Your attention is invited to the translation of the tutorial "5 min quickstart" from the team Angular. The tutorial describes the process of creating a “Hello World” application on the new Angular 2 framework, which recently received beta status.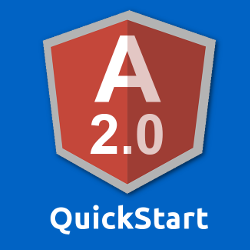
Let's start from scratch and build a super-simple Angular2 application on TypeScript.
Demo
Running a
working example is the best way to see how the application on Angular 2 comes to life.
Clicking this link opens a new tab, loads the example into
plunker and displays a simple message:
My First Angular 2 App
Here is the file structure:
angular2-quickstart ├── app │ ├── app.component.ts │ └── boot.ts ├── index.html └── license.md
Functionally, these are
index.html and two TypeScript files in the
app / folder. Let's do that!
')
Of course, we will not create applications that will run only in plunker. Let's do it as if we did it in real life:
- We will equip our development environment.
- Let's write the root component Angular for our application.
- Download it so that it takes control of the main web page.
- Let's write the main page ( index.html ).
We can really put together QuickStart in five minutes if we follow the instructions and ignore all comments.
But many of us will be interested in “why” and “how” all this happens, and the answer to these questions will take much more time.
Development environment
We need a place (project folder), several libraries, a number of TypeScript settings and an editor with TypeScript support of your choice.
Create a new folder of our project.
mkdir angular2-quickstart cd angular2-quickstart
Add the libraries we need
We recommend using the npm package manager to download and manage libraries.
Don't you have npm? Install it right now because we will use it several times throughout this tutorial.
Add the
package.json file to the project folder and copy the following code into it:
Can't wait to find out the details? We outlined them in application below .
Install packages. Open a terminal window (command prompt on Windows) and run this npm command:
npm install
Creepy red error messages may appear during installation. Ignore them. The installation will be successful. Watch Appendix below if you want to know more.
Configuring TypeScript
We need to configure the TypeScript compiler in a rather specific way.
Add the
tsconfig.json file to the project folder and copy the following into it:
We are researching tsconfig.json in application below .
So, everything is ready. Let's write some code.
Our first component is Angular
Component is the most fundamental concept of Angular. The component adjusts the display (view) - part of the web page, where we show information to the user and respond to his actions.
Technically, a component is a class that controls a display pattern. We will write many such classes when creating Angular applications. This is our first attempt, so we will make everything extremely simple.
Create a subfolder in which the sources will be located
We prefer to store our application code in a subfolder called
app / . Run the following command in the console:
mkdir app cd app
Add a component file
Now add a file called
app.component.ts and copy the following code into it:
Let's take a closer look at this file, starting from the very end, where we define the class.
Component Class
At the very bottom of the file is an empty and
unoccupied class called
AppComponent . When we are ready to create an independent application, we will be able to supplement this class with the properties and logic of the application. Our
AppComponent class
is empty because we don't need it to do something in this QuickStart.
Modules
Applications on Angular are modular. They include many files, each of which has a specific purpose.
Most application files
export something like a component. Our
app.component file exports the
AppComponent class.
The fact of export turns an ordinary file into a module. The file name (without its extension) is usually the name of the module. Thus,
'app.component' is the name of our first module.
More complex applications may have child components that inherit the
AppComponent in the visual tree. More complex applications will have more files and modules, at least as many as they have components.
QuickStart is not complicated: one component is all we need. However, modules play a fundamental organizational role even in such a small application.
Modules rely on other modules. In Angular applications written in TypeScript, when we need something provided by another module, we import it. When another module needs to refer to
AppComponent , it imports
the AppComponent symbol as follows:
Angular is also a collection of library modules. Each library is a module composed of several modules linked by a common direction.
If we need something from Angular, we import it from its library module. And right now we need something to be able to define the metadata for our component.
Component metadata
A class becomes an Angular component when we attach metadata to it. Angular needs metadata to understand how to construct a mapping, and how the component interacts with other parts of the application.
We define component metadata using the Angular
Component function. We access this function by importing it from the Angular core library,
angular2 / core .
In TypeScirpt, we can associate a function and a class by turning the function into a
decorator . To do this, before its name you need to add the @ symbol, and place it right before the class declaration.
@Component tells Angular that this class
is a component of Angular . The configuration object sent to
@Component has two fields:
selector and
template .
