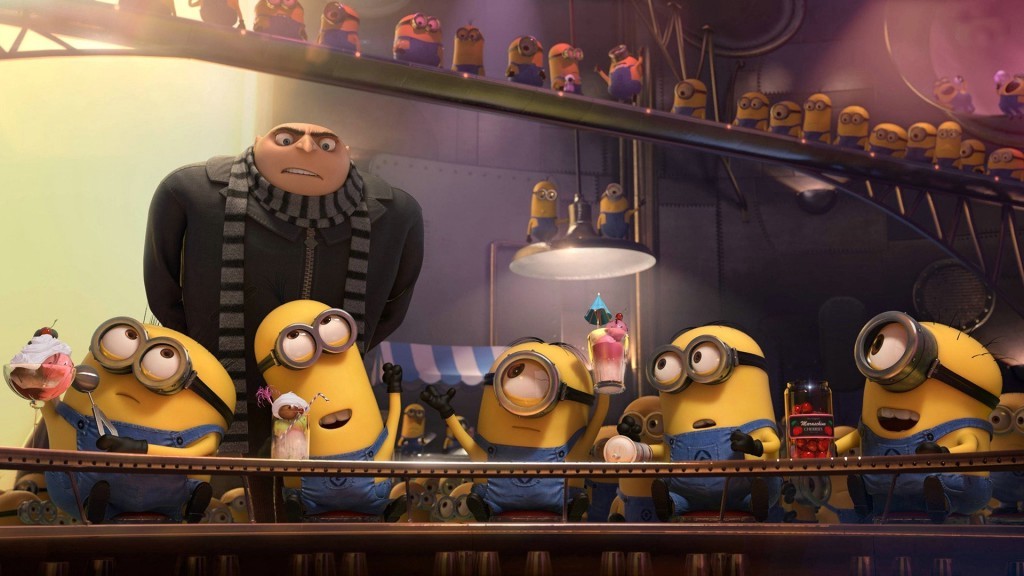
Callbacks Asynchronous. Non-blocking. Let's be honest: all these JS concepts make you tear the hair on your head every time your code AGAIN does not work. I also had such feelings. I needed some simple analogy that would help me more easily understand this abstract idea. Of course, there are many good learning materials online (for example,
this one , or
this one ). But they all usually start right away with fairly complex things.
I needed something closer, understandable.
')
I needed minions.
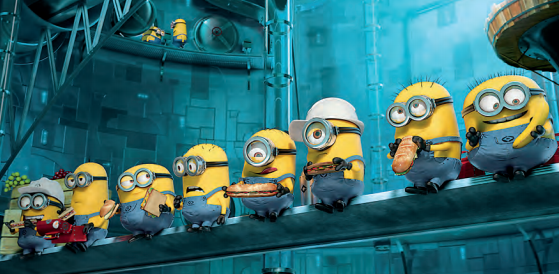
I'm going to explain to everyone who is callbacks, using the example of these funny creatures. In this analogy of my readers will act as the lord of the minions. You can tell them to do anything in your code. BUT!
- There is only one sovereign.
- Minions should receive orders from you. They cannot make their own decisions.
(The official definition of a minion: “someone weak and unimportant, executing the orders of a strong leader or boss”)main idea
Whenever you see “function ()” in jQuery or JavaScript
inside another function or method, imagine that it says “minion ()” instead. Of course, you cannot write like that, because JS does not recognize this command (unless you create a real minion function). But when creating a callback, you actually
give the order to the minions .
An example of minions waiting for your orders:
function myFunction(input, function(err, data){ });
This can be rephrased in:
function myFunction(input, minion(err, data){ });
An example with the usual function, without any minions:
function addOne(data){ return data++; };
JQuery examples
The basics
Example 1:
$('.myButton').click(function(){ $('.secondEl').show(); });
I remind you that this can be rephrased:
Example 2:
$('.myButton').click(minion(){ $('.secondEl').show(); });
What is a callback doing here?
You are an overlord, and you must look after events occurring within the entire file, or even several files. You don’t have time to mess with some jQuery click handler! Therefore, you assign this task to the minion, as shown in Example 2. Now this is a very simple function, you might even be able to do it yourself. And if it were 20 lines long? You can not be distracted by the 20-line function, because you need to accept other instructions from the user! Therefore, you tell the minion to start doing this from the very first line as soon as the user
.myButton
.
.myButton
. Now you can give orders to other minions, which is much more effective than doing everything yourself, forcing important functions to wait until you are free.

Animation
To emphasize the importance of minions, let's consider the sequence of display / hide.
$('button').click(function(){ console.log("One"); $('.firstChild').show(function(){ console.log("Two"); $('.childofChild').show(); }); console.log("Three"); });
If you read the code sequentially and do not attract minions to your side, then “One”, “Two”, “Three” will be displayed in the console. BUT, if you have minions, then “One”, “Three”, “Two” will be displayed on the console. And that's why:
$('button').click(minion(){ console.log("One"); $('.firstChild').show(minion(){ console.log("Two"); $('.childofChild').show(); }); console.log("Three"); });
- Line 1: you gave the order to the first minion and went to look at other events initiated by the user.
- Line 2: the first minion counted the state of the console and went to line 3.
- Line 3: the first minion called for the help of the second minion . This second minion should sit here and wait for the
show()
method to complete, after which it will be possible to continue executing instructions. So now two minions are working for you, simultaneously trying to complete their work with the function as soon as possible! - The first minion jumps to line 7, and the second one still has to execute lines 4 and 5. It reads the state of console.log, and it's done - no more work left. The second minion is a fraction of a millisecond behind, it reads console.log (“Two”), and then makes sure that a child
div
appears in line 5. Now this minion has completed its work.
From here you can take an incredibly important lesson: your callbacks
determine the order in which various actions are performed . Just imagine how powerful this tool is. You can accurately set the order of certain events, in contrast to the need to create one long line of consecutive commands. Callbacks give much more flexibility. If you could not get the minions to execute your orders, then you have to do everything yourself.
In fact, the above jQuery logic
only works for callbacks . For example, in a string, a child
div
should be displayed after displaying the parent
div
.
You cannot display the child if the parent is hidden. And callbacks are the only way to ensure that a child
div
appears
after the parent
div
.
If there were no callbacks in the above example, then line 5 would become the source of the error, since the
show()
method in line 3 had not yet managed to be executed. The first minion, launched on line 1, passes the task to the second minion as lines 4 and 5 are completed, so the
second minion can wait for the show()
method to complete on line 3 before starting work on lines 4 and 5. This ensures that the second minion will start and end the second
show()
after the first
show()
. By that time, the first minion will move to the remaining part of the external function, without waiting for the previous operations to be completed.

