# x <- c(5, 8, 7, 5, 3, 4, 3, 9, 5, 8, 5, 6, 5, 6, 4, 7) # The log posterior density of the Laplace distribution model, when assuming # uniform/flat priors. The Laplace distribution is not part of base R but is # available in the VGAM package. model <- function(pars) { sum(VGAM::dlaplace(x, pars[1], exp(pars[2]), log = TRUE)) } # The Metropolis-Hastings algorithm using a Uniform(-0.5, 0.5) proposal distribution metrop <- function(n_samples, model, inits) { samples <- matrix(NA, nrow = n_samples, ncol = length(inits)) samples[1,] <- inits for(i in 2:n_samples) { curr_log_dens <- model(samples[i - 1, ]) proposal <- samples[i - 1, ] + runif(length(inits), -0.5, 0.5) proposal_log_dens <- model(proposal) if(runif(1) < exp(proposal_log_dens - curr_log_dens)) { samples[i, ] <- proposal } else { samples[i, ] <- samples[i - 1, ] } } samples } samples <- metrop(n_samples = 1000, model, inits = c(0,0)) # Plotting a traceplot plot(samples[,1], type = "l", ylab = expression(Location ~ mu), col = "blue") # Calculating median posterior and 95% CI discarding the first 250 draws as "burnin". quantile(samples[250:1000,1], c(0.025, 0.5, 0.975))
## 2.5% 50% 97.5% ## 4.489 5.184 6.144
CLS
CLS
) and variable definitions. DIM
defines arrays and matrices.75
75
in the screenshot). Since there are no functions in the old BASIC, the line numbering will be used by GOSUB
commands GOSUB
GOSUB
and GOTO.
GOTO.
SAMPLES!
SAMPLES!
In QBasic, all variables are numbers and to change their type you need to add a symbol to the variable name. So THIS$
THIS$
This is a string, and THAT!
THAT!
Is a floating point number. Since the parameters are continuous, most of the variables will end in "!".DATA
command DATA
- This is a good way to put data into the program, you can later retrieve it using the READ.
function READ.
READ.
If you have more than one data set, good luck.RETURN
RETURN
. It is caused by the expression GOSUB
GOSUB
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
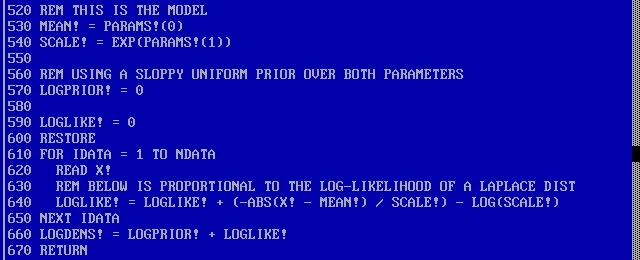
-. , GOSUB 520,
520. RND,
0 1.
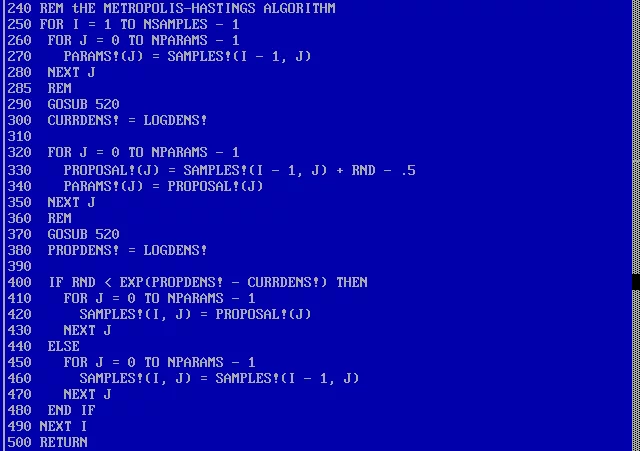
:

SAMPLES!
, 95 .
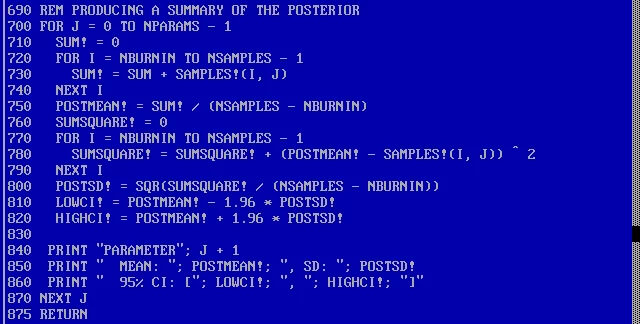
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
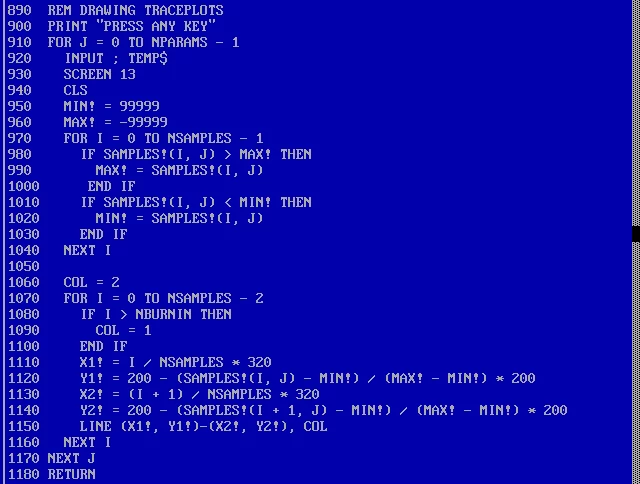
:
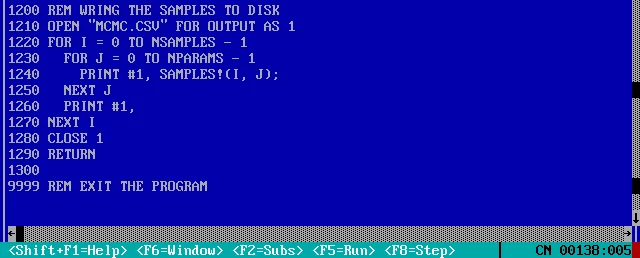
, .
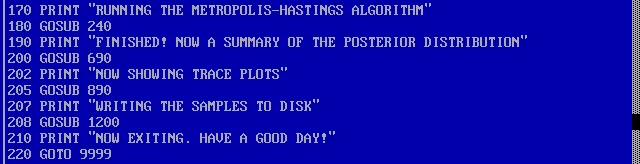
GOTO
, . 33 MHz, 386 ( ), , . :
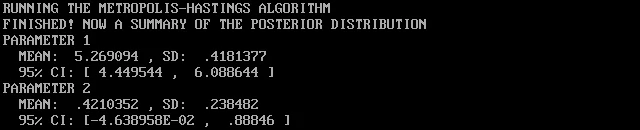
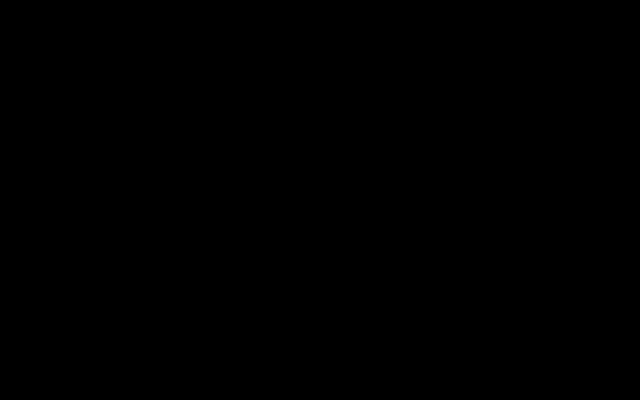
, , 4-5 6. , BASIC R :
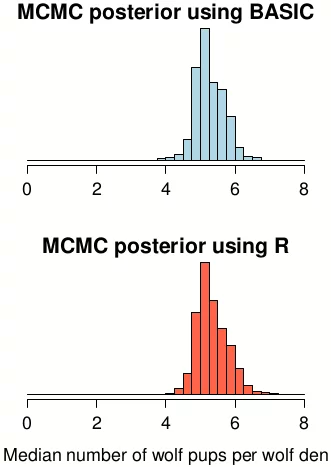
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
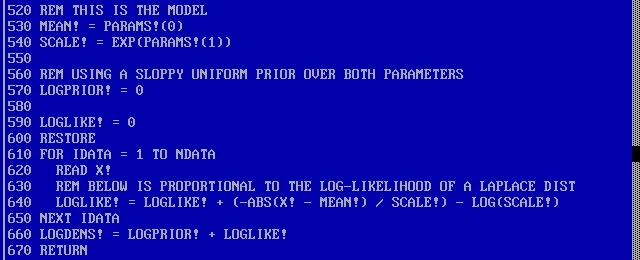
-. , GOSUB 520,
520. RND,
0 1.
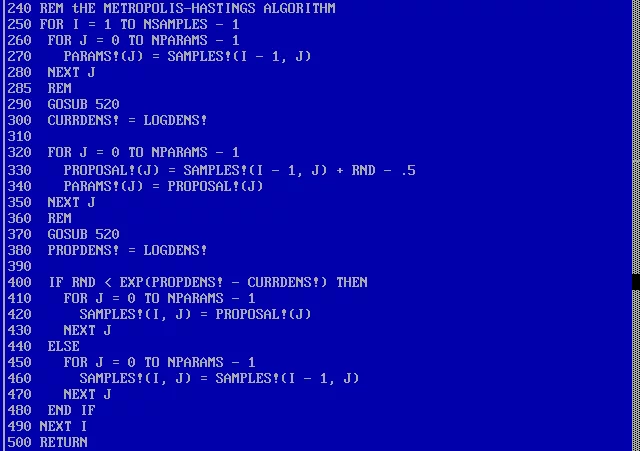
:

SAMPLES!
, 95 .
