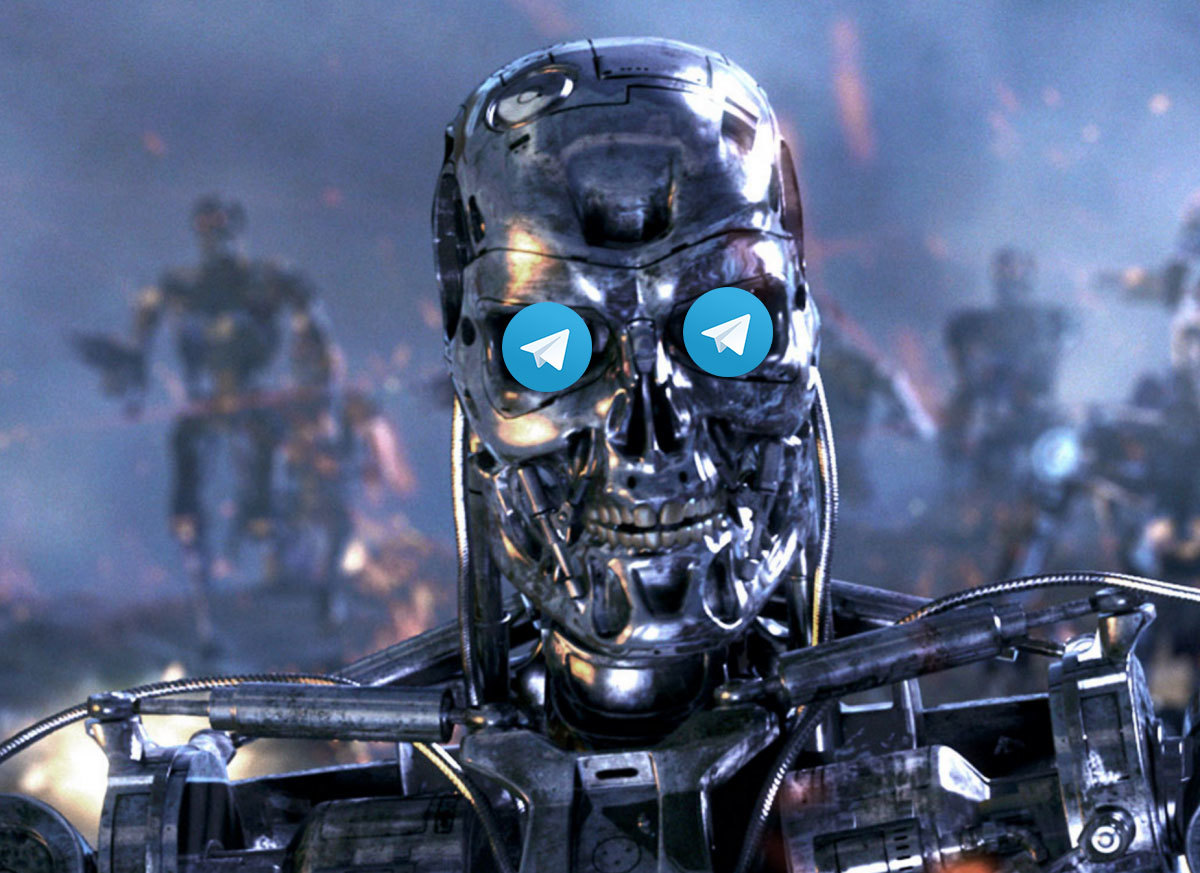
In this series of articles we are implementing a support service for online chats. The system must notify the team of operators about the new message, divide the load on the team of any size, delegate messages depending on priority. It sounds scary. But without panic, I will share our experience in building such a system that fit in just a couple of hundred lines of code. Yes, we will use Telegram-bot. But not because it is fashionable, but because it is super comfortable.
It all started with how we wanted to make a mobile application, where people can write any question in the chats of the establishments around and get a quick answer. Making a chat is not a problem. But what if we want to receive notifications about new messages in real-time, for an instant response to the user?
')
In the first part of the article, I will show on a test example how you can manage the help desk system through a chat bot, why this is a very simple and convenient way. And in the second part of the article we will implement this example in Python.
