Continuation of the electronic version of the article from issue No. 2 for 2016 of the magazine Components and Technologies . Posted by Andrey Kurnits. Link to the first partCreating, running and debugging a primitive program on the Atmel SAM4S microcontroller
Now that the software for development of Atmel SAM4S microcontrollers has been installed and configured on the workstation, you can make sure that the system is working by creating a simple program that will light and turn off the LED on the SAM4S-EK board.
In order to build a workable program for the microcontroller, in addition to the GCC tools, the following components are needed:
- The CMSIS (Cortex Microcontroller Software Interface Standard) library describes a single interface for interacting with the core of ARM Cortex-M microcontrollers - common for ARM Cortex-M microcontrollers of various manufacturers.
- The library for interacting with the periphery of this family of microcontrollers is different for each manufacturer, be it Atmel, STMicroelectronics, NXP, etc.
- The linker script is a file with instructions on how to place the program in the microcontroller’s flash memory, as well as on the service areas (sections) in RAM: stack, heap, etc. For different microcontrollers with different memory sizes, different linker scripts are used respectively.
- The syscalls.c file contains system functions necessary for the operation of the standard C library (implementation newlib). Usually these functions are part of the operating system for which the program is intended. However, in the case of a microcontroller, which most often works without an operating system, the syscalls.c file must be included in the assembly, which contains mostly empty system functions [10].
- In fairness it should be noted that there are many operating systems designed specifically for microcontrollers.
- The initial initialization code (startup code) is responsible for filling the interrupt vector table with corresponding handlers, including the microcontroller interrupt handler (reset handler).
- Also contains an implementation of the interrupt handler for resetting, which resets the necessary memory sections, initializes the standard C library and transfers control to the program's entry point - to the main () function.
Atmel Software Framework Library
To create programs for its microcontrollers, Atmel freely distributes the Atmel Software Framework library (ASF) for C / C + languages, which contains all 5 components from the list above that are required to build the program.
The ASF library provides a high-level interface, one for microcontrollers of different families and architectures: megaAVR, AVR XMEGA, AVR UC3 and SAM. The ASF library contains examples of working with various peripheral units of microcontrollers, as well as with external devices located on various evaluation boards.
Such a variety of supported microcontrollers adversely affected the ease with which the ASF library was mastered. The library turned out to be very complex, confusing and large in size (archive ~ 400 MB, containing more than 70,000 files).
Even though the ASF library can be downloaded separately [8] and it is designed to use the GCC compiler as well, however, the author failed to manually extract the necessary files from the library to create the simplest program for the Atmel SAM4S16C microcontroller.
The author suggests this way:
1.To get a minimal compiled project use the Atmel Studio IDE integrated development environment designed for Windows operating systems and based on Microsoft Visual Studio 2013.
2. In the future, "manually" add the necessary components to the resulting project by copying them from a separately loaded ASF library.
')
Atmel Studio IDE
The Atmel Studio IDE is free and can be downloaded from the official website [
9 ]. During installation, you should select the ARM architecture on the computer or virtual machine running Windows and specify whether you need to install the ASF library (Fig. 5).
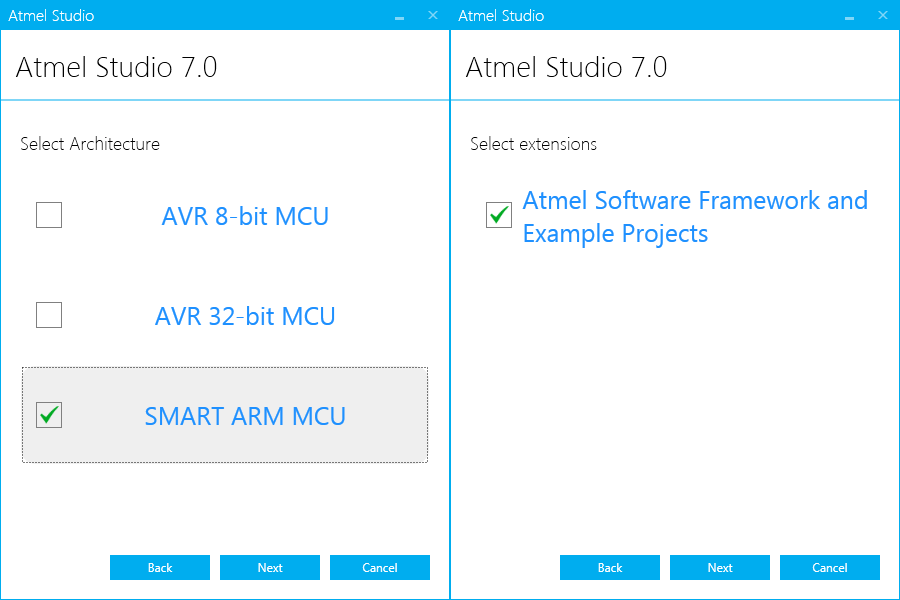
Fig. 5. Features installation Atmel Studio IDE.