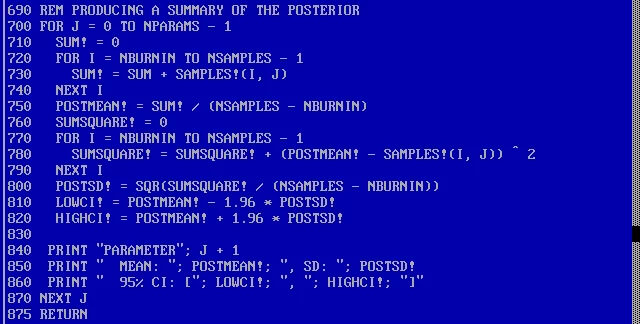
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
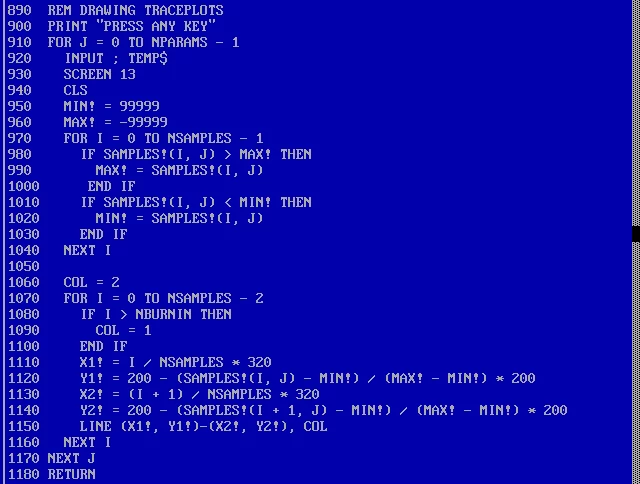
:
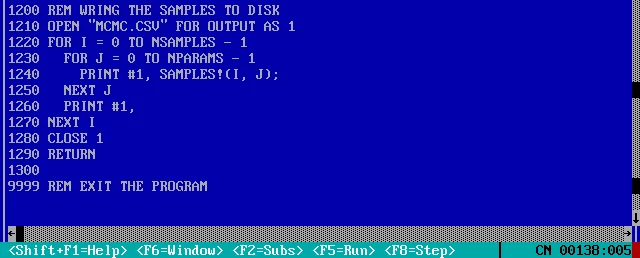
, .
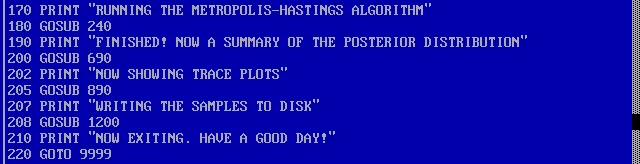
GOTO
, . 33 MHz, 386 ( ), , . :
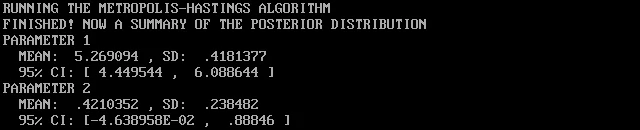
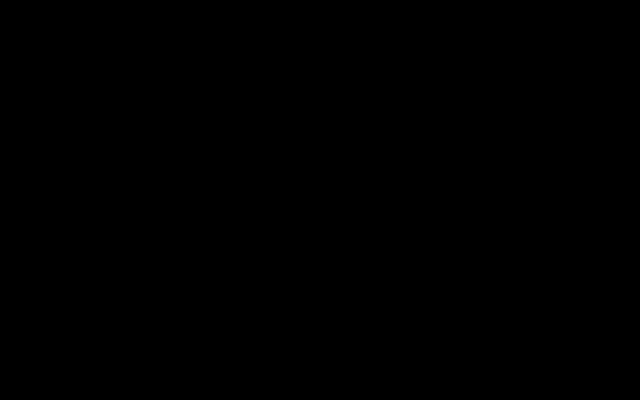
, , 4-5 6. , BASIC R :
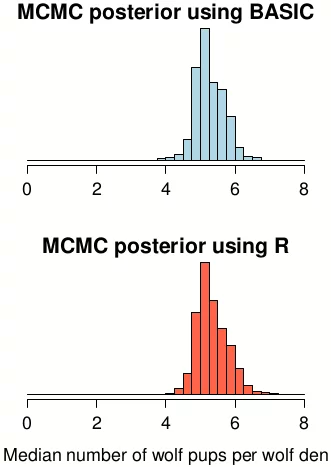
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
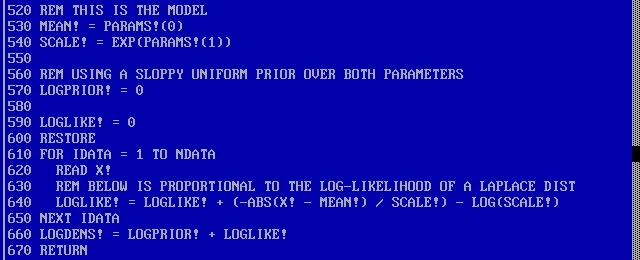
-. , GOSUB 520,
520. RND,
0 1.
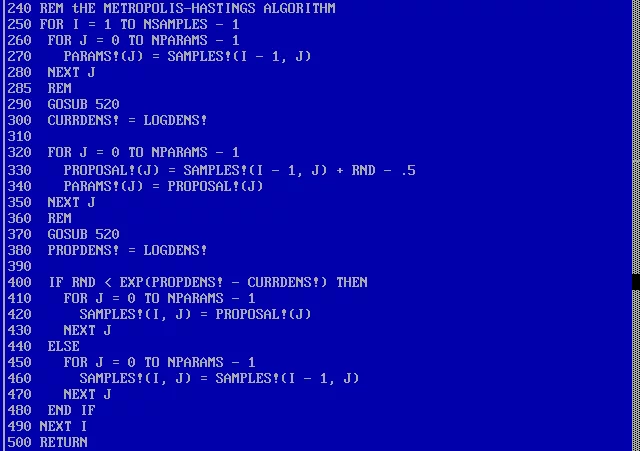
:

SAMPLES!
, 95 .
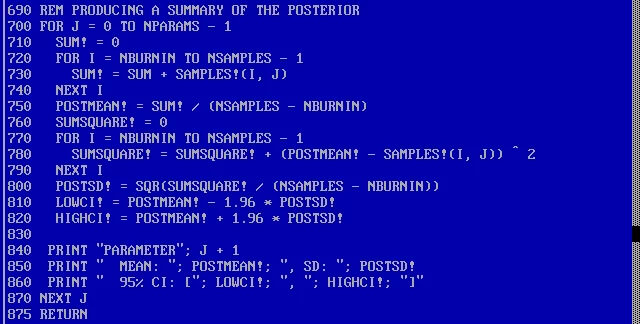
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
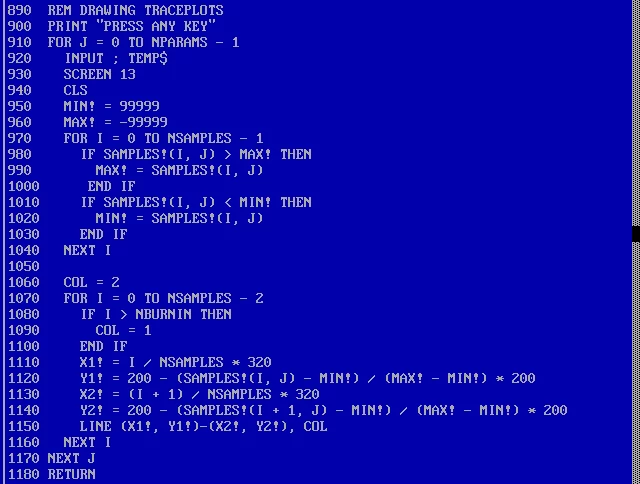
:
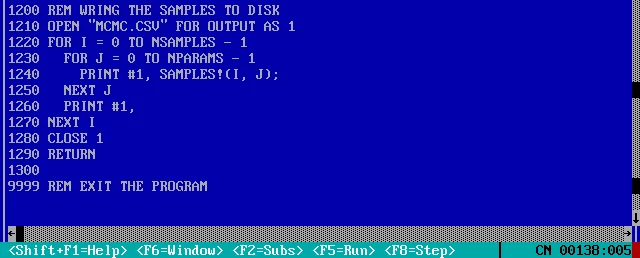
, .
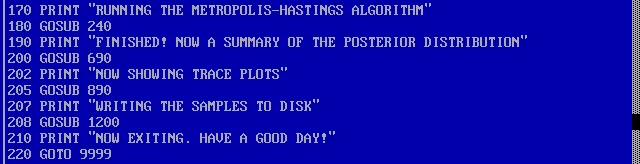
GOTO
, . 33 MHz, 386 ( ), , . :
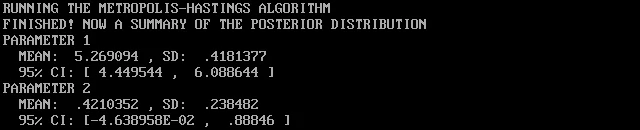
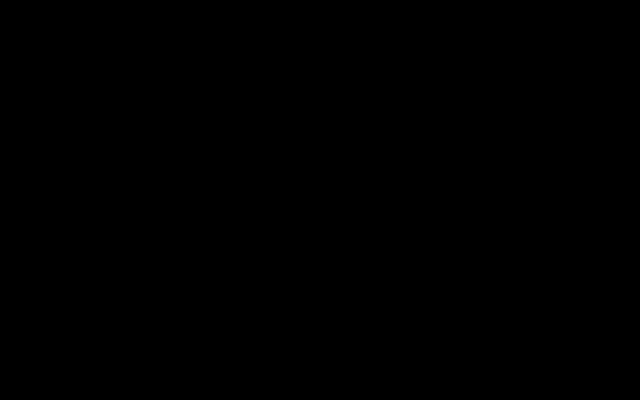
, , 4-5 6. , BASIC R :
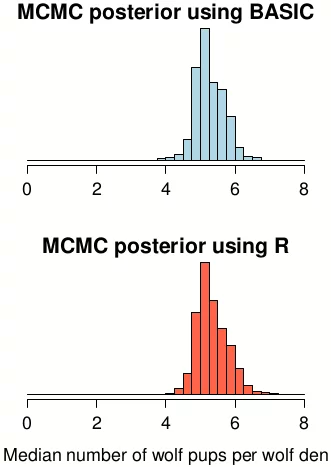
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
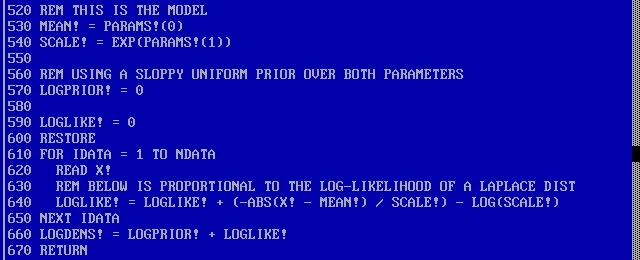
-. , GOSUB 520,
520. RND,
0 1.
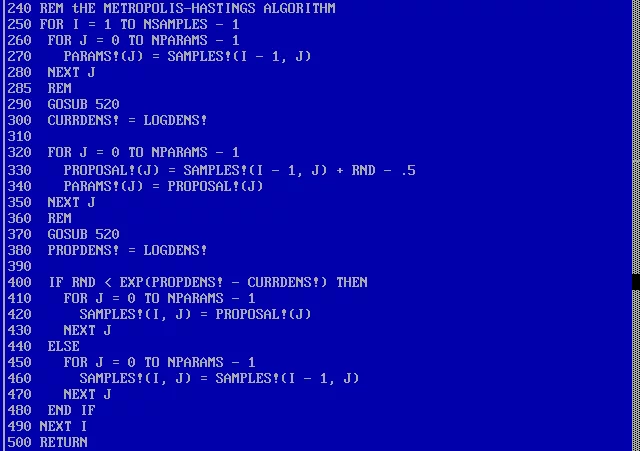
:

SAMPLES!
