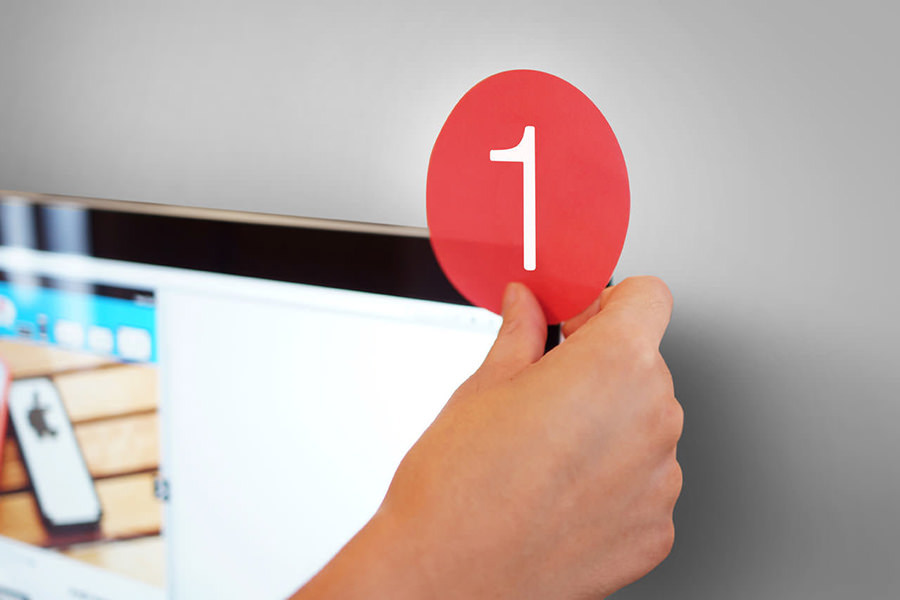
Websites and web applications with constantly updated content should notify about updates in the event that the user left the tab but did not close it.
There are a couple of the most common ways to alert the user. On the one hand, the social networks Facebook, Twitter and LinkedIn indicate a certain number that corresponds to the number of updates. On the other hand, Trello displays a small red circle on top of the favicon.
')
Trello, Facebook and Twitter AlertsIn this lesson, we will recreate such alerts. Check out the
demo to understand what we are going to do.
View on githubDemo (Russian)Use the page title
We will start by adding the number of updates to the page title, as is done on Facebook and Twitter.
There are many ways to extract data (ask a friend of the developer). In our case, we assume that we already know the number of updates and now we can play with JavaScript. At the moment we have zero updates:
var count = 0;
The following is the main part of the code that will change the name of the page. First of all, let's add
document.title,
which gets the current page title:
var title = document.title;
Then we create a new function that will change part of the name:
function changeTitle() { count++; var newTitle = '(' + count + ') ' + title; document.title = newTitle; }
Here you can see that we are using a javascript
++
operator. For demonstration purposes, "
++"
will increase the number of
count
by
1.
Now create a new function that will launch
changeTitle()
:
function newUpdate() { update = setInterval(changeTitle, 2000); } var docBody = document.getElementById('site-body'); docBody.onload = newUpdate;
The function above will check for updates every
2000
milliseconds (2 seconds). The function will start as soon as the page loads.

The first method is fairly straightforward. We get the number of updates and transfer it to the title. You can change the brackets from
( )
to
[ ]
or
{ }
in the
changeTitle()
function.
Use favicon
Now we use the second approach, which changes the
favicon , the Trello web application. For this we need to prepare two options favicon. The first is when there are no updates, the second is when updates have appeared.

We start by binding the favicon to the document.
<link id="favicon" rel="icon" href="img/favicon.gif?v3"/>
Then specify the path to the new favicon using the JavaScript variable.
var iconNew = 'img/favicon-dot.gif';
As in the first method, we create two functions:
function changeFavicon() { document.getElementById('favicon').href = iconNew; } function newUpdate() { update = setInterval(changeFavicon, 3000); setTimeout(function() { clearInterval( update ); }, 3100); } var docBody = document.getElementById('site-body'); docBody.onload = newUpdate;
The first function,
changeFavicon()
, will replace our initial favicon with the one with the red circle. The second function,
newUpdate()
, will execute the first function after a certain period of time.
It seems to have something to look at!The second method is not as complicated as it seems at first glance - on the contrary, it is simpler than the first, where we updated the page name. In addition, we can show some creativity by adding animations to the favicon. But without much fanaticism.
Note : Chrome does not support animated favicon.
Using Favico.js
So, the last way is to use the JavaScript library of
Favico.js , authored by
Miroslav Magda . The library provides a convenient API with many different options.
We begin with specifying the parameters of the new
Favico
: position, animation, background color, text color.
var favicon = new Favico({ position :'up', animation :'popFade', bgColor :'#dd2c00', textColor :'#fff0e2' });
Then add a couple of functions that will start the whole process and ultimately display the badge on favicon.
var num = 0; function generateNum() { num++; return num; } function showFaviconBadge() { var num = generateNum(); favicon.badge(num); } function newUpdate() { update = setInterval(showFaviconBadge, 2000); } var docBody = document.getElementById('site-body'); docBody.onload = newUpdate;
To some extent, our code resembles the first method. We start by creating a function that will
simulate the number of updates. The second function,
showFaviconBadge()
, will insert this number into the badge and display the badge itself in favicon. The last function
newUpdate()
will start the second one at a certain interval.
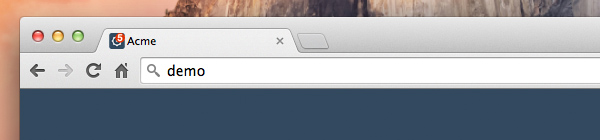
Conclusion
In this lesson, we learned to use the name of the browser tab as a means of notifying users. We recreated the techniques that are used on popular websites such as Facebook, Twitter, and Trello.
Again, you will likely have to make a couple of changes to meet the needs of your project. But the examples provided will serve as a great start!
From the translator . With all the wishes and comments about the translation, please contact me in PM. Thank!