Many articles on performance optimization, first of all, try to “look under the hood” of Angular and overload the reader with information about the internal organization of the framework. Familiarity with the internal mechanisms of work is very important, but in this article I tried to collect the simplest examples that have the greatest impact on application performance and help to solve typical problems as quickly as possible.
What to measure?
It’s convenient to
measure application performance with the
Batarang Chrome browser extension. This tool shows the execution time of each expression:
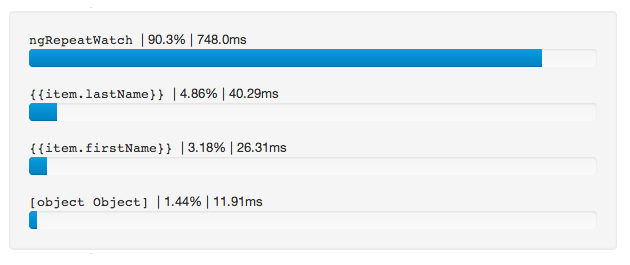
It is convenient to measure the number of watchers themselves by expanding the
Angular watchers .
What to strive for?
The perception of an application depends a lot on the run time of the $ digest cycle. Misko Hevery in his famous response to
stackoverflow says that any changes faster than 50 ms are invisible to humans and, therefore, they can be considered as “instantaneous”. Accordingly, in order for users not to feel “slowing down”, we have to keep within 50–100 ms on each $ digest.
How to achieve this?
In each cycle of $ digest, all watcher functions are called and the entire scope-model is checked for changes. Relatively speaking, the cycle time $ digest = number of observers * their execution time. Thus, in order to optimize the work of $ digest, one must either reduce the number of observers or increase the speed of their calculation.
')
$ scope. $ watch
$scope.myVar = function() { return 2 * 2; } $scope.$watch(myVar, function() { alert('myVar has changed!'); });
Checking the changes in $ scope.myVar will occur
at least once or twice with each $ digest cycle. Therefore, try to avoid costly computations under supervision.
ng-show and ng-switch
If you hide a block with ng-hide or ng-show, the inner elements are not removed from the DOM, but simply hidden through the CSS
display: none style. Therefore, all {{expression}} inside these elements will be evaluated on each pass of $ digest.
Do not watch for variables in invisible elements, or use ng-switch to remove hidden elements from the DOM itself.
{{myName | filter}}
Each filter in AngularJS is executed
at least once or twice with each $ digest cycle. Try not to use heavy calculations in the logic of filters.
$ http
Each time $ http is accessed (and received a response), a $ digest cycle is called. Reduce the number of calls to the server by modifying the transmitted data.
ng-bind
<p>Lorem ipsum dolor sit amet ... mollit anim id {{est}} laborum.</p>
In this example, Angular will monitor not only {{est}}, but also all the text inside <p>. Thus, the entire text (and it can be really big!) Will be stored in memory. To avoid such situations, use the ng-bind binding:
<p>Lorem ipsum dolor sit amet ... mollit anim id <span ng-bing="est"></span> laborum.</p>
ng-repeat
The ng-repeat directive is one of the most expensive in terms of performance. There are no simple ways to optimize it. Therefore, avoid ng-repeat when working with a large data array. Trim the data set before it hits ng-repeat.
{{:: value}}
Starting with version 1.3, AngularJS has such an interesting feature as one-time binding. Normally, values are passed to the DOM like this:
<h1>{{title}}</h1>
To use a one-time binding, you need to add :: before the value:
<h1>{{::title}}</h1>
With a one-time binding, $ watch will be deleted after the first data is output, which means we will win in performance. On the other hand, any model updates will not affect the presentation.
Ui
In the end, the simplest way to optimize Angular is to reduce the number of observers. The mechanism of two-way binding is how convenient, that very often we use it where it is unjustified. Everyone knows about the theoretical limit of "2000 observers", but if we thought about the convenience of users, we would not even get close to this limit.
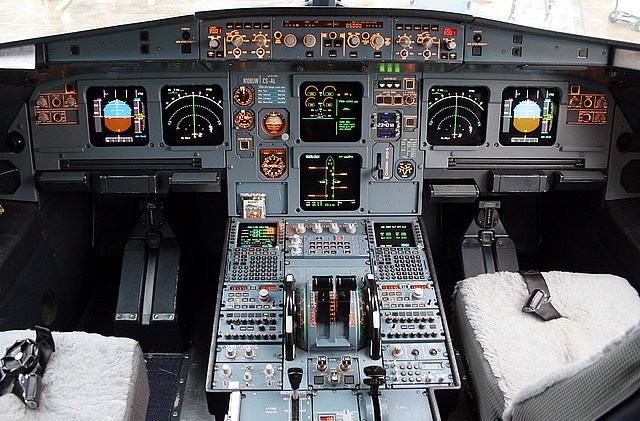
Can our users really understand the simultaneous update of two thousand variables? Can it be better to modify the application so that the user's attention was focused only on the currently important controls? This will not only improve the user experience, but also reduce the number of $ digest cycles performed per unit of time.
Related Links
Accelerate angular.jsSpeeding up AngularJSAngular: Performance