As modern browsers began to support more features, and the web industry is rapidly moving toward mobile devices, there is a need to write compact and optimized code that does not make the user wait for a long time until the site loads. The front-end is good because it contains many simple code strategies and conventions that we can use to ensure optimal performance. In this article we have collected 9 simple tips that will help with the optimization of the code.
I must immediately say that some of the receptions were overseen by us on the western expanses of the Internet, and some were added by us. In any case, there was no such material in Runet. We often have to write large systems and optimize the download speed, so we try to fight for every byte. From here and decided to write about this important topic.
1. Use DocumentFragments or innerHTML instead of mass embeddings in elements.
Operations such as building a DOM structure, after loading the page, increase the load on the browser. Despite the improved performance of browsers, building a DOM tree is slow, therefore, knowledge of these nuances will help you to increase the speed of loading your page. That is why it is important to minimize the creation of a complex DOM tree.
For example, we have an ul element within a page that changes via javascript after calling AJAX to get an element of the JSON list. Often, developers will do something like this:
')
var list = document.querySelector('ul'); ajaxResult.items.forEach(function(item) {
The code above shows the wrong way to fill ul with elements - the speed of the addition of the DOM tree slows down. If you really want to use document.createElement and treat the element as a tree node, it would be more efficient to use DocumentFragement instead.
DocumentFragement is a so-called "mini-parent", "cloud storage" for its children. In our example, DocumentFragement should be considered as an invisible ul element that is outside of the DOM. This will keep your nodes virtual until they are entered into the DOM. The initial code sample will be optimized using DocumentFragement as follows:
var frag = document.createDocumentFragment(); ajaxResult.items.forEach(function(item) {
Adding child elements to a separate DocumentFragement, and then adding this fragment to the parent list is used with just one DOM operation. Thus, it turns out much faster than mass implementations. If you do not need to work with such list items as nodes, it is more advantageous to build HTML with the help of the lines:
var htmlStr = ''; ajaxResult.items.forEach(function(item) {
Thus, injecting the created string through innerHTML, there is still only one DOM operation and less code even than with the DocumentFragment method.
2. Frequently executed events / methods
In most of the work, you need to add event handling, which will occur quite often during user interaction. For example, a window's resize or onmouseover event. If the processing of event data is too resource-intensive, you can give a large load on the browser, and this, in turn, will lead to bad consequences on the user side. This is where the debouncing method came from. Debouncing will limit the number of times a function is performed within a specified time interval. Here is an example of using the debouncing function:
The debounce method returns a function that wraps the response, limiting its execution speed to the limit specified in the second argument. Now your constant answers will not be able to damage the user's browser.
3. Static cache, non essential content in web storage
APIs like Web repositories have become a huge boost and simplification of working with the Cookie API, which developers have been using for many years. One of the strategies you can use when working with memory to store nonessential data was static content. This includes HTML snippets, the content of an article that was loaded using AJAX and other various methods that need to be requested no more than once. Below is a small javascript code for working with web storage:
this[key] = value; } }; return { get: function(key) { return this.isFresh(key); }, set: function(key, value, minutes) { var expDate = new Date(); expDate.setMinutes(expDate.getMinutes() + (minutes || 0)); try { cacheObj.setItem(key, JSON.stringify({ value: value, expires: expDate.getTime() })); } catch(e) {} }, isFresh: function(key) {
This plugin using the basic get and set methods, or through the isFresh method, checks whether the stored value is relevant.
require(['storage'], function(storage) { var content = storage.get('sidebarContent'); if(!content) {
Thus, we get rid of frequent repeated requests to the server. Analyze your sites for material that is not dynamic, but may well be frequently requested. Use this method to improve the effectiveness of the site.
4. Using curl.js
Asynchronous resource loading has become popular due to XMLHttpRequest (an object that is allowed for AJAX), so as not to block resource loading, reduce page load time and allow content to load without reloading it.
