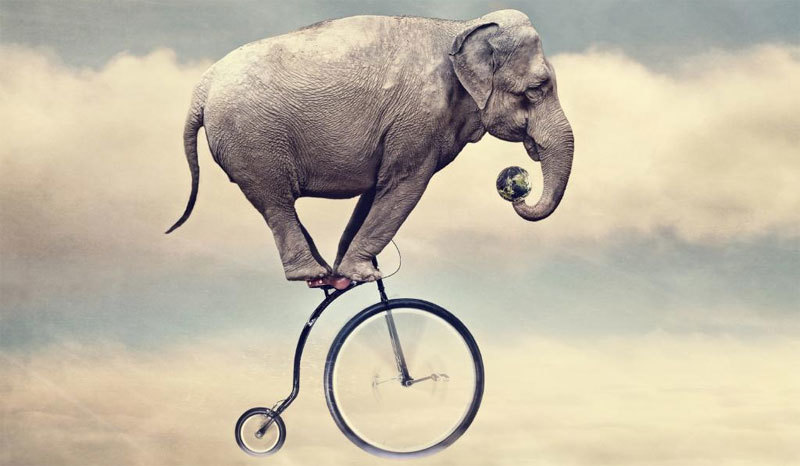
In this document and sample application, I’ll demonstrate how to use the
Intel RealSense R200 camera and the
Enhanced Photography functionality included with the
Intel RealSense SDK .
Requirements
equipment requirements- 4th generation Intel Core processor (based on Haswell microarchitecture)
- 8 GB of free hard disk space
- Intel RealSense R200 Camera
- USB 3.0 port for camera connection
Software Requirements
')
- 64-bit version of Microsoft * 8.1 or later
- Microsoft Visual Studio * 2010–2015 with the latest service pack
- Microsoft .NET * 4.0 Framework for C # Development
- Intel RealSense SDK that can be downloaded here.
Note. This
particular sample project was created in Visual Studio 2015 using the latest release of .NET.
Project structure
In this sample application, I have separated the Intel RealSense SDK functionality from the GUI-level code so that developers can more conveniently focus on the functionality related to the enhanced Enhanced Photography capabilities of the R200 camera. For this, I created the following C # wrapper classes.
- RSStreaming . Shell for the streaming functionality of the Intel RealSense SDK.
- RSEnhancedPhotography . Shell for Enhanced Photography functionality Intel RealSense SDK.
In addition, for simplicity, I created separate WinForms forms for each type of Enhanced Photography function. This creates a certain amount of duplicate code, but it provides clarity in the demonstration of each function Enhanced Photography.
The project structure contains other elements besides those described here. I will take a closer look at all the source code files and their work later in this document.
I create my own events to demonstrate how, to the greatest extent possible, to separate the functionality of the Intel RealSense SDK from the source code of the graphical user interface. Such a solution seems to me more “clean” compared to passing the whole form to the class so that this class can access the properties in the form.
Also, keep in mind that this sample application is not at all the benchmark for perfect software code. There are almost no checks at runtime, there are no try / catch blocks. I tried to make a simple and clear example with a clear code, so I tried to save the code from additional constructions as much as possible.
The forms in the sample application are not very elegant, but they do their job well: they demonstrate how to use Enhanced Photography.
Visual Studio Project Structure
The figure above shows the solution in Visual Studio 2015. The various folders contain the following.
- Forms . Various forms of WinForms, demonstrating the various features of Enhanced Photography.
- Source . Source code distributed with the project.
- Source \ CustomEventArgs . Contains classes derived from EventArgs own class.
- MainForm.CS . The main form of the application.
Simple overall sequence diagram

When you run the sample application, the FormMain form is displayed. In this form, you can start streaming by clicking the Streaming button.

. When this button is pressed, the application starts streaming using the functionality of the
RSStreaming class.
The
RSStreaming class constantly updates
FormMain , causing its internal
OnNewStreamingSample event. This is done for each frame coming from the camera.
You can stop streaming by clicking the Stop Streaming button.

. After that, the streaming simply stops, there are no other commands available except how to turn it on again. If you press the Photo button

, image data is saved to disk and streaming stops. After stopping the streaming and saving the photo to disk, the photo change buttons become active. With their help, you can select one of the Enhanced Photography dialogs, demonstrating the capabilities implemented in this sample application.
