Good day to all, I recently encountered such a problem that when writing text (long text) in a table cell, its Label does not increase proportionally to the amount of text, that is, if there are 3 words, then everything is fine, but if you write 3-4 sentences there , then something like this will appear.

The weekly search for a solution to the problem turned out to be all the same successful and today I will try to explain to you exactly how to solve this problem.
After you have created
NavigationController , made your ViewController a heir from UITableViewController, we need to create a class for our custom cell. Create a new class, call it, for example, customCell and indicate that it is derived from the
UITableViewCell class.
')
#import "customCell.h" @implementation customCell - (void)awakeFromNib { // Initialization code } - (void)setSelected:(BOOL)selected animated:(BOOL)animated { [super setSelected:selected animated:animated]; // Configure the view for the selected state }
In the * .m file, 2 methods will be created immediately, which in our case we will not need.
Next, put in our prototype cell UILabel. We click on it with the mouse and set such settings, it is important that these parameters be exactly like that.
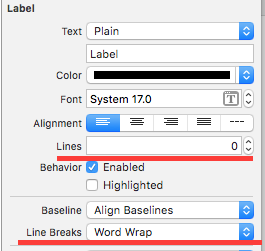
Lines = 0 means that a cell can have an unlimited number of lines, and Line Break = Word Wrap, that words will be transferred to a new line when the place in this one ends. But that's not all, now we need to link our UILabel with the customCell.h file
After that, go back to the ViewController file and use the
heightForRowAtIndexPath delegate.
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
You can perform a calculation in this delegate directly in your ViewController, but I would advise you to transfer all this to customCell.m to save space in the main file.
Go to customCell.h and create a method with a plus sign.
+(CGFloat) heightForText:(NSString*) text;
And back to the .m file for its implementation.
In order for a cell to calculate its height, we need to write
down the attributes of our text, that is, its font size, indents, paragraphs, shadows, and so on, in
NSDictionary . Next, call the method that calculates all the rows and gives the total height of the cell.
boundingRectWithSize
we write the length of the cell into it, with or without indents, height (if you need to limit it) and “shove” your Dictionary with attributes. Sample code below.
+(CGFloat) heightForText:(NSString*) text { CGFloat offset = 1.0; UIFont* font = [UIFont systemFontOfSize:17.f]; NSMutableParagraphStyle* paragraph = [[NSMutableParagraphStyle alloc] init]; [paragraph setLineBreakMode:NSLineBreakByWordWrapping]; NSDictionary* attributes = [NSDictionary dictionaryWithObjectsAndKeys: font , NSFontAttributeName, paragraph, NSParagraphStyleAttributeName, nil]; CGRect rect = [text boundingRectWithSize:CGSizeMake(320 - 2 * offset, CGFLOAT_MAX) options:NSStringDrawingUsesLineFragmentOrigin | NSStringDrawingUsesFontLeading attributes:attributes context:nil]; return CGRectGetHeight(rect) + 2 * offset; }
Now we’ll go back to the ViewController and in the delegate using
return call the cusomCell class method and get information about the height of your cell from it.
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath { return [customCell heightForText: self.textStringHabr]; }
We are checking.
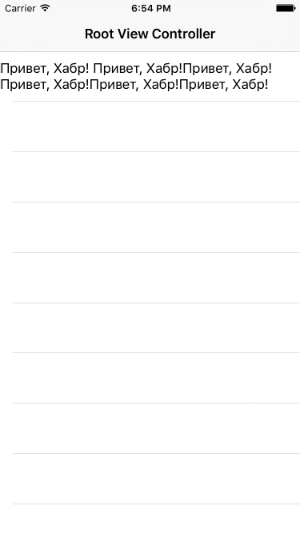
Everything is working!
Now the cell itself calculates the height you need. The main thing is not to forget that we created a custom cell and indicate this in two mandatory tabular methods.
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return 1; } - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString* identifier = @"cell"; customCell* cell = [tableView dequeueReusableCellWithIdentifier:identifier]; if (!cell) { cell = [[customCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:identifier]; } cell.testTextLabel.text = self.textStringHabr; return cell; }
For those who write in Swift, everything is much simpler, Apple saved us from writing this code and everything is done with just two lines of code.
self.tableView.rowHeight = UITableViewAutomaticDimension self.tableView.estimatedRowHeight = 44.0
Information taken from the lessons of
Alexey Skutarenko .
Thank you for your attention, I hope that I could help at least something.