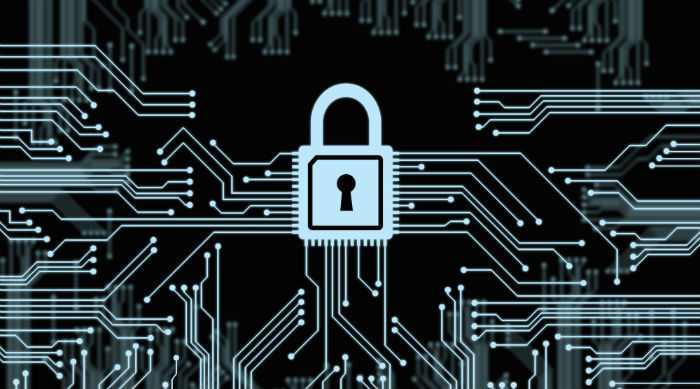
Last year, attackers made 30% more attacks on Russian banks than a year earlier. They tried to withdraw about 6 billion rubles. Often an attack becomes possible due to insufficient security of financial applications.
According to our
statistics , more than half of the remote banking service systems (54%) contained XSS vulnerabilities that allow a MitM attack and intercept access to Internet banking. With mobile banking applications, the situation is no better: 70% of the “wallets” for Android and 50% for iOS in 2014 contained vulnerabilities sufficient to gain access to the account.
')
It is much cheaper to detect vulnerabilities at an early stage than to clear up the consequences of their exploitation. In mid-October, Positive Technologies experts Timur Yunusov and Vladimir Kochetkov conducted a two-day workshop on the safe development of banking applications. Today we present a brief retelling.
Talking about security problems and their possible solutions should start with typical security problems of banking applications.
Access control issues
Such problems arise mainly when implementing the following access control mechanisms:
- identification, authentication, authorization;
- two-factor authentication methods.
Security audits constantly detect such errors as insufficient access control, the ability to gain access to various backend and administrative systems. The most common of these vulnerabilities are found in virtually every bank and banking application.
Often the root of the problems lies in the misuse of crypto protocols and implementations of crypto-primitives (cryptography tools built into the standard .NET libraries, Java, etc.). It is also important to note here that, in principle, the use of low-level crypto-primitives is undesirable, since it is very easy to make a mistake in their configuration and thereby negate all efforts to implement cryptography in a single application.
One of the most striking consequences of such errors is the vulnerability to Padding Oracle attacks, which occurs when using weak modes of block ciphers. Instead of using low-level tools, you should always strive to use high-level libraries like KeyCzar, libsodium.
Another layer of problems associated with the approach of security through obscurity. Each bank uses cryptography (SSL, TLS, etc.) and often encrypts data at the application level (L7). This gives financial organizations the illusion of security, and the thought arises that there is no need to protect anything on the server side now: everything is “wrapped” in cryptography and the attacker simply cannot maliciously send anything to the server.
This is certainly not the case. Cryptography is amenable to reverse engineering, checks in mobile applications are bypassed if the attacker has physical access to the device with the installed banking application. In other words, you can always perform a MitM attack on SSL traffic. Moreover, sometimes vulnerabilities can be exploited even “on top” of cryptography - for example, from the forms on the site.
Workflow management problems
Among the most popular and dangerous errors in managing workflows - and possible attacks based on them - are:
- insufficient process checks;
- race condition and other attacks on atomicity;
- other business logic vulnerabilities;
- CSRF attacks.
This type of problem is the second most frequent detection in banking applications. In order to reduce the likelihood of their occurrence to a minimum and ensure the protection of business logic, it is necessary to formalize each business process. In general, business logic is a bazvord-synonym for the concept “logic of a functional domain”. The subject area is a set of entities, their invariants and rules of interaction with each other.
To avoid the emergence of vulnerabilities in some abstract domain, it is sufficient: a) to have a formalized and consistent description of the invariants of entities and the rules of their interaction; b) implement strict (enforced, without allowable defaults) control over compliance with all invariants and rules of the subject area when entities pass through the boundaries of trust.
Often, the logic of the domain can be expressed as some kind of workflow (workflow or finite automaton), the states of which are sets of admissible invariants of the entities of the domain, and the transition between states is the only way they interact with each other. In this case, we can formulate several specific rules to ensure the security of the implementation of the subject area:
- Recursive paths and loops in the workflow should be avoided.
- It is necessary to take into account possible violation of the integrity of data shared by different streams.
- The current state of the stream must be stored before the boundary of trust, and not behind it (in the case of a two-link, on the server, not on the client)
- It is necessary to implement strict control of the authenticity of the initiator of the transition between workflow states (ineffective control leads, for example, in the case of the Web, to vulnerability for CSRF attacks);
- In case several workflows that share data can work simultaneously, it is necessary to provide granular access to all such data from all such flows.
Flow control problems
Errors in the organization of data flow management can lead to the following serious problems:
- injections (SQL, XSS, XML, XXE, XPath, XQuery, Linq, etc.),
- implementation and execution of arbitrary code on the server side.
The third in terms of detection type of problems of banking applications, although the most extensive. The main drawback here is the inefficient preprocessing of data. It leads to numerous attacks and vulnerabilities: from XSS, which in a banking application can nullify all protection mechanisms (one-time passwords, etc.), to SQL injections, whose presence in financial applications allows you to get absolute access to critical information - accounts, passwords (including one-time) - and carry out the theft of funds.