The
selector property defines the usual CSS selector for the HTML element
my-app , which acts as a host. Angular creates and displays an instance of our
AppComponent each time it encounters a
my-app in parent HTML.
Remember the selector my-app ! We will need this information when we write our index.html .
The
template property contains the component template. A template is a type of HTML that explains Angular how to render a display. Our template is the only line of HTML that declares:
“My First Angular App” .
Now we need to somehow explain Angular that this component needs to be downloaded.
Run it
Add a new file,
boot.ts , to the
app / folder, and copy the following code into it:
We need two things to run the application:
- Angular bootstrap browser function.
- The root component of the application that we just wrote.
We import both. Then we call
bootstrap and pass the
type of the root component AppComponent into it.
You can learn why we import bootstrap from angular2 / platform / browser and why we create a separate boot.ts file in application below .
We asked Angular to launch the application in the browser with our component as the root. However, where does Angular launch it?
Add the index.html page
Angular displays our application in a specific place on our
index.html page. It's time to create this file.
We will not put
index.html in the
app / folder. We will place it
one level higher in the project root folder .
cd ..
Now create the
index.html file and copy the following into it:
<html> <head> <title>Angular 2 QuickStart</title> <script src="node_modules/angular2/bundles/angular2-polyfills.js"></script> <script src="node_modules/systemjs/dist/system.src.js"></script> <script src="node_modules/rxjs/bundles/Rx.js"></script> <script src="node_modules/angular2/bundles/angular2.dev.js"></script> <script> System.config({ packages: { app: { format: 'register', defaultExtension: 'js' } } }); System.import('app/boot') .then(null, console.error.bind(console)); </script> </head> <body> <my-app>Loading...</my-app> </body> </html>
There are 3 sections of HTML that need to be noted:
- We load the JavaScript libraries we need. angular2-polyfills.js and Rx.js are required for Angular 2 to work.
- We set up something called System , and we ask it to import the boot file that we just wrote.
- We add the <my-app> tag to the <body> . This is the place where our application lives!
Something must find and load the modules of our application. We use
SystemJS for this. There are many options, and not to say that SystemJS is the best choice, but we love it and it works.
The specifics of setting up SystemJS is outside of this tutorial. We will briefly describe part of the configuration in
application below .
When Angular calls the
bootstrap function in
boot.js , it reads the
AppComponent metadata, finds the selector
my-app , finds the tag called
my-app, and loads our application into this tag.
Compile and run!
Open a terminal window and enter the following command.
npm start
This command starts two parallel node processes.
- TypeScript compiler in watch mode.
- Static lite-server server that loads index.html in the browser and updates the browser every time the application code changes.
Within a few moments, the browser tab should open and display:
My First Angular 2 App
Congratulations! You are in business!
If you see Loading ... instead, read application "Support for ES6 browsers . "
Let's make some changes
Try changing the message to the “My SECOND Angular 2 app”.
The TypeScript compiler and
lite-server watch your actions. They should detect the change, recompile TypeScript into JavaScript, update the browser tab and display the updated message.
This is an elegant way to develop applications!
We close the terminal window when we’ve done everything to interrupt the compiler and the server at the same time.
Final structure
The final structure of our project folder should look like this:
angular2-quickstart ├── node_modules ├── app │ ├── app.component.ts │ └── boot.ts ├── index.html ├── package.json └── tsconfig.json
And here are our files:
app / app.component.ts import {Component} from 'angular2/core'; @Component({ selector: 'my-app', template: '<h1>My First Angular 2 App</h1>' }) export class AppComponent { }
app / boot.ts import {bootstrap} from 'angular2/platform/browser' import {AppComponent} from './app.component' bootstrap(AppComponent);
index.html <html> <head> <title>Angular 2 QuickStart</title> <script src="node_modules/angular2/bundles/angular2-polyfills.js"></script> <script src="node_modules/systemjs/dist/system.src.js"></script> <script src="node_modules/rxjs/bundles/Rx.js"></script> <script src="node_modules/angular2/bundles/angular2.dev.js"></script> <script> System.config({ packages: { app: { format: 'register', defaultExtension: 'js' } } }); System.import('app/boot') .then(null, console.error.bind(console)); </script> </head> <body> <my-app>Loading...</my-app> </body> </html>
package.json { "name": "angular2-quickstart", "version": "1.0.0", "scripts": { "tsc": "tsc", "tsc:w": "tsc -w", "lite": "lite-server", "start": "concurrent \"npm run tsc:w\" \"npm run lite\" " }, "license": "ISC", "dependencies": { "angular2": "2.0.0-beta.0", "systemjs": "0.19.6", "es6-promise": "^3.0.2", "es6-shim": "^0.33.3", "reflect-metadata": "0.1.2", "rxjs": "5.0.0-beta.0", "zone.js": "0.5.10" }, "devDependencies": { "concurrently": "^1.0.0", "lite-server": "^1.3.1", "typescript": "^1.7.3" } }
tsconfig.json { "compileroptions": { "target": "es5", "module": "system", "moduleresolution": "node", "sourcemap": true, "emitdecoratormetadata": true, "experimentaldecorators": true, "removecomments": false, "noimplicitany": false }, "exclude": [ "node_modules" ] }
Conclusion
Our first application does not do too much. This is, in fact, “Hello World” on Angular 2.