After installation, you should run the Atmel Studio IDE and create a new project by selecting the menu item "File -> New -> Project ...". Next, select the project type “GCC C ASF Board Project”, as shown in Fig. 6
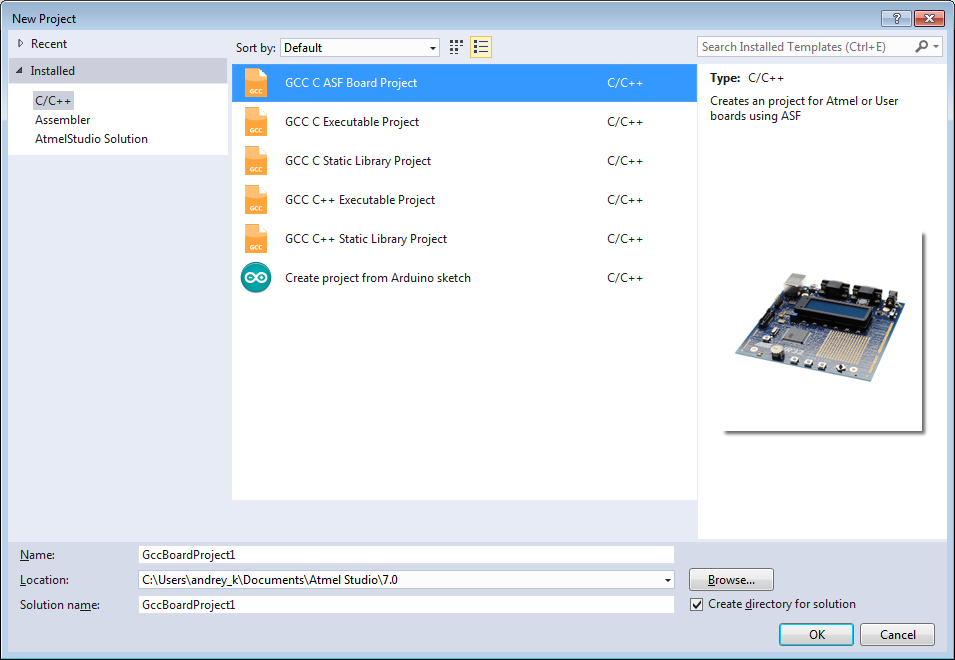
Fig. 6. Select the type of project in the Atmel Studio IDE.
Next, a window opens to select the hardware platform for which the project will be assembled (Fig. 7)

Fig. 7. Microcontroller selection
You can enter the name of the microcontroller, but in this case it is easier to set the name of the evaluation board. To do this, select the “Select by Board” item and select the name “SAM4S-EK” (Fig. 7). In the list below, select the item with the name of the microcontroller "ATSAM4S16C" and click "Ok".
After the project is created, the source code files will be placed in the previously specified (Fig. 6) directory (in the case of the author, c: \ Users \ andrey_k \ Documents \ Atmel Studio \ 7.0 \ GccBoardProject1 \ GccBoardProject1 \ src).
The src directory can now be transferred to the Linux operating system in the project directory, for example, to the ~ / sam / directory. Its structure is shown in fig. eight.

Fig. 8. The structure of the simplest project
Attention should be paid to the location:
- The linker script is the file ~ / sam / src / ASF / sam / utils / linker_scripts / sam4s / sam4s16 / gcc / flash.ld
- The initialization code and the interrupt vector table are located in ~ / sam / src / ASF / sam / utils / cmsis / sam4s / source / templates / gcc / startup_sam4s.c
- The syscalls.c file is located in ~ / sam / src / ASF / sam / utils / syscalls / gcc directory.
Add module from ASF library
At the moment, there is no delay.h component in the project, which is required to get delays for exactly the specified time (this is needed for the LED to “flash”). The following shows how as the project grows, add the necessary components from the ASF library — for example, the delay.h component. To add it, you need to copy from the separately downloaded archive of the ASF library [
8 ] the file common / services / delay / delay.h and the directory with all the files common / services / delay / sam / to the project directory ~ / sam / src / ASF / common / services / delay.
In addition, you must include the component delay.h in the assembly by adding a line at the end of the ~ / sam / src / asf.h file:
#include <delay.h>
As you can see, adding individual components from the ASF library to the project does not present much difficulty.
The code of the simplest program
The listing of the main.c file is shown below:
#include <asf.h> int main (void) { board_init(); sysclk_init(); while (1) { LED_On(LED1_GPIO); delay_ms(500); LED_Off(LED1_GPIO); delay_ms(500); } return 0; }
The board_init () function initializes the input / output (GPIO) ports of the microcontroller in accordance with the external electronic components connected to them, including configuring the input / output ports as outputs for turning on / off the LEDs located on the board. The board_init () function is in the src / ASF / sam / borads / sam4s_ek / init.c file.
You can choose which ports will be initialized, and which will not, using macros in the src / config / conf_borad.h file.
