Creating forms using pure HTML is a rather trivial task and is discussed in detail in every self-respecting textbook. But everything becomes much more difficult if you need, for example, to spread form elements across several tabs. In this case, the framework is indispensable. In this article I will explain how you can create complex web forms using the
Webix UI framework.
')
The basics. How to create a simple form
First let's look at how you can create a form using the
Form Component . In Webix, all interface elements are described via JSON. To create a form, use the object with the
view:"form"
property. For the same object, you need to set the
elements
property — this is an array containing the elements that your form consists of.
Here's how it works:
webix.ui({ view:"form", elements: [ { }, { }, { } ] });
Any other Webix UI component can be used as a form element: a text field, a checkbox, a button, etc.
In order to see how this all works in practice, let's create a simple form as an example. To do this, we need: two
text fields : one for the username and one for the password; one
checkbox to ensure that the user accepts the terms of the agreement; and the last element is
the Submit
button .
So, the code:
webix.ui({ view:"form", id:"myForm", container: "areaA", width: 350, elements: [ {view:"text", label:"Username", name:"username"}, {view:"text", label:"Password", name:"password", type:"password"}, {view:"checkbox", labelRight:"I accept terms of use", name:"accept"}, {view:"button", value: "Submit", width: 150, align:"center", click:submit} ] });
Nothing that we were ready for. Property
view:"form"
says that we are interested in the form. The
label
properties work in the same way as the HTML
label
tag. You can get the value of each form element through the
name
property. To hide the characters entered in the Password field, use the
type:"password"
property
type:"password"
. What is interesting is the
click:submit
property in the button description code. It defines the function that will be called after the button is pressed. This property is optional, but it will be useful to check with its help whether everything is good with the entered data.
Here is a sample code that displays the entered values. It uses the
Webix Message Box to display messages:
function submit(){ webix.message(JSON.stringify($$("myForm").getValues(), null, 2)); }
In this example, using the
getValues ​​() method, we get the values ​​of the Webix elements, and the
JSON.stringify () method is used to convert the received data into a JSON string.
So, everything is ready and we can check the result:

After entering the data, you can check the operation of the form using the Submit button:
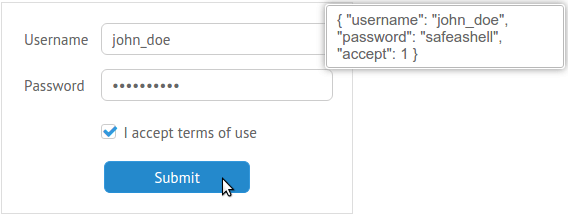
Everything works as it should. Since we now know the basics of creating forms, you can try to create something more complex.
The code and demo can be found here -
webix.com/snippet/2259ec41Using complex controls on the example of Multicombo
Webix provides several controls that help the user with the selection while working with forms. In my opinion, one of the most interesting of them is the
Multicombo . This control allows you to implement a multiple choice of the elements of the drop-down list.
For example, you want to create a page to create a developer summary. Here is a simplified example of a form you can use:
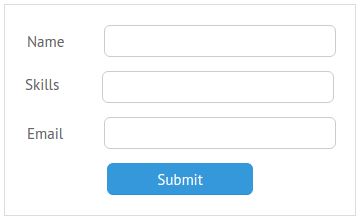
Since it is likely that the user is proficient in more than one programming language and armed with a list of languages, this is the case when you can use Multicombo. Here is an example of a
data.js file that contains the necessary data:
var languages = [ {id:1, lang:"4th Dimension/4D"}, {id:2, lang:"ABAP"}, ]
Let's use Multicombo in the Skills field:
{view:"multicombo", name:"skills", label:"Skills", button: true, suggest: { body:{ data:languages, template:webix.template("#lang#") } }, }
In addition to the properties already familiar to us, there is something new in this example. The
button: true
property will create a selection confirmation button in the drop-down list. To specify the content source for the drop-down list, the
suggest
property is
suggest
. You can, for example, just specify the path to the file:
suggest: "path/to/file/data.js"
. But it is best to use the template in combination with the
data
property, which will allow you to extract exactly the data you need from the target archive.