, 95 .
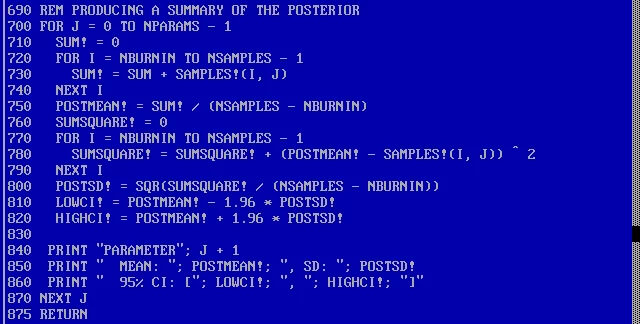
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
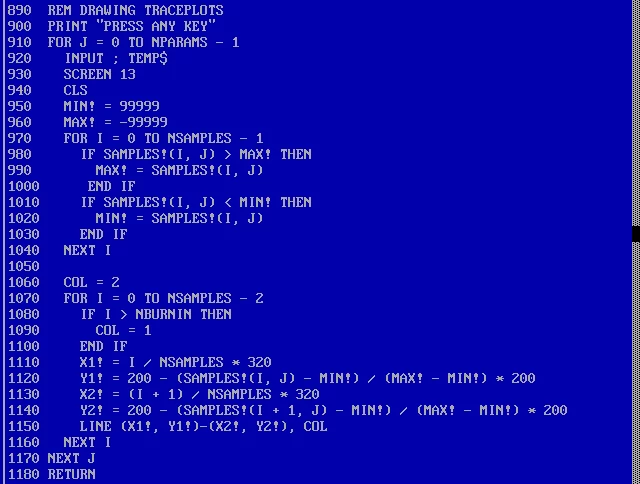
:
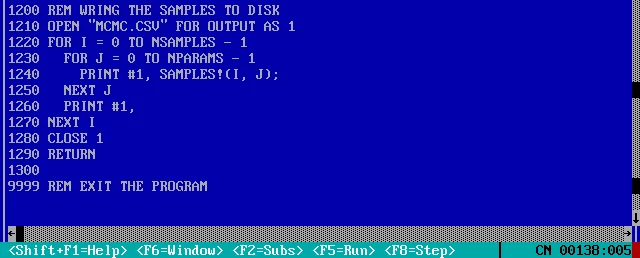
, .
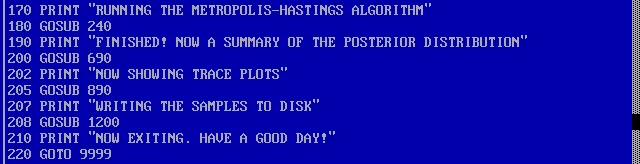
GOTO
, . 33 MHz, 386 ( ), , . :
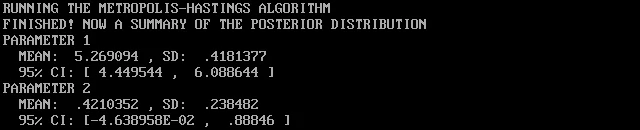
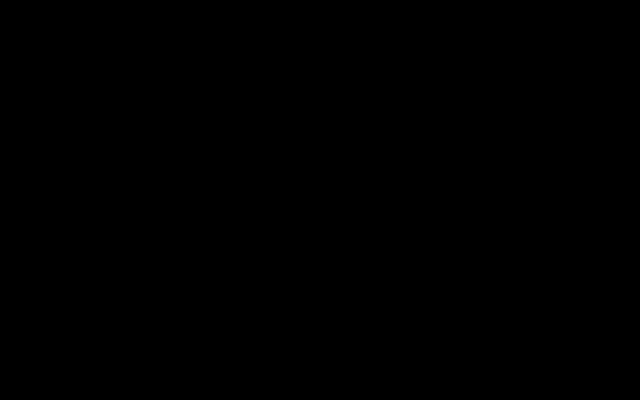
, , 4-5 6. , BASIC R :
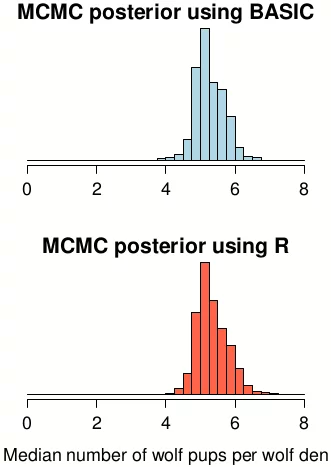
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
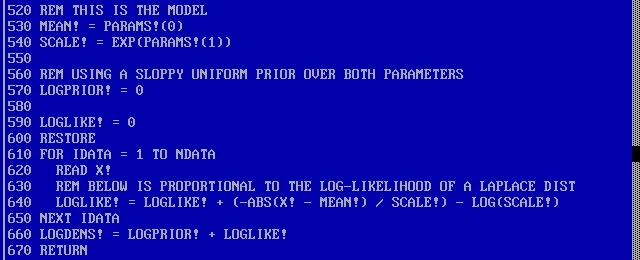
-. , GOSUB 520,
520. RND,
0 1.
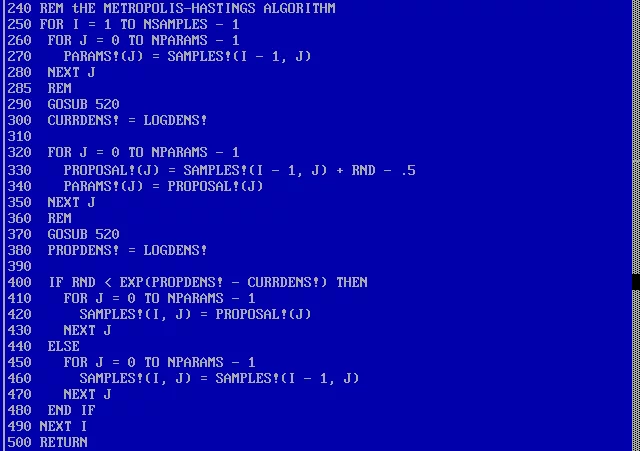
:

SAMPLES!
, 95 .
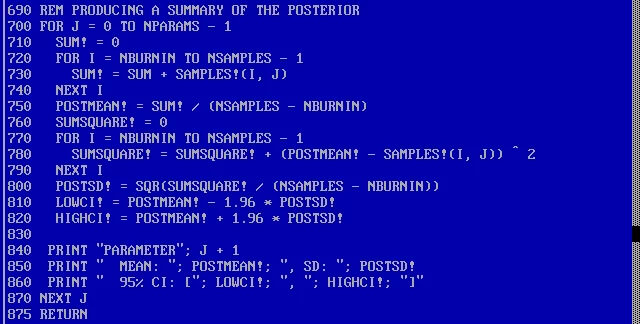
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
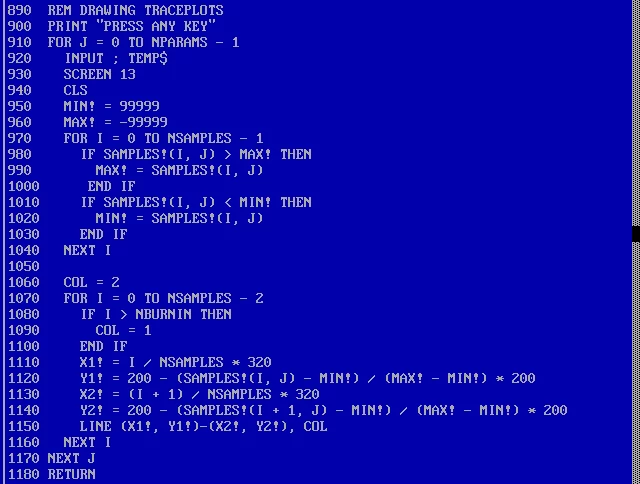
:
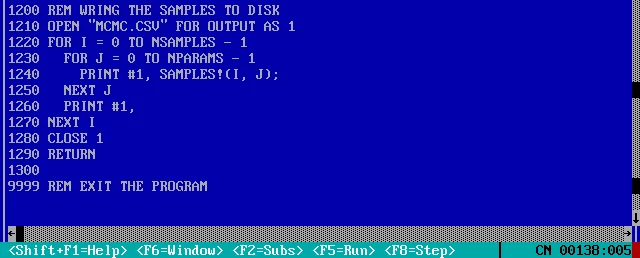
, .
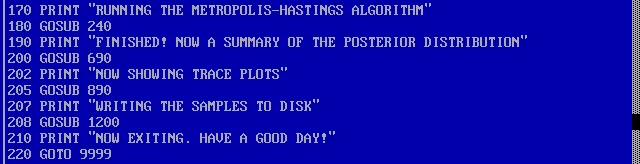
GOTO
, . 33 MHz, 386 ( ), , . :
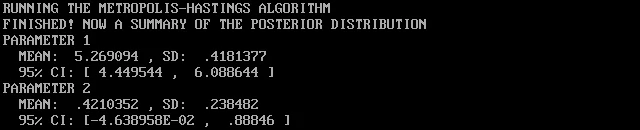
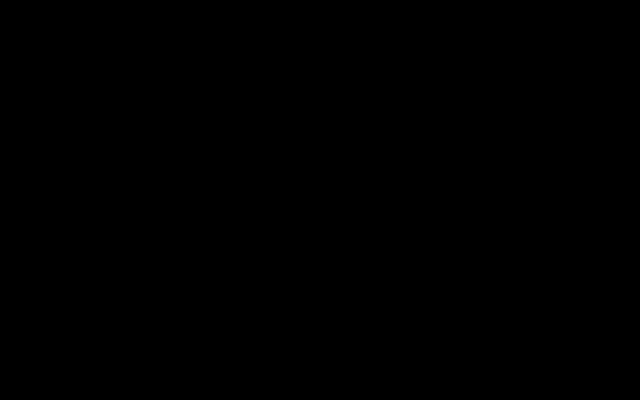
, , 4-5 6. , BASIC R :
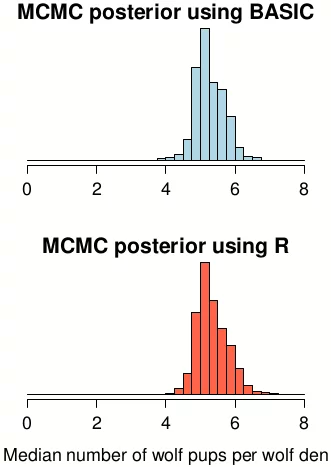
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
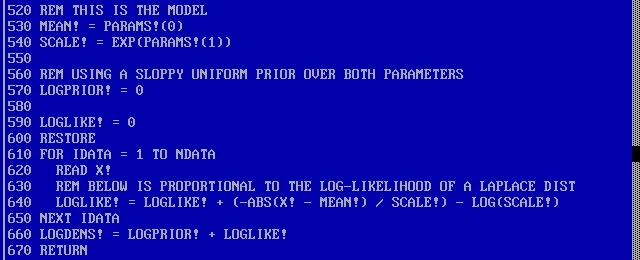
-. , GOSUB 520,
520. RND,
0 1.
