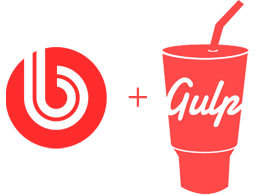
In this article I want to show my frontend development environment, configured under the cmx of Bitrix, using the
Gulp collector. This is primarily a set of tools under the hood of an excellent collector, which will save you from the routine and greatly accelerate the development.
Do not judge strictly
So fate happened that I ran into CMS 1C-Bitrix. I fully understand how cumbersome and “dirty” it is, but I have to deal with what is. Based on this, please do not strongly criticize the article, it is primarily aimed at those who work with this system.
Let's start
The first step is to set up a working environment, namely, to get the
npm package manager that comes with nodejs. Stop, stop young bitriksoidy, do not scatter, on windows it can also be put and through the console will also work, checked. And if you have any questions, google will help you (note the user environment variables, the paths must be correctly spelled for the npm command to be global).
')
Having established npm, we have an opportunity to establish all demanded dependences. To do this, create a file:
package.json
{ "name": "frontend-bitrix", "version": "1.0.0", "description": "", "author": "jmaks", "license": "ISC", "dependencies": { "gulp": "^3.8.11", "gulp-autoprefixer": "^2.1.0", "gulp-minify-css": "^1.0.0", "gulp-rigger": "^0.5.8", "gulp-sass": "^1.3.3", "gulp-uglify": "^1.1.0", "gulp-sourcemaps": "^1.5.0", "gulp-watch": "^4.1.1", "gulp-imagemin": "^2.2.1", "imagemin-pngquant": "^4.0.0", "gulp.spritesmith": "latest", "gulp-plumber": "latest" } }
This file contains a list of all required packages for our collector, consider it in detail:
- gulp - the collector himself
- gulp-autoprefixer - automatically adds vendor prefixes to CSS properties
- gulp-minify-css - compress css code
- gulp-rigger - allows you to import one file into another with a simple construction
- gulp-sass - to compile our SCSS code
- gulp-uglify - compress our js
- gulp-sourcemaps - to generate css sourscemaps that will help us in debugging code
- gulp-watch - monitor file changes
- gulp-imagemin - image compression
- imagemin-pngquant - image compression | working with PNG
- gulp.spritesmith - creating png sprites
- gulp-plumber - catch errors so watch is not interrupted
We also need such a great package manager like
Bower . First we install it using npm (the bower installation goes separately from our dependencies installation, since we need to install bower globally), and then we will similarly create a file with settings:
bower.json
{ "name": "frontend-bitrix", "version": "0.0.1", "authors": [ "jmaks1 <my.mailname@mail.ru>" ], "license": "MIT", "ignore": [ "**/.*", "node_modules", "bower_components", "test", "tests" ], "dependencies": { "jquery": "2.*", "bootstrap-sass": "*" } }
Everything is similar to package.json, there is a dependencies block that contains a list of downloadable packages. Here we will load jquery and bootstrap-sass.
As you have already noticed, I use a bunch of bootstrap and css sass preprocessor.
On the first point - bootstrap, I use it in view of the fact that 1C-Bitrix itself gradually begins to use this CSS framework (well, I’m just used to it).
On the second point - Sass. This preprocessor is more functional than less, the last one I really liked, but it could not compete with Sass.
Create a new project structure
Creating a new store / portal / “something else on Bitrix” I install the necessary Bitrix edition on the local server. During the installation, select any ready-made template from the start-up templates, and then just cancel the installation wizard so as not to clutter up the site with demo data, we end up with the following site structure:
auth
bitrix
upload
Now create partitions for the build and development files. Build is our site template, all files will be collected in it, it should be placed in the / local / directory. For those who are in the tank, this directory is equivalent to / bitix /, that is, we can create components, templates, and so on. The development will be carried out in the / src / directory, inside it we will create a simple structure.
As a result, we get:
auth
bitrix
upload
local
- templates
- - main
src
- fonts
- images
- sprite
- js
- - partials
- styles
- - partials
Everything is trivial:
The local directory contains the basic site template
main .
The src directory contains:
- fonts - fonts
- images - pictures
- sprite - icons for sprite
- js - scripts. At the root of this folder will be the file script.js, which is useful for us to build.
- styles - styles. There is also at the root of template_styles.scss.
In both cases, the files from the partials directory will be additional, we will simply include them in the main file, respectively, we will include all scripts in script.js, and all additional style files in template_styles.scss.
