As a translator, I apologize in advance for any errors in the translation. I would appreciate error messages for their prompt correction.When iOS 7 was first announced, one of the many visual innovations that particularly interested me was the swipe-to-delete gesture in the Mail app. You have long been perfectly familiar with this feature of the application. After you navigate the table cell, you see the basket buttons, additional options (“More”) and mark the letter with a flag. When you click on the “More” button, the action selection panel becomes active, where you can choose one of the letter action options - Reply, Mark with flag, etc.
I think this is a great tool for manipulating table entries. However, as you are well aware that Apple does not provide this opportunity for developers in iOS 7. You can only add a gesture to the function of deleting a record from the table. The remaining options for working with a table entry are limited to the standard Mail application. I have no idea why Apple restricts the use of such a wonderful tool only to its applications. Fortunately, some developers have created solutions (such as
UITableView-Swipe-for-Options ,
MCSwipeTableViewCell ) and made them completely free.
')
In this tutorial, I will use the
SWTableViewCell library. Let's see how it helps us to implement the function swipe-to-show-options (swipe to see options) in your application. SWTableViewCell is fairly easy to use, and if you understand how UITableView works, you should have no problems with SWTableViewCell. In addition, this component supports the display of option buttons when svaype in both directions. You will quickly understand what this means.
So let's get started and create our demo application.
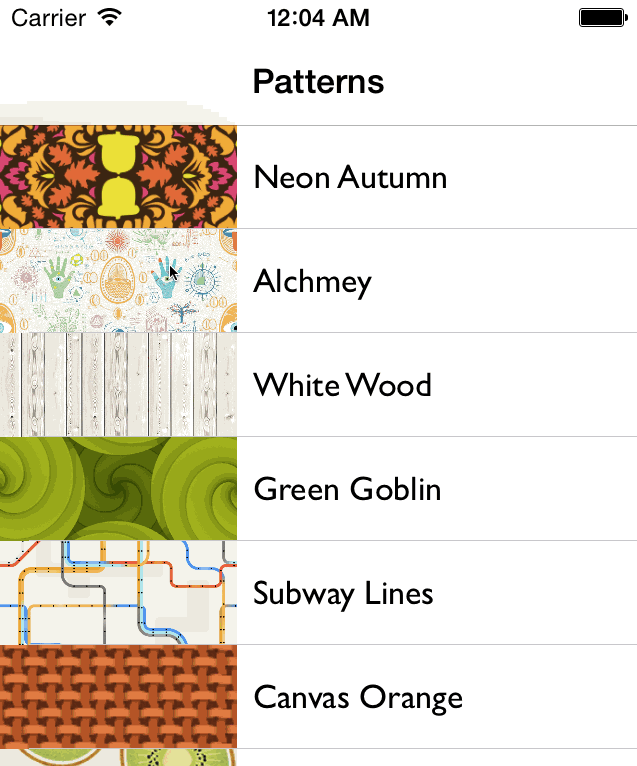
Xcode project template for the demo application
I highly recommend that you create a demo application project from scratch. However, in order to focus exclusively on using the SWTableViewCell library, I have prepared a project template for you. You can
download it here . If you know how to create a custom table view, you need to understand how this template works. After you download it, you can try to compile and run the project. The application should display a list of image patterns (courtesy of
thepatternlibrary.com ).
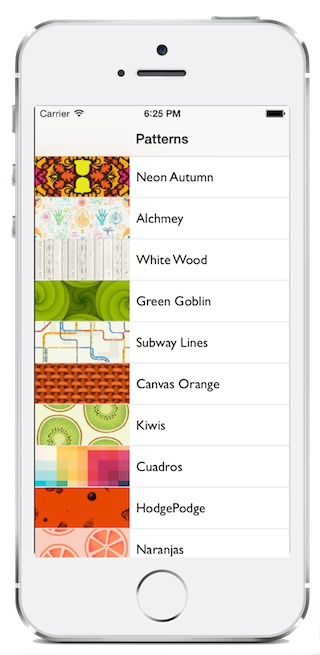
Adding SWTableViewCell library to our project
SWTableViewCell is developed by Chris Wendel and is available for free on GitHub. To use it, first download it from GitHub, unpack the archive and add the unpacked file to the SWTableViewCell or PodFiles group in your project.
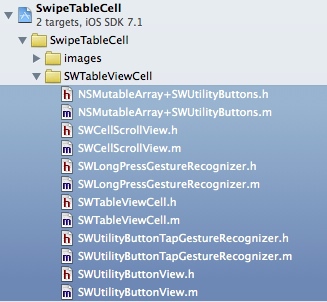
Using SWTableViewCell
The SWTableViewCell library is very simple to use. If you are familiar with UITableView and UITableViewCell, you should not have difficulty using it. Here is what you should do:
1. Choose as a class that will serve the cells of our SWTableViewCell table instead of UITableViewCell. SWTableViewCell is actually inherited from UITableViewCell, providing additional tools for displaying the option buttons when swiping around a table cell.
