June 9, 2016, jQuery 3.0 was officially released, which has been in development since October 2014. Our goal was to create an easier and faster version of jQuery (of course, with backward compatibility). We removed all the old crutches for IE and used some more modern web APIs where needed. jQuery 3.0 is a continuation of the 2.x branch, but with some changes that have long been wanted. Such branches as 1.12 and 2.2 will receive critical patches for some time, but you should not expect a new functionality in them. jQuery 3.0 is the future of jQuery. If suddenly you need support for IE 6-8, you can continue to use the release version 1.12.
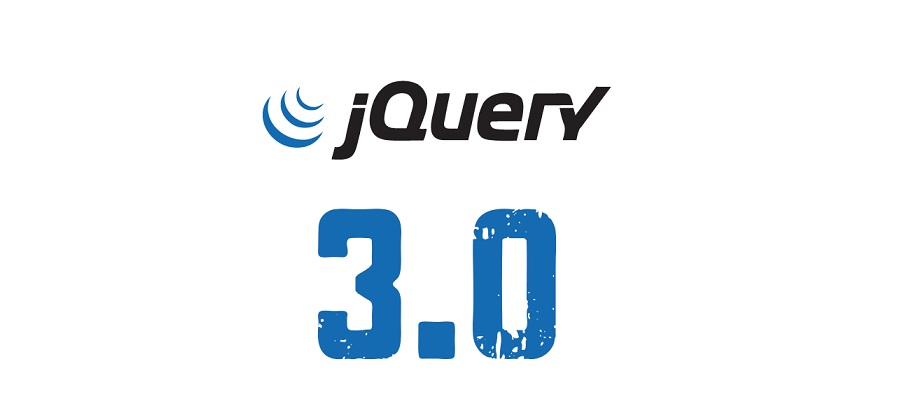
We expect that the upgrade of your projects to version 3.0 will not cause much trouble. Yes, there are several critical changes that justified the main feature of the version, and we hope that this will not greatly affect the update process.
')
To help upgrade, we added a
new upgrade
guide to version 3.0, and the
jQuery Migrate 3.0 plugin will help you identify compatibility issues in your code. Your feedback on the changes will greatly help us and therefore, please try it on your current project.
Of course, jQuery 3.0 files are available from the CDN:
https://code.jquery.com/jquery-3.0.0.jshttps://code.jquery.com/jquery-3.0.0.min.jsYou can also install via npm:
npm install jquery@3.0.0
In addition, we have jQuery Migrate 3.0 release. We strongly recommend using it to troubleshoot issues with modified functionality in jQuery 3.0. Files are also available in CDN:
https://code.jquery.com/jquery-migrate-3.0.0.jshttps://code.jquery.com/jquery-migrate-3.0.0.min.jsAnd in npm:
npm install jquery-migrate@3.0.0
For more information about upgrading jQuery 1.x and jQuery 2.x branches on jQuery 3.0 with the jQuery Migrate plugin, read the
jQuery Migrate 1.4.1 post.
Slim build
We finally added something new to this release. If you do not need AJAX, or prefer to use one of the many libraries focused on AJAX requests, and it is also easier to use a combination of CSS with class manipulations for the whole animation, then along with the regular jQuery version, including AJAX and effect modules, we release "Thin" version that does not contain them. In general, this code is considered obsolete and we just threw it away (just kidding). Nowadays, the size of jQuery is very rarely worried about performance, but the thin version is as much as 6 KB less than usual - 23.6k versus 30k.

These files are also available in the CDN:
https://code.jquery.com/jquery-3.0.0.slim.jshttps://code.jquery.com/jquery-3.0.0.slim.min.jsThis assembly is created using the custom API assembly, which allows you to include or exclude any modules. For more information, read
jQuery README .
Compatible with jQuery UI and jQuery Mobile
Most of the methods will work, but there are a few things that we will implement soon in jQuery UI and jQuery Mobile. If you find a problem, keep in mind that it may have already been published earlier and has been eliminated with the help of the
jQuery Migrate 3.0 plugin. Expect releases soon.
Big changes
This article only highlights the new features, enhancements, and fixes. More details can be found in the
instructions for the upgrade . The full list of fixed issues is available in our
bug tracker on GitHub . If you read the 3.0.0-rc1 blog, the features below have not changed.
jQuery.Deferred is now Promises / A + compatible
JQuery.Deferred objects have been updated for compatibility with Promises / A + and ES2015 Promises and verified using
Promises / A + Compliance Test Suite . This means that in the
.then () method you need to make a few significant changes. Of course, you can restore any use of
.then () by renaming it to
.pipe () , now considered obsolete (and having the same signature).