Problem and solution
Any team of support service operators must respond to inquiries promptly; for this, it must be promptly notified. There is no need to invent something of your own - any modern instant messenger will cope with this task with a bang. I chose Telegram. It is convenient, it works on all major platforms, and the bots' functionality is ahead of even the titans of the messenger market by at least a year. What am I talking about bots?
In our case, the bot is an information channel to which you can easily connect people with whom you can interact with text commands. Once we have created a bot, it is easy to connect people to it, just throw a link to them. However, our bot is exclusively for internal use. We can not allow anyone to access this link. We can’t safely hide the link, but it’s easy to turn on the functionality with a special command with password confirmation.
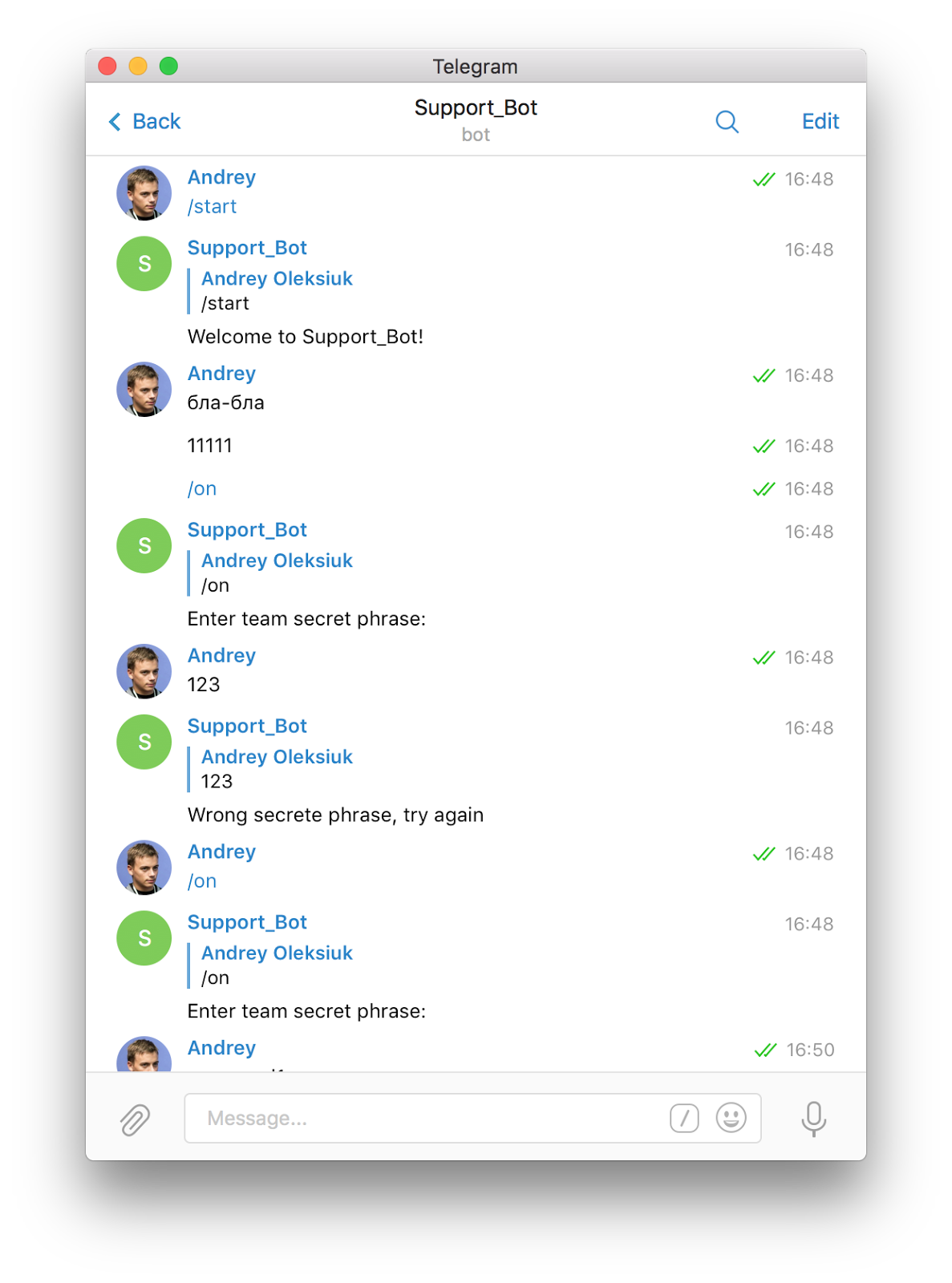
Telegram bot allows you to set your text commands for the bot. We do this: create a command "/ on" which "includes" the bot functionality for the user. But only after confirmation with a password! Well, just in case, you can provide the command "/ off", which disables the functionality.
