From the translator: This article is the second in the translation cycle of the official SFML library manual. Past article can be found here. This series of articles aims to provide people who do not know the original language the opportunity to get acquainted with this library. SFML is a simple and cross-platform multimedia library. SFML provides a simple interface for developing games and other multimedia applications. The original article can be found here . Let's start.Introduction
This article is the first one you should read if you use the Code :: Blocks development environment with the GCC compiler (this is the standard compiler for this development environment). It will tell you how to set up your project.
SFML installation
First you need to download the SFML SDK from the
download page .
There are several versions of GCC for Windows that are incompatible with each other (differences in exception handling, in the threading model, etc.). Make sure you select the package that matches the version of GCC that you are using. If you are not sure, check which of the files (libgcc_s_sjlj-1.dll or libgcc_s_dw2-1.dll) are present in your MinGW / bin directory. If MinGW is installed with Code :: Blocks, you probably have the SJLJ version. If your version of GCC cannot work with a pre-compiled version of the SFML library, you will have to
build SFML yourself . It is not difficult.
Next you have to unpack the archive with SFML in any directory convenient for you. Copying header files and libraries into your MinGW installation is not recommended. It is better to keep the libraries in a separate place, especially if you intend to use several versions of one library or several compilers.
Creating and configuring an SFML project
The first thing you need to do is choose the type of project you are creating. Code :: Blocks offers a wide range of project types, including the SFML project. Do not use it! This type of project has not been updated for quite a long time and is most likely incompatible with the latest versions of SFML. Instead, create an empty project. If you want to get rid of the console, in the project properties go to “Build targets” and in the combo box select “GUI application” instead of “Console application”.
Now you need to tell the compiler where to look for header files (files with the .hpp extension) and the linker, where to look for SFML libraries (files with the .lib extension).
Add the following to the “Build options” tab in the “Search directories” tab:
- Path to SFML headers ( <install-SFML-path> / include ) in Compiler search directories
- Path to the SFML libraries ( <installation path-SFML> / lib ) in Linker search directories
These paths are the same for Debug and Release configurations, so you can set them globally for your project.

The next step is to build your application with the SFML libraries (files with the extension .a). SFML consists of five modules (system, window, graphics, network and audio) and libraries for each of them. Libraries should be added to “Link libraries” to the list of options for building your project, under the “Linker settings” tab. Add all the SFML libraries that you need, for example “sfml-graphics”, “sfml-window” and “sfml-system” (the “lib” prefix and the extension “.a” should be omitted).

It is important to specify the libraries corresponding to the configuration: “sfml-xxx-d” for Debug and “sfml-xxx” for Release, otherwise errors may occur.
When compiling SFML libraries, make sure that you arrange the libraries in the correct order; this is very important for GCC. The rule is that libraries that depend on other libraries should be placed first on the list. Any SFML library depends on sfml-graphics, sfml-window and sfml-system. This is shown in the screenshot above.
The settings above allow you to build your project with a dynamic version of SFML, which requires DLL files. If you want to directly integrate SFML into your executable file, rather than using the build with a dynamic library, you must build a static version of the library. SFML static libraries have the suffix "-s": "sfml-xxx-sd" for Debug configuration and "sfml-xxx-s" for Release.
You also need to define the SFML_STATIC macro in the preprocessor options of your project.
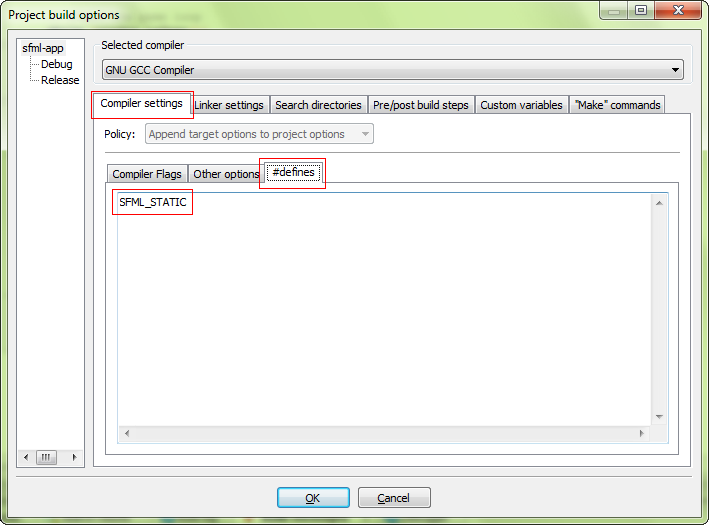
Starting with SFML 2.2 with a static layout, you also need to build all the SFML dependencies. This means that if, for example, you build sfml-window-s or sfml-window-sd, you also have to build opengl32, winmm and gdi32. Some of these libraries may already be listed in the “Inherited values” section, but adding them should not cause any problems.
The table below shows the dependencies for each module, add -d if you want to build the SFML Debug library:
Module | Dependencies |
---|
sfml-graphics-s | - sfml-window-s
- sfml-system-s
- opengl32
- freetype
- jpeg
|
sfml-window-s | - sfml-system-s
- opengl32
- winmm
- gdi32
|
sfml-audio-s | - sfml-system-s
- openal32
- flac
- vorbisenc
- vorbisfile
- vorbis
- ogg
|
sfml-network-s | |
sfml-system-s | |
From the table, you may have noticed that some SFML modules may depend on others, for example, sfml-graphics-s depends on sfml-window-s and sfml-system-s. If you produce the static layout of the SFML library, be sure that all dependencies from the dependency chain have been satisfied. If one of the dependencies is missing, you will get a linker error.
If you're a little confused, don't worry, it’s completely normal for a beginner to be overloaded with all this static binding information. If you don’t get it right the first time, you can try again with all the above in mind. If you still have problems with static binding, you can try searching for a solution in the
FAQ section or on the
forum .
If you do not know the difference between dynamic (also called common) and static libraries and what type of libraries to use, you can find more information on the Internet. There are many good articles / blogs / posts on this topic.
Your project is ready, let's write some code to check that everything works correctly. Place the following code in the main.cpp file:
#include <SFML/Graphics.hpp> int main() { sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!"); sf::CircleShape shape(100.f); shape.setFillColor(sf::Color::Green); while (window.isOpen()) { sf::Event event; while (window.pollEvent(event)) { if (event.type == sf::Event::Closed) window.close(); } window.clear(); window.draw(shape); window.display(); } return 0; }
Now compile the project, and if you linked your program with a dynamic version of SFML, do not forget to copy the files with the extension .DLL (they are located in <SFML installation path / / bin) to the directory where the executable file of your program is located . Run the program, and if everything was done correctly, you should see this:
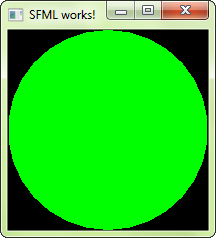
If you are using the sfml-audio module (regardless of its static or dynamic version), you should also copy the external dll OpenAL32.dll. This file can also be found in the
<install-SFML-path> / bin directory .
Next article:
SFML and Linux.