Below is an example of the operation of such a control. After clicking in the text box, a drop-down menu will appear with options available for selection:
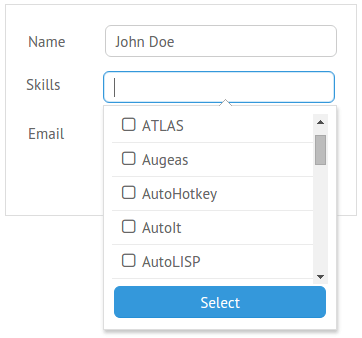
You can scroll through the list and select the options you want or start typing:
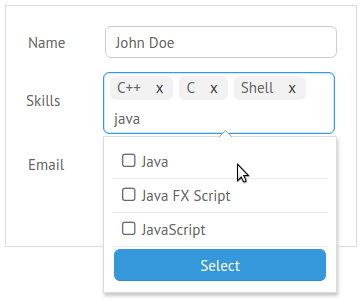
The form from this example will return a list of selected item IDs:

The code and demo can be found here -
webix.com/snippet/63f6328eControls such as
Grid Suggest and
Dataview Suggest may also be useful.
Using a tree as a form element
Webix does not limit you to using text fields, buttons, checkboxes, and other familiar components as form elements. You can put almost any widget into a form. Let's try this with the example of a
Tree . It was not originally intended for use inside a form, so it does not have the
setValue()
and
getValue()
methods necessary to get data about the selected items. How can I fix this? You can use the
protoUI method, which allows you to create new elements based on existing ones:
webix.protoUI({ name:"formTree", setValue:function(value){ this.checkItem(value); }, getValue:function(){ return this.getChecked(); } }, webix.ui.tree);
In this example, we have created a new component called
formTree
. Then we defined the
setValue()
and
getValue()
methods for it. And, finally, indicated that we want to create a new component based on the tree.
Before using it, let's create the necessary data for our tree:
var treedata = [ { id: "1", value: "Web", data: [ { id: "1.1", value: "HTML" }, { id: "1.2", value: "CSS" }, ]}, {id:"2", value:"Scripting", data: [ { id: "2.1", value: "Shell" }, ]}, ]
Now you can add a new element to the form. This can be done in the same way as in the case of any other element:
{view:"formTree", name:"skills", data:treedata, height: 150, threeState: true, template: "{common.icon()} {common.checkbox()} #value#" },
The
threeState:true
property enables
3-state checkboxes , and the template adds checkboxes to tree nodes.
Result:

To check how well the methods defined by us for the new component work:
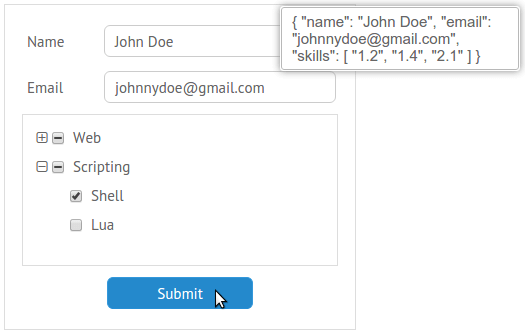
The code and demo can be found here -
webix.com/snippet/9f166141Tabs Forms and Multistep Forms
If you want to create a large enough form, it may be useful to divide it into several tabs or create a multi-step form. Webix allows you to put inside the form of different UI components to achieve the desired result.
Adding Tabs with Tabview
Tabview component allows you to create a set of components with a tabbar used to switch between them. You can use a separate component as the content of each tab or create your own layout using the
rows
and
cols
.
This is how Tabview works:
view:"tabview", cells: [ { header:"First tab label", body: { } }, { header:"Second tab label", body: { rows:[ { }, { } ] } }, ]
The main idea of ​​this approach is to put Tabview with the required fields inside the form. However, it is worth remembering that components common to the entire form (for example, the Submit button or the “I agree” checkbox) should be located outside of Tabview.