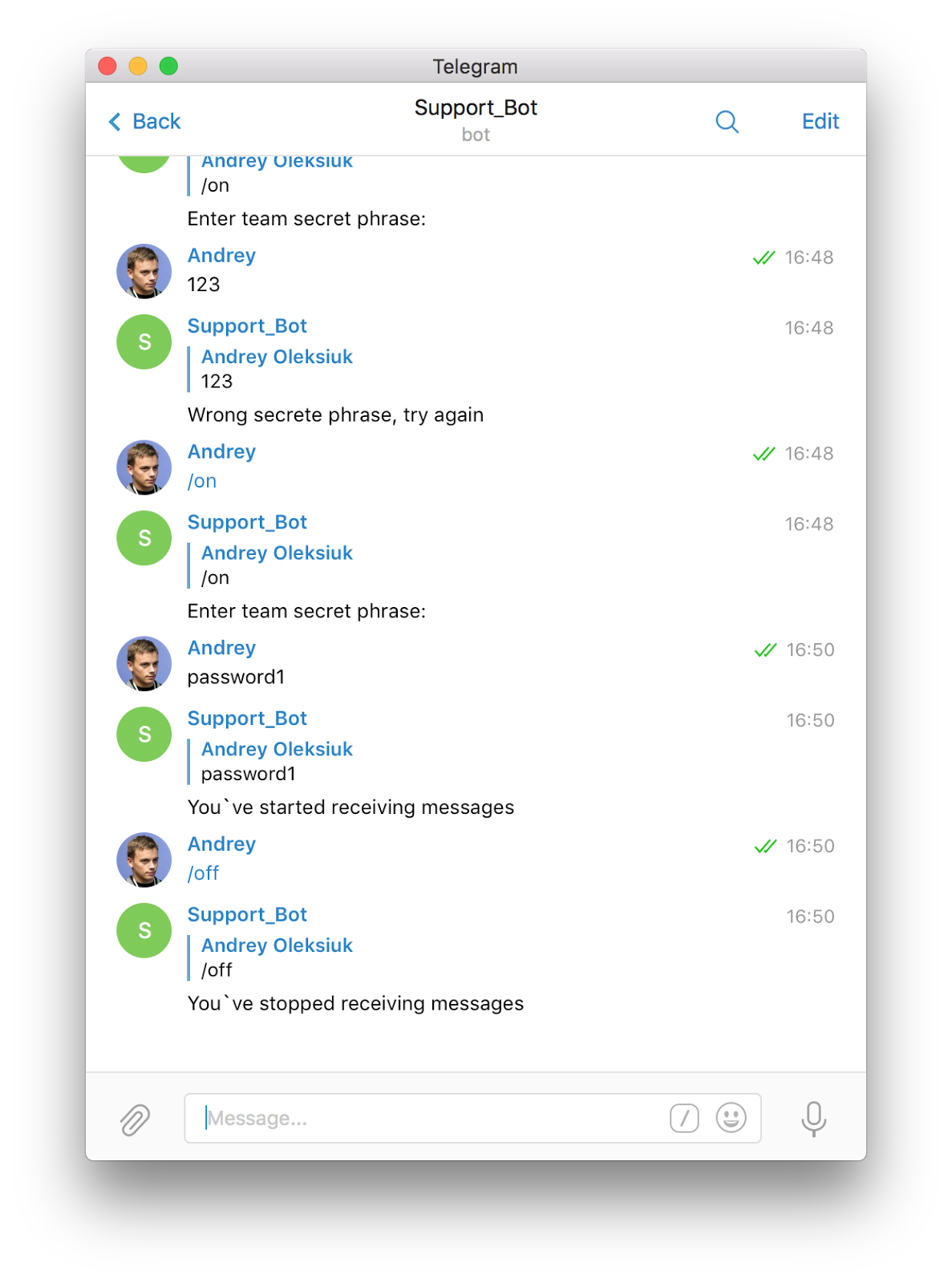
Now our bot knows a group of people - support service operators. For now, let's imagine that we have only one group of operators, each question from the client is very important, so we will send notifications to the whole group. There is no magic in this; of course, Telegram has api for sending messages to people who have connected to our bot.