We will include the files with the
// = construction for script.js:
Scss files are connected using
@import :
// include bootstrap sass @import "../../bower_components/bootstrap-sass/assets/stylesheets/bootstrap"; // include variables @import "partials/variables"; // include sprite @import "partials/sprite"; // include mixin @import "partials/mixin"; // include fonts @import "partials/fonts";
After receiving the project structure, we will add our
bower.json and
package.json files to its root. It remains only to create a configuration file for our collector -
gulpfile.js . This file will be responsible for all the magic.
gulpfile.js
All dependencies that we have specified in the
package.json file are
included inside
gulpfile.js :
'use strict'; var gulp = require('gulp'), watch = require('gulp-watch'), prefixer = require('gulp-autoprefixer'), uglify = require('gulp-uglify'), rigger = require('gulp-rigger'), sass = require('gulp-sass'), sourcemaps = require('gulp-sourcemaps'), cssmin = require('gulp-minify-css'), imagemin = require('gulp-imagemin'), pngquant = require('imagemin-pngquant'), spritesmith = require('gulp.spritesmith'), plumber = require('gulp-plumber');
Create a path object with all the paths so that if necessary it is easy to edit them in one place. They will be used by our task (task - task, set tasks for our manager, and he quickly performs everything).
var path = { build: { js: 'local/templates/main/', css: 'local/templates/main/', images: 'local/templates/main/images/', fonts: 'local/templates/main/fonts/', fontsBootstrap:'local/templates/main/fonts/bootstrap/' }, src: { js: 'src/js/script.js', styles: 'src/styles/template_styles.scss', stylesPartials:'src/styles/partials/', spriteTemplate:'src/sass.template.mustache', images: 'src/images/**/*.*', sprite: 'src/sprite/*.*', fonts: 'src/fonts/**/*.*', fontsBootstrap:'bower_components/bootstrap-sass/assets/fonts/bootstrap/*.*' }, watch: { js: 'src/js/**/*.js', styles:'src/styles/**/*.scss', images:'src/images/**/*.*', sprite:'src/sprite/*.*', fonts: 'src/fonts/**/*.*' } };
By connecting all the dependencies and writing the routes, we will create taskkis that are responsible for all the work of our collector.
Js file collector gulp.task('js:build', function () { gulp.src(path.src.js)
Css file collector gulp.task('styles:build', function () { gulp.src(path.src.styles)
Task, responsible for the compression of all images gulp.task('image:build', function () { gulp.src(path.src.images)
Task Collecting Sprite gulp.task('sprite:build', function() { var spriteData = gulp.src(path.src.sprite) .pipe(spritesmith({ imgName: 'sprite.png', cssName: 'sprite.scss', cssFormat: 'scss', algorithm: 'binary-tree', padding: 20, cssTemplate: path.src.spriteTemplate, cssVarMap: function(sprite) { sprite.name = 's-' + sprite.name } })); spriteData.img.pipe(gulp.dest(path.build.images)); spriteData.css.pipe(gulp.dest(path.src.stylesPartials)); });
It takes all our icons from the sprite folder and generates a sprite, in parallel, based on the sass.template.mustache file, it creates a scss file in which all sizes and icon positions are written.
sass.template.mustache
{{#items}} ${{name}}: {{px.x}}, {{px.y}}, {{px.offset_x}}, {{px.offset_y}}, {{px.width}}, {{px.height}}, {{px.total_width}}, {{px.total_height}}, '{{{escaped_image}}}'; {{/items}}
Create the last two task'a that will launch everything in the work. The first is required to run, the second to monitor file changes so that we do not need to restart our manager all the time.
gulp.task('build', [ 'js:build', 'sprite:build', 'icons:build', 'fonts:build', 'styles:build', 'image:build', ]); gulp.task('watch', function(){ watch([path.watch.js], function(event, cb) { gulp.start('js:build'); }); watch([path.watch.sprite], function(event, cb) { gulp.start('sprite:build'); }); watch([path.watch.styles], function(event, cb) { gulp.start('styles:build'); }); watch([path.watch.fonts], function(event, cb) { gulp.start('fonts:build'); }); watch([path.watch.images], function(event, cb) { gulp.start('image:build'); }); }); gulp.task('default', ['build', 'watch']);
As a result, we get three configuration files in the project root, execute them by writing commands to the console:
- npm install - install all the dependencies required by gulp (the node_modules directory will be created)
- bower install - we will put plugins for our site (the bower_components directory will be created)
- gulp - we will start our collector
profit!
Total
After a successful launch, we got a comfortable development environment. Compressed versions of files, scripts, styles and images will be automatically generated in our template. Download the already customized version from
Github . It will be enough for you to unpack the archive into the directory with the new site and execute commands from the console.
Underwater rocks
Before creating a backup copy of the site, do not forget to put an exception on the directory: node_modules and bower_components, otherwise the backup copy will not be created. These folders are needed only for development, they will not be needed in the “combat” version of the site.
psThe article originates from the article “A
pleasant assembly of the frontend project ” - I recommend to read it.
If anyone is interested, I can add the generation of svg sprites to the collector.