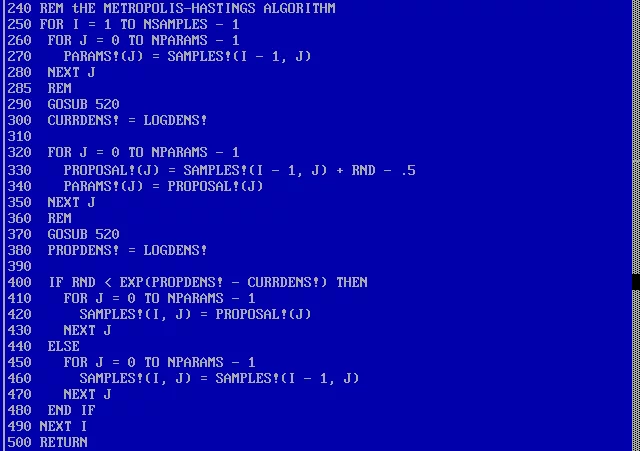
:

SAMPLES!
, 95 .
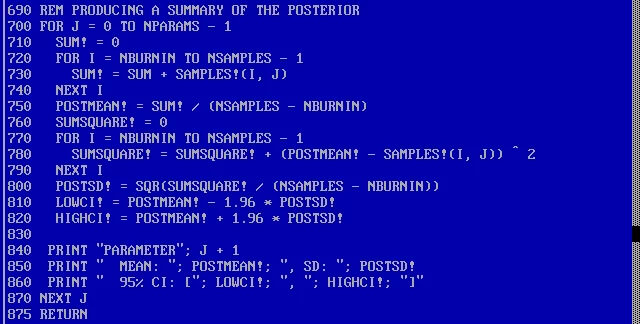
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
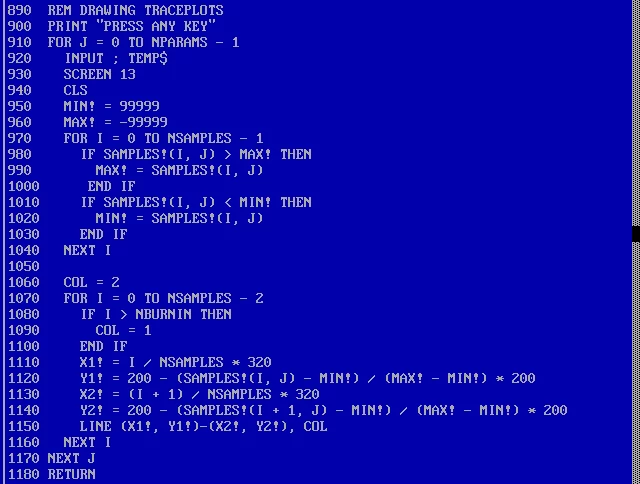
:
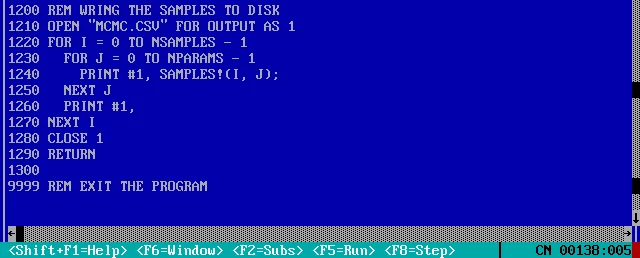
, .
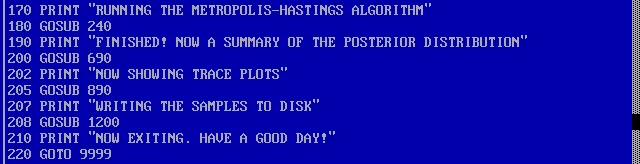
GOTO
, . 33 MHz, 386 ( ), , . :
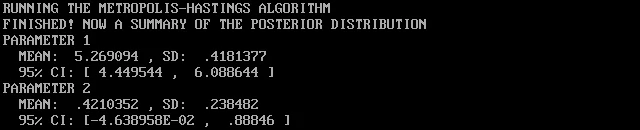
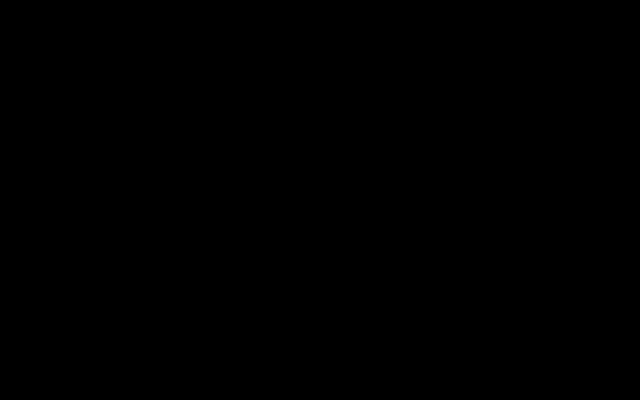
, , 4-5 6. , BASIC R :
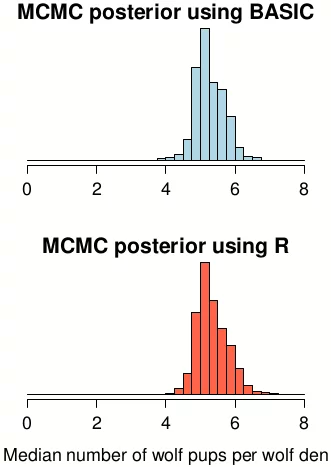
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
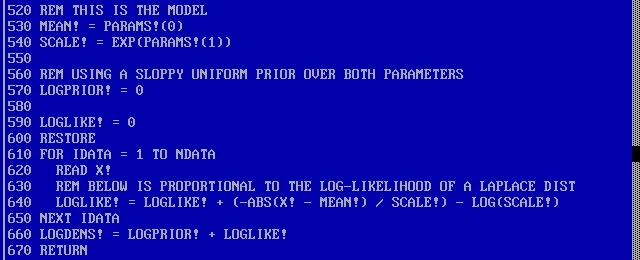
-. , GOSUB 520,
520. RND,
0 1.
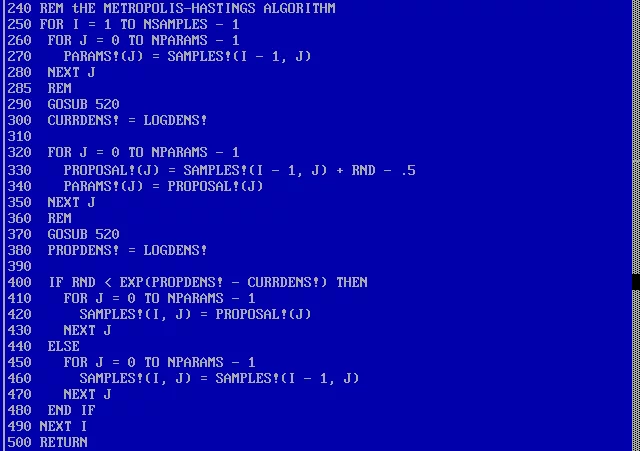
:

SAMPLES!
, 95 .
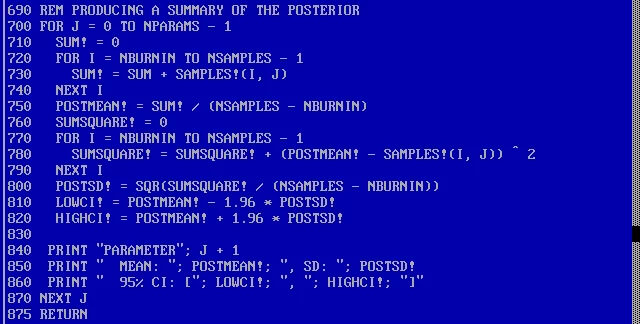
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
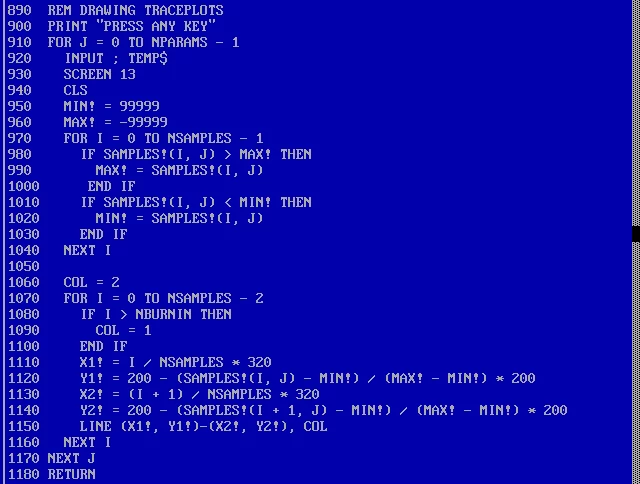
:
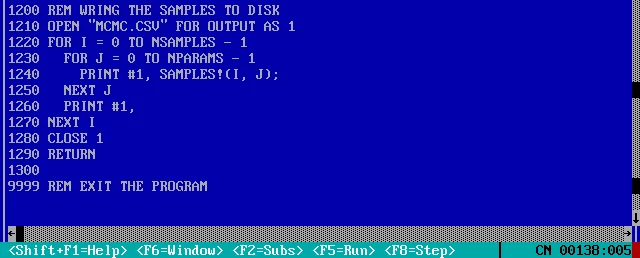
, .
