Suppose we want to add the ability to save data to the classic todo application.
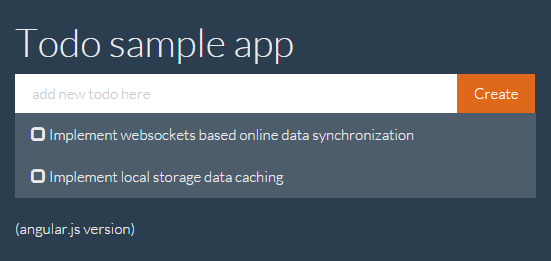
We will need to read the data from the repository and sort it to show the list of tasks, save the data when adding a new task, and delete the data.
To solve this problem, we can use the new databoom.angular.js library to work with the
databoom.space repository from the
Angular.js applications.
')
Example and application code in the sandbox.Service announcement
The first thing we do is register a service. Angulyar offers several ways to make a common code using services. Read more about them
here .
The most simple type is used for our task: factory
angular.module('todoApp', []) .factory('db', databoomSrv('https://samples.databoom.space', 'sandboxdb')) .controller('TodoListController', function (db) { ... });
Reading and sorting the task list
function updateList() { db.load('tasklist', { orderby: "date desc" }) .then(function (data) { todoList.taskList = data }) }
The db.load function ('tasklist', {orderby: “date desc”}) sends the server a request to read the task list (all objects in the 'tasklist' collection). The request is also accompanied by the requirement to sort the data by date in the reverse order. There are also options for filtering data, paginal output, disclosure, etc.
Adding a new task to the database
todoList.addTodo = function () { if (!todoList.todoText)
The db.save ('tasklist', {text: todoList.todoText, done: false, date: new Date ()} function) saves a new task (adds it to the 'tasklist' collection).
Delete task
todoList.delTodo = function (id) { db.del(id).then(updateList) }
The db.del (id) function deletes an object with the specified id.
To save and read data you need only a few lines of code on the client application and not a single action with the server. The databoom server allows you to save data as it is - without first describing the data schema and administering the server.
More API library can be found
here .
Library development
The next version of the library will be able to:
- caching data in the local storage in case of loss of communication with the subsequent synchronization with the server.
- constant online data synchronization between clients (using websockets) - for example, when you add a new task, it immediately becomes visible to everyone.