If you select one of the Enhanced Photo dialog boxes, the sample image stored on the disk is loaded and used. This is explained in more detail later in the document.
Parsing code
The following sections describe the entire application and its progress, describing the various classes.
Forms
FormDepthEnhance . Demonstrates the use of two different depth quality parameters to display depth data. The user can choose low or high quality.
FormDepthPasteOnPlane . This form demonstrates how to use the insert functionality on a plane to import an external image by clicking two points on a flat surface.
FormDepthRefocus . This form shows how to click anywhere on the image and make the image sharp at that location, and the rest of the image is blurred. You can click anywhere in the image; click location becomes a new focus point. You can adjust the simulated aperture of the camera lens by moving the slider.
FormDepthResize . This shows the resizing functionality: you can zoom the image to the size of a color image.
FormMeasure . Shows how to use the Enhanced Photography measurement functions to obtain distance, accuracy, and confidence values.
FormMain . This is the main form of the application. In it, a user can turn on streaming data from an Intel RealSense camera, stop streaming and take a snapshot. You can then apply various Enhanced Photography functions to the captured image.

Source
RSEnhancedPhotography.CS . This is a wrapper class that encapsulates all the Enhanced Photography functionality of Intel RealSense cameras. The purpose of this class is to separate as many features of the Intel RealSense camera from the level of the graphical user interface. This class uses custom events to publish data to the client application. The
RSEnhancedImageArg class gets a new image that needs to be displayed.
RSPaintUtil.CS . This is a static helper class used to draw mouse click points and lines in C # PictureBox controls.
RSPoints.CS . This auxiliary class encloses the PXCMPointI32 point objects and creates functionality for storing points, checking point data, and transferring data about points that should be displayed on the screen.
RSStreaming.CS . This is the wrapper class for streaming Intel RealSense camera data. It sends a stream of data and publishes an event for the client. The event uses the RSSampleArg class to store data that will be used by the client.
RSUtility.CS . This is a static class containing source code. The functionality implemented in it does not belong to any particular class.
Source \ CustomEventArgs Code
RSEnhancedImageArg . Extends
EventArgs , contains a
PXCMImage object. This object will contain an image modified using the Enhanced Photography functionality of the Intel RealSense SDK. This image is displayed in separate
WinForms PictureBox controls.
RSMeasureArg.CS . Expands
EventArgs , contains measurement data returned by Enhanced Photography functionality. This data is used on the
WinForm FormMeasure form to provide the user with measurement data.
RSSampleArg.CS . Expands
EventArgs , contains the
PXCMCapture.Sample object. This object contains the last frame taken by the camera. It is used for streaming data and for displaying it in the
WinForm FormMain form.
Detailed analysis
Now I will go to the description of the auxiliary classes that support forms. I think it's better to learn how the base code works, and not just focus on the work of the forms. Let's start with the first class, RSStreaming.
I will not consider completely small details, such as the functions of receiving and assignment, they are completely understandable. I will not describe any other obvious things.
RSStreaming
RSStreaming is a wrapper class for streaming the Intel RealSense SDK. This class is not too complicated. The challenge is to give a simple example of streaming data from an Intel RealSense camera. It can transmit a stream of an image, as well as take a snapshot and transmit both to the client using events.
public event EventHandler<RSSampleArg> OnNewStreamingSample;
As mentioned earlier in this document, I use events to send data to a client application (on forms). RSStreaming sends data to the client (in this case, to FormMain) using the
OnNewStreamingSample event. It takes one parameter,
RSSampleArg , which will contain the most recent camera sample.
public bool Initialized
A simple getter flag indicating whether the class has been initialized.
public bool IsStreaming
A simple receiving flag indicates that the class is currently streaming data.
public void StartStreaming( )
A common function that the client uses to start streaming data from the camera. Checks if the class has been initialized properly. If so, it calls the
InitCamera () function to initialize the camera.
I would like to note that I use one function, which is usually ignored. As you may have noticed, many examples of streaming typically use a
while loop and
AcquireAccess with some kind of mechanism to stop transmission using a logical flag. This example uses a different approach.