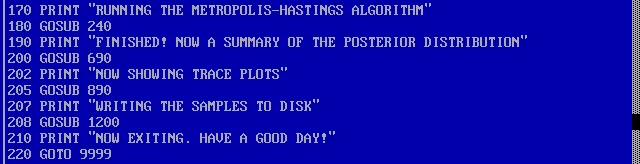
GOTO
, . 33 MHz, 386 ( ), , . :
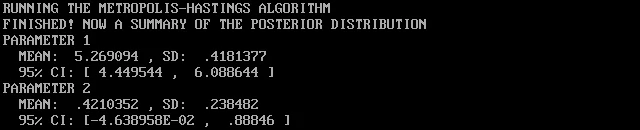
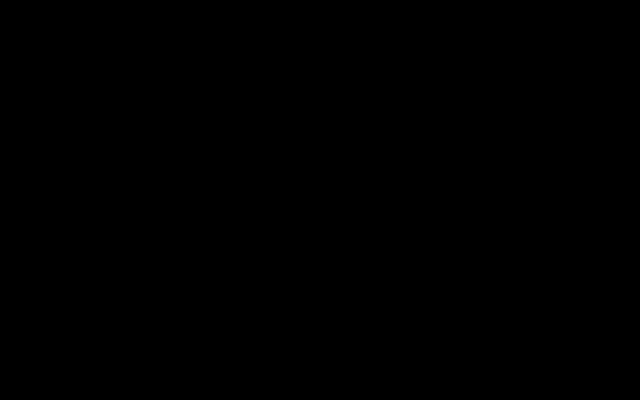
, , 4-5 6. , BASIC R :
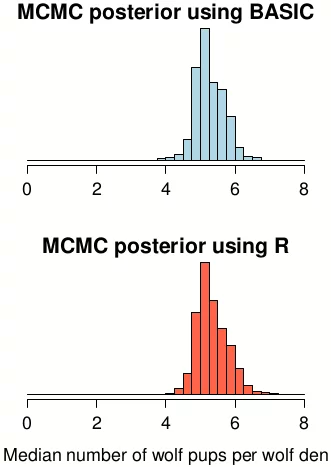
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
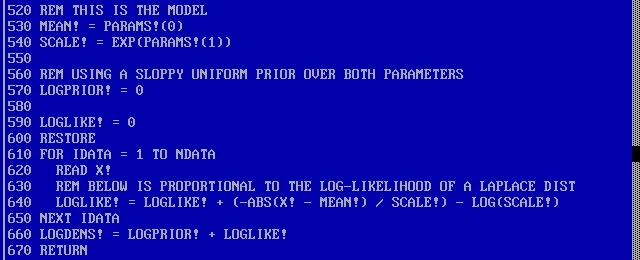
-. , GOSUB 520,
520. RND,
0 1.
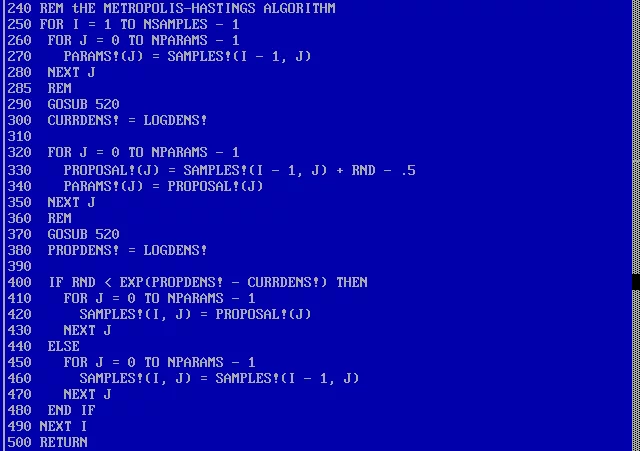
:

SAMPLES!
, 95 .
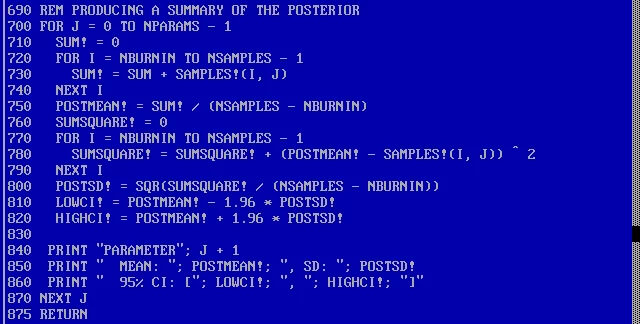
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
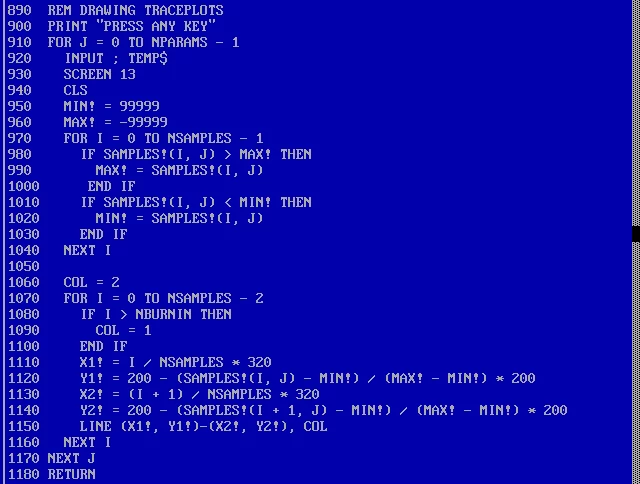
:
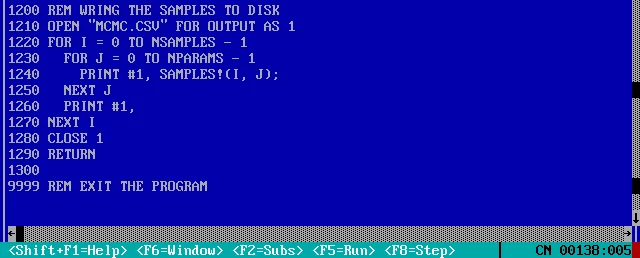
, .
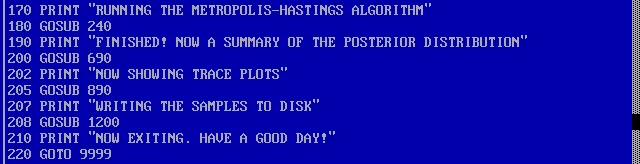
GOTO
, . 33 MHz, 386 ( ), , . :
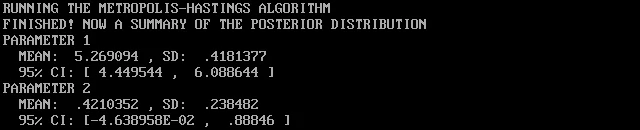
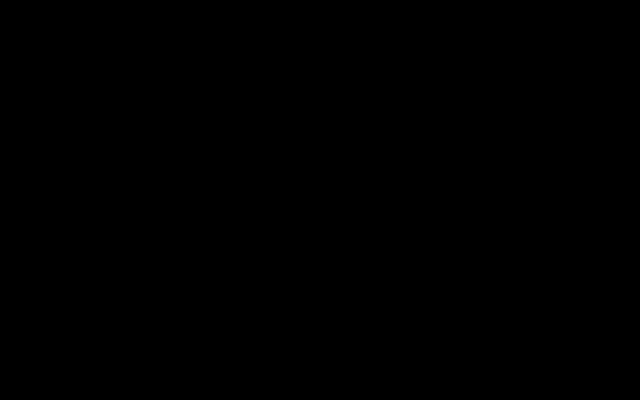
, , 4-5 6. , BASIC R :
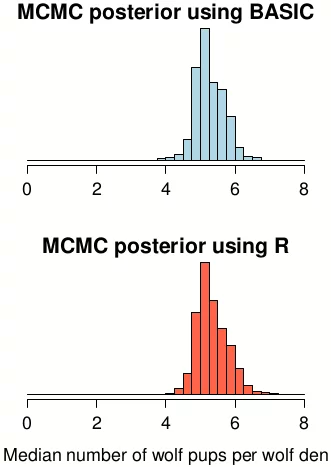
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
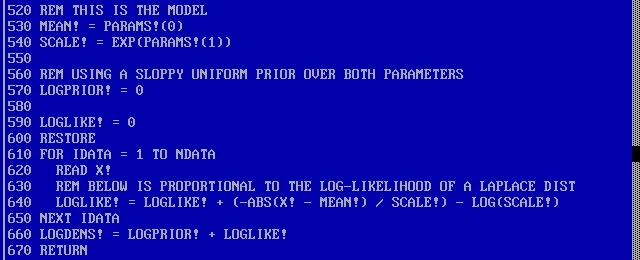
-. , GOSUB 520,
520. RND,
0 1.
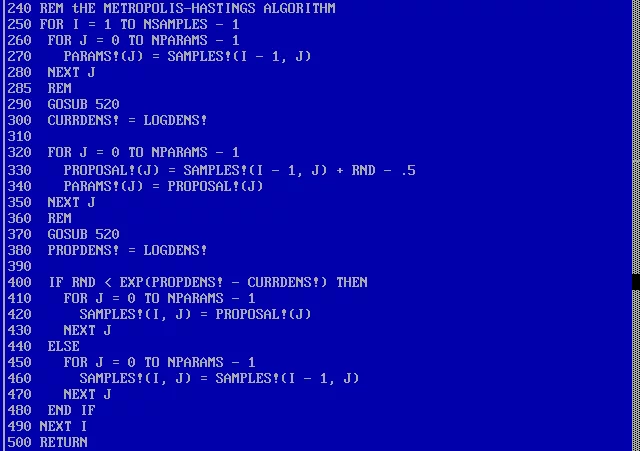
:

SAMPLES!
, 95 .
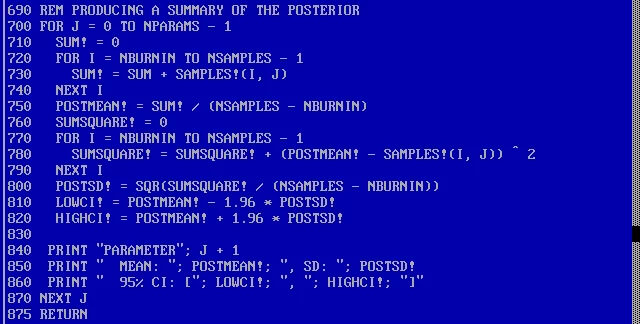
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
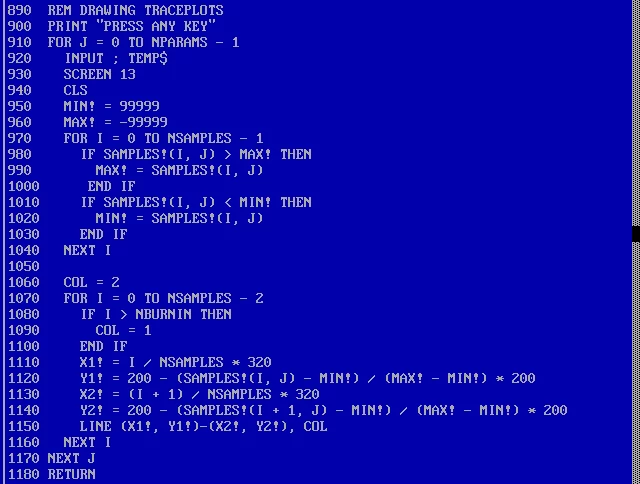
:
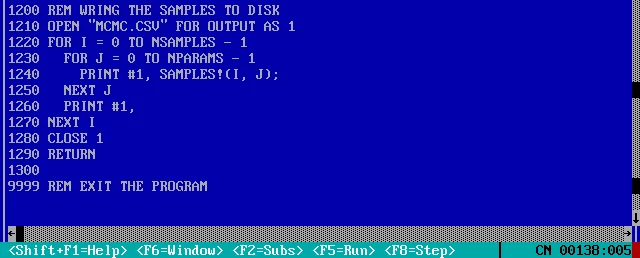
, .
