Hi habrayuzer. In the
I told you how to create a base scene with two meshes - a plane and a sphere. Now let's take a look at all the meshes that the framework offers.
Cube

var box = BABYLON.Mesh.CreateBox("box", 6.0, scene);
Let's look at the parameters:
box - unique identifier for further interaction;
6.0 - the size of the mesh;
scene - the scene of its placement;
Sphere
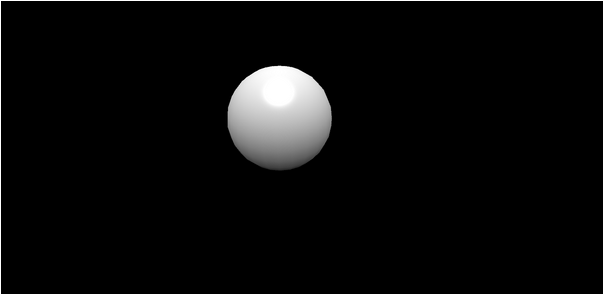
var sphere = BABYLON.Mesh.CreateSphere("shpere", 10.0, 8.0, scene);
Consider the options:
sphere - a unique identifier for further interaction;
10.0 - quality (attention, the more this value, the longer the computer will render the scene);
8.0 - the size;
scene - placement scene;
Plane

var plane = BABYLON.Mesh.CreatePlane("plane1", 10.0, scene);
Consider the options:
plane - a unique identifier for further interaction;
10.0 - dimensions;
scene - placement scene;
')
Disk
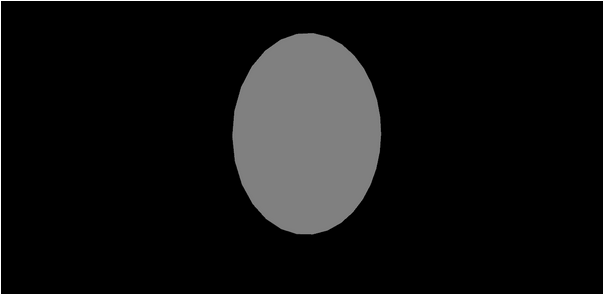
var disc = BABYLON.Mesh.CreateDisc("disc1", 5, 30, scene);
Consider the options:
disk - a unique identifier for further interaction;
5 - size;
30 - quality (attention, the more this value, the longer the computer will render the scene) ;
scene - placement scene;
Cylinder

var cylinder = BABYLON.Mesh.CreateCylinder("cylinder1", 8, 5, 4, 6, 1, scene);
cylinder - a unique identifier for further interaction;
8 - height;
5 - diameter of the “upper” cover;
4 - diameter of the “lower” cover;
6 - tessellation;
1 - subdivs;
scene - placement scene;
Torus
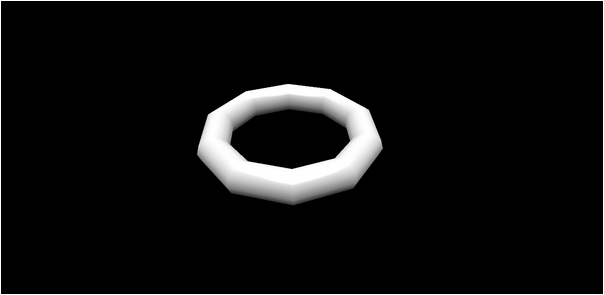
var torus = BABYLON.Mesh.CreateTorus("torus1", 5, 1, 10, scene);
torus - a unique identifier for further interaction;
5 - diameter;
1 - thickness;
10 - tessellation;
scene - placement scene;
Whip
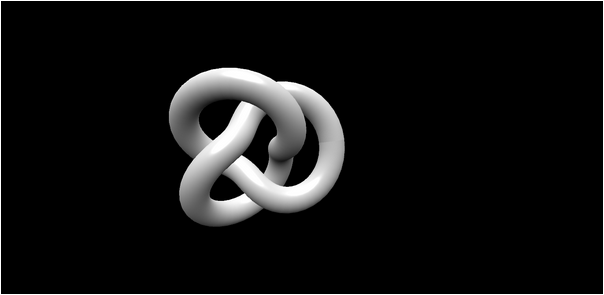
var knot = BABYLON.Mesh.CreateTorusKnot("knot", 2, 0.5, 128, 64, 4, 3, scene);
Consider the parameters (in view of the complexity of the translation, some description of the parameters is left in the author's form)
knot - unique identifier;
2 - radius;
0.5 - tube;
128 - radian segment;
64 - tubular segment
4 - p;
3 - q;
scene - placement scene;
Line (or straight)
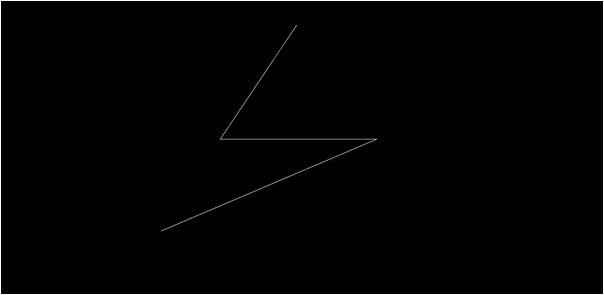
var lines = BABYLON.Mesh.CreateLines("lines1", [ new BABYLON.Vector3(0, 0, 0), new BABYLON.Vector3(10, 0, 0), new BABYLON.Vector3(0, 10, 10), new BABYLON.Vector3(10, 10, 0), new BABYLON.Vector3(10, 0, 10), new BABYLON.Vector3(0, 10, 0), new BABYLON.Vector3(-5, -10, 10) ], scene);
Note that when creating multiple lines at the end of the last line, no comma is entered. Please make sure your code is formatted in the same way.
Land (or playground)
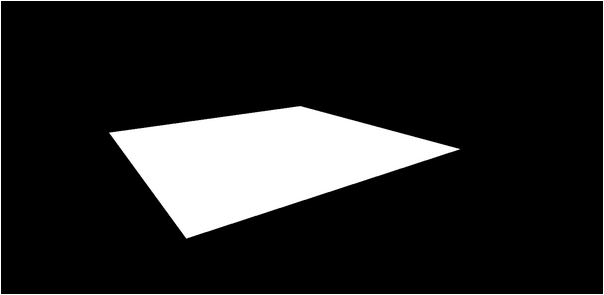
var ground = BABYLON.Mesh.CreateGround("ground1", 6, 8, 2, scene);
ground is a unique identifier for further interaction;
6 - width;
8 - depth;
2 - subdivs;
scene - placement scene;
Accommodation
Now let's see how to place these meshes. BabylonJS uses the standard XYZ coordinate system:
box1.position.x = 10; box1.position.y = -10; box1. position.z = 10;
Or, you can use Vector3:
box1.position = new BABYLON.Vector3(10, -10, 10);
That's all. Try to work with these meshes, you can even build something interesting from them, for example - a turret with a sphere:
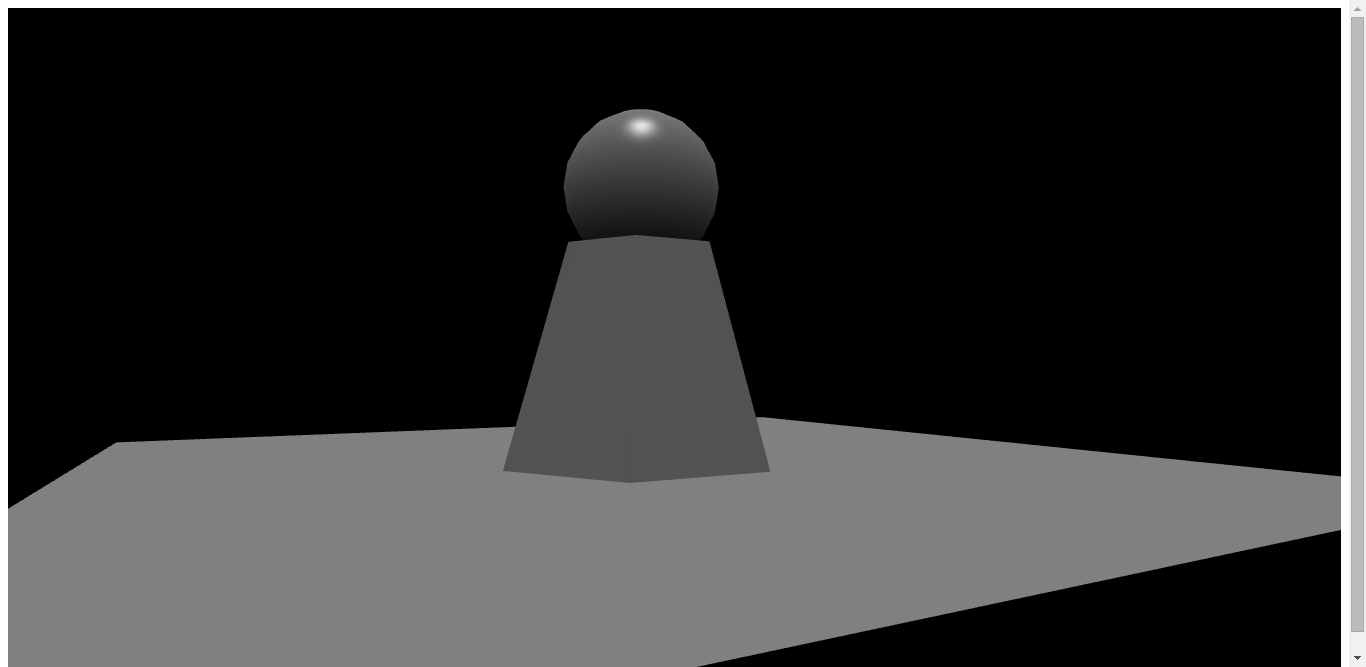
Example | Source