(Note of the lane.: The algorithms given in the article refer to the names of the thousands of degrees on a short scale .)Some time ago I got the opportunity to participate in the development of the
game in the idle genre . What is an idle game? If this is your first time hearing about this
booming genre , try playing
Adventure Capitalist . The Gamasutra website also has several articles (
1 ,
2 ,
3 ,
4 ) that allow you to look at the genre a little deeper.
One of the problems that an idle game developer will inevitably encounter is how to put all these huge numbers on the screen, and more importantly, how the player can figure them out. In this article, we will look at how to rewrite very long numbers as strings that will be convenient for players. This process can be divided into two main parts:
')
- First, we will represent the number in an exponential notation . Some idle game developers stop at this , and some players seem to like it . In Wolfram MathWorld there is a very straightforward algorithm for translating numbers , which we will use. But there is another approach that can be taken if you prefer to play with bits . I just mention it, we will not discuss it in this article. If you want to use it in your game, take a look at the download folder of this article, which has an easy-to-use script for exponential notation:
ScientificNotation.FromDouble(12340000); // .significand == 1.234; .exponent == 7
- Having found the representation of a number in the exponential notation, we can choose a name for its order. This will be the main task of this article.
Download Source Code
The source code is available for download and is ready for use in your idle games. Using it is easy:
LargeNumber.ToString (12340000); // "12.34 million"
Also, a small sample program is included in the download files, showing how large numbers are converted into strings in the game (using the button scripts described in the
previous article ):
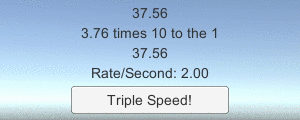
All this is part of a
set of sample code that should accompany some of my articles written earlier, mainly from the tutorials on working with the network in Unity (
1 ,
2 ,
3 ). Download code samples to check how they work!
Standard Number Format Lines
Numbers less than a million are small enough and do not require serious processing. You can rely on the
built-in formatting capabilities of C # numbers , they will do most of the work for us:
double x = 987654.321
x.ToString ("N2"); // "987,654.32"
By adding the
appropriate argument to the ToString method , we almost without difficulty group together delimiters and limited decimal places. We do not need to perform additional divisions or use
the rounding down functions .
Latin prefixes to not very large numbers
When working with large numbers expressed in millions (millions), billions (billions), trillions (trillions), and so on, we know that names change every three digits. Having a number in the exponential notation, we know that it is expressed in millions, if its order is from 6 to 8, in billions - from 9 to 11, and in trillions, if the index is from 12 to 14, and so on. In general, for a number with an exponential notation:
M x 10 N
We need to express
N as follows:
N = 3(U+1) + V, V < 3
Then the final line of the number will be:
M*(10^V) Name(U)+"llion"
where
Name (U) can be obtained from this table of Latin prefixes:
U | Name (U) |
one | mi |
2 | bi |
3 | tri |
four | quadri |
five | quinti |
6 | sexti |
7 | septi |
eight | octi |
9 | noni |
For example, if we process the number 123,000,000,000,000,000, we can express it in an exponential notation like this:
1.23 x 10 17 , M = 1.23 N = 17
By rewriting
N , we get:
17 = 3*(4+1) + 2, U = 4 V = 2
This means that our number looks like:
1.23*(10 2 ) Name(4)+"llion"
Simplifying, we get:
123 quadrillion
Combinations of prefixes for large numbers
Wikipedia has a
helpful article explaining how names are given to large numbers. I will not repeat what is written there. Instead, I will give a description of how to approach the algorithm and present some examples.
Having received the value of the
U number
(comment lane: here and hereinafter the author says N, but this is clearly wrong) , as we did in numbers with Latin prefixes, it is necessary to divide it into bits (units, tens, hundreds), and then formulate The title is as follows:
( ) + () + ( ) + ( ) + ( "a" ) + "llion"
The prefixes of units, tens and hundreds can be taken from the
table in the Wikipedia article. In some cases, it is necessary to add a modifier if, in addition to certain unit prefixes, we put prefixes of tens or hundreds, but in most cases the modifier is not needed. Let's look at a few examples.
If
U = 12 , then for units the figure will be 2, which corresponds to
"duo" , and for tens - 1, corresponding to
"deci" . In the transition from
"duo" to
"deci" modifier is not needed. By the above formula, we get a
“duodecillion” .
If
U = 27 , for units the figure is 7 (corresponds to
"septe" ), for tens, 2 - (corresponds to
"viginti" ). According to the table, the transition from
“septe” to
“viginti” requires the modifier
“m” . Putting it all together, we get
"septemvigintillion" .
Finally, if
U = 30 , then the number for tens is 3, which corresponds to
“triginta” , which has the ending
“a” . Therefore, we discard it and get
"trigintillion" .
If you are trying to understand how all this will look in the code, I will answer: it will be a combination of array searches, several
if constructions and concatenation and string substitution operations. If you are lucky and you work with a language that supports comparisons with patterns, then you can use them.
The source code files contain a working implementation that handles all cases up to U = 999. It has been tested on all names included in the list of large numbers. I urge you to download them if you need ready-to-use code that can be easily inserted into your own projects, or if you want to compare your implementation with a working version.
And if U = 1000?
Then the number is called a
million (approx. Ln .: in the long scale - the quingent billion) .
Big numbers are not big enough
Double-precision floating-point numbers allow operation with numbers up to 10
308 , or U = 101. If your game requires higher numbers, you can try to store numbers with
arbitrary precision . Thus, players can be made millionaires.
But first ask yourself if you really need it. Infinity gives us enough room to develop games, and we don’t have to try to fill in all the space given to us. To paraphrase my college professor: “Think of the largest final number you can think of. Now use the same number as its exponent, and raise this number to the power of yourself. If we now place this number on the axis of real numbers, then compared to infinity, it will still be very close to zero. ”
Will your game become more interesting by going beyond double precision numbers? For some games, the answer may be positive, but in most cases I advise you to think again about the balance and design of the game (
1 ,
2 ,
3 ,
4 ).
If you liked the article, do not forget to download the
folder with the project ! I hope it will be useful in developing your own game. Thanks for reading!