This project demonstrates the definition of a transformer laptop (tablet mode or laptop mode) running Windows 8 (.1), as well as a new control mode using a mouse and touch screen in Windows 10. The control mode using a mouse and touch screen is similar tablet and laptop mode, but in Windows 10, users were able to manually switch the mode, while in Windows 8, the mode switches only depending on the physical state of the device. Therefore, Windows 10 users can use the advanced graphical user interface designed for touch control, even on devices that are not transformers: only the presence of a touch screen is important. This new feature is based on the new
Universal Platform UWP API . You need to add a few lines of code to applications designed for Windows 8 to take advantage of this feature in Windows 10. This document shows how Win32 applications are refined to use the UWP API using WRL (C ++ Windows Runtime Template Libraries) in Windows 10. Inclusion Information UWP applications, see
Microsoft sample code .
Requirements
- Windows 10
- Visual Studio * 2015. New API missing in Visual Studio 2013
Description of the control mode using the mouse and touch screen in Windows 10
Manual setupSwipe the screen from the right edge of the screen to the middle to open the Support Center (the charm-buttons menu in Windows 8).
Touch the “Tablet mode” button to switch between touch mode and mouse control mode.
Selection using equipmentWhen the device-transformer detects a change in physical condition, it notifies the OS.
The OS requests confirmation from the user. If the user confirms, the OS switches the mode.
')

To check, go to “Settings” -> “System” -> “Tablet mode” and check the “Always ask for permission before switching mode” checkbox.
Sample application
Depending on the OS in the sample dialog-based application, the following will occur.
- Windows 10: A manual or automatic mouse control event and the time of this event will be registered during manual or automatic switching.
- Windows 8: physical change events and their time (tablet mode / laptop mode) will be recorded.
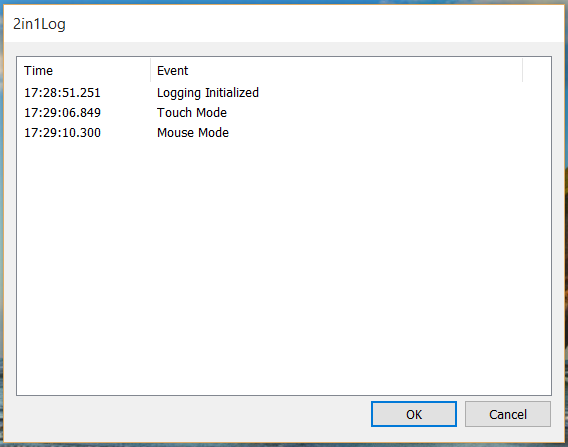
In Windows 8, the
WM_SETTINGCHANGE message
(lParam == “ConvertibleSlateMode”) is transmitted when the physical state changes, and in Windows 10,
WM_SETTINGCHANGE (lParam == “UserInteractionMode”) is transmitted to the top-level window. This also transmits the previous message. The application must determine the OS version and select one or another code depending on it. Otherwise, the application in Windows 10 will respond twice to the messages described above.
void CMy2in1LogDlg::OnSettingChange(UINT uFlags, LPCTSTR lpszSection) { CDialogEx::OnSettingChange(uFlags, lpszSection);
After the application receives a message, it polls the current state, since the message only notifies the OS of the mode change, but not of the current state. There is no Win32 API that allows you to query the new state directly, but you can use the WRL to access Windows RT components from a Win32 application, as shown in the following code snippet.
HRESULT CMy2in1LogDlg::GetUserInteractionMode(int & iMode) { ComPtr<IUIViewSettingsInterop> uiViewSettingsInterop; HRESULT hr = GetActivationFactory( HStringReference(RuntimeClass_Windows_UI_ViewManagement_UIViewSettings).Get(), &uiViewSettingsInterop); if (SUCCEEDED(hr)) { ComPtr<IUIViewSettings> uiViewSettings; hr = uiViewSettingsInterop->GetForWindow(this->m_hWnd, IID_PPV_ARGS(&uiViewSettings)); if (SUCCEEDED(hr)) { UserInteractionMode mode; hr = uiViewSettings->get_UserInteractionMode(&mode); if (SUCCEEDED(hr)) { switch (mode) { case UserInteractionMode_Mouse: iMode = UserInteractionMode_Mouse; break; case UserInteractionMode_Touch: iMode = UserInteractionMode_Touch; break; default: break; } } } } return S_OK; }
Conclusion and other features
This sample code shows the implementation of detecting the operation of a transformer in Windows 8 / 8.1 and Windows 10 using Win32. In Windows Store apps running Windows 8, it was not possible to detect transformer events. In Windows 10, UWP APIs are supported so that universal applications can use the functionality of transformers. Instead of using a similar Win32 API, a method is presented for using the UWP API from a Win32 application. It should be noted that the UWP APIs do not have special notification for this event; they use window resizing events and then check the current state. If the state is different from the saved one, then it is assumed that it has changed. If using Win32 messages is inconvenient (for example, in Java * applications), you can use the window resize event in Java and call the JNI shell to confirm the state.