
A lot of articles have been written about the JavaScript device. First of all, it is
"JavaScript. Kernel." Dmitry Soshnikov ,
translation of an article by Richard Cornford and a
post by Dmitry Frank . But in order to get a good understanding of any technology, it is better to turn to primary sources. In this case, the
ECMA-262 ECMAScript Language Specification . I see this post as a lightweight way to start learning about the standard. I recommend to follow the links, read the text of the specification and make your own schemes.
How short circuits work in JavaScript
The basic structure of ECMAScript is the
execution context ,
lexical environment and
environment record . They are related as follows:
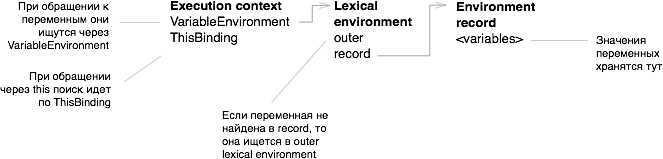
')
In addition to the VariableEnvironment in the execution context, there is also a LexicalEnvironment , more details about the differences can be found in the ECMAScript 5 spec: LexicalEnvironment versus VariableEnvironment .
ThisBinding will be discussed in the next part of the article.
In
environment record stored values of variables. If you declare
var a = 1
, then {a: 1} will appear in the current
record . In the
lexical environment, in addition to
record, there is also an
outer field.
Outer refers to the external
lexical environment , for example, in the case of nested functions.
Searching for variables begins with the current context's
VariableEnvironment . If a variable with the same name is not found in the
record ,
then it is searched in the outer environment by the chain .
When the program is started
, global context and environment are created . The
global object is used as a
record .

When the interpreter encounters the
function keyword, it
creates a FunctionObject . The
scope property of the
functionObject created is written to reference the current
lexical environment .
Writing
function f(){}
practically equivalent to writing
var f = function(){}
except that in the first case the
FunctionObject will be created when entering the block containing
function f()
, and in the second when performing a specific line.

Each time the function is called
, new context , environment and record are created . The context is pushed onto the stack; when the function exits, it is destroyed. The
scope of the called
FunctionObject is written to the
outer of the created
environment . If the function was declared in a global context, then
outer will point to the
global environment .

Now consider the closure when one function returns another.
var x = 1; function f() { var x = 2; function g() { return x; } return g; } f()();
When you call the function
f ,
context and
environment for
f are created , as well as
FunctionObject for
function g
. In
scope , a reference is written to the current
environment from a
VariableEnvironment . When you exit
f, the context is destroyed, but the
environment will remain, since it is referenced from the returned
FunctionObject .
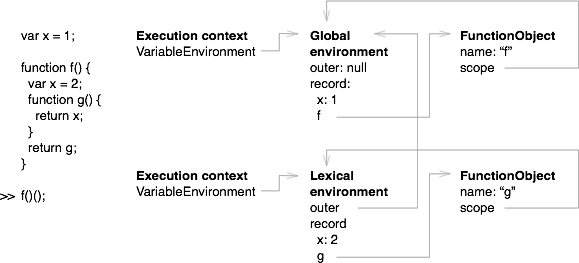
When you call the function
g returned from
f , a context is created and the
environment for
g . The
outer environment is written to the
scope from the called
FunctionObject . The search for the variable
x begins with the current
VariableEnvironment and then continues on
outer . As a result, the value
x = 2
will be returned.
In the next part of the article we will analyze how from the point of view of ECMAScript
this works.