My approach is different: I use the
StreamFrames function in the PXCMSenseManager. It causes SenseManager to run its own internal stream and send data using event handling. This is accomplished by assigning the object to the PXCMSenseManager.Handler function. More on this in the description of the
InitCamera () function
below .
private void InitCamera( )
InitCamera is a private function that initializes the camera and streaming transmission. First we create the PXCMSenseManager and PXCMSession objects. Then we need information about the device (camera), which we obtain using the static RSUtility function
GetDeviceByType () , passing it the session and the desired camera type.
Then I create two PXCMVideoModule.DataDesc objects, one for the color image stream, the other for the depth stream. Then I set up each thread. After setting up the threads, I give the PXCMSenseManager command to enable threads.
As stated in the description of the
StartStreaming () function, I use an event-based approach to control the transmission and reception of data. To do this, I create and initialize the PXCMSenseManager.Handler event handler object and assign it to the
OnNewSample function. Every time the camera shoots a new frame, the
OnNewSample event handler is
called .
Then I initialize SenseManager, send it to the handler object and give the command to use this object and its event handler.
private pxcmStatus OnNewSample( int mid, PXCMCapture.Sample sample )
OnNewSample - event handler for the PXCMSenseManager.Handler object.
Options- Mid . Thread id If several streams are requested using the EnableVideoStream [s] function, then this is PXCCapture.CUID + 0 or PXCCapture.CUID + 1 ...
- PXCMCapture.Sample . Sample camera image.
When this function is called, I get the image from the
Sample argument and put it in a new
RSSampleArg object, then I
trigger the OnNewStreamingSample event for this class. After this event notifies the
FormMain client that the new image is ready to be displayed.
We release the frame and return the desired
pxcmStatus , which in this case is not used.
public void StopStreaming( )
Stops streaming, closing threads and calling
Dispose () .
private void Dispose( )
Frees up resources for garbage collection.
RSEnhancedPhotography
The
RSEnhancedPhotography class was created with the goal of
enclosing all the Enhanced Photography functionality in one user-friendly class. It works on the principle of events. After image processing, an event is created that returns the newly created image or measurement data to the client application or class.
public RSEnhancedPhotography( PXCMPhoto photo )
The constructor initializes some global variables used in the class. The only input parameter is the original photo, taken in the main form. It is fully initialized with image data and is used to initialize the local
_colorPhoto object.
public void Dispose( )
Memory free for garbage collector.
public void SendOriginalImage( )
Returns the original image to the calling application using the
OnImageProcessed event.
public void MeasurePoints( RSPoints points )
MeasurePoints gets the completed
RSPoints object. First, I check that there really are two points in this object: the starting point and the ending point. After this check, a
MeasureData object is
created , which is
sent to the MeasureDistance function's
PXCMEnhancedPhoto objects .
Then I take the data from the filled
measureData object and fill the
RSMeasureArg object. Note the parameter (mesaureData.distance / 10) that converts the value to centimeters. After filling the
arg object, I send it to the client via the
OnImageMeasured event.
public void RefocusOnPoint( RSPoints point, float aperture = 50 )
When using the camera, you can adjust the aperture to adjust the depth of field. With a small aperture, we get a shallow depth of field: all objects in front of and behind the subject will be blurred.
RefocusOnPoint works the same way. This feature allows you to change the focus point on the image.
Of course, for settings of this aperture, values in the range from 0 to 100 are not suitable, but for our example they will fit perfectly. If you want, convert them to the appropriate values of the aperture scale and send me the updated code.
RefocusOnPoint uses the
DepthRefocus feature of the
PXCMEnhancedPhotos class to create a new image with a new depth focus, using the original color photo, the point where the user clicks, and the aperture value. After receiving the
PXCMPhoto , I get the original image by calling
QueryReferenceImage () and providing the PXCMImage to the RSEnahncedImageArg instance. After that, you just need to pass it back to the client application using the
OnImageProcessed event.
public void DepthEnhancement( PXCMEnhancedPhoto.DepthFillQuality quality )
Improves or changes the quality of the image depth between two values: high or low. Quality is indicated by the
DepthFillQuality parameter.
First, you need to initialize the local
PXCMPhoto image by calling the
EnhanceDepth function in PXCMEnhancedPhoto, providing the original
PXCMPhoto image and the desired quality.
Then, to initialize the
PXCMImage , I use
QueryDepthImage in
enhancedPhoto to get the newly created depth image.
