
Cross-platform mobile application development was very popular at the time. This approach was used by most companies during the development of the mobile industry. The main reasons to use cross-platform development were simple - the lack of professional staff on the market, the speed and cost of development. Unfortunately, in most cases, this approach did not justify itself.
But why not give it a second chance? Technology has stepped forward and theoretically we can get a very high quality product. In this article, we will consider in practice how to develop a library for IOS / Android in the Golang language and see what limitations and problems we encountered during the development process.
Our main task is to develop an SDK for building logs and crashes from mobile apps, while the SDK should connect and work with both Android and iOS apps. At the same time, the library should interact with the main
LogPacker service, which aggregates and analyzes data.
')
We decided to use the new features of the Go language to create a cross-platform library. First, our main application is written in Go, and it was easier for us to use this language and not involve Java / Objective-C developers. Secondly, we saved development time and tried the old approach with new features.
What is gomobile?
The
gomobile project provides developers with tools for building code for Android and iOS mobile platforms.
There are now two ways to integrate Go into the mobile environment:
- Writing a full-fledged Go application without UI.
- Generate Java / Objective-C / Swift code from Go.
This feature is supported starting with Golang version 1.5. New
gomobile utility helps to compile Go into mobile applications or compile Java / Objective-C / Swift code.
To begin, choose one of the methods of implementation. The first method does not suit us because of the formulation of the problem - we need a small library, not a separate application. Although the method is very interesting and promising due to the speed of go applications and minimizing resource consumption of mobile devices.
We will select the second method, and generate the Java / Objective-C / Swift code from Go.
Setting up the environment
To begin with we will prepare an environment for development. We need
Go version 1.5 or higher (the higher the better, the Go community makes continuous improvements to Go Mobile).
Next, install the gomobile utility and the Android SDK library. Let's start by installing gomobile:
go get golang.org/x/mobile/cmd/gomobile
Note: On OS X, you must have the Xcode Command Line Tools installed.Next, we initialize gomobile, this can be done once in any working directory:
gomobile init
Note: this command may take a few minutes.To build Java code, we need the Android SDK and installed Java (OpenJDK is enough).
Download and unpack the Android SDK in your home directory, for example ~ / android-sdk, and execute the following command to install the version API:
~/android-sdk/tools/android sdk
Next, set the environment variable:
export ANDROID_HOME=$HOME"/android-sdk"
The environment for developing and building the library is ready. Let's go directly to writing code, and see what limitations we have encountered.
Shared Go code for Android and iOS
The same code can be used for further compilation under Android and iOS. Writing such a cross-platform Go code has its limitations. Currently, we can only use a certain set of data types. When developing an application on Go, this must be taken into account Consider in more detail the supported types:
- int and float;
- string and boolean;
- byte []. The current implementation does not allow using [] byte as a function argument ( https://golang.org/issues/12113 );
- the function must return only supported types, may not return a result, return one type or two types, despite the fact that the second type must be an error;
- interfaces can be used if they use a supported type during export;
- type struct only when all fields comply with the constraints.
Therefore, if the type is not supported by the gomobile bind command, you will see a similar error:
panic: unsupported basic seqType: uint64
Undoubtedly, the set of supported types is very limited, but for the implementation of our SDK this is quite enough.
Build and import in Java / Objective-C / Swift
Gobind generates Java, Objective-C, or Swift code equivalent to Go code. Unfortunately, gomobile does not work for Windows Phone and this should be taken into account at the planning stage.
Usually gobind is not used directly; instead, the code is generated automatically and wrapped in a package with the command `gomobile bind`. This is described in more detail here
golang.org/x/mobile/cmd/gomobile .
Consider some of the commands and features of the compilation process for each platform.
Let's start with the -target flag, which defines a platform for generation. Example for Android:
gomobile bind --target=android .