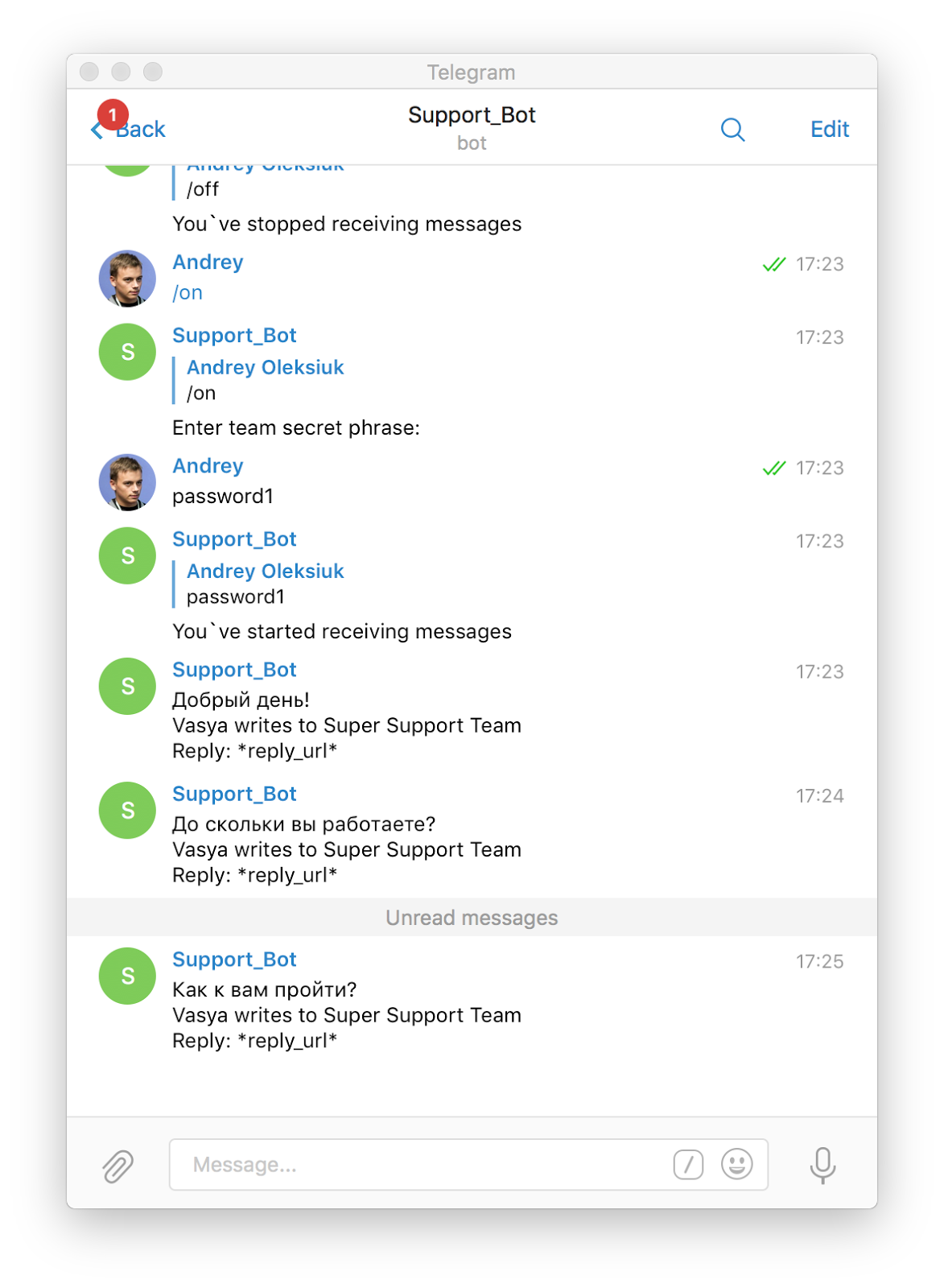
Now each member of the team of operators will receive a notification. In the text of the message, you can insert a link by which you can respond to the client. For example, it may be a link to the web version of the chat through which you provide support.