Vanilla examples from JavaScript / Node.js
Using parameters and callbacks
Now more complex examples! Lines 2 and 14 are just a declaration of functions, so let's move on to line 20, where the whole movement begins. Call the function
speakOrders
with two parameters. The first parameter is an object with states about which you want to receive reports from your minions. The second parameter is a callback called
reportOrders
.
Your minion cannot execute
reportOrders
until you give him such an order. This is exactly how this function is performed. Call
speakOrders
with the instructions on line 20. Let's go to line 14 and see what the function
speakOrders
. Obviously, she sends her instructions to the callback.
In line 20, the function
reportOrders
declared a callback, but it could be anyone.
memorizeOrders
,
tellMySpouse
, you can give the function any name. The use of the word “callback” in the declaration of the function in line 14 is considered good form so that other people can look at the code and understand what it is. But you can use any other word! Here is a minified example:
In both lines 14-15, there is only one minion, replacing the “callback”.
Line 20 : Call
speakOrders
. Pass the named object and destination. The second parameter can be anything - string, function, etc.
Line 14–15 : Determine that the second parameter should be a callback, this is minion (). Every time we call the function
speakOrders
we will know that the second parameter will be a function. In our case,
reportOrders
.
Line 15 : From line 20, we know that your minion should take care of the
reportOrders
function. He receives the parameters of the order - the object. He needs these instructions to create a successful report.
Line 2 : The order variable from line 15 is now referenced inside the function as
minionOrders
. The
reportOrders
function completes, and the name and destination are returned.
Here, callbacks play an important role in clearly tracking the path that an object must follow. Without them, the code would become a monolithic, strictly ordered heap, without any flexibility in terms of reusing functions or changing the order of execution.

Node.js
Take a look at the following example, which uses
Express and
a query module . So far this is the hardest!
var request = require('request'); var app = require('express')(); var results; function logRes(){ console.log(results); } app.get('/storeData', function(req,res){ readResult(logRes) }); function readResult(callback){ request('http://someroute.com/api', function(err, response, body){ results=body; callback(); }); }
Imagine that a user simply sent a GET request on the
/storeData
route. Let's start with line 9. In this example, all previously discussed uses of callbacks are used.
- In line 9 there is a callback in the method, similar to the one analyzed in the example about the click handler in jQuery.
- Line 14 uses asynchronous execution associated with a request for a fake API. This is from an example about animation in jQuery.
- Finally, in line 13, a callback parameter is announced, similar to the example with vanilla JS.
So that there is no misunderstanding, here is a minified example in which numbers are assigned to minions in accordance with the order of execution.
var request = require('request'); var app = require('express')(); var results; function logRes(){ console.log(results); } app.get('/storeData', minion1>(req,res){ readResult(logRes) }); function readResult(minion3){ request('http://someroute.com/api', minion2(err, response, body){ results=body; minion3(); }); }
- Line 9 : The user is sent along the route. You are the boss, you give orders to the first minion. That goes to line 10 and sees the
readResult
function. Now, while the minions are working, you can expect other signals from the user. - Line 14 : The first minion sees the request, sends it to the fake API and orders the second minion to wait for a response. Now the first minion can move on to another job. And since there is nothing more to be done, he retires.
- Line 14 : The second minion takes effect upon completion of the request. He now has three pieces of potentially important information:
err
, response
, body
. - Line 15–16 : The global variable “results” is set to body. Now this variable can be used in other functions. The second minion tells the third that it is time to process the instructions that the third minion received from line 10 . All this time, he was waiting for their execution, and now it's time to execute
logRes()
! - Line 5 : And the instructions are ... console.log. Disappointing. In any case, the third minion did its job.
So how was the third minion called after the second?
If we return to the very beginning, to the very first example, we will see the initialization of the callback on line 13. This means that every time you call the
readResult()
function,
readResult()
must be a callback parameter. Later this callback is applied in line 16, where it uses the result of the request to the API from line 14, because
the request itself has a callback !
Imagine that a callback (the third minion) would be below the 17th line, outside the scope of the request. In this case, it would be the second minion, since it would be executed until the completion of the request. In this case, the answer to the request would not have been received yet, which deprives the whole function of meaning. The point is to first execute the request, and then transmit the received response.
Once again: the use of two separate requests in the
readResult()
function allows you to make sure that the third minion starts working after the request is executed. Callback gives you control that allows you to give this particular order.
Conclusion
You are the lord of the minions, under your command of a horde of squeaking little servants, ready to fulfill your desires. If you can give them the right instructions, they will be able to make your life much easier, doing the hardest things for you.