Recently there was a conference
// BUILD , at which Microsoft traditionally presents new technologies for developers. In the
key report of the first day , one very important idea was expressed - as communication with computers becomes more and more natural, the role of interactive dialogue in natural language increases. It’s not just that we can ask the Cortana voice assistant to ask us to set an alarm, but that many other tasks (order a pizza, book a hotel, buy tickets, etc.) can be decided through dialogue. Moreover, it can be not only a dialogue between the user and the computer: in a more difficult case, a person can ask Cortana to plan a trip, and then Cortana will communicate with other bots by itself, completing the hotel and ticket orders.
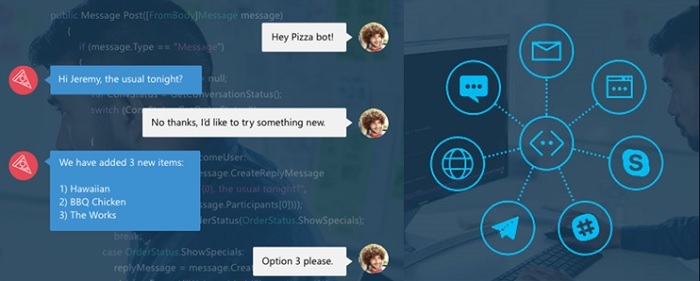
What is interesting here is that the “communication” itself can occur in various places: on the desktop with the help of the Cortana client, but also in other channels traditionally used for chat: in Skype, Telegram, Slack, etc. The personality and memory of our personal assistant does not depend on the communication channel, but is located somewhere in the cloud, ready to chat with us on any of the so-called “conversational canvases”.
')
To create bots, a preliminary version of the
Microsoft Bot Framework and the corresponding APIs were presented, which we will discuss below. We will also show how you can create your simplest bot that supports conversation with the user in English.
The following key elements are used to work with bots:
- Bot Builder SDK (available for C # and for Node.js) is designed to create key bot functionality. It is based on WebAPI, and defines the bot's communication protocol with the outside world. Within the SDK, there is an emulator that allows you to debug bots, as well as a set of classes to simplify the implementation of some key abstractions, such as lengthy dialogs with the state.
- Cognitive Services (formerly known as LUIS, part of Project Oxford ) allows you to simplify the analysis of natural language and the allocation of meaning from text sentences. Using the web interface, we can identify the basic syntax, and automatically assign them the appropriate intentions of the user (intents), to which the bot will then respond.
- Bot Connector allows you to bind our bot to one or more communication channels, such as Skype, Slack, Telegram, etc. To do this, you only need to configure the bot connection on botframework.com .
- Catalog of bots , in which, over time, it will be possible to publish links to various bots.
Example: Meet Murphy Bot
A great example of a bot is Murphy, created within the project
http://www.projectmurphy.net/ . Once logged in, you can install Murphy on Skype and start chatting with him (just make sure you are using the latest version of Skype, which supports bots). This bot can be asked questions like “What if Cindy Crawford were a superman?” (See the example of the dialogue below), or “What if I were a programmer” (in this case, he will ask you to upload your photo).

Hello, Bot! We write to the interlocutor
As an example, let's look at how to create a simple bot based on the Bot Framework. Recall that the heart and brain of a bot is a web service, which is usually located in the cloud.
