Attention! Be careful when upgrading PHP to version 7.0.6! This version fixes several important bugs that your code or the code of your framework could implicitly rely on.
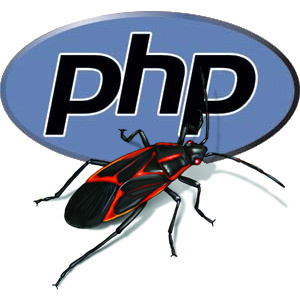
')
What bugs have been fixed?
bugs.php.net/bug.php?id=62059bugs.php.net/bug.php?id=69659bugs.php.net/bug.php?id=71359Test case:
The most concise can be taken from the description of the last bug (
www.pastebucket.com/97499 in the original)
class Test { protected $attributes = [ 'attribute1' => 'value1', ]; public function __get($name) { print "GET\n"; if (array_key_exists($name, $this->attributes)) { return $this->attributes[$name]; } trigger_error("Property $name does not exist"); return null; } public function __isset($name) { print "ISSET\n"; return array_key_exists($name, $this->attributes); } } $obj = new Test(); var_dump($obj->attribute1 ?? 'default');
Is it easier?
Yes.
In certain cases, when using constructions of the isset () and empty () language, the
__isset () magic method call was
not used when it was in the class, but instead __get () was called immediately and the decision was made based on what it returns.
Now it is fixed. One can hope that in all cases of using isset () or empty () on “magic” properties or “magic” keys of an ArrayAccess object array, it will first be called __isset ().
What does this threaten me personally?
The fact that if your class has a __get () method, but no __isset () method, your code will “break”. From now on, in all such cases, isset () will always return false, and empty () === true
What to do?
I would prefer to roll back to 7.0.5, and then accurately refactor the code. It is unlikely that you have many places where there is __get (), but there is no __isset (). Subsequently, it would be nice to add a corresponding rule to your code analyzer and prohibit committing such a toxic code.