ExecutionHandler
component, which will be described in this part of the article, is redundantly simple, since it only executes orders at the current market price. This is an absolutely unrealistic scenario, but it serves as a good initial point for subsequent improvements and complications.FillEvent
and OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
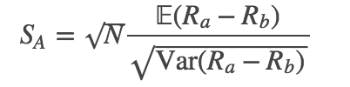
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
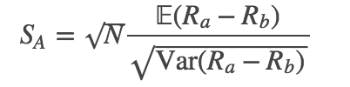
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
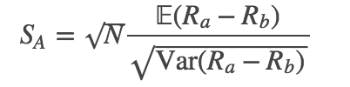
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
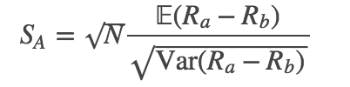
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
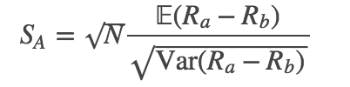
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
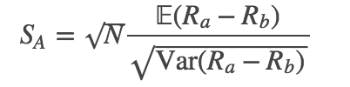
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
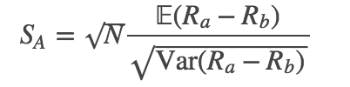
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
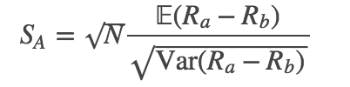
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
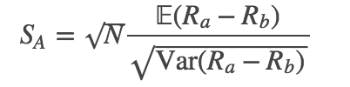
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
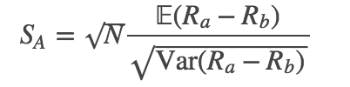
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
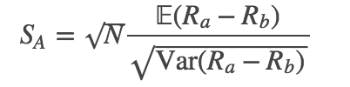
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
OrderEvent:
# execution.py import datetime import Queue from abc import ABCMeta, abstractmethod from event import FillEvent, OrderEvent
ExecutionHandler
execute_order
:
# execution.py class ExecutionHandler(object): """ ExecutionHandler , Portfolio Fill, . . . """ __metaclass__ = ABCMeta @abstractmethod def execute_order(self, event): """ Order , Fill, Events. : event - Event """ raise NotImplementedError("Should implement execute_order()")
. , , . , , , , .
, FillEvent
fill_cost
None
(. execute_order
), NaivePortfolio
( ). βvalueβ, .
ARCA. .
# execution.py class SimulatedExecutionHandler(ExecutionHandler): """ Fill, , .. . """ def __init__(self, events): """ , . : events - Event. """ self.events = events def execute_order(self, event): """ Order Fill, , , / . : event - Event . """ if event.type == 'ORDER': fill_event = FillEvent(datetime.datetime.utcnow(), event.symbol, 'ARCA', event.quantity, event.direction, None) self.events.put(fill_event)
. , .
- . :
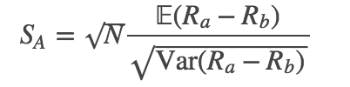
R a , R b β , .
β , . , , .
.
Python
performance.py
, . , , NumPy Pandas:
# performance.py import numpy as np import pandas as pd
, β . , .
252 β . , , , . : 252 β 6.5 = 1638 ( ). , 60: 252β6.5β60 = 98280.
create_sharpe_ratio
Pandas Series returns
.
# performance.py def create_sharpe_ratio(returns, periods=252): """ , ( ). : returns - Series Pandas - (252), (252*6.5), (252*6.5*60) ... """ return np.sqrt(periods) * (np.mean(returns)) / np.std(returns)
, ( ) , .
create_drawdowns
, , β . β «», .
pandas Series, . HWM (high water mark) β , .
HWM . , , , HWM. :
# performance.py def create_drawdowns(equity_curve): """ PnL . pnl_returns pandas Series. : pnl - pandas Series, . : drawdown, duration - """ # # High Water Mark # hwm = [0] eq_idx = equity_curve.index drawdown = pd.Series(index = eq_idx) duration = pd.Series(index = eq_idx) # for t in range(1, len(eq_idx)): cur_hwm = max(hwm[t-1], equity_curve[t]) hwm.append(cur_hwm) drawdown[t]= hwm[t] - equity_curve[t] duration[t]= 0 if drawdown[t] == 0 else duration[t-1] + 1 return drawdown.max(), duration.max()
, , . . , , Portfolio
, .
portfolio.py
:
# portfolio.py .. # from performance import create_sharpe_ratio, create_drawdowns
Portfolio
β , . NaivePortfolio
. output_summary_stats
β .
. pandas, :
# portfolio.py .. .. class NaivePortfolio(object): .. .. def output_summary_stats(self): """ β . """ total_return = self.equity_curve['equity_curve'][-1] returns = self.equity_curve['returns'] pnl = self.equity_curve['equity_curve'] sharpe_ratio = create_sharpe_ratio(returns) max_dd, dd_duration = create_drawdowns(pnl) stats = [("Total Return", "%0.2f%%" % ((total_return - 1.0) * 100.0)), ("Sharpe Ratio", "%0.2f" % sharpe_ratio), ("Max Drawdown", "%0.2f%%" % (max_dd * 100.0)), ("Drawdown Duration", "%d" % dd_duration)] return stats
. . performance.py
output_summary_stats
.
β¦
PS . ITinvest - .
Source: https://habr.com/ru/post/268929/
All Articles