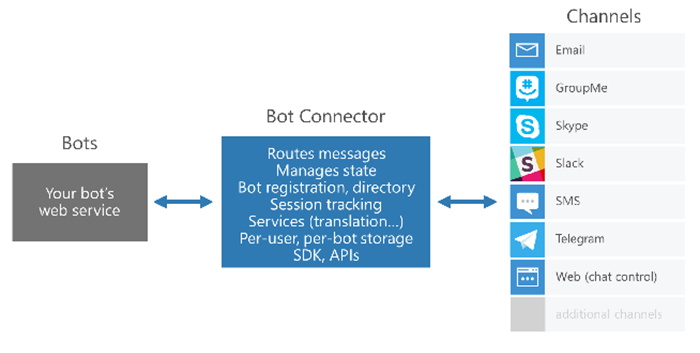
To create such a service, the easiest way is to download the
Bot Framework Template for Visual Studio (make sure you have Visual Studio 2015 Update 1 with web tools). Put the resulting zip file into the directory with the Visual Studio templates, usually it is “% USERPROFILE% \ Documents \ Visual Studio 2015 \ Templates \ ProjectTemplates \ Visual C #". Then in Visual Studio you can create a new project of the
Bot Application type:
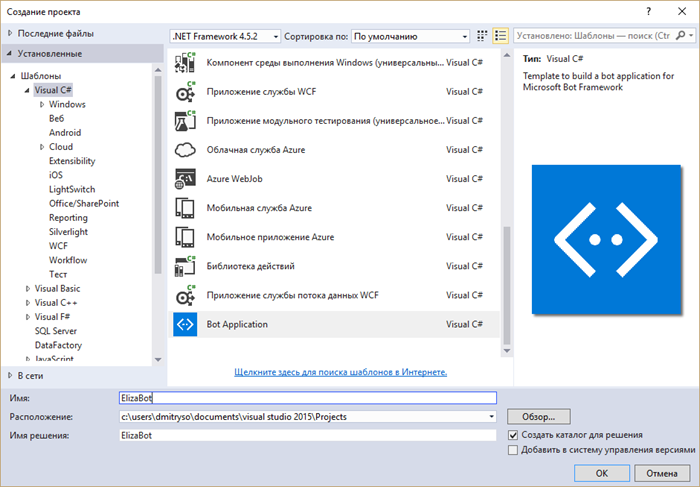
In the created project, the
Post method in the file
Controllers / MessagesController.cs is responsible for the main functionality of the bot. For the simplest Hello-bot, we implement this method as follows:
public async Task<Message> Post([FromBody]Message message) { if (message.Type == "Message") { var reply = message.Text.ToLower() == "hello" ? "Hello!" : "I do not understand you!"; return message.CreateReplyMessage(reply); } else { return HandleSystemMessage(message); } }
To test the bot, run the project on the local web server (F5), copy the address and port from the address bar of the opened browser, and open it in the
Microsoft Bot Framework Emulator (you must first download and install it). Please note that you need to add the path
/ api / messages to the address bar of the site.

To make the bot do something a little more useful, I used the C # port of the famous
Eliza program. You can download the chatbot
in our GitHub repository . In the first version, the bot build scheme is not much different from the above program, only the Eliza library method is called to generate a response.
This simple implementation is not very suitable for supporting long conversations with the user, since the Post method is used to process all user requests, and there is no state separation between them. To do this, you can explicitly look at the session identifier (
message.ConversationID ), use BotUserData / BotConversationData objects, or use more advanced APIs that support dialogs (we will talk about them in future articles). In the meantime, we will not pay attention to these details, considering that the bot is stateless.
To run the bot in the cloud, we first need to publish the resulting Web API in Azure. Then you should go to
http://dev.botframework.com and register a new bot. It is important to come up with a unique AppID for the bot, and get App Secret generated automatically.
After that, you need to take an important step - in the
Web.Config file of our application, you need to add the App ID and App Key:
<configuration> <appSettings> <add key="AppId" value="YourAppId" /> <add key="AppSecret" value="YourAppSecret" /> </appSettings>
After that, you need to re-deploy the bot in the cloud so that Web Config is updated.

