If you have an android application that you are going to publish on Google Play or it has already been published, as well as if you are only developing it, and it is in closed beta testing, and the customer / tester periodically needs to collect and transfer the assembly by hand, it is perhaps better automate this process
You can independently understand the
documentation in English, but if the process is difficult or something does not work, I hope the publication will be useful
Before you begin, you must manually publish the first version of the application.
Access setting
Open the
Google Play Developer Console and go to
Settings →
API accessThe console must be linked to the Google API project. If you do not have a project, then at the moment only the button Create new project will be available from the interface.

')
If there is, then a list of these projects will be available. The link button, of course, binds them
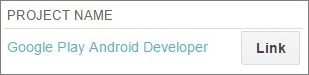
But let's consider an example with the creation of a new project. You can create it from the
Google API Console or directly from the Google Play Developer Console, which you already have, click the
Create new project button
If this is your first time creating a Google API project, a popup window will appear, here
Accept All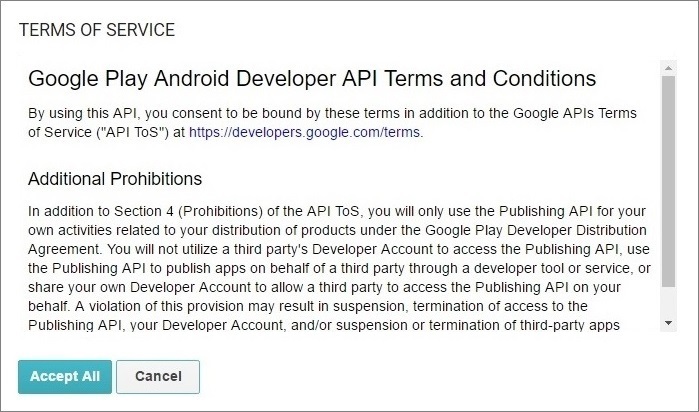
Great, now you have a project and a service account, which in this case is created automatically

Now you need to allow this account to work with Google Play and give it a role. This can also be done right from here, click
Grant access . You will be transferred to the User accounts & right menu (seen in the background) and a popup window will open, where the email and other required fields will already be filled out. Click
Add user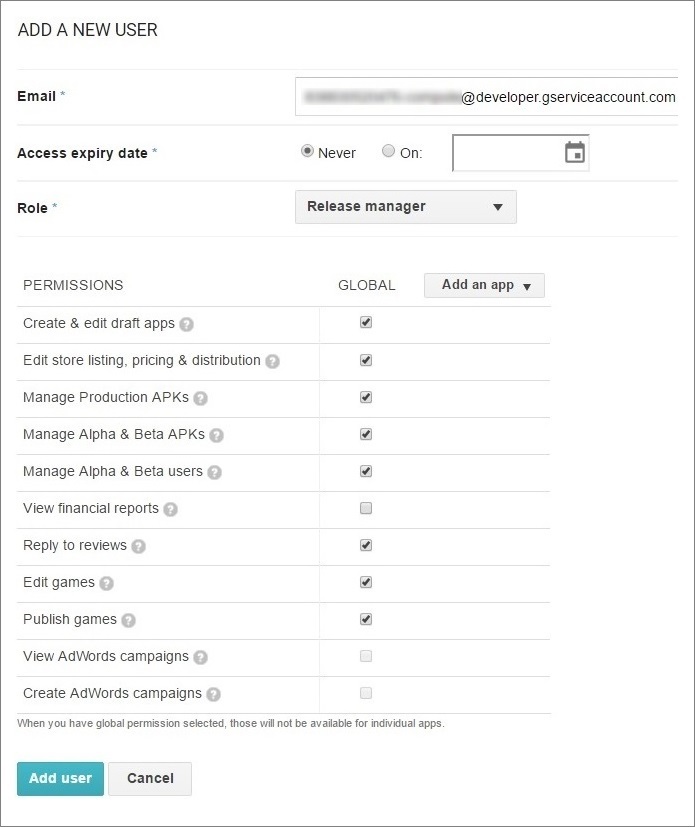
Now you see that your user has been successfully added.