This command will generate a file from the current
.aar code. Importing this file into Android Studio is very simple:
- File> New> New Module> Import .JAR or .AAR package
- File> Project Structure> app -> Dependencies -> Add Module Dependency
- Add import: import go.logpackermobilesdk.Logpackermobilesdk
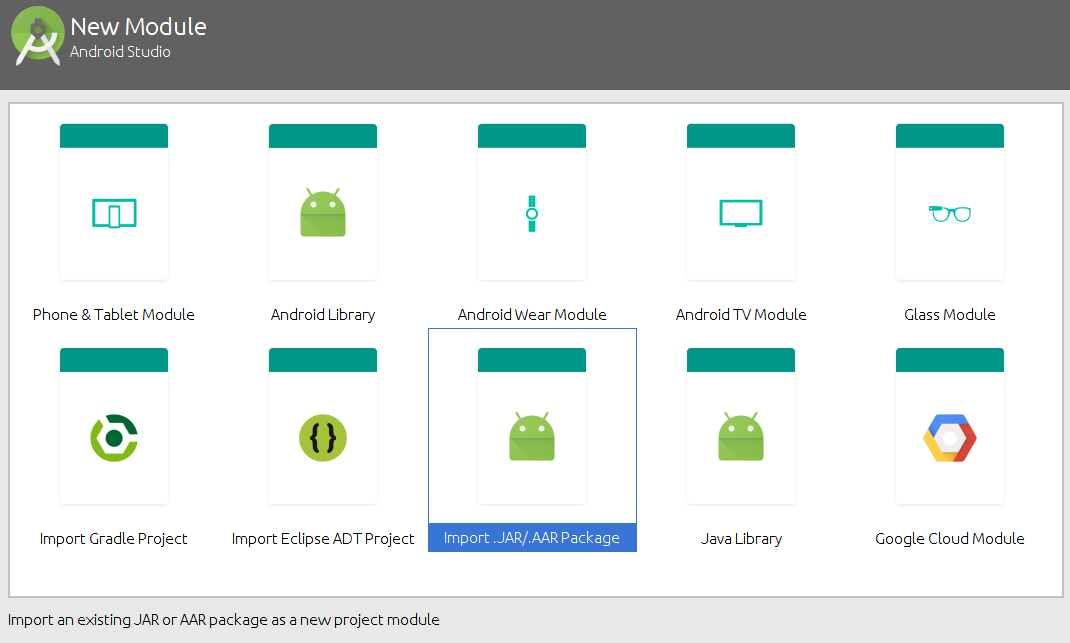
Note: In Java, the name of the import package always starts with go.A similar command is used to build Objective-C / Swift code:
gomobile bind --target=ios .
A
.framework folder will be created in the current directory.
This works for both Objective-C and Swift. Move the .framework folder to the Xcode file browser and add import to the project:
#import "Logpackermobilesdk/Logpackermobilesdk.h"
Note: Go allows you to create not only the SDK, but also compile the application itself into apk / ipa files from the main.go file, but without the support of the native mobile UI. Undoubtedly, this is a very interesting experiment of the Go-community.Using connected packages
Gomobile bind automatically creates functions getSomething (), setSomething (). Also all exported functions will be publicly available.
For example, using our library in Android Studio:
import go.logpackermobilesdk.Logpackermobilesdk;
It is for Objective-C:
#import "ViewController.h" #import "Logpackermobilesdk/Logpackermobilesdk.h" @interface ViewController () @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; GoLogpackermobilesdkClient *client; NSError *error; GoLogpackermobilesdkNewClient(@"https://logpacker.mywebsite.com", @"dev", [[UIDevice currentDevice] systemVersion], &client, &error); GoLogpackermobilesdkMessage *msg; msg = client.newMessage; msg.message = @"Crash is here!"; // Use another optional setters for msg object GoLogpackermobilesdkResult *result; [client send:(msg) ret0_:(&result) error:(&error)]; }
As you can see from the examples above, we ended up with standard libraries. The configuration of the libraries in the application is extremely simple and familiar to developers.
Conclusion
Everyone understands that the creation of separate teams to develop for each mobile platform is not the easiest and cheapest pleasure. But to create a quality product it is necessary at this time. On the other hand, we were able to perform our small task in the framework of cross-platform development and took advantage of all its advantages:
- Minimal development resources.
- Development speed
- Simple solution support in the future.
The downside is that we could not build the library under Windows Phone, but we knew this in advance.
We hope that soon there will be a simple way to write full applications and SDK in Golang language.
You can familiarize yourself with our practices by tiling
our repository .