After that, I create a new
RSEnhancedImageArg object to send to the client using
OnImageProcessed .
public void DepthResize( )
This is a simplified way to resize a depth image. In this case, the size of the depth image becomes the same as the color image in the original PXCMPhoto object created in the constructor.
First I need size information from a color photo. To do this, I request the original PXCMPhoto object specified in the constructor. Then I create an instance of the PhotoUtils object containing the DepthResize function. After obtaining the size of the original image, I save the width and height values in the required PXCMSizeI32 object.
The following is a simple process: you need to give PXCMEnhancedPhoto a command to resize the image by calling the
DepthResize function and specifying the PXCMPhoto object and the desired size.
After resizing, everything happens in the usual way. Create an image by polling the
enhancedPhoto , fill in RSEnhancedArg, and send it to the client using
OnImageProcessed .
public void PastOnPlane( RSPoints points )
This feature shows how you can insert a PXCMPhoto image on a flat surface. In this case, the inserted image adapts to its surroundings. For example, if you insert an image on a wall, it will look vertical and be at the same angle. If you insert an image on the surface of the table, it will look as if it is on the table.
First you need to make sure that there are two valid points, it is necessary for functionality.
Then we load the image that needs to be inserted using the
LoadBitmap function in the RSUtility.
The main object for this is the
PXCMEnhancedPhoto.Paster class. This is a new class in the R5 release. In the Paster class, there is a PasteOnPlane function, which was previously in the PXCMEnhancedPhoto class, and has now been moved to this new class.
In this function, I check that the value returned from
PastOnPlane is different from null. The fact is that we have no guarantee that the
PasteOnPlane function
succeeded in performing the action. For example, if the surface between two points is not flat, the function will not work as desired. I just want to make sure that I am not using a null object.
If a successful result is returned, I get the original image, save it, pass it to the RSEnhancedImageArg object and publish it in the client application.
RSUtility
RSUtility is a static auxiliary class containing functionality not related to any other classes.
public static PXCMCapture.DeviceInfo GetDeviceByType(PXCMSession session, PXCMCapture.DeviceModel deviceType)
This is an auxiliary function designed to obtain detailed information about the device, in this case about the R200 camera. This functionality is demonstrated in a variety of RealSense examples.
First we set the filter, specifying that we need a sensor as the main group, then as a subgroup we specify the video recording sensor.
In one system there can be several devices, therefore it is necessary to go through all possible devices sequentially. For each iteration, I
populate the current
PXCMSession.ImplDesc data in the
currentGroup object. If there is no error, go to the next step, where we fill the
PXCMCapture object.
Having prepared the
PXCMCapture object, I
loop through the various devices connected to this object. I check if the information about the current device matches the camera we need. If yes, the cycle is interrupted. If not, go to the information about the next device connected to the
PXCMCapture , until all devices are checked.
When the device is found, we return it to the client, which in this case is an
RSStreaming object.
public static Bitmap ToRGBBitmap( PXCMCapture.Sample sample )
A polymorphic function simply calls
ToRGBBitmap (a PXCMImage image), passing it the image of an example argument.
public static Bitmap ToRGBBitmap( PXCMImage image )
A simple function that uses the functionality of PXCMImage objects to get raster data using the
PXCMImage.ImageData object. Data is retrieved, stored in a .NET raster object, and returned to the client.
public static PXCMImage LoadBitmap( PXCMSession session )
This feature is used to support
PasteOnPlanes . It loads the predefined bitmap into the PXCMImage object and returns it to the client.
First, it gets the path to the file and checks for the presence of the file. If not, it returns null. If the file exists, it creates a new .NET raster object, loading it from a file.
The
PXCMImage.ImageInfo object
is used to store basic information about the raster image. It is used when creating the PXCMImage object. These are the specifications of the image that we will create.
Then we need a
PXCMImage.ImageData object containing the data of the actual bitmap. A .NET BitmapData object is created and initialized with data that describes the format and structure of the data we need.