1 fix
Added a callback function (callback) to
.then ( ). Previously, you had to raise an exception to execute the callback function. In this case, any data based on the return of the answer will never return as an exception.
Example: uncaught exceptions vs. rejection values var deferred = jQuery.Deferred(); deferred.then(function() { console.log("first callback"); throw new Error("error in callback"); }) .then(function() { console.log("second callback"); }, function(err) { console.log("rejection callback", err instanceof Error); }); deferred.resolve();
2 fix
Previously, when registering "first callback", an error was received and all subsequent code stopped working. Neither the second callback nor the third were registered. The new, compatible with the standards "kolbek", if successful returns
true .
err is the failure value of the first callback.
The permission state Deferred created by .then () is now monitored by exception callbacks returning values ​​and non-thenable. In the previous version, rejection values ​​were returned.
Example: returns from rejection callbacks var deferred = jQuery.Deferred(); deferred.then(null, function(value) { console.log("rejection callback 1", value); return "value2"; }) .then(function(value) { console.log("success callback 2", value); throw new Error("exception value"); }, function(value) { console.log("rejection callback 2", value); }) .then(null, function(value) { console.log("rejection callback 3", value); }); deferred.reject("value1");
Earlier, the log contained “rejection callback 1 value1”, “rejection callback 2 value2”, and “rejection callback 3 undefined”.
Compatible with new standards, the method will write logs of the form: “rejection callback 1 value1”, “success callback 2 value2 ″, and“ rejection callback 3 [object Error] ”.
3 fix
Callback is always called asynchronously, even if Deferred was returned. Previously, they were synchronous.
Example: async vs sync var deferred = jQuery.Deferred(); deferred.resolve(); deferred.then(function() { console.log("success callback"); }); console.log("after binding");
Previously, the log contained “success callback” then “after binding”. Now it will look like “after binding” and then “success callback”.
ATTENTION! While caught exceptions have debugging advantages in the browser, this is a much more “friendly” method to investigate the cause of callbacks. Keep in mind that this always imposes a responsibility on you to add at least one callback to handle failures. Otherwise, errors may go unnoticed ...
We have developed a Deferreds compatible plug-in to help with debates - Promises / A +. If you do not see the necessary error information in the console to determine its source, check whether the
jQuery Deferred Reporter Plugin plugin is installed.
jQuery.when has also been updated to accept any thenable object that includes its own Promise objects.
https://github.com/jquery/jquery/issues/1722https://github.com/jquery/jquery/issues/2102Added .catch () to Deferreds
The
catch () method has been added as an alias for
.then (null, fn) .
https://github.com/jquery/jquery/issues/2102In case of errors, the main thing is not to be silent.
Perhaps you have ever asked the crazy question "what is the window shift?".
In the past, jQuery sometimes tried to return such a thing instead of error handling. In this particular case, it asks to move the window until it is in the position
{top: 0, left: 0} . With jQuery 3.0, such cases will throw errors, ignoring these reckless responses.
https://github.com/jquery/jquery/issues/1784Removed obsolete event aliases
.load ,
.unload and
.error removed. Use
.on () instead .
https://github.com/jquery/jquery/issues/2286Now using animation
requestAnimationFrameOn platforms that support the
requestAnimationFrame API, which is now everywhere, except IE <= 9 and Android <4.4, jQuery will now use it to implement animation. This should increase the smoothness of rendering, and reduce the amount of CPU time spent, therefore, saving battery power on portable devices.
Using
requestAnimationFrame a few years ago would have created
serious compatibility problems with existing code, so we had to eliminate it at that time. Now there is the possibility of pausing the execution of the animation at the time when the browser tab "goes" out of sight. For example, switching to another tab. However, any code that depends on animation always runs in near real time and creates an unrealistic load.
Massive accelerators for some custom jQuery selectors
Thanks to the detective work of Paul Irish from Google, we were able to identify some cases where we missed a lot of work with user selectors like, for example
,: visible was used many times in the same document. This rare case allows you to speed up the work up to 17 times!
Keep in mind that even with this improvement, using the
: visible and
: hidden selectors can be expensive, because they depend on the browser, which determines whether it is visible on the page now. This may require, at worst, a full CSS recalculation and page layout! At that time, while we did not hinder their use, we recommend checking your pages to find performance problems.
These changes actually turned it into 1.12 / 2.2, but we would like to improve it in jQuery 3.0.
https://github.com/jquery/jquery/issues/2042As mentioned above,
the upgrade guide is now available to anyone who wants to use this version. In addition to the basic instructions on the upgrade, it also includes a more detailed description of the remaining changes.
