Code reuse and compatibility for various platforms in our time is a rather topical issue. In addition, MSFT has recently been trying to please the developers of various platforms and programming languages. That is why, probably, the capabilities of the universal Windows platform allow using more than one type of library. Under the cut about which libraries, how and why you can use in applications UWP.
Class Library - Class Library for Windows Store Applications
.Net library with classes and methods available for Windows Store apps. Can only be used by UWP applications written in .Net languages. That is, JavaScript, for example, disappears.
Note that the .WWP application cannot use the usual .Net class libraries by itself.
The list of differences and changes that need to be made when converting .Net code to .Net for Windows Store applications can be found at:
.NET for Windows Store apps overview')
There are quite a few changes and differences, although there is also a certain similarity. Often, only the namespaces are different, and the methods and properties remain the same. This is done for the convenience of porting existing code. Of course, not all .Net functionality is available in Windows Store applications. Partly for security reasons or because of the fact that he did not want to duplicate it conceptually in UWP.
Class Library Portable - PCL (Portable Class Library)
Some libraries are available in these libraries, depending on which platform you choose. Target platforms are selected when creating a project.
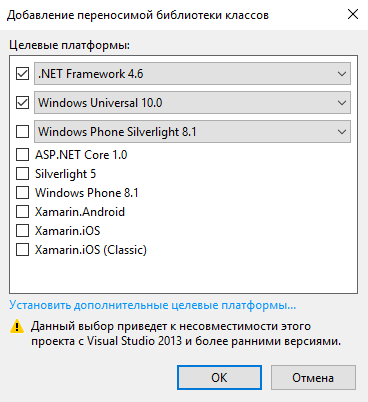
These target platforms can be changed at any time in the project properties.
Since the code will be one for multiple platforms, all types and members must be present in the selected platforms and must not have any platform specificity. Also they should not be deprecated or flagged for an exception.
Windows Runtime Component -Windows Runtime Component
A component that can be used with any of the WinRT platform languages. Component extension ends in .winmd. But let it not confuse you, the component is very often used as a library, and the metadata it contains allows it to work with all WinRT languages. This is the main advantage.
For example, I can show you how in the created Class Library project through properties you can select the Runtime Component as the result of the build:
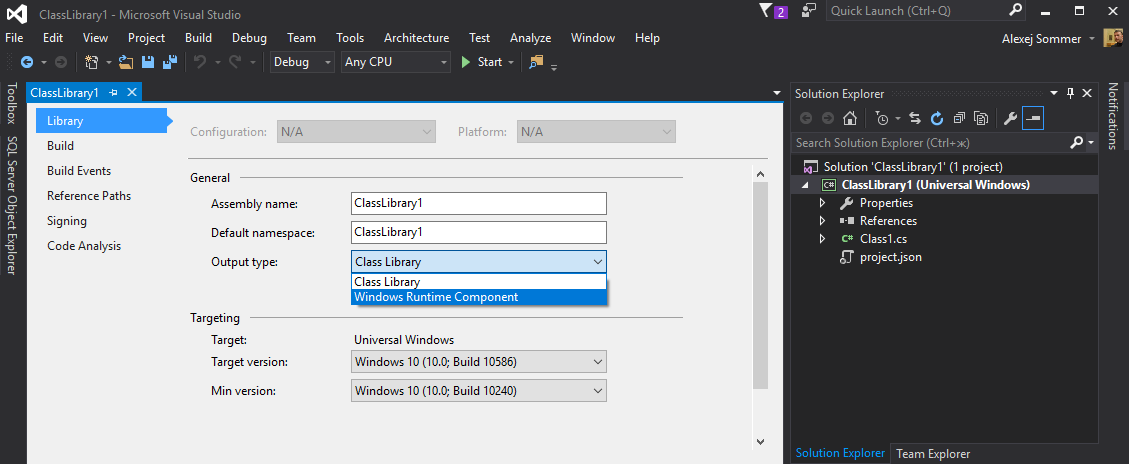
But in this case, you will lose cross-language compatibility and will be able to work with such a library only from C # and VB.
Runtime Component is based on COM. It turns out that there is an opportunity to write a native Windows Runtime component in a managed language.
All WinRT languages ​​use their own data types. But in the Runtime component, the data must be unified.
