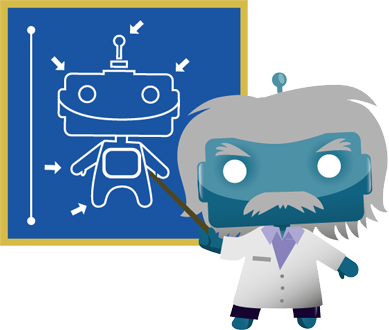
Recently I had to integrate in-game purchases into my game and the question arose, what kind of plug-in for this use under Unity3D? There are things like OpenIAB, Prime31, Unibill and Soomla. Smaller things will not be taken into account, because some of them either are not updated at all, or have some critical problems. Who wants to know why the choice fell on Soomla, and how to integrate it, please under the cat.
So, as you can see, there are a lot of plug-ins and by the name of the topic you can see which system I chose in the end, but why? Let's consider each system separately.
Openiab
Pros :
- Free
- Openness
- Large community
- Simplicity
- Good documentation
- Support for iOS and Android (Later it turned out that it was not)
Cons :
- Almost no information about working with iOS
- Updates are very rare (Last was 4 months ago)
Opinion :
I sin, I previously worked with this system, but only under Android, but when I decided to try to work with the iOS side, I was disappointed, OpenIAB simply did not work, because its events did not respond, and how to initialize the store, because this system had to be abandoned, but it should be given its due, it worked flawlessly for Android, but there was no doubt about this, because even the original c ++ version was written only this OS.
')
Unibill
Pros :
- Multiple store support
- Excellent documentation
- Simplicity and ease of learning
Cons :
- Price ($ 175)
- The community as such is almost absent
Opinion :
With Unibill, everything turned out to be easy. Immediately there were questions about the price, $ 175 is not really small money, especially considering that there are cheaper analogues, so there is no right away, but if there is finance and you don’t want to spend a lot of time, then this is a great option.
Prime [31]
Pros :
- Excellent documentation
- Simplicity and ease of learning
- Supports many shops, but with a reservation.
- Big community.
- Under each platform, the price of the plug is $ 70.
Opinion :
Plug-ins from prime [31] are famous for their quality, but the price also leaves much to be desired, $ 70 for each platform is not at all the ultimate dream, but there is already a proven technology with a huge community and excellent documentation, and so on. I needed a plug-in just under 2 platforms, the price went lower than that of Unibill, but still this option was chosen, not the following.
Soomla
Pros :
- Excellent documentation
- Simplicity and ease of learning
- Supports major mobile platforms.
- Big community.
- Free
Cons :
- Work through their personal account
Opinion :
Soomla also seemed just an excellent option, because it has all the advantages of prime plugins [31], but at the same time this system is completely free, although it was embarrassing to have to work through the personal account on their website, but in fact, there is almost nothing to do there, because the choice I stopped at this option.
Project Integration
The first thing to do (Suddenly!) Is to download the plugin itself, from the Asset Store, from the official site, there is no difference. Both there and there the plug-in is updated almost simultaneously.
After importing a plug-in into the project, the first thing to do is to set the settings in the editor.

In Soomla Secret, you must specify the Game Key, which you will receive when creating the application in your Soomla account.
Without this, nothing will work!
Debug Native and Unity Debug is your personal choice. In Android Settings, the package name is automatically determined, and then you must actually choose which Android stores you use. I think the API Key is clear where to get. Receipt Validation is also your choice. That's all, the basis we set up, it's time to go to the scripts.