For the first time, we did everything as simple as possible: we wrote a small component, Angular, added some JavaScript libraries to
index.html, and started a static file server. In general, this is all that we expected from the “Hello World” application.
We have more serious ambitions
The good news is that all the fuss with the installation does not concern us. Perhaps we touch
package.json a little bit to update the libraries. We will open
index.html only if we need to add a library or css-stylefile.
We are ready for the next step, and now our task is to design an application that demonstrates how great things can be done with Angular 2.
Join us in the
tutorial "Heroic tour" !
Applications
The remainder of this chapter is devoted to a set of applications that spell out some points in more detail, which we briefly touched on above.
There is no critical material here. Read on if you are interested in details.
Application: Support for ES6 browsers
Angular 2 relies on some features of the ES2015 standard, most of which are already included in modern browsers. However, some browsers (such as IE11) require polish (shim) to support this functionality. Try loading the following polyfills
in front of other scripts in
index.html :
<script src="node_modules/es6-shim/es6-shim.js"></script>
↑ Application: package.json
npm is a popular package manager for nodes, and many Angular developers use it to download and manage the libraries their applications need.
We defined the packages we need in the npm
package.json file.
The Angular command suggests using the packages specified in the
dependencies and
devDependencies sections in this file:
You can choose other packages. We recommend this particular set because we know that all its components work well together. Play for us now, and later experiment at your pleasure, choosing options that will fit your experience and taste.
Package.json may contain an optional
scripts section in which we can define useful commands for developing and building. We include several such scripts in the
package.json we offer:
We have already seen how you can run the compiler and the server at the same time using this command:
npm start
We run npm scripts in the following format:
npm run +
script name . Here is a description of what the scripts do:
- npm run tsc - run a TypeScript compiler on one pass
- npm run tsc: w - starts the TypeScript compiler in the observing mode; the process continues to work, recompiling the project at the moment when it notes the changes made to the TypeScript files.
- npm run lite - launches lite-server , a lightweight static file server written and maintained by John Papa with great support for Angular applications that use routing.
Appendix: Warnings and npm errors
All is well when any console messages starting with
npm ERR! missing
at the end of npm install There may be several
npm WARN messages during the operation of the command — and this is an excellent result.
We often see the
npm WARN message after the
gyp ERR series
! Ignore him. A package may attempt to recompile itself using
node-gyp . If this attempt fails, the package is restored (usually to the previous version), and everything works.
All is well as long as there is not a single message
npm ERR! at the very end of
npm install .
Application: TypeScript Configuration
We added a configuration file (
tsconfig.json ) to our project to explain to the compiler how to generate javascript files. You can learn more about
tsconfig.json from the official
TypeScript wiki .
The options and flags that we added to the file are the most important.
I would like to discuss a bit about the
noImplicitAny flag. TypeScript developers disagree on whether it should be set to
true or
false . There is no exact answer here, and we can always change the flag later. But our choice can seriously affect large projects, so it is worthy of discussion.
When
noImplicitAny is set to
false , the compiler, if it cannot infer the type of the variable, depending on how the variable is used, hiddenly sets the type of the variable to
any . This means "hidden (implicit)
any ."
When
noImplicitAny is set to
true , and the TypeScript compiler cannot infer a type, it still generates a javascript file, but also reports an error.