For more information about how Bitmap.Lockbits work, see
here . The PXCMImage object releases access to image data to free up used memory; the bitmap is unlocked, the PXCMImage is returned to the client.
public static int GetDepthAtClickPoint( PXCMPhoto photo, PXCMPointI32 point )
This feature accepts
PXCMPhoto and
PXCMPointI32 .
public static bool SavePhoto( PXCMSession session, PXCMCapture.Sample sample )
Saves the photo to disk. Uses this session to create a new PXCMPhoto object with save to disk functionality. The PXCMPhoto object uses import from the preview sample to import image data into itself. Find out if a file exists; if so, delete it and save the file.
public static PXCMPhoto OpenPhoto( PXCMSession session )
Simple function: checks if the XDM photo exists on the hard disk; if so, use the
PXCMSession object to create PXCMPhoto. The photo object then loads the XDM file. The function then returns PXCMPhoto to the client.
Rspaintutil
The RSPaintUtil class is a helper class that is responsible for drawing points and lines in photos. In addition, through the use of this class, duplication of code between different forms based on this functionality is reduced.
This is a good starting point. If present, creates a new .NET Point object, specifying the x, y values of the starting point.
Calls the
DrawCircle function to draw a circle around the point of the click.
static public void DrawTwoPointsAndLine( RSPoints points, PaintEventArgs e )
Checks that both points are valid and creates two new .NET Point objects. This function draws circles at these points, calling DrawCircle for each point. Then a line is drawn between them using DrawLine.
static private void DrawCircle( Point p, PaintEventArgs e )
This function draws a circle around a .Net Point object with x, y coordinates. This is done by creating a new Pen object. To draw a circle, you need a rectangle. It determines the size of the circle to draw. For this, I created a function that draws a bounding box. After creating the rectangle, I use the
DrawEllipse function and draw event arguments to draw a circle the size of a rectangle.
static private void DrawLine( Point pointA, Point pointB, PaintEventArgs e )
As in the case with a circle, you need to create a .NET Pen object, select its mode and draw a line between the start and end points using the
DrawLine function and event arguments.
static public Rectangle BuildBoundingRectangle( Point p )
Creates a .NET rectangle object whose center is a point with x, y coordinates in p. For this, a new Rectangle object is created. I wanted the bounding box to be 10 x 10 pixels. The value 10 x 10 I chose arbitrarily.
Rspoints
RSPoints is a simple shell for managing two different possible points. The dots indicate where the user clicked the PXCMPhoto photo that is displayed in the .NET PictureBox control.
It uses two PXCMPointI32 objects that represent the starting and ending points. In some cases, the
RSPoints instance will only need a starting point, for example for the functionality of
RefocusOnPoint . In other cases, two valid points are needed for various functions, such as MeasurePoints, where a start point and an end point are needed.
This class works in two modes: in single point mode, if actions are performed for which only one valid point is sufficient, or in multipoint mode, if available start and end points are required, for example, for
MeasurePoints .
public RSPoints( )
The constructor turns on single point mode, then calls
ResetPoints to reset all x, y values.
public void AddPoint( int x, int y )
This is about the same as adding an object to an array or to a list. However, as you can see, there is neither an array nor a list. Just two points. I decided to make this class similar to the list, so I decided to add an array for points. Is this class well thought out? Perhaps not very well, but so far it doesn’t worry me much: it’s just a supporting class, it does what I need, and the rest is not so important.
In single point mode,
AddPoints always replaces the starting point. This is done using the
ResetPoints object.
If we work with the mode of one point, then I do not need to add other points. It is better to clear the existing starting point and set a new one.
RSSampleArg
RSSAmpleArg inherits from
EventArgs . This class is intended for use with
RSStreaming . When
RSStreaming is streaming, an instance of this class will be created with each new frame and filled with data from the camera, then transmitted to the client using the event class.
public RSSampleArg( PXCMCapture.Sample sample )
The constructor initializes the local object
PXCMCapture.Sample _sample class with this parameter.
public PXCMCapture.Sample Sample
A simple getting method returns a local object
PXCMCapture.Sample .
RSEnhancedImageArg
RSEnhancedImageArg inherits from
EventArgs . This class is intended for use with
RSEnhancedPhotography . After
RSEnhancedPhotography completes the image change, an instance of
RSEnhancedImageArg is created, it is filled with the newly created image and sent to the client via the event class the client is subscribed to.
public RSEnhancedImageArg( PXCMImage image )
The form constructor initializes a single variable in this class — the PXCMImage object.
public PXCMImage EnhancedImage
A simple getter method returns an instance of PXCMImage.