2. Modify the
cellForRowAtIndexPath method
: to create new buttons - SWTableViewCell provides two properties with the names
leftUtilityButtons and
rightUtilityButtons . These two properties provide a set of option buttons that are shown when swiping left and right, respectively. You must first create an array of option buttons and assign them all the necessary properties. It is not necessary to assign sets of buttons at once to both the
leftUtilityButtons and
rightUtilityButtons properties .
3. Implement the SWTableViewCellDelegate protocol. Methods of this protocol allow developers to control the actions of additional buttons. When the user makes a swipe to a table cell, this cell will show a set of option buttons. Depending on the direction of the svayp, when you click on the option button, one of the
didTriggerRightUtilityButtonWithIndex: or
didTriggerLeftUtilityButtonWithIndex: methods will be called. You must implement these methods to respond to button presses.
So let's change the application template to use SWTableViewCell.
Changing the class of the serving Table View Cell to SWTableViewCell
First, open the CustomTableViewCell.h file and change the parent class to SWTableViewCell. Your code should look something like this after making changes:
#import <UIKit/UIKit.h> #import "SWTableViewCell.h" @interface CustomTableViewCell : SWTableViewCell @property (weak, nonatomic) IBOutlet UIImageView *patternImageView; @property (weak, nonatomic) IBOutlet UILabel *patternLabel; @end
Creating option buttons
Now we will change the
cellForRowAtIndexPath: method in the
SwipeTableViewController.m file to create cell option buttons. But before we do this, add the import statement to the library and specify the implement SWTableViewCellDelegate protocol in the SwipeTableViewController.h file. We will talk about protocol implementation a bit later, in the next section, to begin with, let's make changes to the code. After making changes, it should look something like this:
#import <UIKit/UIKit.h> #import "SWTableViewCell.h" @interface SwipeTableViewController : UITableViewController <SWTableViewCellDelegate> @end
Open the SwipeTableViewController.m file and make changes to the
cellForRowAtIndexPath method
: - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString *cellIdentifier = @"Cell"; CustomTableViewCell *cell = (CustomTableViewCell *)[tableView dequeueReusableCellWithIdentifier:cellIdentifier forIndexPath:indexPath];
In this example, we have created two sets of option buttons. One of them will be shown when swipe left, the other when swipe right. We created a set of buttons for sharing and placed it in the leftUtilityButtons variable, and also we created the More and Delete buttons and placed them in the rightUtilityButtons variable. As you can see from the code above, you can simply create an array of NSMutableArray and its elements add the necessary buttons to it using the
sw_addUtilityButtonWithColor: method.
Now you are ready to compile and run the test application. After launch, try to navigate to any cell in the table and you should see the cell option buttons. However, the buttons do not work and we know why. We have not implemented some of the SWTableViewCellDelegate protocol methods. Well, let's move on.
SWTableViewCellDelegate protocol implementation
The SWTableViewCellDelegate protocol provides methods for controlling the actions of pressing the option buttons. The attentive reader, of course, noticed that SwipeTableViewController is designated as the SWTableViewCell delegate in the code above. Well, let's implement the following two methods in SwipeTableViewController.m:
1. The
didTriggerLeftUtilityButtonWithIndex method
: - triggered when any of the option buttons on the left side of the cell is pressed
2.
didTriggerRightUtilityButtonWithIndex method
: - it works when any of the option buttons on the right side of the cell is pressed
Add the following code to SwipeTableViewController.m:
- (void)swipeableTableViewCell:(SWTableViewCell *)cell didTriggerLeftUtilityButtonWithIndex:(NSInteger)index { switch (index) { case 0: { UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Bookmark" message:@"Save to favorites successfully" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil]; [alertView show]; break; } case 1: { UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Email sent" message:@"Just sent the image to your INBOX" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil]; [alertView show]; break; } case 2: { UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Facebook Sharing" message:@"Just shared the pattern image on Facebook" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil]; [alertView show]; break; } case 3: { UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Twitter Sharing" message:@"Just shared the pattern image on Twitter" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles: nil]; [alertView show]; } default: break; } }
To preserve the simplicity of our example, we simply show an alert when any of the sharing buttons is pressed. In a real application, you can implement real sharing on Facebook and Twitter, for example.
For the option buttons on the right side of the cell, we implemented the
didTriggerRightUtilityButtonWithIndex: method with the following code:
- (void)swipeableTableViewCell:(SWTableViewCell *)cell didTriggerRightUtilityButtonWithIndex:(NSInteger)index { switch (index) { case 0: {
Again, the code is simple and straightforward. When the “More” button is pressed (Advanced), the application shows an action sheet (action sheet) containing a list of social networks for sharing. For the “delete” option, we just need to remove the element from the list of templates and the patternImages array, and then call the
deleteRowsAtIndexPaths: method to delete the record from the displayed table.
That's all! Now you can compile and run your application. The option buttons should work something like this:

Let's sum up
SWTableViewCell makes it very easy to implement the swipe-for-options function for tables. When developing your next application, consider implementing this feature. I think it will raise the level of your application for the user.
And although we can implement this functionality with the help of many third-party libraries or
implement it on our own , I hope that Apple will consider the possibility of providing this tool to developers in future versions of iOS.
You can
download the finished project , if you suddenly need it.