
Introduction
Good afternoon, dear readers! Most recently, I completed the development of one of my applications on Qt, and I wanted to create a professional installation program so that everything was “as in adults”. As it turned out, this is not easy, given that there is almost no information on the deployment toolkit's official website. This article describes some of the steps involved in preparing programs for Qt version 5.2 or higher for distribution to computers of other users. So, here's a guide plan:
- Preparing a Qt project for deployment
- Layout of the distribution package of the program
- Code signing and installer creation
Let's not waste time and get down to work.
1. Preparing a Qt project for deployment
To make it easier to follow the instructions, create a simple Qt Widgets project. All subsequent operations will relate to this project. The following is the contents of the application source files:
HelloWorld.proQT += core gui widgets TARGET = HelloWorld TEMPLATE = app SOURCES += main.cpp
main.cpp #include <QApplication> #include <QLabel> int main(int argc, char *argv[]) { QApplication a(argc, argv); QLabel label("Hello, world!"); label.setAlignment(Qt::AlignCenter); label.resize(200, 50); label.show(); return a.exec(); }
Programs look especially high-quality and professional if they carry with them metadata about the developer, software version, copyright, language, and much more. For example, let’s refer to the properties of the Photoshop.exe file by the well-known Adobe Photoshop system. The figure below shows the properties of this file:
')

You can add this information using
the resource file . The resource file has the
.rc extension and carries the text code describing the resources used in the application. Such scripts are used in Visual Studio projects based on WinAPI, and contain various descriptors for icons, strings, identifiers, and more. In Qt projects, all this makes little sense, but the inclusion of general information about the program is still necessary. Below are the source code of the resource file and the contents of the project file, which will also need to be changed:
resources.rc IDI_ICON1 ICON "icon.ico" #include <windows.h> #define VER_FILEVERSION 1,0,0,0 #define VER_FILEVERSION_STR "1.0.0.0\0" #define VER_PRODUCTVERSION 1,0,0 #define VER_PRODUCTVERSION_STR "1.0.0\0" #define VER_FILEDESCRIPTION_STR "HelloWorld" #define VER_INTERNALNAME_STR "Sensor" #define VER_LEGALCOPYRIGHT_STR "Copyright (C) 2015, MyCompany" #define VER_ORIGINALFILENAME_STR "HelloWorld.exe" #define VER_PRODUCTNAME_STR "Hello World" VS_VERSION_INFO VERSIONINFO FILEVERSION VER_FILEVERSION PRODUCTVERSION VER_PRODUCTVERSION BEGIN BLOCK "StringFileInfo" BEGIN BLOCK "040904E4" BEGIN VALUE "FileDescription", VER_FILEDESCRIPTION_STR VALUE "FileVersion", VER_FILEVERSION_STR VALUE "InternalName", VER_INTERNALNAME_STR VALUE "LegalCopyright", VER_LEGALCOPYRIGHT_STR VALUE "OriginalFilename", VER_ORIGINALFILENAME_STR VALUE "ProductName", VER_PRODUCTNAME_STR VALUE "ProductVersion", VER_PRODUCTVERSION_STR END END BLOCK "VarFileInfo" BEGIN VALUE "Translation", 0x409, 1252 END END
HelloWorld.pro QT += core gui widgets TARGET = HelloWorld TEMPLATE = app SOURCES += main.cpp RC_FILE = resources.rc
In this example, it is important not to forget to add the
resources.rc and
icon.ico files to the folder with the project source files. The figure below shows the program properties window after assembly:

Sometimes it is necessary that the program runs as an administrator. Within Qt, this can be accomplished by using simple instructions in a project file. Below is the code that allows the program to request administrator rights at startup:
HelloWorld.pro QT += core gui widgets TARGET = HelloWorld TEMPLATE = app SOURCES += main.cpp RC_FILE = resources.rc win32 { CONFIG += embed_manifest_exe QMAKE_LFLAGS_WINDOWS += /MANIFESTUAC:"level='requireAdministrator'" }
It should be noted that all the above instructions will be guaranteed to work only when using the Visual Studio build kit. Detailed information on resource files can be found on the MSDN portal in the
“About Resource Files” section .
2. Layout of the distribution kit of the program
Creating an application distribution kit, taking into account all its files that should be installed on users' computers, is probably the most difficult step of the deployment. It is required to carefully analyze the executable file of the program for dependencies, take care of the translation files, do not forget about the application resources. The
windeployqt.exe utility that comes with the build kit will help solve some of these problems. This tool works on the command line and supports some configuration options. The figure below shows the command line window with the utility running:

The last parameter must specify the path to the binary files of the assembled application or the names of these files. The table below lists the utility parameters that can be used when working with it:
Parameter | Description |
- ?, -h, --help | Help output |
-v, --version | Display version information |
--dir < directory > | Use specified directory instead of file directory |
--libdir < path > | Directory to which libraries will be copied |
--debug | Use debug versions of files |
--release | Use files for release |
--release-with-debug-info | Use files for debug release. |
--force | Replace existing files |
--dry-run | To carry out work in order to verify |
--no-plugins | Skip copying plugins |
--no-libraries | Skip copying libraries |
--qmldir < directory > | Scan import QML, starting from the specified directory |
--no-quick-import | Skip Qt Quick |
--no-translations | Skip copying translation files |
--no-system-d3d-compiler | Skip copying Direct3D compiler |
- compiler-runtime | Copy compiler dependencies |
--no-compiler-runtime | Skip compiler dependencies |
--webkit2 | Copy WebKit2 files |
--no-webkit2 | Skip WebKit2 |
--json | Print output in JSON format |
--angle | Copy ANGLE files |
--no-angle | Skip ANGLE |
--list < mode > | Print only the names of the copied files. Modes: source , target , relative , mapping
|
--verbose < level > | Debug level |
- < library name > | Add the specified library |
--no- < library name > | Do not add the specified library |
After running the utility, various libraries and service files should appear near the executable file of the program, which will allow the application to start and work correctly on many computers. The figure below shows the Windows Explorer window with the distribution structure:
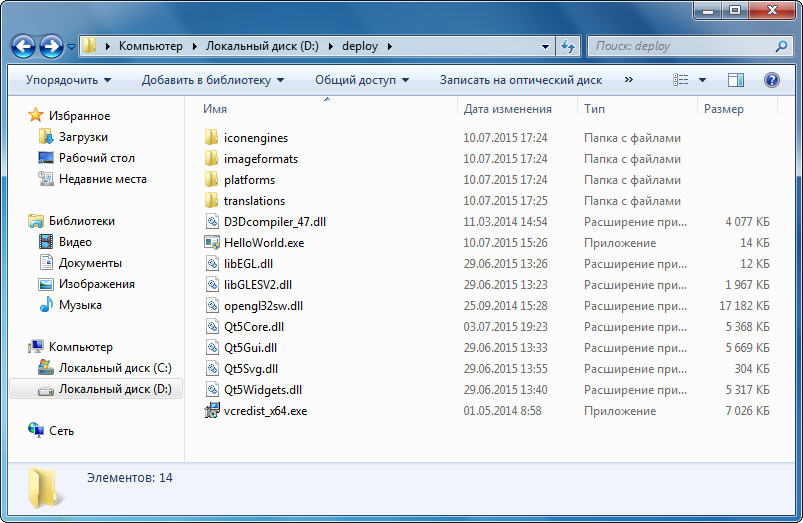
It should be noted that before using
windeployqt.exe, you must add the path to this file to the PATH environment variable, otherwise this tool will not work.
3. Code signing and installer creation
After compiling the distribution package of the program, we will try to launch the application on behalf of the administrator. The figure below shows the message of the
User Account Control System Service
(UAC) with a warning about the launch of an application of an unknown publisher:

This warning discourages users and creates a bad reputation for the application. To remedy the situation, you need to sign the program files with a special certificate. Developers, signing their programs, provide additional guarantees for the reliability of applications from the point of view of information security. Open source software developers can get certificates for their projects for free, for example, on the
Certum website. To use the certificate, you need a special program that will sign the application files. To do this, you can use the handy tool
DigiCert Certificate Utility . The figure below shows the window of this program with a list of certificates for signing the code:
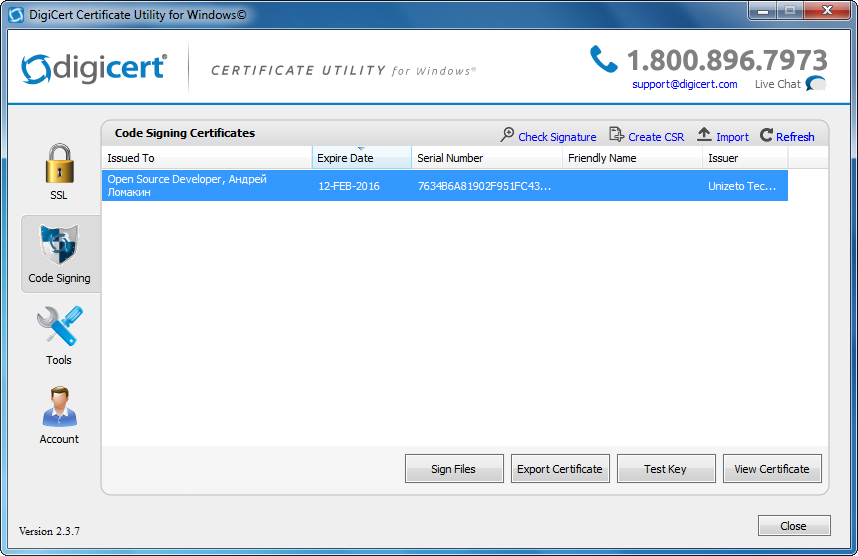
After using this utility, it is worthwhile to try again to run the application as an administrator. Below is a UAC message with information about the publisher of the program:

After the work done, it's time to think about the choice of the installer creation system for the prepared application. There are both paid and free products for this task. The table below lists some tools for creating installation programs:
Paid | Free |
InstallShield | Qt Installer Framework |
Setup Factory | NSIS |
Setupbuilder | Inno setup |
Smart Install Maker | WiX |
It is difficult to make any recommendations about the choice of a particular system. Open source developers are likely to choose free tools, while paid products are often used by commercial companies.
Conclusion
In conclusion, I must say that the preparation of annexes for release should be approached with great responsibility. Before using the program, the user goes through the installation of the product on the computer. The information that he receives at the same time, should make a favorable impression.