RSMeasureArg
RSMeasureArg inherits from
EventArgs . This event is used in the
MeasurePoints function of the
RSEnhancedPhotography classes. After calculating the distance with the
MeasurePoints function
, I use an instance of this class to store the returned distance, validity, and accuracy data in it. After filling this object, it is used with the
Event object to send data to the client application.
public RSMeasureArg(float distance, float confidence, float precision)
Options
- Floating point number The measured distance between two points.
- Floating point number The confidence level of the measurement in the SDK.
- Floating point number The level of accuracy in the SDK.
The constructor fills the local data with input parameters.
public float Distance
A simple get method returns the distance between two points.
public float Confidence
A simple getting method returns the confidence level calculated when processing the distance between two points in the SDK.
public float Precision
A simple acquisition method returns the level of accuracy calculated when processing the distance between two points in the SDK.
Formmain
FormMain is the main form of the application. With its help, the user can turn on and stop the streaming, as well as take a picture, which will be used by other forms for various Enhanced Photography functions.
This form uses the
RSStreamingRGB object to stream data from the camera. The data is then displayed in the .NET PictureBox control using an Intel RealSense SDK helper object called
D2D1Render .
The form receives updates from
RSStreamingRGB by subscribing to the
OnNewStreamingSample event
RSStreaming .
public MainForm( )
This is a form constructor. It initializes global form objects, sets an event handler for data coming from
RSStreamingRGB , and sets the desired state of the buttons.
private void rsRGBStream_NewSample( object sender, RSSampleArg sampleArg )
Event handler for the
RSStreamingRGB object. Checks whether a color image should be viewed and passes the corresponding selection to the
_render object.
It then checks if a new photo needs to be taken; if necessary, use
RSUtility to save the photo to disk.
private void btnStream_Click( object sender, EventArgs e )
Event handler when you click the Stream button. Gives the
RSStreamingRGB object a command to start streaming, sets buttons according to the application state.
private void btnStopStream_Click( object sender, EventArgs e )
The Stop Streaming button event handler calls the
StopStreaming function.
private void btnTakeDepthPhoto_Click( object sender, EventArgs e )
Event handler for snapshot button. Sets the snapshot flag to true so that the stream event handler saves the current image to disk, then instructs the form to stop streaming.
private void EnableEnhancedPhotoButtons( bool enable )
Sets the buttons according to the state of the application using an enable value.
Perhaps this function will surprise you because there is no multi-threaded processing. . ? , (
RSStreamingRGB ), , .
StartStreaming RSStreaming .
_senseManager.StreamFrames( false );
( Intel RealSense SDK) . - .
, , . Windows . , .
EnableEnhancedButtons , . , .
EnableEnhancedPhotobuttons . .
,
InvokeRequired else, . , .
private void EnableStreamButtons( bool enable )
,
EnableEnhancedPhotoButtons , : .
private void StopStreaming( )
, RSStreaming . , . .
.
private void btnExit_Click( object sender, EventArgs e )
Exit.
Close() .
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
FormClosing . ,
RSStreaming . , .
private void btnDepthResize_Click( object sender, EventArgs e ) private void btnDepthEnhance_Click( object sender, EventArgs e ) private void btnRefocus_Click( object sender, EventArgs e ) private void btnPasteOnPlane_Click( object sender, EventArgs e ) private void btnBlending_Click( object sender, EventArgs e )
. , , . ,
RSUtility.OpenPhoto .
OpenPhoto , . , , . Everything is quite simple.
private void btn_MouseEnter( object sender, EventArgs e )
Start Streaming, Take Photo Stop Streaming. , , Tag. , , , .
private void btn_MouseLeave( object sender, EventArgs e )
.
FormMeasure
FormMeasure , Enhanced Photography.
RSEnhancedPhotography Enhanced Photography Intel RealSense SDK.
public FormMeasure( PXCMPhoto photo )
. PXCMPhoto, .
OnImageMeasured .
private void InitStatStrip( )
. 0, 0 x, y.
private void rsEnhanced_OnImageMeasured(object sender, RSMeasureArg e)
OnImageMeasured RSEnhancedPhotography .
RSMeasuredArg , . .
private void pictureBox1_MouseClick( object sender, MouseEventArgs e )
. , . , , /. . , . , .
,
UpdatePointsStatus .