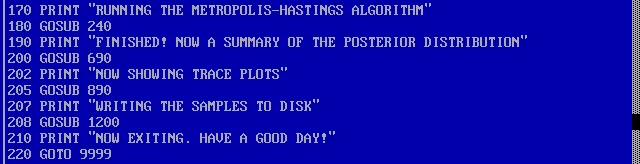
GOTO
, . 33 MHz, 386 ( ), , . :
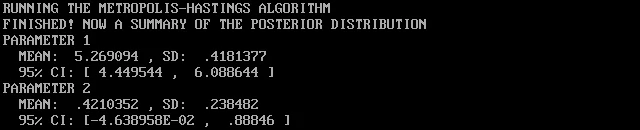
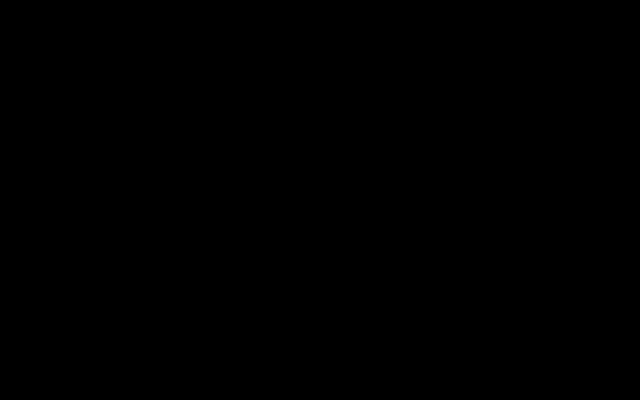
, , 4-5 6. , BASIC R :
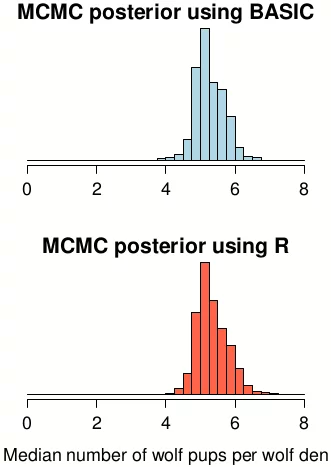
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
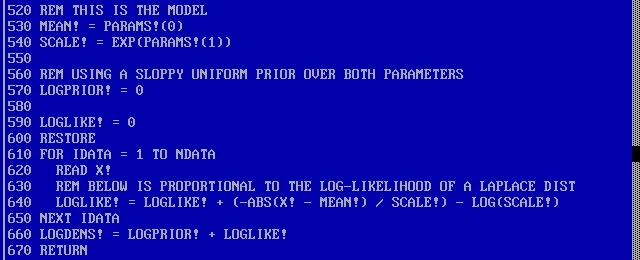
-. , GOSUB 520,
520. RND,
0 1.
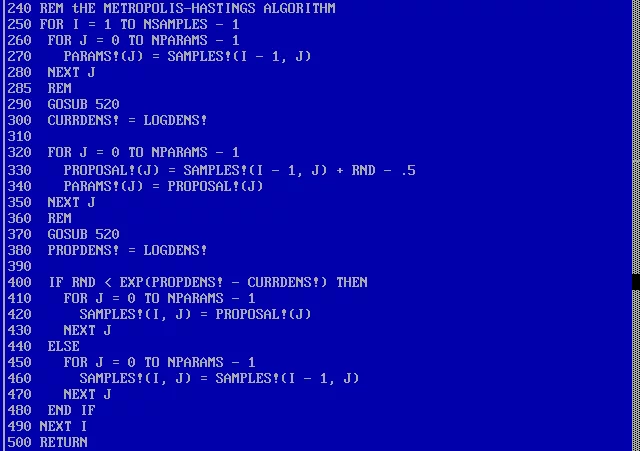
:

SAMPLES!
, 95 .
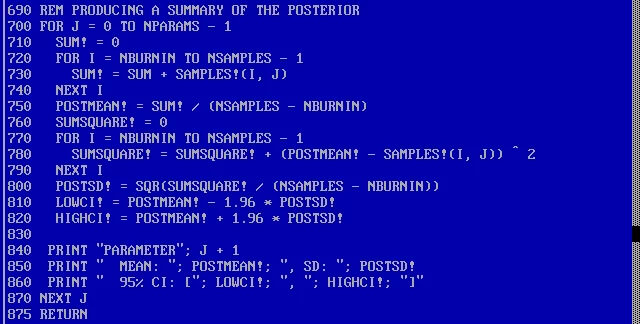
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
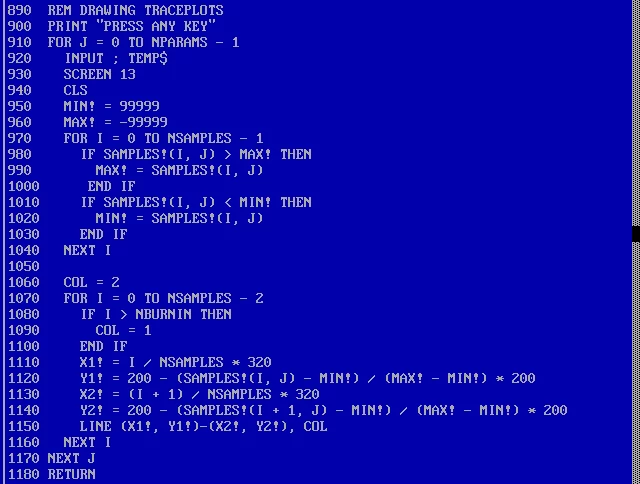
:
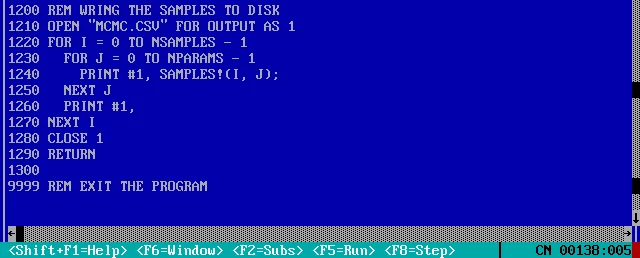
, .
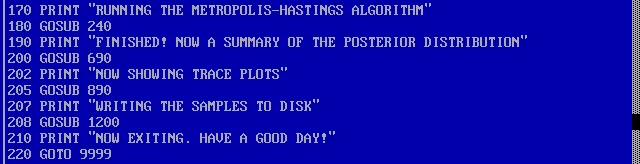
GOTO
, . 33 MHz, 386 ( ), , . :
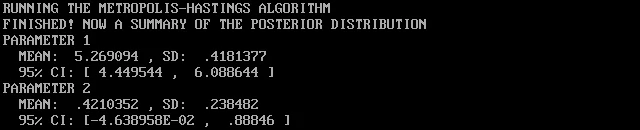
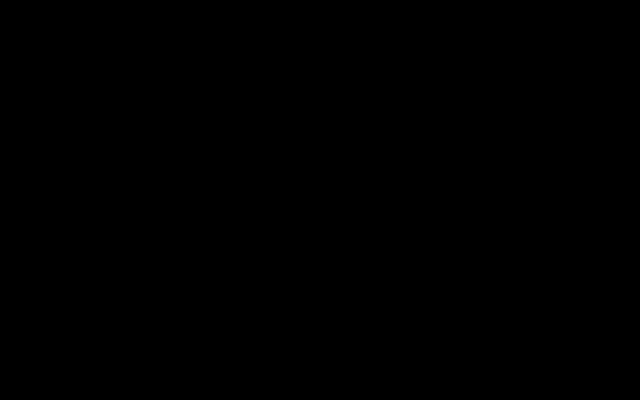
, , 4-5 6. , BASIC R :
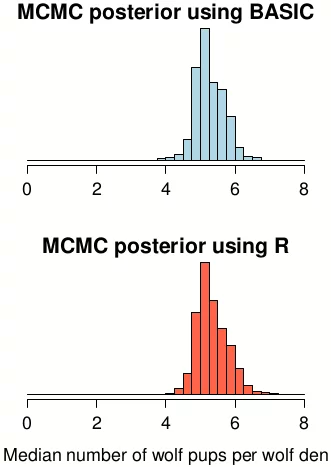
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
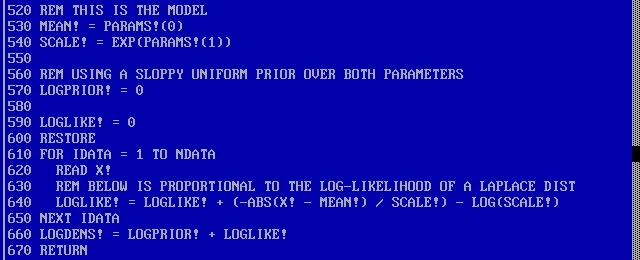
-. , GOSUB 520,
520. RND,
0 1.
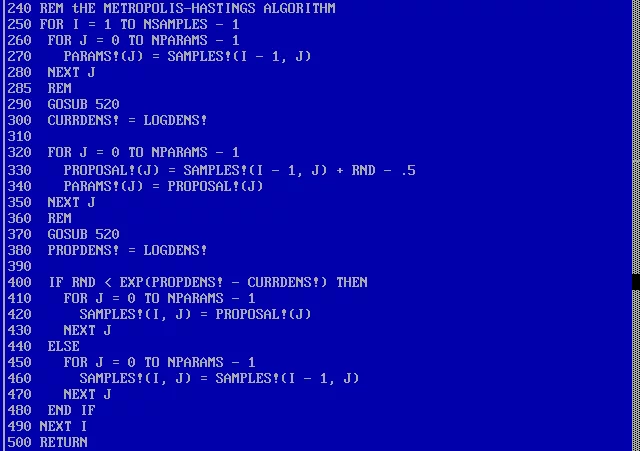
:

SAMPLES!
, 95 .
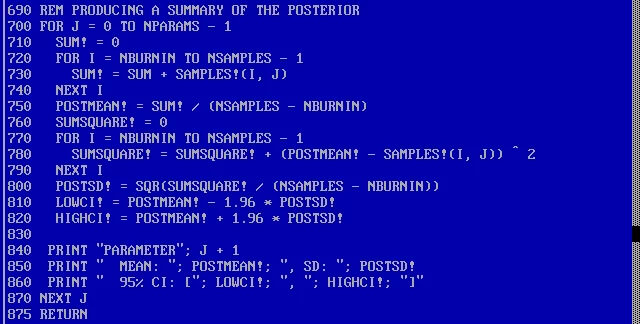
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
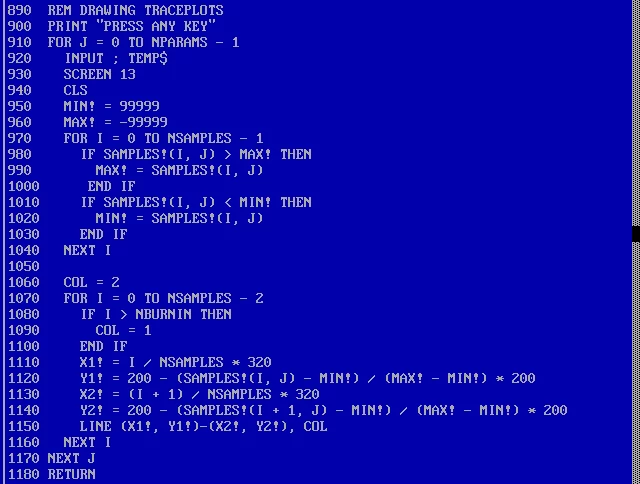
:
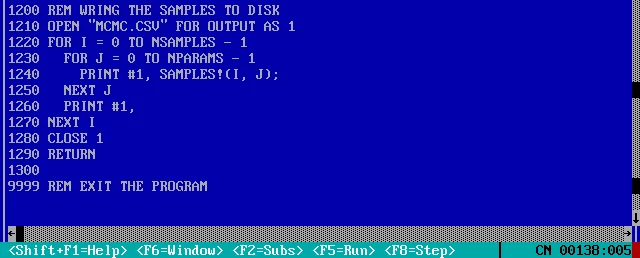
, .
