Hi, Habr! The time has come when you can say that the
“new build system” Gradle is the industry standard for Android development. The tool is made so simple and convenient that most developers will not experience difficulties without even knowing how it works, and what additional features it has - the problems that arise are easily solved with the help of 5 minutes on StackOverflow, by copying the "magic code" into the configuration files . Perhaps, including because of this, not all developers study Gradle in detail and are not aware of many of its useful features that make life much easier.
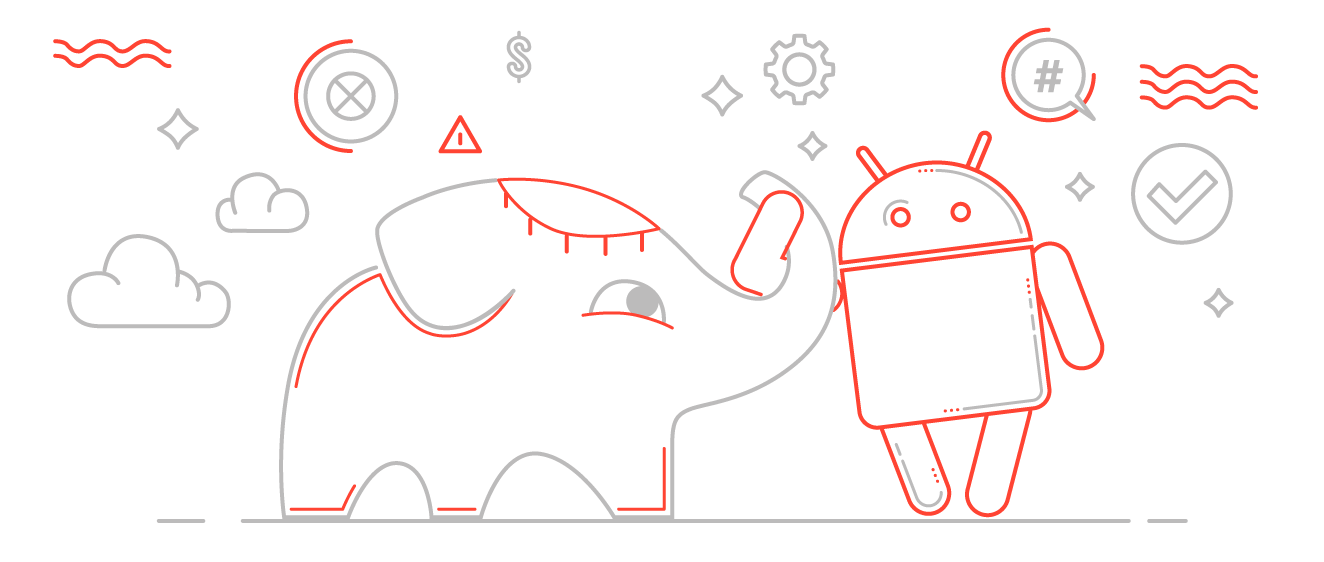
It will be about:
- Increase speed
- BuildConfig Extensions
- Using variables
- Turning off Crashlytics
- Reduced number of resource configurations
1. Increase speed
Assembly time directly affects the speed of development. Tests show that each version, starting with Gradle 2.0, has become slower than the previous one. However, then the developers corrected and worked hard on the speed in Gradle 2.4.
1. Therefore, first of all, you should make sure that you are using the current version of Gradle 2.4+
')
sm:~ sm$ gradle -v Gradle 2.4
2. Then, after making sure that you are trying to speed up your working machine, and not the CI server, turn on the
Gradle daemon - this will give a significant increase in assembly speed.
Configuration lines should be added to the ./%project%/gradle.properties file, if you want to distribute the configuration to all projects, you must configure the file in your user's home folder
~ / .gradle / gradle.properties
org.gradle.daemon=true #
Why on the CI server you should not include Gradle DaemonThe Gradle daemon allows you to do builds more quickly, but when Gradle reuses the runtime of the previous build, for this reason, the critical requirements for CI are not met. Namely, stability and predictability, runtime purity and complete isolation from previous builds.
3. After that, having checked that the modules of your project do not use each other as dependencies, thereby creating cross-references, you can safely turn
on the parallel execution mode , which will also speed up the assembly speed up to ~ 30%.
org.gradle.parallel=true #
If the project uses many modules, it is also worth turning
on the configuration mode if necessary :
org.gradle.configureondemand=true
The more modules in your project, the more you will notice the acceleration, on a small number of modules it may not be.
2. Extend BuildConfig
As you know, the build configuration file (build.gradle) provides the ability to identify
Product Flavors and Build Types , which gives us a lot of options for separating assemblies by purpose. For example, “Build with test server”, “Build with battle server”, ”Build with logging” and others. Using them to extend BuildConfig (which is generated every time you build) gives us tremendous flexibility. For example, convenient switching between the back-end server with which our application works; enable / disable certain functionality - for example, logs.
In build.gradle:
android { ... buildTypes { debug { buildConfigField "String", "SERVER_PREFIX", "\"test.\"" } release { buildConfigField "String", "SERVER_PREFIX", "\"\"" } } }
In java code:
Difference between Product Flavors and Build TypesProduct Flavor is a mechanism that allows us to define various options for building an application. One project can have different variants of assembly (flavors), when choosing a variant (flavor), the generated application will change.
Build Type - the configuration of how the application will be packaged. Each application has two default Build Type debug and release. You can do others. Ideologically, the Build Type is not intended to change the application, only packaging. Actually, this is the main difference, which translates into different
sets of parameters that are provided to customize Product Flavors and Build Types.
3. We use variables
Time does not stand still, which means tools, libraries and Android tend to be updated. And if the application develops, then you have to open build.gradle and change at least compileSdkVersion, buildToolsVersion, Android Support Library versions and Google Play Services. And if we have a lot of modules in the project or different parts of the Google Play Services library, this leads to a lot of changes, and you can easily lose time because of a typo in some of the files. In addition, it is possible to use different libraries and tools in different projects, which is bad and can cause problems. Gradle-variables will help to avoid this situation.
Add to the top build.gradle
In the others ./%module%/build.gradle they can be used, it will look something like this:
android { compileSdkVersion compileSdkProjectVersion buildToolsVersion buildToolsProjectVersion
4. Turn off Crashlytics
In most cases, it is only necessary to collect crashes in the Release assemblies that we release for users / testing. The developer uses the debug build for himself, and the crashes will be visible to him in the log file, which means that in order not to clutter up the list of real crashes among users, you must turn off Crashlytics for Debug builds.
The task can be performed with a banal check of the assembly type:
But this is not the best solution, because The Fabric Gradle plugin will still spend time generating and embedding a unique build id into the application resources so that the Crashlytics back-end then understands which build sent the data. Therefore, we apply a more convenient solution that will speed up the build time of the debug version of the application.
In build.gradle:
android {
After that, debug assemblies will not receive an id, and the build process will speed up, but be aware that if the developer attempts to initialize Crashlytics in such an assembly, the application will drop with the output:
com.crashlytics.android.core.CrashlyticsMissingDependencyException: This app relies on Crashlytics. Please sign up for access at https://fabric.io/sign_up`
Those. Be sure to leave a check for the build type and use Crashlytics only for Release builds or use the solution provided in the Crashlytics documentation on the Fabric website:
5. Reduce the number of resource configurations.
In the applications we develop, we often use third-party libraries, for example, Android Support Library, Google Play Services and others. Many of the libraries come with various internal resources that are absolutely not needed in our applications. For example, Google Play Services comes with a translation into languages that your application does not support. Probably, you also do not want to support mdpi or tvdpi-resolution in your application.
Thanks to the Android Gradle Plugin, we can install the languages and permissions that are used in the application, the rest will be excluded, which will reduce the weight of the application.
If necessary, you can approach more radically and start using multi-APK, especially when a new convenient
Splits mechanism appeared.
Instead of conclusion
We’ll finish this rubric of useful tips on Gradle, there are a lot of them, but, in my opinion, the above mentioned are the most interesting. Surely you have your own, it will be interesting to see them in the comments :) That's it, thank you for your attention!