In this QuickStart and in many other examples of this Developer Guide, we set
noImplicitAny to
false .
Developers who prefer stricter typing should set
noImplicitAny to
true . We can still set the type of the variable to the any position if this seems like the best choice, but we have to do it explicitly after thinking about the need for this step.
If we set
noImplicitAny to
true , we can also get hidden indexing errors. If it seems to us that this is more annoying than helpful, we can turn them off using the following flag.
"suppressImplicitAnyIndexErrors":true
↑ Application: SystemJS Configuration
QuickStart uses
SystemJS to load applications and library modules. However, do not forget that he has working alternatives, such as the one highly valued by the
webpack community. SystemJS is a good choice, but we want to give a clear understanding that this is only a choice, not a preference.
All module loaders require configuration, and any configuration of the bootloader becomes more complicated the more diverse the file structure becomes, to the point that we begin to think about combining files to improve performance.
We recommend that you understand the boot loader you choose.
Learn more about SystemJS configuration here .
Given these warnings, what can we do?
<script> System.config({ packages: { app: { format: 'register', defaultExtension: 'js' } } }); System.import('app/boot') .then(null, console.error.bind(console)); </script>
The
packages node tells SystemJS what to do if it sees a request for a module from the
app / folder.
Our QuickStart creates such requests every time a similar import statement is found in any TypeScript file of an application:
Please note that the module name (after
from ) does not contain a file extension. The
packages configuration sets the SystemJS default extension as 'js', that is, a JavaScript file.
This is reasonable because we compile TypeScript into JavaScript
before running the application.
In the plunker demo, we compile into JavaScript right in the browser on the fly. This is not bad for a demo, but it cannot be our choice for development or release.
We recommend compiling with JavaScript during the build phase before running the application, for several reasons:
- We can see errors and warnings of compile time that are hidden from us in the browser.
- Precompilation simplifies the process of loading modules, and it is much easier to find a problem when compilation is a separate external process.
- Precompilation gives great performance because the browser does not need to waste time compiling.
- Our development moves faster, because we just recompile the changed files. The difference will become noticeable when our application contains many files.
- Precompilation is suitable for a continuous development process - building, testing, deployment.
Calling System.import causes SystemJS to import the boot file ( boot.js ... after compiling boot.ts , remember?) Boot is the file where we ask Angular to run the application. We also catch and log launch errors in the console.All other modules are loaded using a request that is created by the import statement or by Angular itself.↑ : boot.ts
We import the bootstrap function from angular2 / platform / browser , not from angular2 / core . There is a reason for this.We can call the “core” only those features that are the same for all target platforms. Indeed, many Angular applications can only be launched in the browser, and we will call the bootstrap function from this library for the most time. Completely “core” -fich - if we write exclusively for the browser.But it’s quite possible to load a component in a different environment. We can download it on a mobile device using Apache Cordova . We may want to render the home page of our application on the server to increase performance or promoteSEO promotion .These platforms require other variants of the bootstrap function, which we will load from another library.Why create a separate boot.ts file?
The boot.ts file is small. This is just a quickstart. We can easily put these few lines in the app.component file and save ourselves from unnecessary complexity.But we do not do this for reasons that we believe are weighty:- Getting it right is easy.
- Convenience testing.
- Convenience reuse.
- Segregation of duties.
- Exploring import and export.
It's simple
Yes, this is an extra step and an additional file. How difficult is it in general?We’ll see that a separate boot.ts file is preferable for most applications - even if it’s not so important for QuickStart. Let's develop good habits now, as long as the price is low.Convenience Testing
We need to think about the convenience of testing from the very beginning, even if we know that we will never test QuickStart.It is difficult to test a component when the bootstrap function is present in the same file . Every time we load a component file for testing, the bootstrap function tries to load the application in the browser. This causes an error, because we did not expect the launch of the whole application - only a component.Moving the bootstrap function to boot.ts removes the spurious error and leaves us with a clean component file.Reuse
We refactor, rename and move files during the evolution of our application. We will not be able to do any of this until the file calls bootstrap . We can not move it. We cannot reuse the component in another application. We cannot render a component on the server for increased performance.Segregation of duties
The task of the component is to represent the display and control it.Running an application has nothing to do with display control. This is a completely different duty. The problems that we encountered in testing and re-using come precisely from this unnecessary mixing of duties.Import Export
boot.ts , Angular: - . , Angular.