Now you need to generate a pair of public and private keys so that you can work with libraries and publish new versions of the application. To do this, open the
list of projects in the Google API Console and click on the one you need, by default it is called
Google Play Android Developer
Then click the menu item
Credentials →
Create credentials , select
Service account key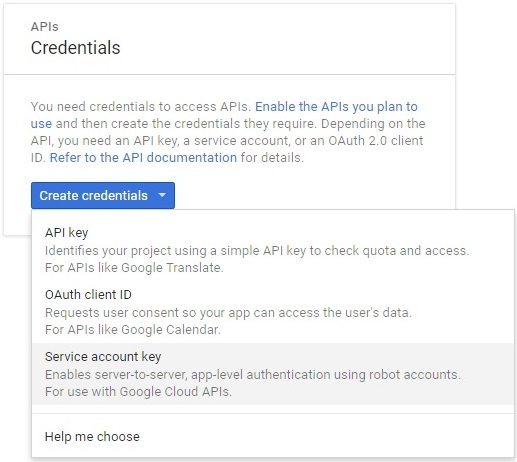
Select the
default service account from the
Compute Engine list and transfer the radio button to
P12 . Click
Create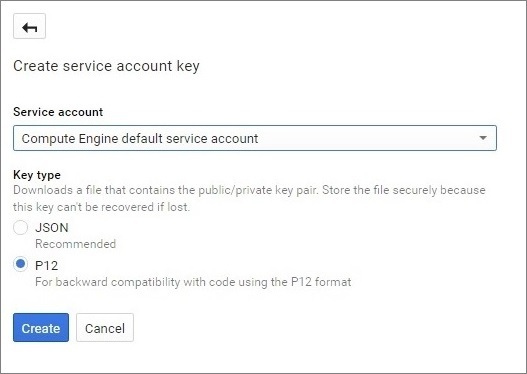
With all settings, a pair of public and private keys with the name
Google Play Android Developer-% id% .p12 slice was saved on the computer


Let's start programming
Now Google provides libraries in java and python to work with the Google Play Developer API. We will consider an option on java, for this
download the zip-archive with libraries and examples
Open your IDE and create a project, I called mine ProductionManager, connect the following libraries:
- google-api-client-1.19.0.jar
- google-api-services-androidpublisher-v2-rev20141111-1.19.0.jar
- google-http-client-1.19.0.jar
- google-http-client-jackson2-1.19.0.jar
- google-oauth-client-1.19.0.jar
- jackson-core-2.1.3.jar
Copy the
Google Play Android Developer file -
% id% .p12 piece into the resource folder of your project, I renamed it so that there were no spaces, it seems I had an exception when I tried to read it. If you keep the source code of this project in VCS where many people have access, it is probably better to store the file on a continuous integration server, here is at your discretion.
Before building and publishing the application, I make a request to find out the latest version of the code (versionCode) and update it, because I like them to go in an arithmetic progression with step 1 (those are just 1, 2, 3 ...), you can just substitute the buildNumber from the CI server or revesionNumber from your VCS, also at your discretion
Version updateprivate Edits getApplicationEdits() throws Exception { String applicationName = "ApplicationName"; String serviceAccountId = "--@developer.gserviceaccount.com" HttpTransport httpTransport = GoogleNetHttpTransport.newTrustedTransport(); JsonFactory jsonFactory = JacksonFactory.getDefaultInstance(); KeyStore keyStore = SecurityUtils.getPkcs12KeyStore(); InputStream inputStream = ProductionManager.class.getResourceAsStream("/resources/key.p12"); PrivateKey privateKey = SecurityUtils.loadPrivateKeyFromKeyStore(keyStore, inputStream, "notasecret", "privatekey", "notasecret"); inputStream.close(); GoogleCredential credential = new GoogleCredential .Builder() .setTransport(httpTransport) .setJsonFactory(jsonFactory) .setServiceAccountId(serviceAccountId) .setServiceAccountScopes(Collections.singleton(AndroidPublisherScopes.ANDROIDPUBLISHER)) .setServiceAccountPrivateKey(privateKey) .build(); AndroidPublisher androidPublisher = new AndroidPublisher .Builder(httpTransport, jsonFactory, credential) .setApplicationName(applicationName) .build(); return androidPublisher.edits(); } private void updateVersionCode() throws Exception { String packageName = "com.package.name"; Edits edits = getApplicationEdits(); String editId = edits .insert(packageName, null) .execute() .getId(); ApksListResponse apksResponse = edits .apks() .list(packageName, editId) .execute(); List<Integer> versions = new ArrayList<Integer>(); List<Apk> apks = apksResponse.getApks(); if (apks == null) { throw new Exception("responsed list of apks is null"); } for (Apk apk : apks) { versions.add(apk.getVersionCode()); } if (versions.isEmpty()) { throw new Exception("previous versions are not detected"); } int lastVersionCode = Collections.max(versions); if (lastVersionCode == 0) { throw new Exception("version is 0"); }
Application publications private void publishApplication() throws Exception { String packageName = "com.package.name"; String track = "beta";
Compile the executable jar. Now you need to configure the creation of the installation file and its publication on your CI server, I use TeamCity. So, we will have 4 build steps:
- Update versioncode. Run the jar file with the parameter that you will process and call the updateVersionCode () method. This is how it looks on TeamCity

- Update versionName
- Generation apk. You can use the command line, you can select the special step, there is a gradle and IntelliJ IDEA, in the second case you need to specify the name artifact
- The publication of the application. Just run the jar file with a different parameter and call the publishApplication () method
Thanks for attention