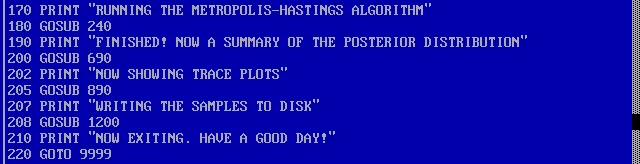
GOTO
, . 33 MHz, 386 ( ), , . :
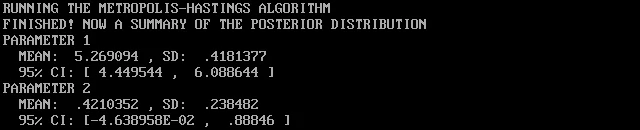
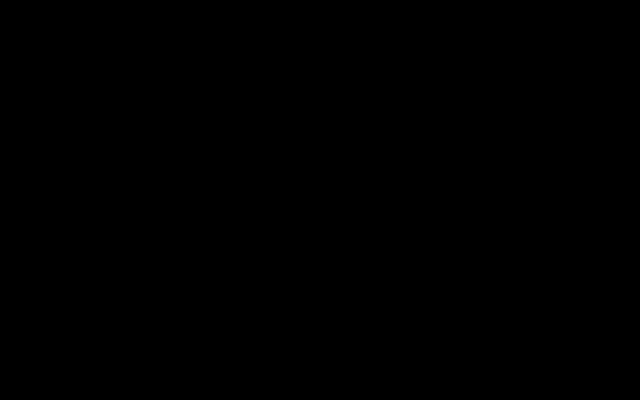
, , 4-5 6. , BASIC R :
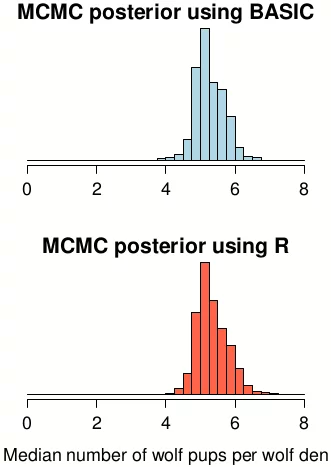
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
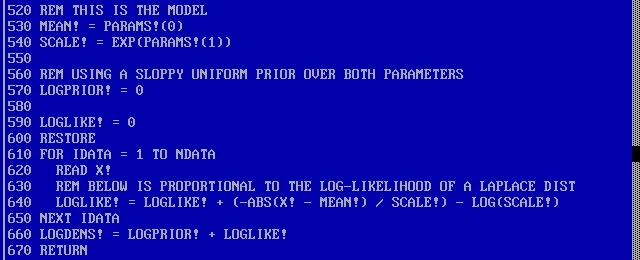
-. , GOSUB 520,
520. RND,
0 1.
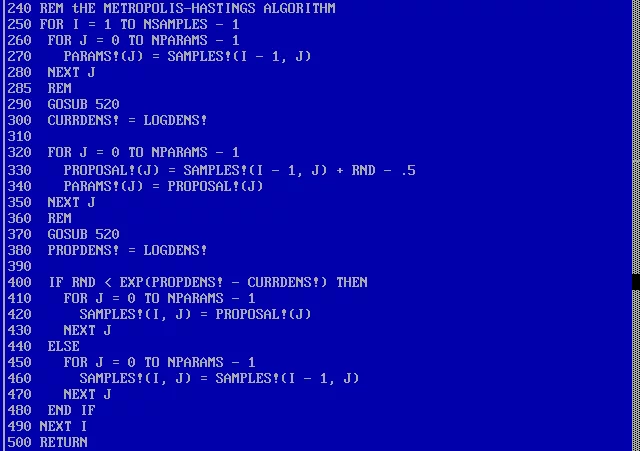
:

SAMPLES!
, 95 .
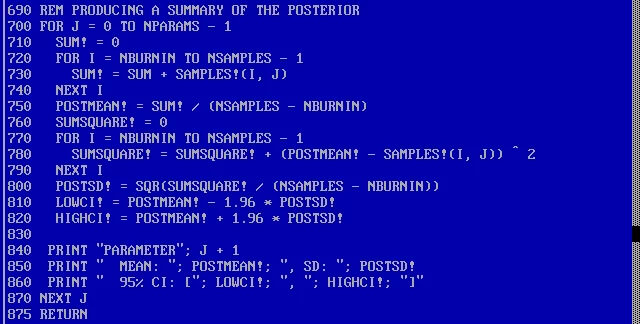
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
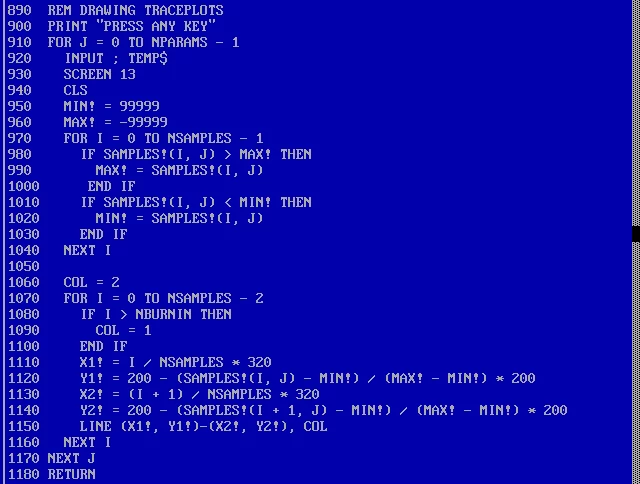
:
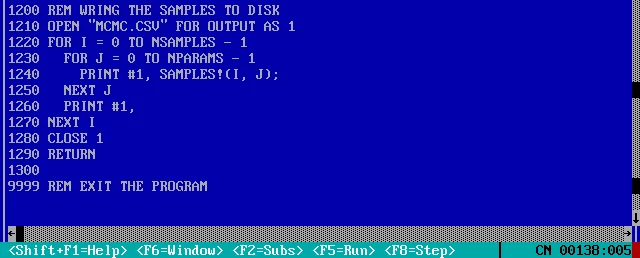
, .
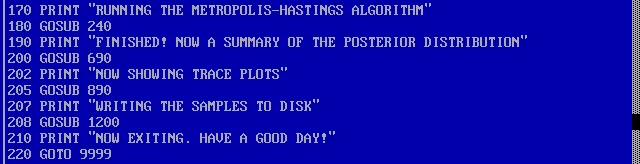
GOTO
, . 33 MHz, 386 ( ), , . :
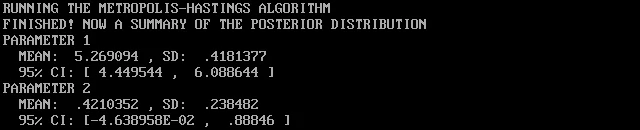
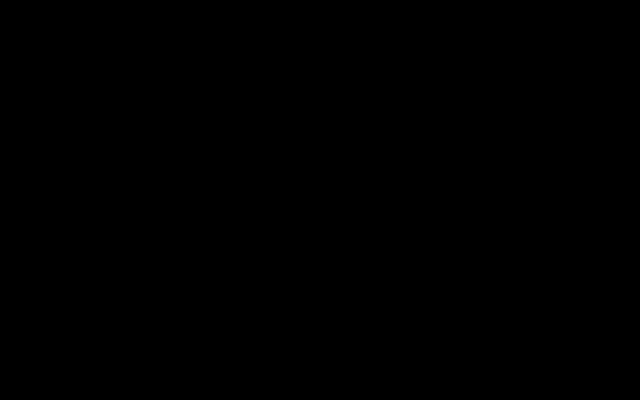
, , 4-5 6. , BASIC R :
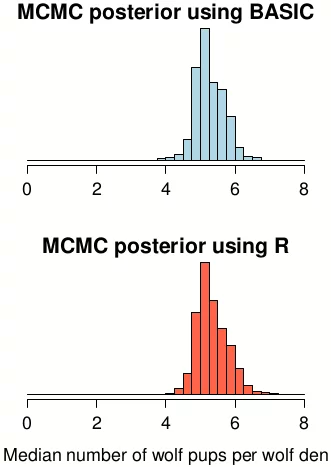
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
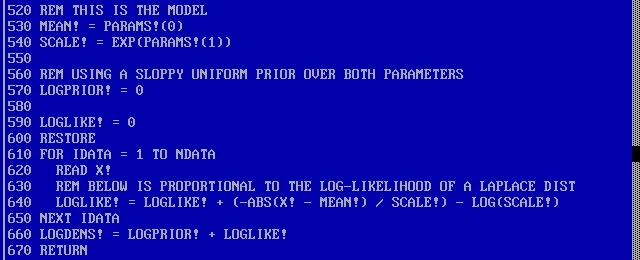
-. , GOSUB 520,
520. RND,
0 1.
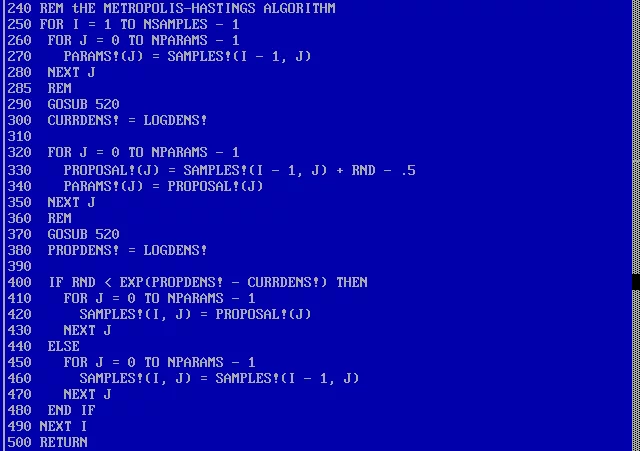
:

SAMPLES!
, 95 .