Primitive types are more or less similar in all languages. Mostly only JavaScript is different.
Table mapping can be found on the links:
Windows Runtime base data types.NET Framework mappings of Windows Runtime typesWinRT languages ​​are different and therefore it is sometimes necessary to come to some common denominator. For example, in .Net and JavaScript applications, strings are immutable, and in C ++ they are mutable. In the Windows Runtime component, the immutable lines.
Restrictions / rules:
- Public elements must be sealed.
- Public classes or interfaces cannot be generic or implement a non-WinRT interface.
- Public classes cannot be derived from WinRT types.
- All fields, parameters, and returned values ​​of public members must be of type Windows Runtime.
- The root namespace must match the name of the assembly and cannot begin with “Windows”.
- WRC does not support polymorphism.
- Methods in the Windows Runtime can be overloaded, but in the case of the same number of parameters they must be marked with an attribute. Official example:
public string OverloadExample(string s) { return s; } [Windows.Foundation.Metadata.DefaultOverload()] public int OverloadExample(int x) { return x; }
The Class Library for Windows Store applications has the least restrictions, so if you have an application in .Net languages, then it is better to choose this library.
Practical example:
If your UWP application uses WebView to display web sites, then you have the opportunity to use a class in your application that will be accessible from JavaScript in the downloaded web pages.
And this class must be located inside the Windows Runtime component. This is where cross-language compatibility is needed.
A component is created, and from the main project containing the WebView page, it is referred to. Inside a component, a public class or classes can be labeled with the [AllowForWeb] attribute. All public methods of such classes will be available from JavaScript loaded into the WebView page. It is necessary only at the beginning of navigation to the page to add this class using the
AddWebAllowedObject method.
Yes, of course, there is an option to use window.external.notify in the JS code, but there are certain security restrictions that allow these calls to be made only from trusted https sites.
I will repeat a little and I will duplicate the example with MSDN. In the class WinRT component add method SomeValueFromJS
using Windows.Foundation.Metadata; namespace MyRuntimeComponent { [AllowForWeb] public sealed class MyNativeClass { public void SomeValueFromJS(string value) { } } }
Add a webview in XAML
<WebView x:Name="webView" Source="https:// www.contoso.com/script.htm" NavigationStarting="webView_NavigationStarting"/>
And at the beginning of navigation we will implement our class in JavaScript
private void webView_NavigationStarting(WebView sender, WebViewNavigationStartingEventArgs args) { if (args.Uri.Host == "www.contoso.com") { webView.AddWebAllowedObject("csharpclass", new MyNativeClass()); } }
Now from the JS code, you can call this method like this:
csharpclass.nativeMethod("hello!");
It would seem that everything is normal, but bad luck, it may often be necessary to transfer data to a page from a WebView. The project with WebView already refers to the WinRT component and therefore we can’t add a backward link due to circular dependency. WRC restrictions do not allow us to use some options. As a solution, you can create an intermediate layer in the form of the Class Library for Windows Store applications, which has much less restrictions. A link to this library containing a class called BridgeClass can be made from the main project and from the project of the Windows Runtime component.
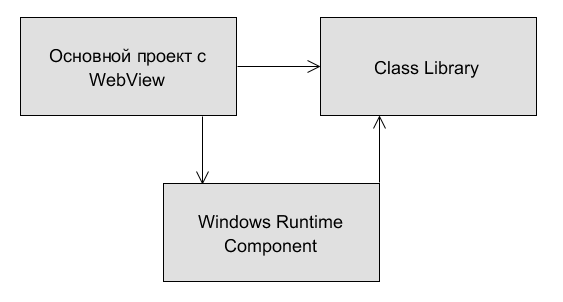
Opportunities .Net allow us to create affordable event
public static event Action<string>
and subscribe to an event in the main project. Let's look at the code. BridgeClass class code:
public class BridgeClass { public static event Action<string> MessageReceived; public static void Broadcast(string message) { if (MessageReceived != null) MessageReceived(message); } }
In the main project, in the code-behind page with WebView, subscribe to the event:
BridgeClass.MessageReceived += ShowMessage;
And we realize:
void ShowMessage(string msg) { }
Now from the method available for JS we can transfer the value to C #:
public void SomeValueFromJS(string value) { BridgeClass.Broadcast(value); }