This is where John Hannah’s great plugin will be used. Curl.js. The curl loader is a typical asynchronous loader, but with a few other configuration options, useful plugins, and much more. Here are some examples of using curl.js:
When you start using this method, instead of simple asynchronous loaders, the difference will be noticeable immediately, but, more importantly, users will see the difference!
For example, we only load Twitter, Facebook and Google Plus widgets if a DIV with CSS-social class exists. If this block is not present, we will not load the widgets. Consequently, using the principle of “check - if necessary before loading - we will load” will save the user from downloading several completely unnecessary kilobytes.
5. Use Array.prototype.join instead of string concatenation.
One micro client side optimization with Array.prototype.join replaces the usual concatenation. For example, in paragraph 1 (see above), elementary string concatenation was used. And this is how it will look like after this optimization.
var items = []; ajaxResult.items.forEach(function(item) {
6. Use CSS Animations whenever possible.
It can be argued that the growth of JavaScript libraries such as jQuery and MooTools led to complex animations. Today, many developers still use JavaScript to animate elements, despite the fact that the corresponding browsers support CSS animations through transform and keyframe.
CSS animations are more efficient than JavaScript animations. CSS animations also have the added benefit of (usually) - much less code. Many CSS animations are processed by the GPU, and are thus smoother. For example:
.myAnimation { animation: someAnimation 1s; transform: translate3d(0, 0, 0); }
Similar CSS animation properties improve performance and quality of animation using sub-pixel interpolation. This animation processor is easier to perform, since the graphics engine is involved, it is very important for mobile devices. However, the most acceptable would be the use of CSS animation along with the connection control via js. For example, when the animation rules are written in CSS, and with the help of JavaScript we change the input parameters (color, background, size, etc.)
7. Use event delegation
Imagine an unordered list of ul that can contain any number of child li elements, and each li must do something with a mouse click. You can add event handlers to individual elements, but what if elements are added or removed frequently? You have to deal with adding and removing event handlers, as well as the elements themselves. This is where we need to delegate events.
Event delegation replaces the need to add event handlers to individual elements instead of hosting a single event handler for the parent. When this event is triggered, the event.target checks if the conditions are met to call the event handling function.
Here is an incredibly simple example of what is happening:
All JavaScript frameworks ensure that a selector is delegated. The fact is that you avoid placing event handlers on separate elements by setting one on the parent. This method is more efficient and less costly.
8. Use data URL's instead of SRC’s
The speed of loading the page depends on the use of sprites and writing compact code. The number of requests sent by this page also play a role in performance. Minimizing queries helps your website load faster and one of the ways to eliminate queries (apart from using sprites) is to use the Data URL instead of the SRC image attributes:
<!—… --> <img src="/images/logo.png" /> <img src="data: image/jpeg;base64,/9j/4AAQSkZJRgABAgAAZABkAAD/7AARRHVja3kAAQAEAAAAPAAA/+4ADkFkb2 JlAGTAAAAAAf/bAIQABgQEBAUEBgUFBgkGBQYJCwgGBggLDAoKCwoKDBAMDAwMDAwQDA4PEA8ODBMTFBQTExwbGxscHx8fHx8fH x8fHwEHBwcNDA0YEBAYGhURFRofHx8fHx8fHx8fHx8fHx8fH ...." />
Of course, the page size will increase, but you reduce the waiting time and the number of requests to the server. Most browsers now support Data URL's in CSS files (for background images), so this method can generally be widely used.
9. Use Media Queries to load background images of unusual sizes.
The previous paragraph mentioned the use of sprites images to speed up the loading site. But what about the adaptive site, where you need to scale the images, whether it's a background image, a search icon, etc.? To carry out such operations with sprites is quite inconvenient and takes a lot of time. This is where media queries come to the rescue.
CSS
supports have wider support, and CSS media queries are close to CSS “logic. CSS media queries are most often used to adjust (adjust) CSS properties for a device (usually to fit the width of the screen); With this method, we often change the width or floating position of the element. Why not change the background image based on this method?
.someElement { background-image: url(sunset.jpg); } @media only screen and (max-width : 1024px) { .someElement { background-image: url(sunset-small.jpg); } }
The fragment above loads a smaller image if the user uses a mobile device (meaning the file size, not the aspect ratio). In this way, it is enough to render a smaller sprite, which eliminates the need to manipulate images programmatically or cut a sprite for mobile devices, etc.
Most countries charge for the data used (not unlimited), which means you can save money by reducing download time.
Small updates, big stridesVery often, developers put themselves and their style code in the first place instead of thinking first about their users. Many small updates are built to improve user comfort, so every bit of download optimization improves the impression of your site. Google has released a fairly convenient extension for your browser that will help you analyze the speed of your site and find out what else can be optimized to increase the page load. This extension is called PageSpeed ​​Insights. You can use it through the standard developer panel. This widget will show you a minimal analysis of your portal and give advice on its optimization, for example:
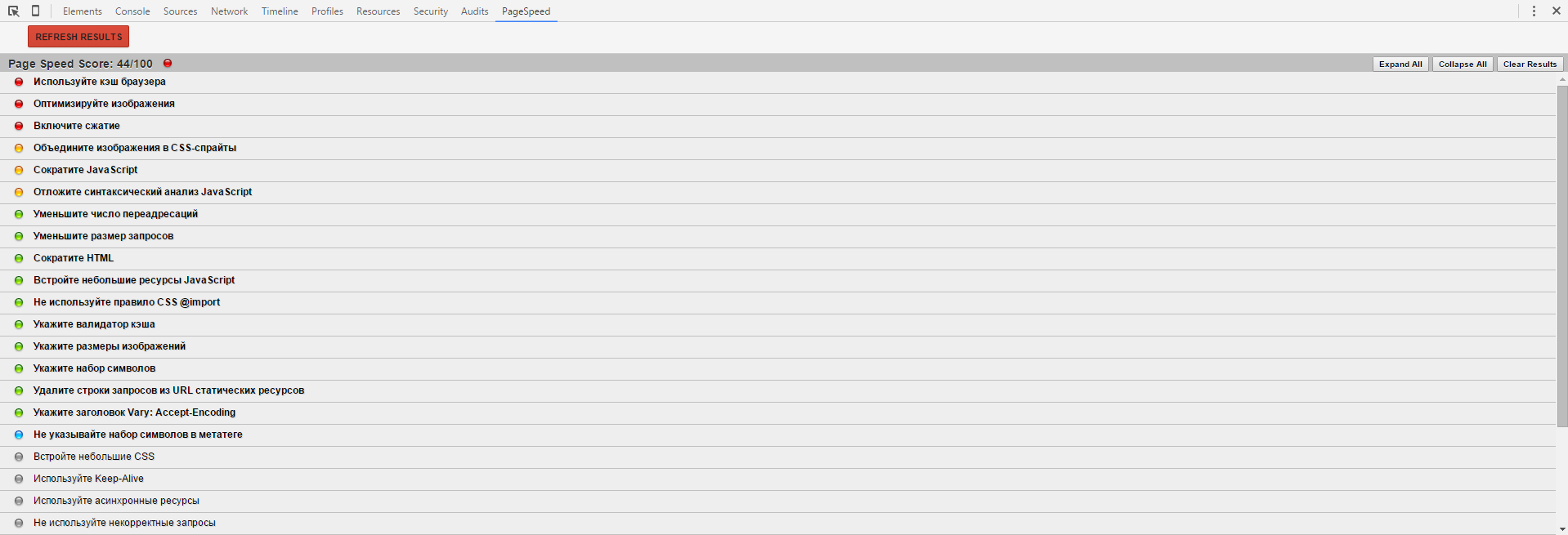
In any case, you need to optimize the site as much as possible. The faster it works, the more users will be satisfied, the more the site will earn. Unfortunately, not everyone thinks about it. This is even evident in demand: we are often ordered by usability audits or Back-End, and rarely anyone asks for a Front-End audit, although it is absolutely not difficult to do and can provide a good field for improvement.