We need to actually describe our products in a separate class, so that we can buy them, for example:
Initialization of purchasesusing Soomla.Store;// Soomla using Soomla;// Soomla public class dataIAP : IStoreAssets { // IStoreAssets public int GetVersion() { // GetVersion, , return 0; } public VirtualCurrency[] GetCurrencies() { // , return new VirtualCurrency[]{ITEM_OBJECT}; } public VirtualCurrencyPack[] GetCurrencyPacks(){ // return new VirtualCurrencyPack[]{PACK_1_OBJECT, PACK_2_OBJECT}; } public VirtualGood[] GetGoods() { return new VirtualGood[0]; // } public VirtualCategory[] GetCategories() { return new VirtualCategory[0]; // } public const string ITEM_ID = "ITEM_ID";// ID public const string PACK_1 = "ID_PACK_1";// id, (App Store, Google Play) public const string PACK_2= "ID_PACK_2";// id, (App Store, Google Play) public static VirtualCurrency ITEM_OBJECT = new VirtualCurrency(// "ITEM_ID ",// "ITEM_COIN", // ITEM_ID //id ); public static VirtualCurrencyPack PACK_1_OBJECT = new VirtualCurrencyPack(// "PACK_1",// "",// PACK_1 ,// id 100, // ITEM_ID ,//id new PurchaseWithMarket(PACK_1 , 1.99) // id , ( , ) ); public static VirtualCurrencyPack PACK_2_OBJECT = new VirtualCurrencyPack(// "PACK_2", "", PACK_2, 200, ITEM_ID , new PurchaseWithMarket(PACK_2, 5.99) ); }
Thanks to the comments, more or less everything is clear, but I missed 2 things that are virtual 'benefits' and categories, they are basically needed by those who want to track the sale of even simple virtual purchases, not for real money, but even for the currency you sell for real money through the personal cabinet sumla, which we will talk about later.
So, we have registered all our in-game purchases, but how now to transfer this data to the plugin and how to make purchases?
And this is quite simple:
Soomla Initialization using Soomla; using Soomla.Store; public class Test { public Test () { SoomlaStore.Initialize (new dataIAP ());// #if UNITY_ANDROID SoomlaStore.StartIabServiceInBg (); // Android, #endif } public void PayPack_1() { SoomlaStore.BuyMarketItem (StoreInfo.CurrencyPacks [0].ItemId, "");// } public void PayPack_2() { SoomlaStore.BuyMarketItem (StoreInfo.CurrencyPacks [1].ItemId, "");// } public void Quit() { SoomlaStore.StopIabServiceInBg (); } }
Very simple initialization, but we are also interested in the events that are available to us, and here they are (Basic):
Developments using System; using System.Collections.Generic; using Soomla; using Soomla.Store; public class ExampleEventHandler { // public ExampleEventHandler () { //, StoreEvents.OnMarketPurchase += onMarketPurchase; //, StoreEvents.OnItemPurchased += onItemPurchased; //, StoreEvents.OnBillingSupported += onBillingSupported; //, StoreEvents.OnBillingNotSupported += onBillingNotSupported; //, StoreEvents.OnMarketPurchaseStarted += onMarketPurchaseStarted; //, StoreEvents.OnItemPurchaseStarted += onItemPurchaseStarted; //, StoreEvents.OnMarketPurchaseCancelled += onMarketPurchaseCancelled; //, StoreEvents.OnSoomlaStoreInitialized += onSoomlaStoreInitialized; //, Soomla StoreEvents.OnUnexpectedStoreError += onUnexpectedStoreError; // #if UNITY_ANDROID && !UNITY_EDITOR StoreEvents.OnIabServiceStarted += onIabServiceStarted; StoreEvents.OnIabServiceStopped += onIabServiceStopped; #endif } public void onUnexpectedStoreError(int errorCode) { SoomlaUtils.LogError ("ExampleEventHandler", "error with code: " + errorCode); } public void onMarketPurchase(PurchasableVirtualItem pvi, string payload, Dictionary<string, string> extra) { } public void onItemPurchased(PurchasableVirtualItem pvi, string payload) { } public void onBillingSupported() { } public void onBillingNotSupported() { } public void onMarketPurchaseStarted(PurchasableVirtualItem pvi) { } public void onItemPurchaseStarted(PurchasableVirtualItem pvi) { } public void onMarketPurchaseCancelled(PurchasableVirtualItem pvi) { } public void onCurrencyBalanceChanged(VirtualCurrency virtualCurrency, int balance, int amountAdded) { } public void onSoomlaStoreInitialized() { } #if UNITY_ANDROID && !UNITY_EDITOR public void onIabServiceStarted() { } public void onIabServiceStopped() { } #endif }
That's it, the system is fully operational.
But now a little about the personal account of Soomla. There you can track all transactions of your users, both with real money and with in-game currency, in order to need goods and categories, so that it is easier to keep track of everything, but to work with this thing you need to work with Soomla Highway. But this article is not about that.
And a few words about testing. Testing purchases on android is easy, you have filled the build, for example, for a beta test and test it for health, but on ios you must create an account for the sandbox:
