Good morning, dear readers.
We will start the week with such an informative, albeit somewhat abstract translation, which will help us to clarify how interested the audience is in publishing books on ASP.NET. Practice has shown that Peter’s books,
Developing Applications Using ASP.NET and AJAX and
Programming Using Microsoft ASP.NET 4 , have not been in great demand. However, it is quite possible that, based on the results of this post, we still decide to aim a blow at ASP.NET 5 and MVC 6.
Updated on April 30, 2015.
In the first edition of this post, I wrote that Microsoft does not plan to support VB.NET in ASP.NET 5 / MVC 6. Indeed, at first it was, but my post caused a strong reaction in the VB.NET community. So, apparently, VB.NET again in the cage!
www.infoq.com/news/2015/04/VB-Core')
I spent the last couple of weeks writing sample code for ASP.NET 5 / MVC 6. I was just amazed at the depth of changes made to the ASP.NET 5 beta version that is currently relevant. ASP.NET 5 is the most significant new release in the history of the entire ASP.NET framework - we can say that the entire framework has been rewritten.
In this article I will focus on 10 changes in ASP.NET 5, which seem to me the most significant. The list is very subjective. If you would like to mention any other changes - describe them in the comments.
1. ASP.NET on OSX and Linux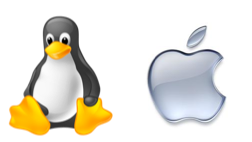
For the first time in the history of ASP.NET, ASP.NET 5 applications can run on OSX and Linux operating systems. Once again, ASP.NET 5 applications can run on Windows, OSX and Linux. This fact opens ASP.NET for many developers and designers who previously could not use this framework.
Traditional ASP.NET audience is professional programmers working in large corporations. Clients of such corporations are tightly chained to their computers with Windows.
The situation is completely different in startups, where it is customary to work with OSX / Linux. Whenever I get to a start-up conference, it turns out that the entire audience is working with a Macbook Pro. As a rule, these people do not work with ASP.NET.
In addition, client-side designers and developers — at a minimum, those who work outside of corporate dungeons — also love working with a Macbook. When I come to the jQuery conference, there is only one Macbook Pro everywhere (the following picture is taken from the jQuery theme blog).
Now that ASP.NET 5 runs on Windows, OSX and Linux, everything will change. For the first time, building applications using ASP.NET 5 is available for any developers and designers. And they can create in their favorite development environments, for example, in Sublime Text and WebStorm (Visual Studio is not required).
Check out the OmniSharp project and see how different text editors can be used with ASP.NET 5 — in particular, Sublime Text, Atom, Emacs, and Brackets:
www.omnisharp.net2. No more web formsI love ASP.NET web forms. In my entire life, I have spent hundreds — or even thousands — of hours programming applications with Web Forms. However, now it is time to say goodbye to them. There will be no more web forms in ASP.NET 5.
Applications with Web Forms can be further programmed in Visual Studio 2015, setting the target platform as .NET 4.6 framework. However, applications with web forms do not allow working with any of the new cool features of ASP.NET 5 described in this list. If you do not want to stay on the sidelines of historical progress, now is the time to finally rewrite your application from Web Forms to ASP.NET MVC.
3. Tag HelpersTag Helpers (helpers) - probably the same opportunity that can most strongly affect the views that you create in an ASP.NET MVC application. Tag Helpers is a more convenient alternative than traditional MVC helpers.
Consider the following MVC view, which contains a form for creating a new product:
@model MyProject.Models.Product @using (Html.BeginForm()) { <div> @Html.LabelFor(m => p.Name, "Name:") @Html.TextBoxFor(m => p.Name) </div> <input type="submit" value="Create" /> }
In the above example, the Html.BeginForm () Html.LabelFor () and Html.TextBoxFor () helpers are used to create the form. These helpers hardly met an HTML designer.
Here is how exactly the same form is created using Tag Helpers:
@model MyProject.Models.Product @addtaghelper "Microsoft.AspNet.Mvc.TagHelpers" <form asp-controller="Products" asp-action="Create" method="post"> <div> <label asp-for="Name">Name:</label> <input asp-for="Name" /> </div> <input type="submit" value="Save" /> </form>
Please note: this updated version of the form contains only HTML elements (at least, it seems so at first glance). For example, the form has an INPUT element, not an Html.TextBoxFor () helper. The client side designer would have liked this page. All the intricacies of this view are related to its asp-for attributes. These attributes are used to complement the elements of the ASP.NET MVC server functionality.
In the following article, Damien Edwards demonstrates a whole site where nothing but Tag Helpers is used:
github.com/DamianEdwards/TagHelperStarterWeb4. Components of viewsGoodbye subcontrollers, long live the components of the views (View Components)!
In previous versions of ASP.NET MVC, we used the Html.Action () helper to call the subcontroller. Suppose you want to display an advertising banner in several views at once. In this case, it would be necessary to create a subcontroller that would contain the logic for returning a particular banner, and the subcontroller would work as a result of calling the Html.Action () helper from the view.
The subcontrollers — the Html.Action () helper — are not present in the current MVC 6 beta. Instead, MVC 6 uses an alternative technology called View Components (view components).
Here's how to create a presentation component that displays one of two advertising banners depending on the time of day:
using Microsoft.AspNet.Mvc; using System; namespace Partials.Components { public class BannerAd : ViewComponent { public IViewComponentResult Invoke() { var adText = "Buy more coffee!"; if (DateTime.Now.Hour > 18) { adText = "Buy more warm milk!"; } return View("_Advertisement", adText); } } }
In the period up to 17.00, the submission component returns a partial template called _Advertising with the advertising text “Buy more coffee!”. In the period after 5 pm this text is changed to “Buy more warm milk!”.
Here is what the partial template for the _Address is:
@model string <div style="border:2px solid green;padding:15px"> @Model </div>
Finally, here’s how you can use the BannerAd view component in the MVC view:
@Component.Invoke("BannerAd")
The components of views are very similar to subcontrollers. However, subcontrollers have always been weird. They were supposed to work as action controllers, but in practice they were not. Components of representations look much more natural.
5. GruntJS, NPM and Bower Support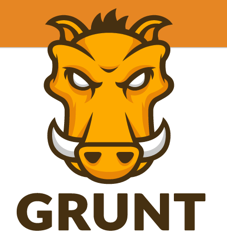
Client-side developers will sincerely love ASP.NET 5 due to the fact that GruntJS (and, ultimately, Gulp) is supported in this framework.
GruntJS is a task manager that allows you to collect resources for the client side, for example, JavaScript and CSS files. For example, GruntJS can be used to concatenate and shrink JavaScript files when executing each build in Visual Studio.
There are thousands of GruntJS plug-ins that allow you to solve an unimaginable number of tasks (currently there are 4,334 such modules in the GruntJS plug-in repository):
gruntjs.com/pluginsFor example, here you will find plugins for running JavaScript unit tests, validating the quality of JavaScript code (jshint), compiling LESS and Sass files in CSS, compiling TypeScript in JavaScript, and reducing images.
To support Microsoft GruntJS, it was necessary to support two new package managers (other than NuGet). First, since the GruntJS plugins are distributed as NPM packages, Microsoft added support for NPM packages.
Second, since many client resources, such as Twitter Bootstrap, jQuery, Polymer, and AngularJS, are distributed through Bower, Microsoft has added support for Bower.
Thus, you can run GruntJS using plugins from NPM, and client resources from Bower.
6. Unified MVC and Web API controllersIn previous versions of ASP.NET MVC, MVC controllers were different from Web API controllers. The MVC controller used the base class System.Web.MVC.Controller, and the Web API controller used the base class System.Web.Http.ApiController.
In MVC 6, there is only one Controller class, which is the base class for all MVC and Web API controllers. This is a class of Microsoft.AspNet.Mvc.Controller.
MVC 6 controllers return IActionResult interfaces. When used as an MVC controller, an IActionResult can be a representation. In the case of a Web API controller, an IActionResult can be data (for example, a list of products). The same controller can have actions that return both views and data.
In MVC 6, both MVC controllers and Web API controllers use the same routes. You can use either routes that operate under the agreements, or routes based on attributes. They apply to all controllers in a project.
7. AngularJSAngularJS is one of the most popular client frameworks for creating single-page applications (SPA). In Visual Studio 2015, there are templates for creating AngularJS modules, controllers, directives and factories.
Due to the support of GruntJS in ASP.NET 5, ASP.NET turns out to be an excellent server framework for building AngularJS client applications. You can automatically combine and shrink all of your AngularJS files during each build. You can interact with the MVC 6 controller from $ resource AngularJS using REST.
8. Framework for dependency injection in ASP.NETASP.NET 5 has built-in support for dependency injection and the service locator pattern. Thus, you no longer depend on third-party frameworks for dependency injection, for example, from Ninject or AutoFac.
Suppose you have created an IRepository interface and an EFRepository class that implements this interface. In this case, you can bind the EFRepository class to the IRepository interface using the ConfigureServices () method of the Startup.cs class like this:
services.AddTransient<IRepository, EFRepository>();
By linking EFRepository and IRepository, you can inject a constructor dependency into your MVC controllers (and any other class) with the help of similar code:
public class ProductsController : Controller { private IRepository _repo; public ProductsController(IRepository repo) { _repo = repo; } }
In the above code, the IRepository interface is passed to the constructor for the ProductsController. This built-in framework for dependency injection in ASP.NET passes the EFRepository to the ProductsController, since the IRepository interface was associated with the EFRepository.
In addition, you can use the “Service Locator” pattern. Whenever it is possible to access the HttpContext, you can access any registered services. For example, you can extract the EFRepository by applying the following code instead of the MVC action controller:
var repo = this.Context.ApplicationServices.GetRequiredService<IRepository>();
9. xUnit.netFarewell to Visual Studio Unit Testing Framework, long live xUnit.net!
In previous versions of ASP.NET MVC, the Visual Studio Unit Testing Framework (sometimes called mstest) was used by default for testing. To describe a unit test, it uses the attributes [TestClass] and [TestMethod]:
[TestClass] public class CalculatorTests { [TestMethod] public void TestAddNumbers() {
In ASP.NET 5, the xUnit.net framework is used for unit testing. In it, instead of [TestMethod], the [Fact] attribute is used, and the [TestClass] attribute is missing:
public class CalculatorTests { [Fact] public void AddNumbers() {
After working with the source code ASP.NET 5, you will see that xUnit.net is used for extensive ASP.NET testing. For example, in the MVC repository there are unit tests written using xUnit.net. The MVC repository (and its unit tests) can be found here:
github.com/aspnet/mvcASP.NET uses the xUnit.net branch located here:
github.com/aspnet/xunit