There are a lot of JavaScript frameworks, libraries and various tools around. What to choose? Looking at this diversity, the developer is not easy to answer this question.
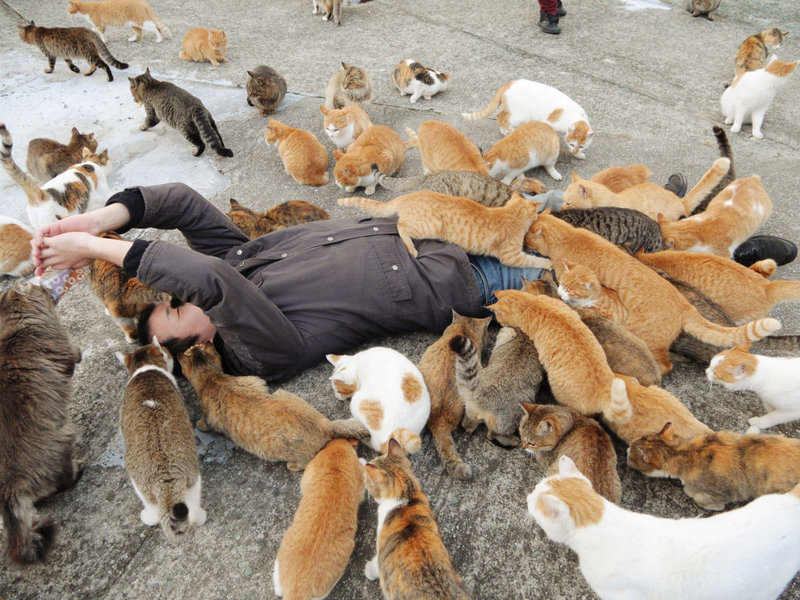
I know from experience: the first step of a new project, for example, creating a module or command-line tool, is to set up the working environment. Some people like this step, some cannot stand it, but even in those and others, preliminary preparation often takes an inadmissibly long time. Especially when you need to carefully check everything and make all mechanisms work together smoothly and stably.
Of course, you can include webpack, eslint, jasmine, or even TypeScript in the JS developer’s arsenal. With this approach, finding and fixing errors will not be problems. But the thing is that simple tools that require almost no adjustment are perfect for solving most auxiliary tasks. The environment collected from them will allow you to get started as quickly as possible, will ensure control of the correctness of the code and timely notification of errors.
')
When I think about the minimal JS environment, four things immediately come to mind: testing the program and analyzing the code, tracking file changes during work and checking the project before committing transactions to the version control system.
Now I will talk in detail about how, from scratch, to create a development environment that will allow you to begin productive work in a matter of minutes.
Initializing the Node.js project and the Git repository
First create a directory and go to it. Here the
$_
construct holds the argument of the last command.
$ mkdir awesome-module && cd $_
Now we initialize the Node.js project with default settings.
npm init --yes
Create folders and files
$ mkdir lib test $ touch index.js lib/meaningOfLife.js test/index.test.js test/meaningOfLife.test.js
Now, we initialize the Git repository and commit the first transaction.
$ git init $ git add -A; git commit -am "Awesome Module, day one"
We have prepared the structure of the project, we will deal with the tools.
Installation tools
We will use four simple modules, each of which is designed to solve one problem:
Why did I choose them? Just because they do not require any configuration at all. This approach perfectly unloads the head, opening the way to useful ideas relating to the development. Install the modules by running the following command in the project folder:
$ npm i
After installation (the speed of which depends only on the NPM mood), do not forget to create a
.gitignore
file and add the
node_modules
folder to
node_modules
. It is not needed in the repository.
Project Setup
By themselves, our tools do not need to be set up, but the project, nevertheless, will have to be set up. True, everything is extremely simple. Open
package.json
and add the following scripts to it:
"scripts": { "test": "ava", "lint": "standard", "dev": "chokidar '**/*.js' -c 'standard && ava'" }, "pre-commit": ["test", "lint"],
That's all. As soon as you execute the
npm run dev
chokidar
,
chokidar
will begin to monitor the status of the files, launching, if necessary, the
standard
for static analysis, and the
ava
for testing the code. If problems are found, you will immediately find out. The same applies to making changes to the repository. Our environment will not allow to commit a transaction until the project is successfully analyzed and tested.
Here I would like to draw attention to two things.
- You do not need to set
standard
or ava
globally, since they are executed from within the context of the node
.
- Since we use
&&
instead of ;
in the dev
script, the tests will not be called until the code passes the test with the static analyzer. It speeds up the work.
How to use it
The development environment is designed for programming in development style through testing (TDD). As soon as the
dev
script is run, you can create tests, they will be automatically added to the test code set, without the need to restart the file monitoring tool or perform any other operations.
Let's create the first test.
// test/meaningOfLife.test.js const test = require('ava') const meaningOfLife = require('../lib/meaningOfLife') test('Real meaning of life', (t) => { t.is(meaningOfLife(), 42) })
After saving this file, the system immediately reports that one of the tests failed. Fix it.
// lib/meaningOfLife.js module.exports = () => 42
Now everything works as it should! There is simply no place. Create a module that multiplies the numeric parameters. We will immediately prepare a unit test for it, and also see if the new module works correctly with
meaningOfLife
. This, by the way, will be an integration test, not a unit test. Here is the module code:
// lib/multiply.js module.exports = (number) => { if (typeof number !== 'number') throw new TypeError('Only numbers can be multiplied!') return number * 2 }
Here is a test for it:
// test/multiply.test.js const test = require('ava') const multiply = require('../lib/multiply') test('Can multiply numbers', (t) => { t.is(multiply(10), 20) }) test('Throws when you try to multiply non-number value', (t) => { t.throws(() => multiply('ohai!'), 'Only numbers can be multiplied!') })
Now let's test everything together:
// test/index.test.js const test = require('ava') const awesomeModule = require('../index') test('Works nicely together', (t) => { t.is(awesomeModule(), 84) })
And we add the following to index.js:
// index.js const meaningOfLife = require('./lib/meaningOfLife') const multiply = require('./lib/multiply') module.exports = () => multiply(meaningOfLife())
Tests passed! As you can see, thanks to the simplicity of the tools, we very quickly started working on the project, spending quite a bit of time preparing the environment.
Results
New sparkling tools often literally hypnotize programmers, forcing to forget about the main thing. And the main thing is simplification and acceleration of work and struggle with errors. Usually, much more often than it may seem, the simplest means are enough to solve these problems. Those that allow you to spend time on creating programs, and not on setting up auxiliary systems.
When the project starts to grow, you may feel that you need something much more advanced than the simple combination of ava, standard, chokidar-cli and precommit-hook. But in most cases, however, nothing else is needed. And those simple and understandable tools that I have described will serve you faithfully for a very, very long time.
And in what environment do you create programs?