The sysclk_init () function is responsible for initializing the microcontroller clock unit. After reset, the built-in 4 MHz RC generator is turned on [
12 ]. The sysclk_init () function activates a generator operating from an external crystal oscillator at 12 MHz, and also adjusts the PLL phase locked loop so that the clock frequency of the microcontroller core is 120 MHz.
You can change the clocking settings by changing the macro definitions in the src / config / conf_clock.h file.
Then, in an infinite loop, the functions of turning on and off the evaluation LEDs are successively called: LED_On () and LED_Off (). As an argument, the LED1_GPIO macro is passed, which corresponds to the green LED connected to the port PA20. The LED_On (), LED_Off () and LED1_GPIO macros functions are defined in the source files in the src / ASF / sam / borads / sam4s_ek / directory, which contains, among other things, the description of connecting LEDs to the microcontroller pins.
Calling the function delay_ms (500), which is included in the previously added module delay.h, results in a delay of 0.5 seconds. The delay is implemented by empty microcontroller cycles, and there is no need to worry about accounting for the microcontroller clocking frequency - the current frequency is taken into account inside the ASF library after calling the sysclk_init () function.
Build with QBS Automation
To get the executable firmware file you need to compile a fairly large number of files. Atmel offers to use makefiles, which are instructions to the GCC compiler.
The author proposes to use QBS [1 ]’s build automation environment built into Qt Creator. To do this, you will need to create a QBS project file containing information about the source files to be compiled.
One of the advantages of QBS is that there is no need to manually enter the name of each file with the source code in the QBS file - it is enough to specify the directories of the files, the rest will be done by the system itself.
QBS text file import qbs import qbs.FileInfo import qbs.ModUtils Project { CppApplication { type: ["application", "ucfw" ] Depends { name: "cpp" } consoleApplication: true cpp.positionIndependentCode: false cpp.executableSuffix: ".elf" cpp.includePaths: [ "src", "src/ASF/common/boards", "src/ASF/common/services/clock", "src/ASF/common/services/clock/sam4s", "src/ASF/common/services/gpio", "src/ASF/common/services/gpio/sam_gpio", "src/ASF/common/services/ioport", "src/ASF/common/services/ioport/sam", "src/ASF/common/services/delay", "src/ASF/common/services/delay/sam", "src/ASF/common/utils", "src/ASF/common/utils/interrupt", "src/ASF/sam/boards", "src/ASF/sam/boards/sam4s_ek", "src/ASF/sam/drivers/pio", "src/ASF/sam/drivers/pmc", "src/ASF/sam/utils", "src/ASF/sam/utils/cmsis/sam4s/include", "src/ASF/sam/utils/cmsis/sam4s/include/component", "src/ASF/sam/utils/cmsis/sam4s/include/instance", "src/ASF/sam/utils/cmsis/sam4s/include/pio", "src/ASF/sam/utils/header_files", "src/ASF/sam/utils/preprocessor", "src/ASF/thirdparty/CMSIS/Include", "src/config" ] cpp.defines: [ "__SAM4SA16C__", "BOARD = SAM4S_EK" ] cpp.commonCompilerFlags: [ "-mcpu=cortex-m4", "-mthumb", "-mfloat-abi=soft" ] cpp.linkerFlags: [ "-mcpu=cortex-m4", "-mthumb", "-mfloat-abi=soft", "-Xlinker", "--gc-sections" ]
In case of a successful release of an assembly (release — that is, not for debugging, final), the QBS file contains instructions to perform the following actions:
- Print the size of the firmware to the console,
- convert firmware file from ELF format to Intel HEX format
- Upload the file to the target microcontroller via the SAM-ICE adapter using the OpenOCD program
Conclusion
As a result of the above actions, an ecosystem has been created for the development of Atmel SAM4S microcontrollers, consisting entirely of freely distributed programs. Ecosystem is easy to modify to use another ARM microcontroller from Atmel.
Download the finished project at [
11 ]. To use it, you will need the Ubuntu operating system or a derivative of it (Lubuntu, Kubuntu, Xubuntu) with the installed packages:
- GCC tools for ARM microcontrollers [1]
- Qt Creator Development Environment [1]
- OpenOCD program (installation described above)
Literature
- Kurnits A. Development for STM32 microcontrollers in the environment of the Linux operating system // Components and technologies. 2015. â„– 10.
- www.sourceforge.net/projects/openocd
- www.we.easyelectronics.ru/CADSoft/ubuntueclipse-code-sourcery-openocd-j-link-arm-ilibystryy-start-dlya-somnevayuschihsya.html
- www.microsin.net/programming/arm/openocdmanual-part1.html
- www.microsin.net/programming/ARM/openocdmanual-part2.html
- www.microsin.net/programming/ARM/openocdmanual-part3.html
- www.openocd.org/doc/html/General-Commands.html
- www.asf.atmel.com/docs/latest/download.html
- www.atmel.com/tools/ATMELSTUDIO.aspx
- www.sourceware.org/newlib/libc.html#Syscalls
- www.e-kit.ru
- www.atmel.com/images/atmel-11100-32-bit%20cortex-m4-microcontroller-sam4s_datasheet.pdf