Changelog
List of changes hereBrowser support
- Internet Explorer: 9+
- Chrome, Edge, Firefox, Safari: current and previous versions
- Opera: current version
- Safari Mobile iOS: 7+
- Android 4.0+
Ajax
Attributes
- Avoid infinite recursion on non-string attributes ( # 3133 , e06fda6 )
- Added support comment and fixed hook with link @ tabIndex ( 9cb89bf )
- Collapse / expand the space for assigning values ​​when selecting ( # 2978 , 7052698 )
- Removed parent redundancy check ( b43a368 )
- Fixed selected option in IE <= 11 ( # 2732 , 780cac8 )
CSS
- In IE 11 does not work inside the iframe in full screen mode ( # 3041 , ff1a082 )
- Switch individual items as visible if they do not have the display: none parameter ( # 2863 , 755e7cc )
- Make sure elem.ownerDocument.defaultView is not null ( # 2866 , 35c3148 )
- Add an animation counter iteration in cssNumber ( # 2792 , df822ca )
- Restore cascade override behavior in .show ( # 2654 , # 2308 , dba93f7 )
- Stop Firefox from disabling disabling items as hidden-cascading ( # 2833 , fe05cf3 )
Core
Deferred
- Separate the two paths in jQuery.when ( # 3029 , 356a3bc )
- JQuery.when raw casts ( # 3082 , 7f1e593 )
- Remove default callback context ( # 3060 , 7608437 )
- Warn on exceptions that are likely programming errors ( # 2736 , 36a7cf9 )
- Propagate progress correctly from unwrapped promises ( # 3062 , d5dae25 )
- Make jQuery.when synchronous when possible ( # 3100 , de71e97 )
- Remove undocumented progress notifications in $ .when ( # 2710 , bdf1b8f )
- Give better stack diagnostics on exceptions ( 07c11c0 )
Dimensions
- Add tests for negative borders & paddings ( f00dd0f )
Docs
- Fix various spelling errors ( aae4411 )
- Update support comments related to IE ( 693f1b5 )
- ( 5430c54 )
- Updated links to https where they are supported. ( b0b280c )
- Update support comments to follow the new syntax ( 6072d15 )
- Use https where possible ( 1de8346 )
- Use HTTPS URLs for jsfiddle & jsbin ( 63a303f )
- Add FAQ to reduce noise in issues ( dbdc4b7 )
- Add a note about loading source with AMD ( # 2714 , e0c25ab )
- Add note about code organization with AMD ( # 2750 , dbc4608 )
- API tenets ( # 2320 , 6054139 )
Effects
Event
- Allow constructing a jQuery.Event without a target ( # 3139 , 2df590e )
- Add touch event properties, eliminates need for a plugin ( # 3104 , f595808 )
- Add the most commonly used pointer event properties ( 7d21f02 )
- Remove fixHooks, propHooks; switch to ES5 getter with addProp ( # 3103 , # 1746 , e61fccb )
- Make event dispatch optimizable by JavaScript engines ( 9f268ca )
- Evaluate delegate selectors at add time ( # 3071 , 7fd36ea )
- Cover invalid delegation selector edge cases ( e8825a5 )
- Fix chaining .on () with null handlers ( # 2846 , 17f0e26 )
- Remove pageX / pageY fill for event object ( # 3092 , 931f45f )
Events
- Don't execute native stop (Immediate) Propagation from simulation ( # 3111 , 94efb79 )
Manipulation
Offset
- Resolve strict mode ClientRect “no setter” exception ( 3befe59 )
Selector
Serialize
- Treat literal and function-returned null / undefined the same ( # 3005 , 9fdbdd3 )
- Reduce size ( 91850ec )
Support
Tests
- Take Safari 9.1 into account ( 234a2d8 )
- Limit selection to # qunit-fixture in attributes.js ( ddb2c06 )
- Set Edge's expected support for clearCloneStyle to true ( 28f0329 )
- Fix Deferred tests in Android 5.0's stock Chrome browser & Yandex.Browser ( 5c01cb1 )
- Add additional test for jQuery.isPlainObject ( 728ea2f )
- Build: update QUnit and fix incorrect test ( b97c8d3 )
- Fix manipulation tests in Android 4.4 ( 0b0d4c6 )
- Remove side-effects of one attributes test ( f9ea869 )
- Account for new offset tests ( f52fa81 )
- Make iframe tests wait after checking isReady ( 08d73d7 )
- Refactor testIframe () for DRYer and more consistent ( e5ffcb0 )
- Weaken sync-assumption from jQuery.when to jQuery.ready.then ( f496182 )
- Test element position outside view ( # 2909 , a2f63ff )
- Make the regex catching Safari 9.0 / 9.1 more resilient ( 7f2ebd2 )
Traversing