( ),
MeasurePoints(…) RSEnhancedPhoto , . , ,
ResetPoints() RSPoint .
invalidate picturebox, . , . .
private void UpdatePointStatus( )
; .
private void pictureBox1_MouseMove( object sender, MouseEventArgs e )
; , . x, y .
private void pictureBox1_Paint( object sender, PaintEventArgs e )
picturebox. .
, . , .
private void Cleanup( )
.
private void btnExit_Click( object sender, EventArgs e )
Exit .
private void FormMeasure_FormClosing( object sender, FormClosingEventArgs e )
. .
FormDepthEnhance
, ; picturebox . .
public FormDepthEnhance( PXCMPhoto photo )
, , picturebox , .
private void rdo_Click( object sender, System.EventArgs e )
. DepthEnhancement(…) RSEnhancedPhoto, , .
private void _rsEnhanced_OnImageProcessed( object sender, RSEnhancedImageArg e )
OnImageProcessed ,
RSEnhancedPhotography . picturebox renderer.
private void btnExit_Click( object sender, System.EventArgs e )
Exit .
private void Cleanup( )
, .
private void FormDepthScaling_FormClosing( object sender, FormClosingEventArgs e )
. .
FormDepthResize
. PXCMPhoto.
public FormDepthResize( PXCMPhoto photo )
. , , ,
OnImageProcessed .
private void btnResize_Click( object sender, System.EventArgs e )
DepthResize(…) RSEnahancedPhoto .
private void _rsEnhanced_OnImageProcessed( object sender, RSEnhancedImageArg e )
OnImageProcessed picturebox , renderer.
private void btnExit_Click( object sender, System.EventArgs e )
Exit. .
private void Cleanup( )
, .
private void FormDepthResize_FormClosing( object sender, FormClosingEventArgs e )
Cleanup.
FormDepthRefocus
FormDepthRefocus Enhanced Photography Intel RealSense SDK. , : , .
, .
public FormDepthRefocus( PXCMPhoto photo )
, .
OnImageProcessed picturebox.
private void pictureBox1_MouseClick( object sender, MouseEventArgs e )
picturebox. ,
RefocusOnPoint(…) .
RSPoints ResPoints() .
private void tbAperture_Scroll( object sender, System.EventArgs e )
. , , ,
RefocusOnPoint , : .
private void _rsEnhanced_OnImageProcessed( object sender, RSEnhancedImageArg e )
OnImageProcessed RSEnhancedObjects . renderer picturebox.
private void btnExit_Click( object sender, System.EventArgs e )
Exit. .
private void Cleanup( )
, .
private void FormDepthResize_FormClosing( object sender, FormClosingEventArgs e )
Cleanup.
FormDepthPasteOnPlane
: , . . Intel RealSense SDK , .
public FormDepthPasteOnPlane( PXCMPhoto photo )
, .
OnImageProcessed picturebox.
private void _rsEnhanced_OnImageProcessed( object sender, RSEnhancedImageArg e )
OnImageProcessed RSEnhancedObjects . renderer picturebox.
private void pictureBox1_MouseClick( object sender, MouseEventArgs e )
. , . , , /. . picturebox, .
, . , .
( ),
MeasurePasteOnPlane(…) RSEnhancedPhoto, . , ,
ResetPoints() RSPoint.
private void btnExit_Click( object sender, System.EventArgs e )
Exit. .
private void Cleanup( )
, .
private void FormDepthPasteOnPlane_FormClosing( object sender, FormClosingEventArgs e )
Cleanup.
FormDepthBlending
FormDepthBlending . , , , , Z , .
public FormDepthBlending( PXCMPhoto photo )
, .
OnImageProcessed picturebox.
private void pictureBox1_MouseClick( object sender, MouseEventArgs e )
picturebox. , — . , . , Blend(), PXCMPhoto.
private void Blend( )
, ,
DepthBlend RSEnhancedPhotograpy .
private void tbBlend_Scroll( object sender, EventArgs e )
.
Blend() .
private void _rsEnhanced_OnImageProcessed( object sender, RSEnhancedImageArg e )
OnImageProcessed RSEnhancedObjects . renderer picturebox.
private void btnExit_Click( object sender, System.EventArgs e )
Exit. .
private void Cleanup( )
, .
private void FormDepthBlending_FormClosing( object sender, FormClosingEventArgs e )
Cleanup.
Conclusion
, Enhanced Photography, SDK. , . Thanks for attention.