There are three approaches to preprocessing data:
- typing - coercion of string data to specific types in terms of OOP and further use of these types in the code (parameterization of SQL queries, for example, is an implicit implementation of typing SQL literals);
- sanitization - converting input string data into a view that is safe for use as output (examples are various HtmlEncode, UrlEncode, addslashes, etc.);
- Validation - checking data for compliance with any criteria; two types of validation are possible: syntactic (for example, checking for regular expression compliance) and semantic (for example, checking numbers for entering a specific range).
The order of preference for these approaches is precisely this. That is, where typification is impossible, the possibility of sanitization should be considered, and where sanitization is also impossible, validation should be introduced. This is necessary in order to maximally distance themselves from changing the semantics of the code. In addition, it is necessary to adhere to the rule: “typing / validation at the entrance (as close as possible to the beginning of the code execution flow), sanitization at the output (as close as possible to the place in the code in which data goes outside)”.
Consider a few examples of the application of the approaches described above.
Typing
Suppose we have the following code:
var parm = Request.Params["parm1"]; if (Request.Params["cond1"] == "true") { return; } if (Request.Params["cond2"] == "true") { parm = Request.Params["parm2"]; } else { parm = "<div>Harmless value</div>"; } Response.Write("<a href=\"" + parm + "\">");
Here, a dangerous value is written to
parm
, which leads to the emergence of a vulnerability for XSS attacks, but the context of its use allows typing.
var typedParm = new Uri(Request.Params["parm2"]); var parm = Request.Params["parm1"]; if (Request.Params["cond1"] == "true") { return; } if (Request.Params["cond2"] == "true") { parm = typedParm.GetComponents( UriComponents.HttpRequestUrl, UriFormat.UriEscaped); } else { parm = "<div>Harmless value</div>"; } Response.Write("<a href=\"" + parm + "\">");

Sanitization
We have the following code:
var parm = Request.Params["parm1"]; if (Request.Params["cond1"] == "true") { return; } if (Request.Params["cond2"] == "true") { parm = Request.Params["parm2"]; } else { parm = "<div>Harmless value</div>"; } Response.Write("Selected parameter: " + parm);
It is easy to see that dangerous value is also written here in
parm
. In this case, typing is impossible, but sanitization is possible in the context of a dangerous operation.
var parm = Request.Params["parm1"]; if (Request.Params["cond1"] == "true") { return; } if (Request.Params["cond2"] == "true") { parm = HttpUtility.HtmlEncode(Request.Params["parm2"]); } else { parm = "<div>Harmless value</div>"; } Response.Write("Selected parameter: " + parm);
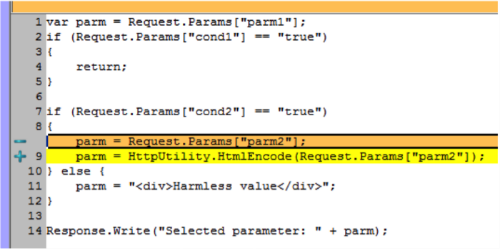
Validation
In the example below (vulnerability to buffer overflow attacks), typing and sanitization are impossible, which means that you need to apply validation:
const int BufferSize = 16; public unsafe struct Buffer { public fixed char Items [BufferSize]; } static void Main(string[] args) { var buffer = new Buffer(); var argument = args[0].ToCharArray(); if (argument.Length < BufferSize) { return; } for (var i = 0; i < argument.Length; i++) { unsafe { buffer.Items[i] = argument[i]; } } }
The code with validation will look like this:
const int BufferSize = 16; public unsafe struct Buffer { public fixed char Items [BufferSize]; } static void Main(string[] args) { Func<int, int> __ai_bkfoepld_validator = index => { if (index >= BufferSize) { throw new IndexOutOfRangeException(); } return index; }; var buffer = new Buffer(); var argument = args[0].ToCharArray(); if (argument.Length < BufferSize) { return; } for (var i = 0; i < argument.Length; i++) { unsafe { buffer.Items[__ai_bkfoepld_validator(i)] = argument[i]; } } }

Infrastructure problems and their solutions
There are also a number of infrastructure problems that can lead to successful attacks on banking systems. Among them:
- application dos
- environmental issues
- third-party software, modules and plugins.
There are also successful attacks with the help of much more trivial unclosed FTP, or IBM / Tomcat admins, etc.
And here is what should be done to improve the security of banking applications at the stage of their development and deployment:
- It is necessary to treat each component of the infrastructure as compromised.
- TLS (not SSL) should be used everywhere, even inside the infrastructure.
- Each infrastructure component must be deployed and configured in accordance with the official security guide (if any) or best practices.
- The use of specialized tools for the analysis of security and compliance with standards (for example, MaxPatrol ) also allows you to significantly increase the level of security.
- All code must be signed, even if the infrastructure does not require it.
- All plug-ins and third-party untrusted modules must be executed in dedicated sandboxes.
Subject areas of banking applications
The experts also told the audience about various banking applications that are not related to the server part of the RBS systems, and possible problems with them:
- Plug-ins and client applications - security errors of various plug-ins and client applications that were not originally related to banking can lead to problems.
- Mobile applications and their typical security problems - as a rule, mobile applications are protected worse than desktop counterparts. Generally speaking, the server part must be equally unified for applications of any type, but often this is not the case.
- Operator stations and applications - often hackers do not even have to break into complex security systems in order to break into the internal network, you just have to deceive the employees of the enterprise - the operators of these systems.
- Development of client attacks - you can steal money from bank customers using targeted attacks on the clients themselves.
Conclusion
Despite the fact that banking applications are causing ever more intense interest of intruders, and there are a number of difficulties in the way of ensuring their security, often problems can be prevented even at the stage of developing a software product.
And you can do this without any extra effort, simply by following the best practices of developing secure software.