Translator: This article is the third in the translation cycle of the official SFML library manual. Past article can be found here. This series of articles aims to provide people who do not know the original language the opportunity to get acquainted with this library. SFML is a simple and cross-platform multimedia library. SFML provides a simple interface for developing games and other multimedia applications. The original article can be found here . Let's start.Introduction
This article is the first one you should read if you use SFML on Linux. It will tell you how to set up your project.
SFML installation
There are different approaches to installing SFML on Linux:
- Installing from your distribution repository
- Downloading a precompiled SDK and copying files manually
- Getting the source code, build and install
The first option is preferable. If the version of SFML you want to install is available in the official repository, then install it using the batch manager of your operating system. For example, in Debian you should do the following:
sudo apt-get install libsfml-dev
The third option requires more work: you need to satisfy all SFML dependencies. Make sure CMake is installed and run several commands. The result will be a package adapted for your system. If you want to go this route, read the
SFML self-help article .
Finally, the second option is an excellent choice for quick installation if SFML is not available as an official package. Download the SDK from the
download page . Unzip it and copy the files to the right place: either to a separate path in your personal directory (for example,
/ home / me / sfml ), or to a standard directory (for example,
/ usr / local ).
Compiling a program using SFML
In this article we will not talk about integrated development environments such as Code :: Blocks or Eclipse. We will review the commands needed to compile and link to an executable file using SFML. Writing a Makefile or setting up a project in the IDE is beyond the scope of this article. According to the topics there are enough materials on the Internet.
If you are using Code :: Blocks, you can use
the Code :: Blocks article for Windows ; most of the guidelines should be suitable for Linux. You may not tell the compiler and linker where to look for header files and libraries if you installed SFML in the standard way.
Create a source file. In this article we will call it "main.cpp". Place the following code in the main.cpp file:
#include <SFML/Graphics.hpp> int main() { sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!"); sf::CircleShape shape(100.f); shape.setFillColor(sf::Color::Green); while (window.isOpen()) { sf::Event event; while (window.pollEvent(event)) { if (event.type == sf::Event::Closed) window.close(); } window.clear(); window.draw(shape); window.display(); } return 0; }
Now let's compile it:
g++ -c main.cpp
In case SFML is installed along a non-standard path, you need to tell the compiler where to find the SFML header files (files with the .hpp extension):
g++ -c main.cpp -I<---SFML>/include
Here
<SFML path> is the directory where you copied SFML. For example
/ home / me / sfml .
Then you need to associate the compiled file with the SFML library in order to get the final executable file. SFML consists of five modules: system, window, graphics, audio and network and libraries for each of them. To design your application with the SFML library, you must add the "-lsfml-xxx" option to the build command. For example, "-lsfml-graphics" for the graphics module (the "lib" prefix and the ".so" extension of the library should be omitted).
g++ main.o -o sfml-app -lsfml-graphics -lsfml-window -lsfml-system
If you installed SFML by a non-standard path, you need to tell the linker where to find the SFML libraries (files with the .so extension).
g++ main.o -o sfml-app -L<---SFML>/lib -lsfml-graphics -lsfml-window -lsfml-system
Now we can run the compiled program:
./sfml-app
If SFML is installed along a non-standard path, you need to tell the dynamic linker where to find the SFML libraries by defining the environment variable LD_LIBRARY_PATH:
export LD_LIBRARY_PATH=<sfml-install-path>/lib && ./sfml-app
Run the program, and if everything was done correctly, you should see this:
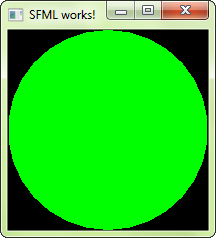
Next article:
SFML and Xcode (Mac OS X)