The system we developed works correctly when the support operator is alone. Of course, we can send notifications to any number of people. But how to understand that someone already responds to the client's request? How to distribute the load so that there is no confusion? We will do this in the next article using ... buttons in the chat. Not so long ago, Telegram published Bot Api 2.0. Now it became possible to add buttons to messages and track user clicks on them. What we will do in the next article. Well, a screenshot for the preview of what we will do.
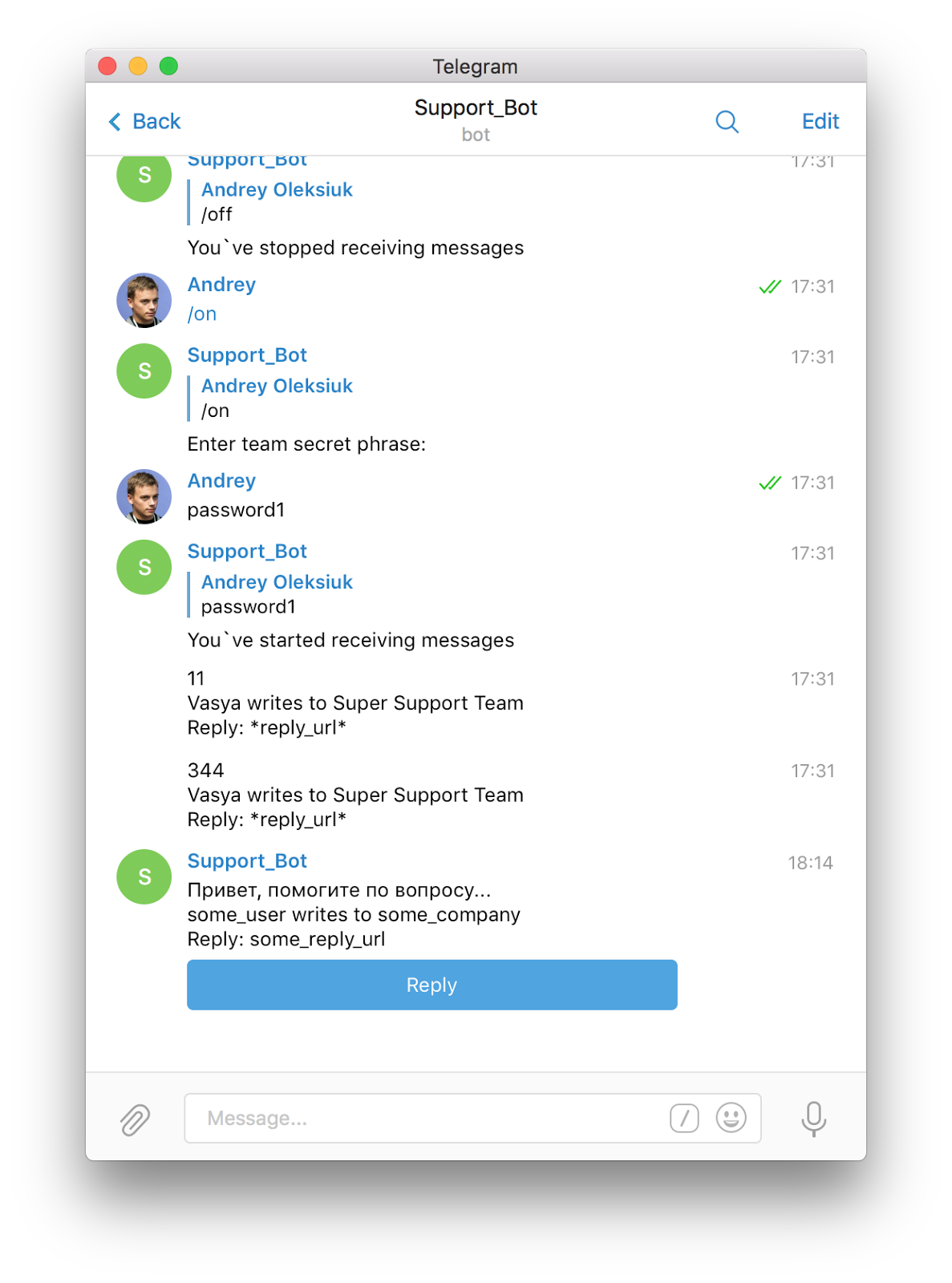
Implementation
Everything you need to create a bot can be read in the
documentation . After we created the bot, proceed to the code. We will use the Python
library - Telegram API wrapper. It allows you to write message processing in the usual functions with decorators, which is quite convenient. The full code of our test case is available
here , as the following articles will be published, I will update it.
