It is no secret that they like to ask tricky questions at interviews. Not always adequate, not always relevant to reality, but the fact remains that they are asking. Of course, a question is a question, and sometimes a question, seemingly stupid at first glance, is actually aimed at checking how well you know the language you write.
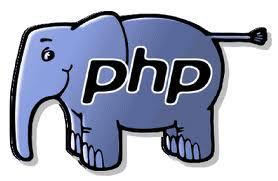
Let's try to sort out “by the bones” one of these questions -
what does the word “static” mean in PHP and why does it apply?The static keyword has three different meanings in PHP. We analyze them in chronological order, as they appeared in the language.
')
The first value is a static local variable.
function foo() { $a = 0; echo $a; $a = $a + 1; } foo();
In PHP, variables are local. This means that the variable defined and received within the function (method) exists only during the execution of this function (method). When you exit the method, the local variable is destroyed, and when you re-enter, it is created anew. In the code above, such a local variable is the $ a variable - it exists only inside the foo () function and is created anew each time this function is called. The increment of a variable in this code is meaningless, since on the very next line of code the function ends its work and the value of the variable will be lost. No matter how many times we call the foo () function, it will always output 0 ...
However, everything changes if we assign the static keyword to the assignment:
function foo() { static $a = 0; echo $a; $a = $a + 1; } foo();
The static keyword written before assigning a value to a local variable results in the following effects:
- Assignment is performed only once, when the function is first called
- The value of a variable marked in this way is saved after the function ends.
- On subsequent function calls, instead of assignment, the variable gets the previously saved value.
This use of the word static is called a
static local variable .
Pitfalls of static variables
Of course, as always in PHP, it does not do without “pitfalls”.
The first stone is that you can only assign constants or constant expressions to a static variable. Here is the code:
static $a = bar();
will inevitably lead to parser error. Fortunately, starting from version 5.6, it became possible to assign not only constants, but also constant expressions (for example, “1 + 2” or “[1, 2, 3]”), that is, such expressions that are independent of other code and can be calculated at compile time
Stone two - the methods exist in a single copy.It's all a little more complicated. To understand the essence of the code below:
class A { public function foo() { static $x = 0; echo ++$x; } } $a1 = new A; $a2 = new A; $a1->foo();
Contrary to the intuitive expectation of “different objects - different methods”, we clearly see in this example that dynamic methods in PHP “do not multiply”. Even if we have a hundred objects of this class, the method will exist only in one instance, just at each call it will be forwarded to a different $ this.
Such behavior may be unexpected for an unprepared developer and cause errors. It should be noted that the inheritance of the class (and method) leads to the fact that after all a new method is created:
class A { public function foo() { static $x = 0; echo ++$x; } } class B extends A { } $a1 = new A; $b1 = new B; $a1->foo();
Conclusion: Dynamic methods in PHP exist in the context of classes, not objects. And only in runtime there is a substitution "$ this = current_object"Second value - static properties and methods of classes
In the PHP object model, it is possible to set properties and methods not only for objects — instances of a class, but also for a class as a whole. The static keyword is also used for this:
class A { public static $x = 'foo'; public static function test() { return 42; } } echo A::$x;
To access such properties and methods, double-colon constructions (“Paamayim Nekudotayim”) are used, such as CLASS_NAME :: $Variable name and CLASS_NAME :: Method_name ().
It goes without saying that static properties and static methods have their own characteristics and their own “pitfalls” that you need to know.
Feature one, banal - no $ this. Actually, this stems from the very definition of a static method — since it is associated with a class, and not an object, the pseudo-variable $ this is not available in it, indicating the current object in dynamic methods. Which is completely logical.
However, you need to know that, unlike other languages, PHP does not define the situation “in the static method $ $ is written” at the stage of parsing or compilation. Such an error can occur only in runtime if you try to execute code with $ this inside a static method.
A code like this:
class A { public $id = 42; static public function foo() { echo $this->id; } }
will not lead to any errors unless you try to use the foo () method in an inappropriate way:
$a = new A; $a->foo();
(and immediately get "Fatal error: Using $ this when not in object context")
The second feature - static is not an axiom! class A { static public function foo() { echo 42; } } $a = new A; $a->foo();
So, yes. A static method, if it does not contain in the code of $ this, it is quite possible to call it in a dynamic context, as a method of an object. This is not a PHP error.
The reverse is not quite true:
class A { public function foo() { echo 42; } } A::foo();
A dynamic method that does not use $ this can be executed in a static context. However, you will receive the “Non-static method A :: foo () should not be called statically” warning of the E_STRICT level. Here you decide - or strictly follow the standards of the code, or suppress warnings. The first, of course, is preferable.
And by the way, everything written above applies only to methods. Using a static property through "->" is impossible and leads to a fatal error.
The third meaning seems to be the most difficult - late static binding
The developers of the PHP language did not stop at the two meanings of the “static” keyword and in version 5.3 added another “feature” of the language, which is implemented with the same word! It is called “late static binding” or LSB (Late Static Binding).
The simplest example is to understand the essence of LSB:
class Model { public static $table = 'table'; public static function getTable() { return self::$table; } } echo Model::getTable();
The self keyword in PHP always means "the name of the class where the word is written." In this case, self is replaced with the Model class, and self :: $ table with Model :: $ table.
This language feature is called “early static linking”. Why early? Because the binding of self and a specific class name occurs not in runtime, but at earlier stages - parsing and compiling code. Well, "static" - because we are talking about static properties and methods.
Let's change our code a bit:
class Model { public static $table = 'table'; public static function getTable() { return self::$table; } } class User extends Model { public static $table = 'users'; } echo User::getTable();
Now you understand why PHP behaves unintuitively in this situation. self was associated with the Model class when nothing was known about the User class, and therefore points to Model.
How to be?
To solve this dilemma, the mechanism of tying the “late” at the runtime stage was invented. It works very simply - it is enough to write “static” instead of the word “self” and the connection will be established with the class that calls this code, and not with where it is written:
class Model { public static $table = 'table'; public static function getTable() { return static::$table; } } class User extends Model { public static $table = 'users'; } echo User::getTable();
This is the mysterious "later static binding".
It should be noted that for more convenience in PHP, in addition to the word "static", there is also a special function get_called_class (), which will inform you in the context of which class your code is currently running.
Successful interviews!The list of useful links to the manual: