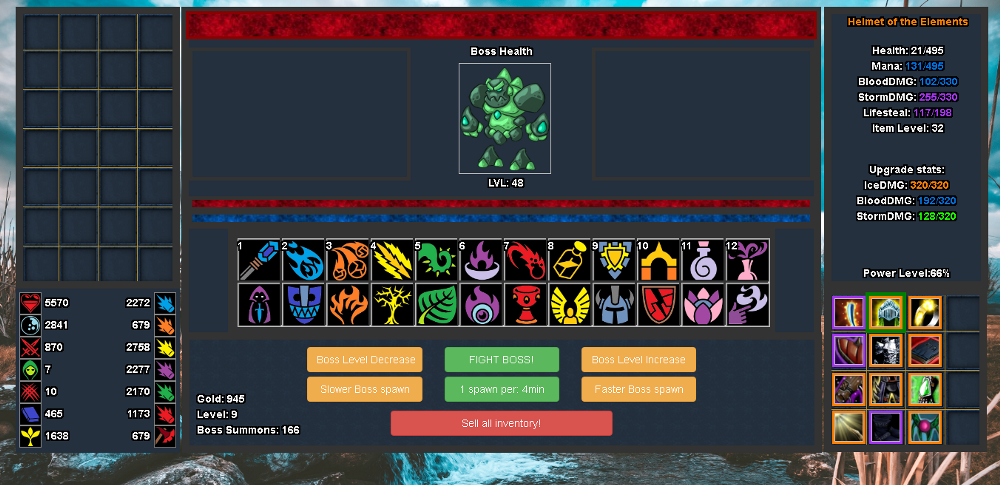
So, you want to try to create a game, but a little scared. Don't worry, I was scared too.
I was afraid to use objects, for example. They were such a big scary thing that I put off for later. But now I use them all the time.
')
I'm going to show you all the steps I went through when creating my role-playing game in JavaScript.
Keep in mind that I'm new (only 2 months in programming), so some of my decisions can be improved. I will try to give you the basics from which to start.
Here is my game on CodePen (I note that it is not yet optimized for mobile devices).
First , select the goal of your game. Is this a puzzle? RPG? Hack & slash? Well, now think about the technical difficulties of its creation. A puzzle game will require a lot of complex JavaScript code, Hack & slash requires careful balancing and so on.
Also decide whether you want it to be a browser game, a mobile game, or both.
For example, my game cannot fit well on the screen of a mobile phone, since the player has 24 spells. It is inconvenient to click these small buttons on a small screen, so I will need to redo this mobile game.
Second , write down everything you need to actually make the game. For me it was:
- inventory systems;
- stock generator;
- player parameters;
- system saves.
Thirdly , start making your game by solving these problems one by one.
Need help creating the game itself?
It is much easier to break the game into small tasks. You do not make the game, you make the inventory system. Then you make a combat system. And so on.
Except when you are good at drawing or want to spend months or years to learn how to draw, use these tools to create assets that will make your game look good:
Problems I encountered and how I solved them
Sprite lists
Are you planning to have more than 20 images in your game? If so, you do not want each of these 20 images to be associated with another. You may not think that 20 images is a lot, but what if you decide to add another 50? That's when sprite lists come in handy: add some photos there, copy the CSS file into your project and just add the class to the element that matches the desired image.
Saving game state
Do you want your game saved? You can choose between browser storage and storing things on the server. Servers require special knowledge. If you don’t have them, I recommend using LocalStorage. It saves the game until the user deletes it with some sort of cleaning tool. Here is how I did it:

In essence, save all your data into one object, then update your items when loading. Use JSON string conversion and analyze it later.
Modulate your code
Find out which part of the game is difficult to encode, and which part of the code is worth modulating. I mistakenly began to encode spells that went quickly. I needed 24 functions along with 24 ifCritical functions.
I swear I didn't write this ... Uh, they made me
It even has a function that adds custom functions.Now you may ask how the second spell works? I have a playerAttack () function that uses a spell object to do different things:
- First, it launches an update of the spell function that calls the spell object. Then the spell takes your statistics and turns them into “damage”, “mana”, etc.
- She checks the damage. If it is greater than 0, it deals damage to the boss and displays the damage that the spell has committed and its amount. She does it for everything else too. You might think that checking for more damage 0 is useless, but you will think about it again when the game tells you that you did 0 damage and therefore recovered 0 mana.
- Runs a user-defined function, if the spell has one. It can be used to give a spell special effects that are not possible with a normal attack function.
Game cycle
My game cycle checks and updates things: player statistics, when a player is dead, when a player has a new level, when a boss is killed, etc.
You must understand it yourself. I think this is a good experience. Think about what and when to check and update. For example, I set the level check for every 20 seconds, because the level is not such an important thing.
But there is still the death of the boss. I run her check every second since the start of the battle. Why? So that the player does not have to wait 20 seconds until the boss dies. For some things, the cycle is not even needed. Functions can simply be called when they are needed. Take, for example, the spell function. It is needed only when the player uses the spell.
Here is what I found out:
- Objects are good. When you need to save data, you just need to save the object, not 50 individual variables.
- Always set time-outs and intervals as variables so that you can clear them later - do not do this only if these are permanent effects and you are sure that you will not have to clear them later.
- One big javascript file is not the wisest idea. CodePen allows you to use only one JavaScript file, but ideally, you should split everything into modules.
- If you are not worried about performance, you can simply copy and paste an object when it needs to be updated — there is no need to update half the values ​​separately. If the object is huge, you can even define it first as a variable, like: var object ; and then build it using some other features when you want to update it. I did it with my spells. Each time a player uses a spell, the updateSpell () function first determines the object of the spell again, calculates all damage and statistics, and then triggers the spell.
Fun facts that I had to put up with:
- The ability to mana at the boss level, because if she were at the player level, I would punish the players every time they had a higher level. It would also make higher level bosses much more difficult than I would like.
- The elements are created with all the statistics, but they are not displayed if they are equal to 0. Thus, I do not need to check whether they are defined and I can not show statistics if they are equal to 0. Double victory!
- I have a lot of simplified buffs and debuffs. There are buffstat, nerfStat, totalStat and stat variables. Therefore, buffs and debuffs are never in the stack.
- With bosses, skills don't nerf up to zero. Everything is much more complicated. Parameters start from 9999999, then check if it is less than zero. If so, he equates them to zero. So if you manage to reach a level where the parameters will be expressed in billions, I may have to add more zeros.
All this has taught me that I need to plan a game, running a little ahead, even if I just create a fun project to expand my skills and abilities. In addition, I now have a much better understanding of how errors occur: sometimes you are not aware of all the borderline cases that can block your path. And then the bugs bite.
Bugs and exploits
It startled me and scared a little. I could not believe that there were bugs in my flawless creation.
Of course, I'm exaggerating a bit. But I underestimated the number of things that could go wrong, even if I did not realize it.
Here are a few bugs and exploits that have come to mind:
- You can change the level of the boss during a battle with him, and the cycle then works better.
- Indicators of experience and mana sometimes overflow.
- You can attack the boss before the battle begins. Here is a slap in the face!
- Mana can become negative and it will not allow you to carry out even basic attacks, which are the main way to restore mana.
- Treatment temporarily increases the maximum amount of health.
- One spell was actually inoperative due to CSS problems.
- If you do not attack in combat, your spells will be on endless recharge.
It all sounds awful, doesn't it? In the MMORPG, these things will spoil everything from the very first day! Well, the good news is that most of them were easily repairable - as a rule, it was enough to write less than one line of code. Other errors, however, required me to completely redo the entire system. With the spell system, I came from writing as many as three functions for each spell to what I needed to describe just a small object that takes only a few seconds to edit.
Make your game in JavaScript and have fun.
Publication support is the Edison company, which develops geolocation games with orcs and demons and conducted a technical audit and finalization of the economic game .