For example:
view:"form", elements:[ {view:"tabview", height:250, cells:[ {header:"Personal Info", body:{ rows:[ {view:"text", label:"Name", name:"name"}, ] } }, {header:"Delivery Details", body:{ rows:[ ] } } ] }, {view:"button", value: "Submit", width: 150, align:"center", click:submit} ]
This is all you need to create a form with tabs. Below is the result:
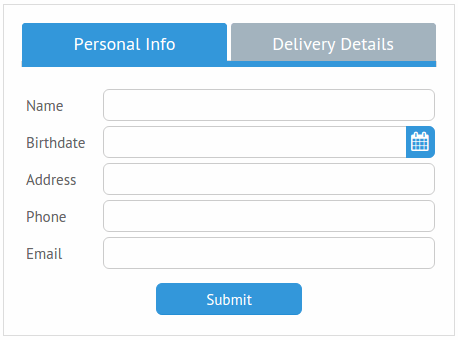
The best part of this whole story is that you don’t need to write any additional code in order for the different parts of the form to work as a unit. Just put Tabview inside the form and don't forget about the
name
property for each of its elements.
Submit button confirms this:
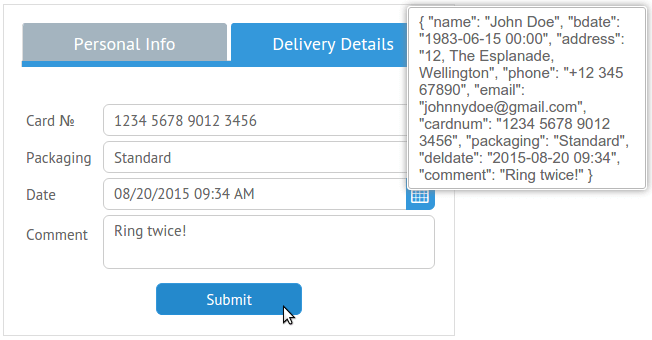
The code and demo can be found here -
webix.com/snippet/79eb2712As an alternative to tabs, you can try, for example,
Accordion .
Creating a multi-step form with Multiview
Multiview allows you to create a sequence of elements and view them one by one, which saves screen space. Moreover, the switching takes place with the animation effect! You can create tabs to switch between components, but since we are interested in a multi-step form, let's use the buttons for our example.
First of all, we need functions for switching between form elements:
function next(){ var parentCell = this.getParentView().getParentView(); var index = $$("formCells").index(parentCell); var next = $$("formCells").getChildViews()[index+1] if(next) next.show(); } function back(){ $$("formCells").back(); }
This code uses the ID value of the Multiview element, so be sure to set it. As for the form code, it practically does not change. We just need to change the contents of the
elements
array. The Multiview component itself is created in a similar way to the Tabview element from the previous example. This time we need to add an additional row inside this component, which will contain control buttons.
Here's how to do it:
{view:"multiview", id:"formCells", cells: [ {rows:[ {}, {cols:[ {}, {view:"button", value:"Next", click:next} ]} ]}, {rows:[ {}, {cols:[ {view:"button", value:"Back", click:back}, {view:"button", value:"Next", click:next} ]} ]}, {rows:[ {}, {cols:[ {view:"button", value:"Back", click:back }, {view:"button", value:"Submit", click:submit} ]} ]} ]}
Let's look at the result:
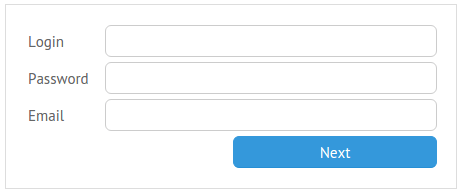
After clicking the Next button, the following part of the form will appear:
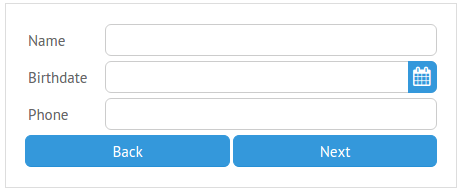
And now let's check the entered data:

Everything is working.
The code and demo can be found here -
webix.com/snippet/39464194Conclusion
Webix allows you to create almost any combination of components. Any widget or control can be placed inside the form. If you are not satisfied with the functionality of a specific component, you can create your own using it with
protoUI
and, again, place it into a form. Thus, almost nothing limits your imagination.