vulnerability of passwords after decrypting the repository. It is advisable to decrypt the entire repository so that the user can view all their credentials, but displaying all passwords in plain text is hardly acceptable.
The account password is decrypted only when the user requires it. This limits the vulnerability of the password in the RAM and on the screen.
Encryption algorithms
Since our requirements for encryption are already defined, it is time to choose certain encryption algorithms, and in this regard, the existing requirements for our application significantly limit the available options. The Tutorial Password Manager program should work both on platforms that support Intel SGX and without Intel SGX, and third-party libraries are not allowed. This means that you must select the algorithm, key size, and size of the authentication tag, which are supported in the Windows CNG API and in the trusted encryption library Intel SGX. In practice, this means that only one possible algorithm remains at our disposal: AES-GCM with a 128-bit key. This is probably not the best encryption mode for use in an application, especially since the
128-bit GCM authentication tag's strength is less than 128 bits , but for our purposes this will be enough. Remember: our task is to create not a commercial product, but a tutorial on using Intel SGX.
The choice of GCM affects other encryption characteristics in our application, in particular, the length of the initialization vector (12 bytes is the optimal value for this algorithm) and the tag of authenticity.
Requirements for the solution and principles of operation | - AES-GCM encryption algorithm
- 128-bit key
- 128-bit authentication tag
- No additional validated data (AAD)
- 96-bit initialization vectors (IV)
|
Encryption keys and user authentication
After selecting the encryption algorithm, you can go to the encryption and user authentication key. How does a user authenticate with Password Manager to open their repository?
The easiest way is to generate an encryption key directly from a password phrase or user password using the key generation function (KDF). This simple approach works well, but it has one major drawback: if the user changes the password, the encryption key will also change with it. Instead, we use a more common approach and encrypt the encryption key.
In this case, the primary encryption key is randomly generated based on a source with high entropy and never changes. The passphrase or user password is used to generate a secondary encryption key, and this secondary key is used to encrypt the primary key. This approach has several important advantages:
- Data will not have to re-encrypt when changing the password or pass phrase of the user.
- The encryption key never changes, so you can, for example, write it on paper in hexadecimal format and store it in a physically secure place. In this case, the data can be decrypted even if the user forgets the password. Since the key never changes, it is enough to write it down only once.
- Theoretically, you can provide data access to multiple users. Each user will encrypt a copy of the primary key with his own passphrase.
Not all of these features are important for Tutorial Password Manager, but this is a sensible security practice.
Requirements for the solution and principles of operation | - The storage key is generated by the RDSEED instruction or by using the initial value assignment form RDRAND in systems that do not support RDSEED.
- The keystore is encrypted with the master key.
- The master key is generated from the user's password phrase using the key generation function (KDF).
- The KDF function is based on the SHA-256 algorithm.
|
Here, the primary key is called the storage key, and the secondary key formed from the user's password phrase is called the master key. The user is authenticated by entering his passphrase, and the password manager generates the master key from it. If the master key successfully decrypts the repository key, the user is tested and you can decrypt the repository. If the passphrase is incorrect, decryption of the storage key is impossible, which does not allow decrypting the storage.
The last requirement for creating a key generation function based on the SHA-256 algorithm is due to the constraint arising from the need to use a hashing algorithm, which is supported in the Windows API CNG and in the Intel SGX encryption library.
Account Information
The last of the basic requirements concerns what exactly is placed in the repository. In this tutorial, we'll go the easy way. In fig. Figure 2 shows the layout of the main user interface window.
Requirements for the solution and principles of operation |
- The vault will contain account information including the following:
- Account / Provider Name
- Account / Provider URL
- Username ID
- Authentication password
- The storage will contain a fixed number of accounts.
|
Figure 2. A preliminary layout of the main window of the Tutorial Password Manager.The last requirement is dedicated to simplifying the code. If you fix the number of accounts in the repository, you can more easily determine the upper limit of the repository size. This will be important when designing an enclave. Of course, real password managers are deprived of such luxury, but in the training manual we can well afford it.
In further releases
In the
third part of this tutorial, we will take a closer look at the Tutorial Password Manager app for Intel SGX. We will determine the secrets, decide which parts of the application should be inside the enclave, how the enclave will interact with the main application and how the enclave affects the object model. Follow the news!
Read the first part of the tutorial in this series,
Intel Software Guard Extensions Extensions> Tutorial: Part 1, Intel SGX Foundation , or a list of all the published training materials in the article
Introducing the Intel Software Guard Extensions series of training materials .