Having done this, you can test the performance of the bot in the properties panel on the Bot Framework website, or use the emulator that we already know, although in this case you will need to enter the correct AppID and App Secret:
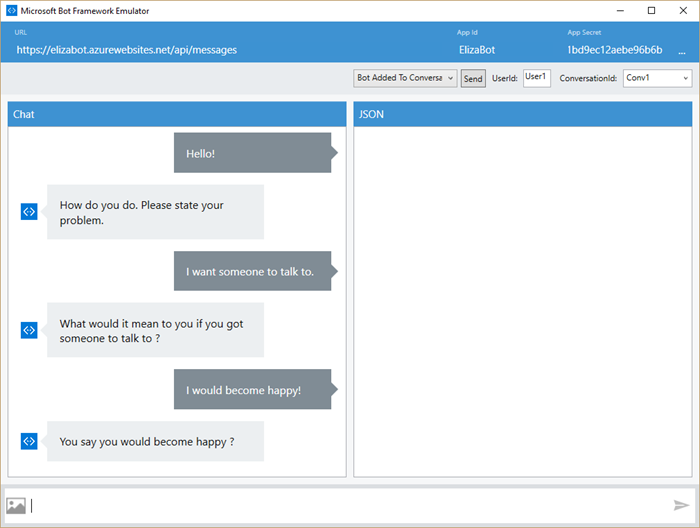
Now that the bot is working in the emulator, you can bind it to various communication channels. To do this, you need to add and configure these channels in the bot control panel on the Bot Framework website. To do this, select the preferred communication channel, and follow the instructions:
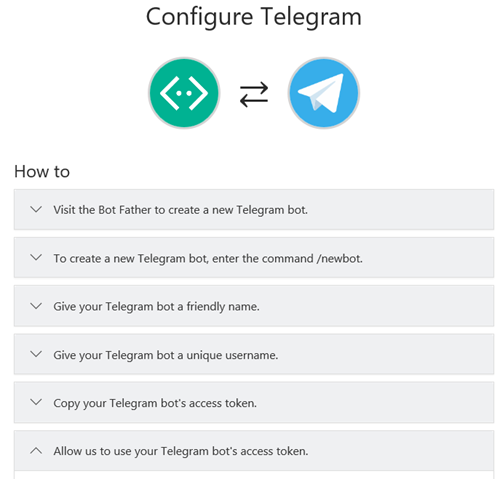
For example, in the case of Telegram, you need to create a new bot in Telegram using a conversation with a special bot Bot Father, then get Access Tokem, and provide it to the Bot Framework website. After this, Bot Connector will take over all intermediary operations between the Telegram API and your bot, and you will be able to communicate with your Telegram bot. By the way, my bot is called in
@ElllizaBot, you can talk to him!
You can find out more about creating a bot from scratch with the help of the Bot Framework
in this English-speaking report in BUILD .
Channel abstraction
The main beauty of the Bot Framework API is that we can now write bots, not focusing on any one channel of communication. In fact, the Bot Framework gives us the right level of abstraction for implementing conversation mechanisms and dialogues, and the Bot Connector takes on a concrete connection with various communication tools. This division allows the programmer to write a bot once, and to communicate with him from everywhere. We can formulate this principle as follows, paraphrasing the slogan known from the world of Java:
Write Once - Chat Everywhere .
A few words about Skype Bot API
Another news announced on // BUILD is the availability of a separate Skype API for creating bots. In fact, it is possible to create Skype bots through the Bot Framework, but the Bot Framework’s capabilities so far allow us to work only with text (plus some types of attachemts), while the Skype Bot API is somewhat broader and allows us to create video bots. When choosing a technology, you should give preference to the Bot Framework, because of its versatility, but if you want to create a truly amazing bot specifically for Skype, take a look at the
Skype Bot SDK .
Conclusion
Dialogue is a natural form of human interaction, and it may be that it will become a natural form of human-computer interaction. Microsoft offers a convenient software abstraction in the form of the Bot Framework, which allows you to start simply by creating your chat bots that work with many channels of communication at once. This is not only promising, but also very entertaining! Let's experiment with the creation of bots for now, and in a few years (or months) we will see if bots are a substitute for websites or mobile applications, as
analysts say .
Here are our other articles on similar topics :