@bot.message_handler(commands=['start', 'help']) def send_welcome(message): bot.reply_to(message, "Welcome to Support_Bot!")
This is the code that responds to the "/ start", "/ help" commands, and also welcomes new bot users (when the bot is first opened, the "/ start" command is automatically sent).
@bot.message_handler(commands=['on']) def subscribe_chat(message): if message.chat.id in team_users: bot.reply_to(message, "You are already an operator") else: user_step[message.chat.id] = TEAM_USER_LOGGING bot.reply_to(message, "Enter team secret phrase:")
This is the processing of non-standard commands ("/ start" and "/ help" is the default for all bots). We created a handler for the "/ on" command. After processing, we ask you to enter a password.
@bot.message_handler(func=lambda message: user_step.get(message.chat.id) == TEAM_USER_LOGGING) def team_user_login(message): if message.text == 'password1': team_users.add(TeamUser(message.chat.id)) user_step[message.chat.id] = TEAM_USER_ACCEPTED bot.reply_to(message, "You`ve started receiving messages") else: bot.reply_to(message, "Wrong secrete phrase, try again")
This function checks the password for validity. But how to understand that the last message was a password? In the handler "/ on" we save the status of the dialog in a global variable. Decorators of message handlers can receive lambda functions that receive incoming messages and if the lambda function returns True, go to the handler. In fact, in our case, the message text is not so important, but we check the status of the global variable. If the user previously called the "/ on" command, then it is necessary to interpret his message as a password. If the password passes verification - save the so-called chat id somewhere, for example, in a file. Using this id, we will later send messages to the chat operator.
@bot.message_handler(commands=['off']) def team_user_logout(message): if message.chat.id not in team_users: bot.reply_to(message, "You are not an operator anyway") else: team_users.remove_by_chat_id(message.chat.id) bot.reply_to(message, "You`ve stopped receiving messages")
Similarly, we implement the command to disable the operator who no longer wants to receive notifications.
def process(message): text = '%s\n%s writes to %s\nReply: %s' %\ (message, 'Vasya', 'Super Support Team', '*reply_url*') for user in team_users: bot.send_message(user.chat_id, text, disable_web_page_preview=True)
Well, sending messages is even easier. Since we can transmit various necessary information in the messages, including links, we will not force the Telegram application to attempt to parse them, this will only interfere. Using the flag disable_web_page_preview = True Telegram will not try to go through our links and display a preview image in the chat.
threading.Thread(target=bot.polling).start()
We start the bot with one line (polling method, webhook-i are still available, but for starters, this will be enough for the head).
In this article, we made a bot, taught him to add operators only after entering a password, also taught him to send to everyone in the group of notification operators. I will continue the cycle and tell you how to remove the possible confusion “who responds to what request”, how to make the division of operators into groups, delegate messages to a specific group based on the bot, as well as answer customer questions without leaving the Telegram chat. We use a similar system in our application (
Android and
iOS ). And, of course, we will study the new cool features of Telegram Bot API 2.0. I hope our experience will be useful.