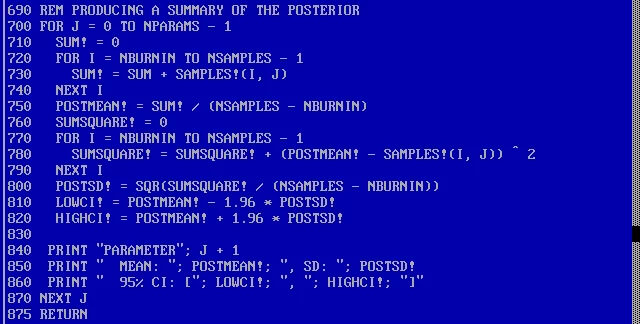
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
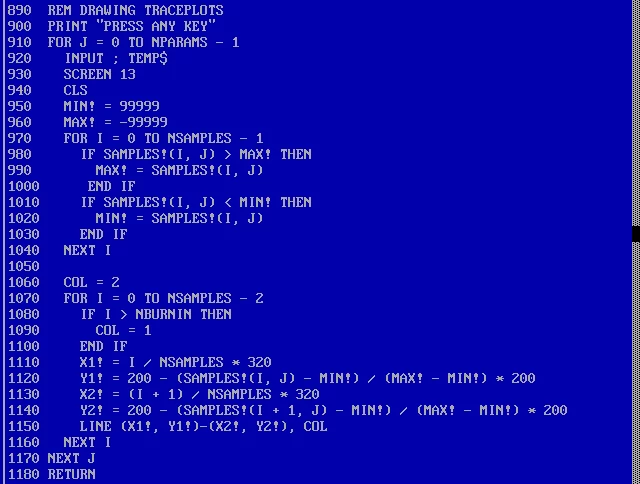
:
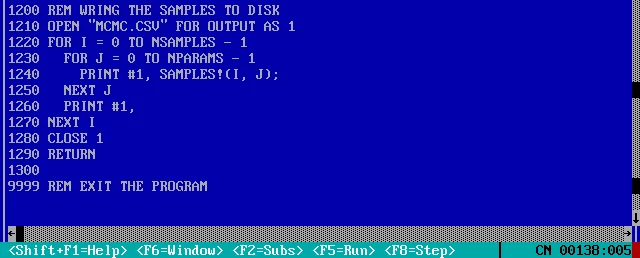
, .
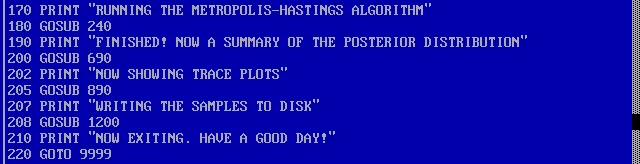
GOTO
, . 33 MHz, 386 ( ), , . :
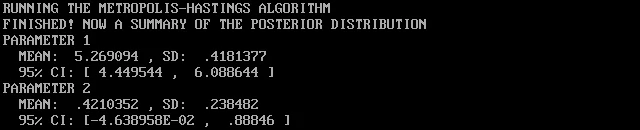
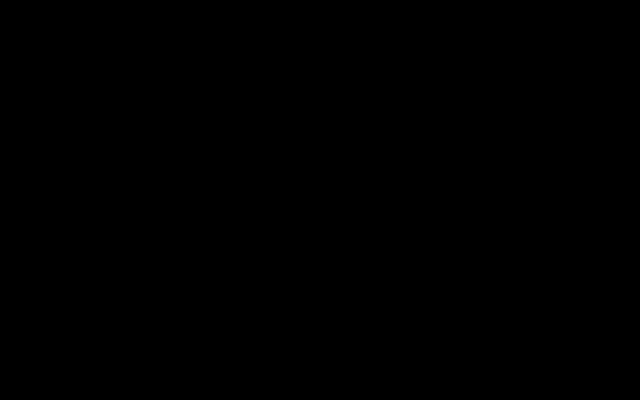
, , 4-5 6. , BASIC R :
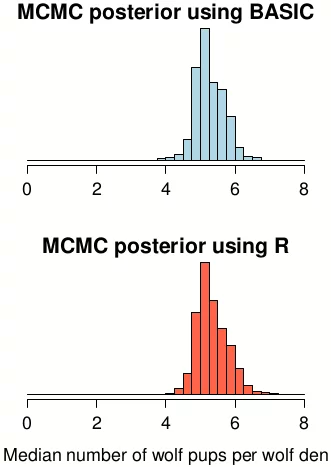
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
,
, RETURN
, GOSUB.
-, . , . , . , PARAMS!
LOGDENS!.
READ X!,
DATA
RESTORE
READ.
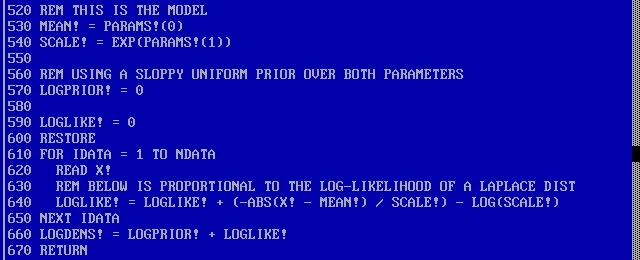
-. , GOSUB 520,
520. RND,
0 1.
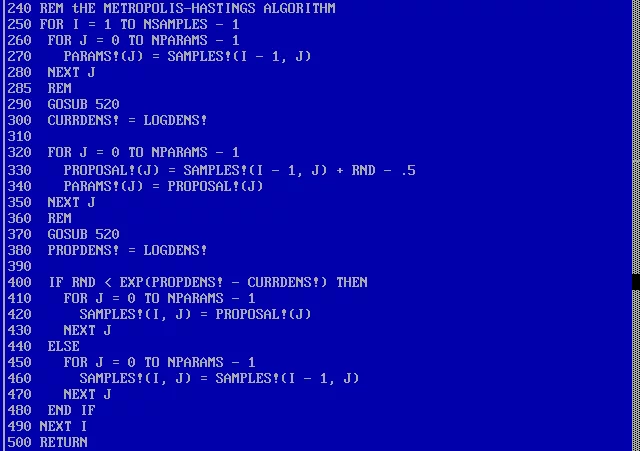
:

SAMPLES!
, 95 .
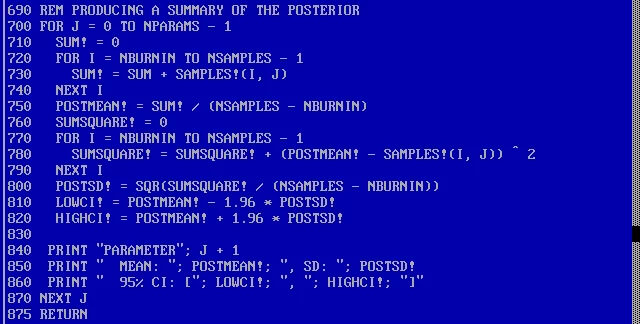
: PRINT "HELLO", "YOU!" HELLO YOU!, PRINT "HELLO"; "YOU!" HELLOYOU! "," ";" .
, , . , . , 320 x 200. : LINE (, )-(, ).
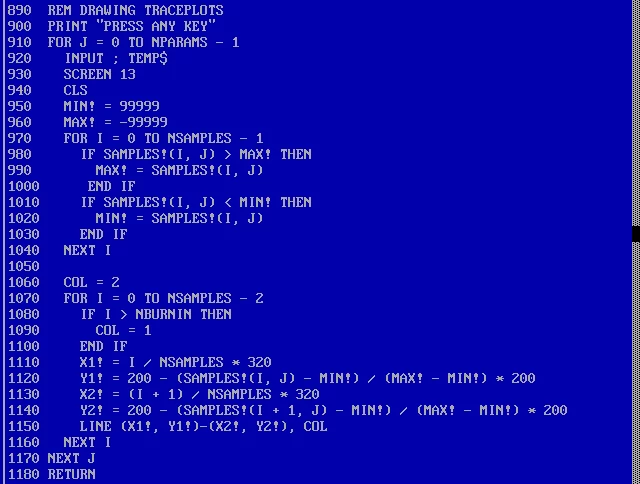
:
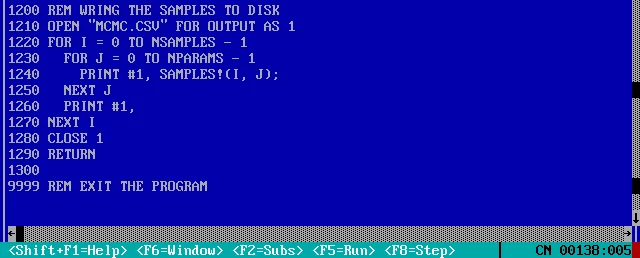
, .
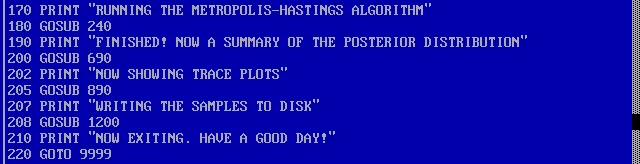
GOTO
, . 33 MHz, 386 ( ), , . :
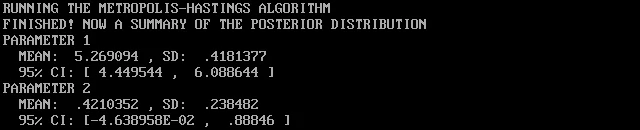
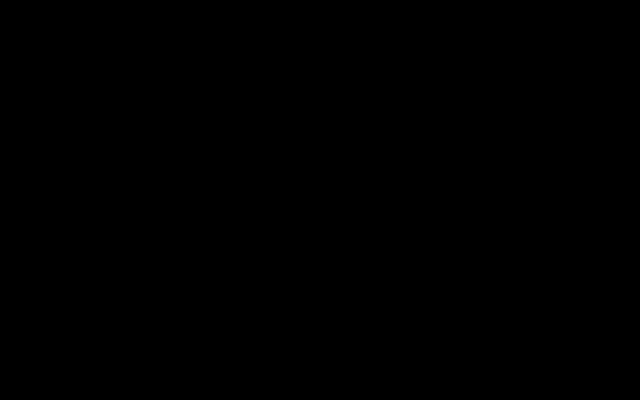
, , 4-5 6. , BASIC R :
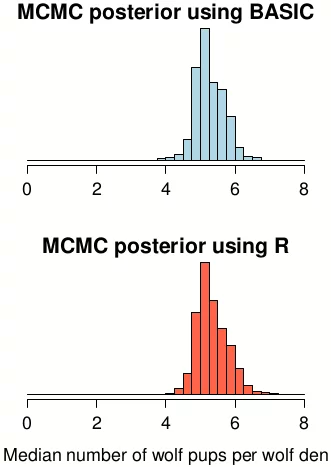
( MCMCDEMO.BAS ),
, 20 . GOTO
GOSUB
- . ( END IF
NEXT I.
) 386 . , .
Source: https://habr.com/ru/post/264587/
All Articles