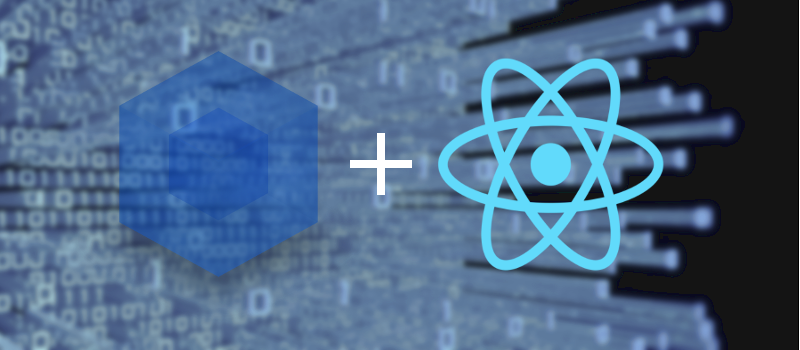
I did not find any clear instructions for building a webpack for production. Therefore, I decided to write this article. I hope it will come in handy.
There are many script collectors. I chose Webpack for myself according to the following criteria:
- Customization flexibility
- A large number of plug-ins and loaders
- Lazy loading
- Using es6 and es7 syntax using babel-loader
Among the shortcomings, I would highlight the lack of clear
documentation . For those who have never come across a Webpack, I recommend the
screencast from Ilya Kantor . In this article I want to talk about the build configuration of the project, written in React + Redux.
I will not go deep into bases, and I will tell, what plug-ins I use for assembly.
')
NoErrorsPlugin is a standard Webpack plugin that prevents scripts from being overwritten if they contain errors. This saves from the destruction of the old assembly as a consequence of non-working code in production. It is connected as standard to an array with plugins:
new webpack.NoErrorsPlugin()
EnvironmentPlugin - plugin for exporting the environment in client scripts, which is very convenient for debugging and logging. Connection:
new webpack.EnvironmentPlugin("NODE_ENV")
Use in code:
var env = process.env.NODE_ENV;
DefinePlugin - plugin for declaring its variables when building. For example, for cutting out pieces of common config code with a server.
Connection:
new webpack.DefinePlugin({ cutCode: JSON.stringify(true) })
Using:
if (typeof cutCode === 'undefined') {
CommonsChunkPlugin - makes shared libraries for chunks in a separate chunk. Convenient for caching external libraries and reducing the weight of chunks. What is chunks and Lazy loading is described
here . Connection:
new webpack.optimize.CommonsChunkPlugin({ children: true, async: true, })
DedupePlugin - finds common library dependencies and summarizes them. Connection:
new webpack.optimize.DedupePlugin()
UglifyJsPlugin - a plugin that compresses scripts. Parameters for this plugin can be found
here .
Connection:
new webpack.optimize.UglifyJsPlugin({
My version:
new webpack.optimize.UglifyJsPlugin({ beautify: false, comments: false, compress: { sequences : true, booleans : true, loops : true, unused : true, warnings : false, drop_console: true, unsafe : true } })
OccurrenceOrderPlugin is a plugin that minimizes the id used by the webpack to load chunks and other things. Connection:
new webpack.optimize.OccurrenceOrderPlugin()
CompressionPlugin is a third-party plugin for script compression, for example, in gzip format. Most browsers accept gzip files when setting headers for a Content-Encoding variable response: gzip. Parameters
here .
Installation:
npm install compression-webpack-plugin
Connection:
CompressionPlugin = require("compression-webpack-plugin"); plugins: [
WebpackShellPlugin - third-party plugin to run commands before and after assembly. For example, to clean old files after assembly.
Installation:
npm install webpack-shell-plugin
Connection:
new WebpackShellPlugin({ onBuildStart: ['echo "Webpack Start"'], onBuildEnd: [ `node ./node_modules/clean-scripts-after-build --path ${__dirname + '/public/js/'} --bundleName bundle_${config.jsVersion}.js` ] })
Separately, I want to highlight the connection babel-loader. He transpyls jsx, es6, es7 to es5 syntax.
Installation:
npm install babel-loader babel-preset-es2015 babel-preset-react babel-preset-stage-0
Connection:
wpConfig = { entry : ...., output : {
And yes, do not forget to use the production environment. The React and Redux code is written in such a way that the webpack cuts pieces of code that are not needed in production.
PS I got my bundle from 2.6 mb to 160 kb. I would be happy to see all the comments, since there can be a lot of variations of the settings.